In today’s fast-paced development landscape, effective version controlling is no longer a luxury, it’s a necessity. Version control systems (VCS) like Git have revolutionized how developers collaborate, track changes, and revert to previous states of a project. Among its many features, the ability to navigate between commits is a critical tool that can save hours of debugging and restore stability to a project. In this article, we’ll explore everything you need to know about version controlling and how to manage commits effectively.
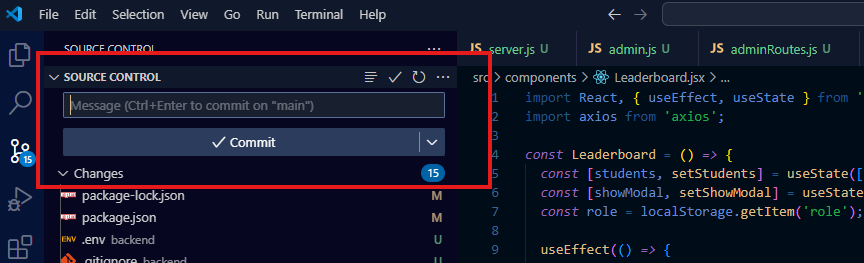
TL;DR
Version control systems like Git allow developers to track changes, collaborate, and revert to previous states of a project. Navigating between commits is essential for debugging, restoring stability, and testing changes. Key commands include git log
, git checkout
, git revert
, and git reset
. Following best practices like clear commit messages, frequent commits, and proper branching ensures efficient version control. The FAQs at the end address common concerns and techniques for navigating commits effectively.
What is Version Controlling?
Version controlling refers to the practice of managing changes to a codebase over time. It allows developers to track modifications, collaborate with team members, and maintain a history of changes that can be referenced or reverted to at any point.
The key benefits of version controlling include:
- Change Tracking: Every modification is logged, making it easy to understand what was changed and why.
- Collaboration: Teams can work on the same project without overwriting each other’s work.
- Recovery: Easily undo mistakes by reverting to a previous commit.
- Branching and Merging: Experiment with new features without affecting the main codebase.
Understanding Commits
In version control, a commit is a snapshot of your code at a specific point in time. Think of it as saving a file but instead of overwriting the previous version, you’re creating a new one that exists alongside the old.
Key attributes of a commit include:
- Unique Hash: A unique identifier (SHA-1 hash) that makes each commit traceable.
- Commit Message: A short description of the changes made.
- Parent Commit: A reference to the commit(s) that came before it.
- Tree Structure: A representation of your project’s file hierarchy at the time of the commit.
Why Navigate Between Commits?
Navigating back and forth between commits is essential for:
- Debugging Issues: Isolating the commit that introduced a bug.
- Restoring Stability: Reverting to a previous, functional state of the project.
- Code Reviews: Analyzing changes between commits to ensure quality.
- Experimentation: Testing new features without affecting stable versions.
By understanding how to efficiently navigate commits, you’ll unlock the full potential of your version control system.
How to Move Between Commits in Git
Viewing Commit History
Before navigating commits, it’s important to understand the history of your repository. Use the following command:
git log
This displays a chronological list of commits, including their hash, author, date, and commit message.
For a more concise view:
git log --oneline
Checking Out a Commit
To switch your working directory to a specific commit, use:
git checkout <commit-hash>
This puts your repository in a “detached HEAD” state, meaning you’re not on a branch but directly on that commit. While in this state, avoid making changes unless you plan to create a new branch.
Going Back to the Latest Commit
After inspecting an older commit, you can return to the latest commit on your branch:
git checkout <branch-name>
Reverting Changes
To undo the changes introduced by a specific commit:
git revert <commit-hash>
This creates a new commit that negates the changes from the specified commit, preserving the history.
Resetting to a Previous Commit
To completely reset your branch to a previous commit:
Soft Reset: Keeps changes in the staging area.
git reset --soft <commit-hash>
Mixed Reset: Keeps changes in the working directory but unstages them.
git reset --mixed <commit-hash>
Hard Reset: Discards all changes.
git reset --hard <commit-hash>
Rebasing for Cleanup
To rewrite commit history:
git rebase -i <commit-hash>
This allows you to squash, edit, or reorder commits.
Best Practices for Version Controlling
- Write Clear Commit Messages
- Use short yet descriptive messages. For example:
Fix login bug
orAdd user authentication feature.
- Use short yet descriptive messages. For example:
- Commit Frequently
- Commit your changes in logical increments. Avoid making a single large commit for multiple changes.
- Use Branches
- Create separate branches for features, bug fixes, and experiments to keep your
main
branch clean.
- Create separate branches for features, bug fixes, and experiments to keep your
- Regularly Pull and Push
- Sync your changes with the remote repository to avoid conflicts.
- Avoid Force Pushing
- Unless absolutely necessary, avoid using
git push --force
, as it can overwrite other developers’ work.
- Unless absolutely necessary, avoid using
- Tag Important Commits
- Use tags to mark significant points in your project, such as releases.
git tag -a v1.0 -m "Initial release"
git push origin v1.0
FAQs
What is the difference between git revert
and git reset
?
git revert
creates a new commit that undoes the changes of a specific commit, preserving the history.git reset
moves the HEAD to a previous commit, with the option to keep or discard changes.
What is a “detached HEAD” state?
- A detached HEAD state occurs when you check out a specific commit instead of a branch. While in this state, you can view or test the code at that commit but should avoid making changes unless creating a new branch.
How can I find the commit that introduced a bug?
- Use Git’s
bisect
feature to perform a binary search for the problematic commit:
git bisect start