Solidity is the primary programming language for Ethereum smart contracts. While it offers a high-level, developer-friendly syntax, it also provides access to low-level assembly, which allows developers to optimize contracts for performance and gas efficiency. Assembly in Solidity, often referred to as “inline assembly,” gives developers more control over the Ethereum Virtual Machine (EVM) by directly manipulating the low-level operations. While using assembly can enhance contract efficiency, it requires careful handling to avoid security risks and inefficiencies.
In this article, we’ll explore how Solidity assembly works, why it’s used, common operations, and optimization strategies, along with practical use cases and best practices for developers looking to enhance their smart contracts.
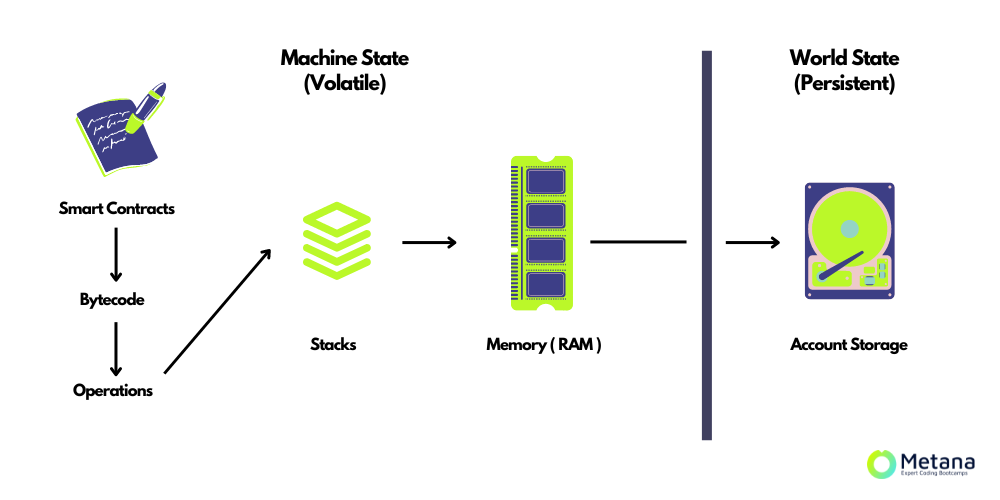
What Is Solidity Assembly?
Solidity assembly refers to low-level, EVM-specific instructions that developers can write directly in their contracts. It’s an alternative to Solidity’s high-level constructs and provides more fine-grained control over how code interacts with the EVM.
The inline assembly syntax in Solidity is accessed using the assembly
keyword. The language used within this context is based on the EVM instruction set, enabling the direct manipulation of variables, storage, and memory. While Solidity’s high-level code is already compiled to these low-level instructions, developers can use assembly to optimize certain operations that might not be as efficient when written in high-level code.
Example of Inline Assembly in Solidity:
function add(uint256 a, uint256 b) public pure returns (uint256) {
assembly {
let result := add(a, b)
mstore(0x80, result)
return(0x80, 32)
}
}
In this example, we are directly using the add
opcode, bypassing Solidity’s built-in addition method.
Why Use Solidity Assembly?
While Solidity offers an abstraction over the EVM, there are cases where developers need to use assembly for better control, particularly when it comes to optimizing for gas costs and performance. Some common reasons for using assembly include:
- Gas Optimization: Low-level operations in the EVM can sometimes be more gas-efficient than Solidity’s high-level equivalents. By writing certain operations in assembly, developers can reduce the gas costs of executing their contracts.
- Custom Logic: Certain custom behaviors or complex operations may require low-level access to the EVM, which is only possible through assembly.
- Performance Improvements: By using assembly, developers can optimize loops, conditional checks, and mathematical operations for performance.
- Handling Raw Data: Assembly is often used to manage low-level data types, such as manipulating raw bytes or encoding and decoding function inputs and outputs.
However, using assembly comes with a trade-off. It increases the complexity of the contract, making it harder to read and maintain, and it can introduce vulnerabilities if not used correctly.
Common Low-Level Operations in Solidity Assembly
When writing assembly, developers work with raw EVM instructions known as opcodes. Some of the most common low-level operations include:
- Memory Manipulation: Using assembly, developers can directly manage memory using commands like
mstore
,mload
, andmsize
. Memory is where temporary data is stored during the execution of a contract. Example:assembly { let x := mload(0x40) // Load memory from a specific location mstore(x, 42) // Store value 42 in memory }
- Storage Manipulation: Storage in the EVM is more permanent but also more expensive to use. In assembly, the
sstore
andsload
operations are used to manage storage. Example:assembly { sstore(0x0, 100) // Store 100 at storage location 0 }
- Arithmetic and Logic Operations: Low-level mathematical operations, such as
add
,mul
, andsub
, can be directly accessed in assembly. Example:assembly { let sum := add(10, 20) // Add 10 and 20 }
- Conditional Jumps: Control flow can be managed using conditional jumps (
jump
andjumpi
) and labels in assembly, allowing for more complex logic. Example:assembly { let x := 1 let y := 2 jumpi(equal, eq(x, y)) x := add(x, y) equal: }
Optimization Techniques Using Assembly
The primary motivation for using Solidity assembly is to optimize gas costs and improve performance. Here are some key optimization techniques:
- Reduce Expensive Operations: Solidity’s high-level functions often incur significant gas costs due to the abstractions involved. For instance, operations involving storage access (e.g., reading or writing to state variables) are expensive. Using assembly to batch memory operations before writing to storage can save gas.
- Inlined Function Logic: Instead of relying on Solidity’s function call mechanics, which include overhead, developers can use assembly to implement the logic directly in the contract’s bytecode. This avoids the function call stack and reduces gas.
- Avoid Redundant Memory Allocation: Efficient memory usage can save gas. By avoiding redundant memory allocations and reusing memory slots in assembly, developers can optimize gas costs.
- Avoid Using Solidity’s Built-In Types: Solidity types such as
struct
andarray
come with additional overhead for management. In assembly, developers can bypass these abstractions and handle data directly in memory and storage, saving gas. - Optimizing Loops: In certain scenarios, writing loop logic in assembly can reduce the number of gas-consuming instructions compared to Solidity’s native loop constructs.
Use Cases for Solidity Assembly
Assembly is generally used for advanced contract optimization or when direct EVM interaction is necessary. Here are some common use cases:
- Optimizing Gas-Intensive Contracts: In contracts with frequent interactions, such as DeFi protocols or NFT platforms, even small gas optimizations can lead to significant savings over time.
- Custom Cryptographic Functions: Cryptographic operations, such as signature verification, often require direct access to the EVM’s cryptographic functions. Writing these in assembly can significantly improve performance.
- Token Standards and Contract Proxies: Assembly is often used in the development of token standards like ERC-20 or proxy contracts (such as those used in upgradeable contracts) to manipulate function selectors and delegate calls.
Best Practices for Solidity Assembly
While assembly provides powerful optimization capabilities, it should be used sparingly and carefully. Here are some best practices:
- Use Assembly Only When Necessary: If an operation can be done efficiently in Solidity’s high-level syntax, there’s no need to use assembly. Reserve it for specific use cases where optimization is critical.
- Test Extensively: Assembly is more error-prone than high-level Solidity, so thorough testing is crucial. Ensure that all edge cases and potential vulnerabilities are covered.
- Avoid Complex Logic in Assembly: Keep assembly logic simple. Complex logic increases the chances of introducing errors and security vulnerabilities.
- Leverage Audits: Whenever assembly is used, having the contract audited by professionals is essential to catch potential issues that might not be obvious in low-level code.
- Document Your Code: Because assembly code can be difficult to understand, it’s important to document your intentions clearly to help future developers (or even yourself) understand the purpose of the code.
FAQs
What is Solidity assembly?
- Solidity assembly is a low-level language that allows developers to write EVM-specific instructions directly in smart contracts, offering greater control over how the code interacts with the Ethereum Virtual Machine.
Why should I use assembly in Solidity?
- Assembly is used to optimize contracts for gas efficiency, improve performance, handle custom low-level logic, or manipulate memory and storage more directly.
How can assembly help in gas optimization?
- Assembly allows developers to bypass Solidity’s abstractions, reducing gas costs by performing more efficient low-level operations, such as direct memory manipulation or avoiding redundant function calls.
What are the risks of using assembly in Solidity?
- Assembly is more error-prone than high-level Solidity and can introduce security vulnerabilities. It also makes the code harder to read, maintain, and audit.
When should I avoid using assembly?
- Avoid using assembly when high-level Solidity can achieve the same result efficiently. Use it only when specific optimizations are necessary, and ensure the logic remains simple and well-documented.