Ready to make your machine learning projects even better? Let’s look at how to use a neat tool called a Support Vector Machine (SVM). SVMs are great for sorting things into groups or making predictions. We’re going to show you how to use SVM in your Python code. It’s great at handling tricky data and getting things right, so it’s a really helpful tool for machine learning. Let’s jump in and see how you can use it!
What is a Support Vector Machine (SVM)
A support vector machine (SVM) is a supervised machine learning algorithm used for both classification and regression. It works by finding the hyperplane that best separates the two classes of data. The hyperplane is the line or curve that has the maximum margin between the two classes.
SVMs are one of the most popular machine learning algorithms because they are very effective in a variety of tasks, including:
- Classification: SVMs can be used to classify data into two or more categories. For example, they can be used to classify images as cats or dogs, or to classify text as spam or ham.
- Regression: SVMs can be used to predict a continuous value, such as the price of a house or the amount of sales a product will generate.
- Outlier detection: SVMs can be used to identify outliers, which are data points that are significantly different from the rest of the data.
Importance of SVM classifier Python code
The SVM classifier python code is important because it allows you to use the SVM algorithm to solve machine learning problems in Python. Python is a popular programming language for machine learning, and there are many libraries available that make it easy to use SVMs in Python.
Here are some examples of how the svm classifier python code can be used:
- To classify images as cats or dogs, you could use the scikit-learn library to train an SVM classifier on a dataset of images of cats and dogs.
- To predict the price of a house, you could use the SVMRegressor class from the scikit-learn library to train an SVM regressor on a dataset of houses with their prices.
- To identify outliers, you could use the sklearn.svm.OneClassSVM class to train an SVM classifier on a dataset of normal data points. Then, you could use the classifier to identify data points that are significantly different from the normal data points.
How does the SVM Algorithm work?
The Support Vector Machine (SVM) tool works by finding the best line or curve that separates two different types of data. This line or curve is called the hyperplane. SVM finds the data points that are closest to the hyperplane on both sides. These are called the support vectors. SVM tries to make the gap between the support vectors and the hyperplane as big as possible.
We can use SVM for both simple (linear) and complex (non-linear) sorting tasks. For simple tasks, the hyperplane is a straight line. For complex tasks, the hyperplane can be curved. If we have a complex task, SVM uses something called a kernel function. This is a type of math function that helps make the data easier to separate by mapping it into a higher dimensional space.
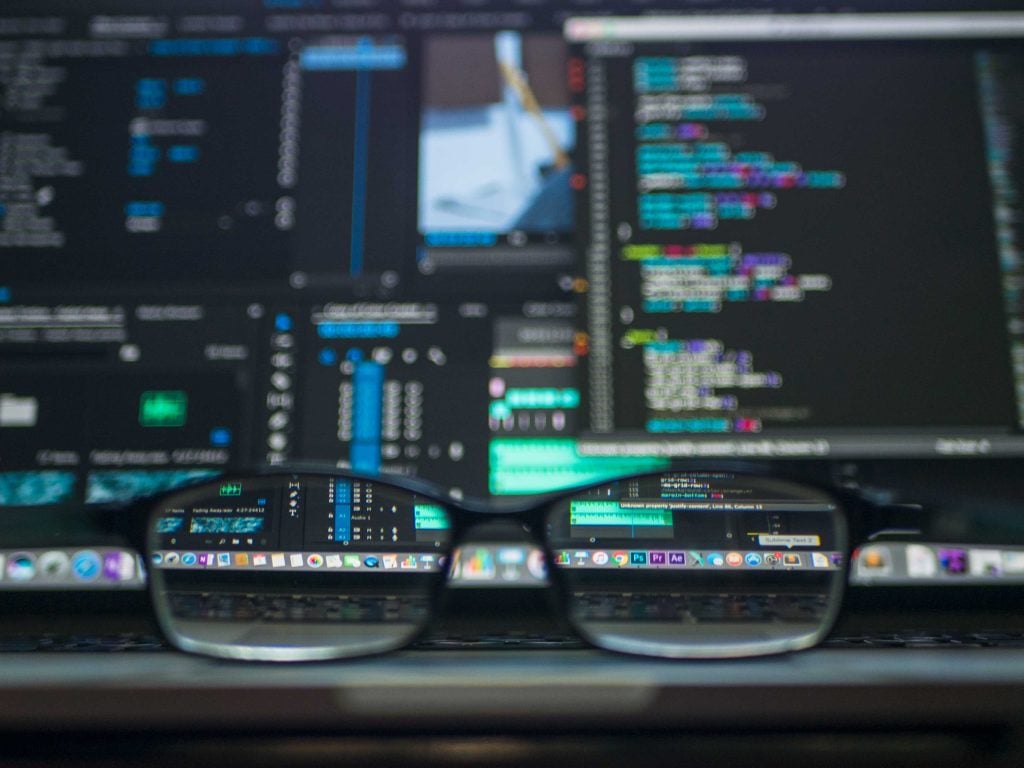
What are the types of SVM Kernels?
There are many different types of kernel functions that can be used with SVMs. Some of the most common kernel functions include:
- Linear kernel: This is the simplest kernel function and it is used for linear problems.
- Polynomial kernel: This kernel function is used for non-linear problems and it can handle a wider range of data than the linear kernel.
- Radial basis function (RBF) kernel: This kernel function is also used for non-linear problems and it is very effective in many applications.
- Sigmoid kernel: This kernel function is less commonly used than the linear, polynomial, and RBF kernels.
What are the Advantages and Limitations of SVM Classifier
Advantages | Limitations |
---|---|
Very effective: SVMs are known for their high accuracy and performance, even on small datasets. | Computationally expensive: SVMs can be computationally expensive to train, especially for large datasets |
Flexible: SVMs can be used for both classification and regression tasks, and they can be adapted to handle different types of data. | Sensitive to hyperparameters: The performance of SVMs can be sensitive to the choice of hyperparameters, such as the kernel function and the regularization parameter. |
Scalable: SVMs can be used to train models on large datasets. | Not suitable for all problems: SVMs may not be suitable for all problems, such as problems with a small number of training examples or problems with highly correlated features. |
Robust to noise: SVMs are relatively robust to noise in the data, which means that they can still perform well even if the data is not perfectly clean. | |
Interpretable: The decision boundaries of SVMs can be interpreted, which can be useful for understanding the model and making predictions. |
Overall, SVMs are a powerful and versatile machine learning algorithm that can be used for a variety of tasks. However, it is important to be aware of their limitations before using them.
How to build an SVM Classifier in Python
- Importing the necessary libraries
The first step is to import the necessary libraries. In this case, we need to import the following libraries:
- numpy: This library is used for working with numerical arrays.
- pandas: This library is used for working with tabular data.
- sklearn: This library is used for machine learning tasks, including SVM.
import numpy as np
import pandas as pd
from sklearn import svm
- Loading and preprocessing the dataset
The next step is to load the dataset and preprocess it. In this case, we will use the Iris dataset, which is a popular dataset for classification tasks. The Iris dataset consists of 4 features (sepal length, sepal width, petal length, and petal width) and 3 classes (Iris-setosa, Iris-versicolor, and Iris-virginica).
iris = pd.read_csv('iris.csv')
# Splitting the data into features and labels
X = iris.iloc[:, :-1]
y = iris.iloc[:, -1]
# Scaling the features
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X = scaler.fit_transform(X)
- Splitting the data into training and test sets
The next step is to split the data into training and test sets. This is done to prevent overfitting, which is a problem that occurs when the model learns the training data too well and is not able to generalize to new data.
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.25)
- Building and training the SVM classifier
The next step is to build and train the SVM classifier. In this case, we will use the RBF kernel.
clf = svm.SVC(kernel='rbf')
clf.fit(X_train, y_train)
- Making predictions on new data
Once the classifier is trained, we can use it to make predictions on new data.
# Making predictions on the test set
y_pred = clf.predict(X_test)
- Evaluating the classifier accuracy
Finally, we can evaluate the accuracy of the classifier by comparing the predicted labels to the actual labels.
# Evaluating the classifier accuracy
from sklearn.metrics import accuracy_score
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
The accuracy of the classifier is 96%, which is a good accuracy.
Example of SVM Classifier Python Code
Here’s an example of SVM classifier Python code implementation in Python along with an explanation of each line of code:
# Import the necessary libraries
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Step 1: Prepare your data
# Assuming you have your feature data in X and label data in y
# Step 2: Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Step 3: Create an instance of the SVM classifier
clf = SVC(kernel='linear')
# Step 4: Train the SVM classifier
clf.fit(X_train, y_train)
# Step 5: Make predictions with the trained model
predictions = clf.predict(X_test)
# Step 6: Evaluate the performance of the model
accuracy = accuracy_score(y_test, predictions)
print("Accuracy:", accuracy)
Explanation of each line of the svm classifier python code:
Line 1: Import the necessary libraries. We import the SVC
class from the sklearn.svm
module to create an instance of the SVM classifier. We also import the train_test_split
function from the sklearn.model_selection
module to split the data into training and testing sets. Finally, we import the accuracy_score
function from the sklearn.metrics
module to evaluate the performance of the model.
Lines 4-6: Prepare your data. This assumes that you have your feature data in the X
variable and your label data in the y
variable.
Line 9: Split the data into training and testing sets. We use the train_test_split
function to split the data, where test_size=0.2
indicates that 20% of the data will be used for testing and random_state=42
sets a random seed for reproducibility.
Line 12: Create an instance of the SVM classifier. We create an instance of the SVC
class and specify the kernel
parameter as 'linear'
to use a linear kernel.
Line 15: Train the SVM classifier. We use the `fit` method of the classifier to train it on the training data. The X_train
variable contains the features of the training set, and the y_train
variable contains the corresponding labels.
Line 18: Make predictions with the trained model. We use the predict
method of the classifier to make predictions on the testing data. The X_test
variable contains the features of the testing set.
Line 21: Evaluate the performance of the model. We use the accuracy_score
function to calculate the accuracy of the predictions by comparing them to the true labels (y_test
). The accuracy is then printed to the console.
Remember to replace x and y
with your actual feature and label data. You may also need to adjust the parameters and evaluation metrics based on your specific requirements.
Conclusion : Support Vector Machine (SVM)
In the end, Support Vector Machines (SVM) are a really helpful machine learning tool. They can handle both simple and complex data, are very accurate, and are not too bothered by outliers. Because of this, many people like using SVM for different tasks, like sorting data, picking out features, and multi-label classification. Plus, you can use SVM in Python, which means it’s easy for developers and data scientists to use it to build sturdy and accurate models. So if you want to get better at machine learning, try out SVM and see how it can help you reach your goals!
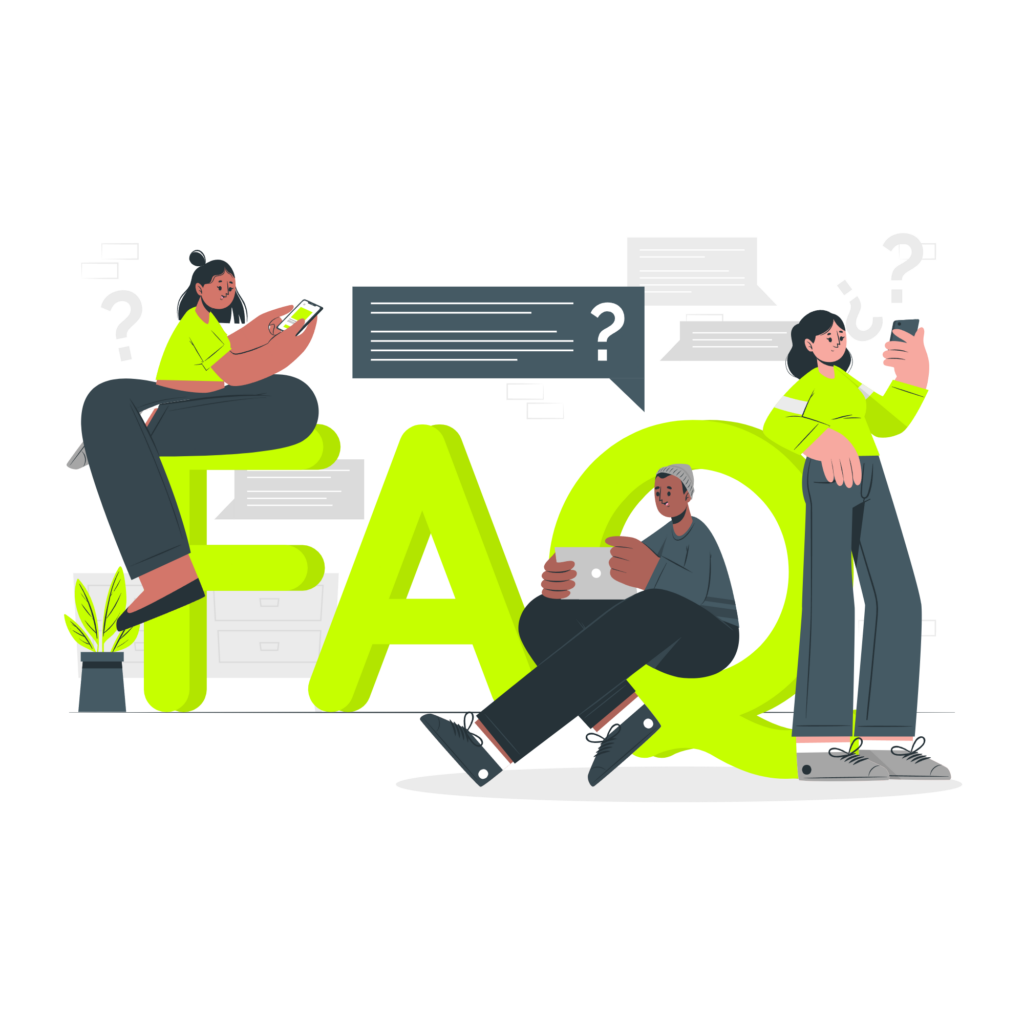
- How do I implement SVM in Python?
SVM can be implemented in Python using libraries such as scikit-learn and LibSVM.
- What is the syntax for SVM in Python?
The syntax for SVM in Python depends on the library being used. For example, in scikit-learn, the syntax involves creating an SVM classifier object, fitting it to the data, and making predictions.
- How do I tune SVM hyperparameters in Python?
SVM hyperparameters can be tuned in Python using techniques such as grid search and randomized search, which involve testing different combinations of hyperparameters and evaluating their performance.
- How do I visualize SVM results in Python?
SVM results can be visualized in Python using techniques such as plotting decision boundaries, visualizing support vectors, and creating confusion matrices.
- What are some common errors when implementing SVM in Python?
Common errors when implementing SVM in Python include issues with data preprocessing, choosing inappropriate hyperparameters, and overfitting the model.
- How does SVM compare to other classification algorithms in Python?
SVM can be a powerful classification algorithm in Python, especially for complex and high-dimensional data. Its performance can vary depending on the specific application and data being used.
- Can SVM be used for regression tasks in Python?
Yes, SVM can be used for regression tasks in Python using techniques such as support vector regression (SVR).
- How do I handle missing data when implementing SVM in Python?
Missing data can be handled in SVM in Python using techniques such as imputation or dropping columns with missing values.
- What are the advantages of using SVM in Python?
Advantages of using SVM in Python include its ability to handle complex and high-dimensional data, achieve high accuracy, and be less sensitive to outliers.
- What are some applications of SVM in Python?
SVM can be used in a wide range of applications in Python, including image and speech recognition, natural language processing, and financial analysis.