TLDR:
CLI commands are text-based instructions that allow users to interact with computers through a command-line interface instead of using a graphical interface. Learning CLI commands can significantly improve productivity by enabling faster task execution, powerful automation capabilities, and more precise control over your operating system. This guide covers essential CLI commands, their syntax, and practical usage across different operating systems.
Understanding What CLI Commands Are
The Command Line Interface (CLI) represents one of the most fundamental ways to interact with computers. Understanding what are CLI commands is essential for anyone looking to master computer operations. Unlike the Graphical User Interface (GUI) that most users are familiar with, CLI commands are text-based instructions typed directly into a terminal or command prompt. These commands tell your computer exactly what to do without the need for visual elements like buttons or menus.
Learning what CLI commands are and how to use them is increasingly important in today’s computing environment. While GUIs are user-friendly and intuitive, they often lack the power, flexibility, and efficiency that CLI commands provide. Developers, system administrators, and power users rely on CLI commands daily to perform tasks more efficiently than possible through graphical interfaces.
Why Should You Learn What CLI Commands Are?
Efficiency and Speed
One of the primary reasons to learn what CLI commands are is the incredible speed advantage they offer. Once you become familiar with common commands, you can execute tasks much faster than navigating through multiple menus and dialogs in a GUI. For example, creating multiple folders with specific naming patterns can be done in seconds using a single CLI command, compared to multiple clicks in a file explorer.
Automation Capabilities
CLI commands excel at automation. Understanding what CLI commands are opens the door to creating scripts that can automate repetitive tasks. Instead of manually performing the same series of actions repeatedly, you can write a script containing multiple CLI commands that execute in sequence, saving hours of work over time.
Control and Flexibility
CLI commands provide direct access to your operating system without the abstraction layers present in GUIs. This gives you more precise control over your computer’s operations. Many advanced functions are only accessible via CLI commands, making them essential for certain specialized tasks.
Professional Growth
Mastering what CLI commands are and how to use them effectively is a valuable skill in the tech industry. It’s often considered a prerequisite for roles in software development, system administration, cybersecurity, and other technical fields. The knowledge of CLI commands demonstrates technical proficiency and problem-solving abilities.
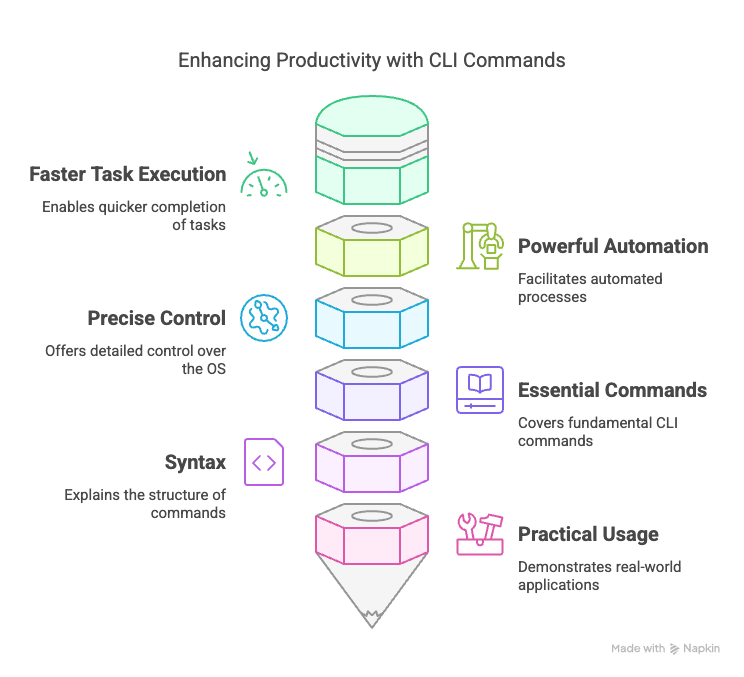
Essential Components of CLI Commands
The Command Prompt
Before learning what CLI commands are, it’s important to understand the command prompt. This is the symbol or text that indicates your system is ready to receive input. Common prompts include:
$
(Linux/macOS for regular users)#
(Linux/macOS for root/administrator users)>
orC:\>
(Windows Command Prompt)
Basic Command Structure
CLI commands typically follow a consistent structure:
command [options] [arguments]
- Command: The specific instruction you want the computer to execute
- Options: Also called flags or switches, these modify how the command behaves
- Arguments: The targets or inputs for the command, such as file names or paths
What Are CLI Commands You Should Know First?
File System Navigation Commands
Learning what CLI commands are for navigating the file system is essential for beginners:
cd
(Change Directory): Moves you between folders- Example:
cd Documents
moves into the Documents folder - Example:
cd ..
moves up one level in the directory hierarchy
- Example:
ls
(Linux/macOS) ordir
(Windows): Lists files and folders in the current directory- Example:
ls -la
shows all files (including hidden ones) with detailed information
- Example:
pwd
(Print Working Directory): Displays your current location in the file system (Linux/macOS)- Example:
pwd
might show/home/username/Documents
- Example:
File Management Commands
Understanding what CLI commands are used for file operations allows you to manipulate files efficiently:
mkdir
(Make Directory): Creates new folders- Example:
mkdir Projects
creates a new folder named “Projects”
- Example:
touch
(Linux/macOS): Creates empty files- Example:
touch newfile.txt
creates an empty text file
- Example:
cp
(Copy, Linux/macOS) orcopy
(Windows): Copies files or directories- Example:
cp file.txt backup/
copies file.txt to the backup folder
- Example:
mv
(Move, Linux/macOS) ormove
(Windows): Moves files or renames them- Example:
mv oldname.txt newname.txt
renames a file
- Example:
rm
(Remove, Linux/macOS) ordel
(Windows): Deletes files- Example:
rm unwanted.txt
deletes the specified file
- Example:
File Content Commands
These CLI commands help you work with file contents:
cat
(Concatenate, Linux/macOS): Displays file contents- Example:
cat readme.txt
shows the contents of readme.txt
- Example:
more
orless
(Linux/macOS/Windows): Displays file contents one screen at a time- Example:
more logfile.txt
shows the content with paging
- Example:
grep
(Linux/macOS) orfindstr
(Windows): Searches for specific text within files- Example:
grep "error" log.txt
finds all occurrences of “error” in log.txt
- Example:
System Information Commands
CLI commands for system information help you monitor your computer:
top
(Linux/macOS) ortasklist
(Windows): Shows running processes- Example:
top
displays a dynamic list of running processes
- Example:
df
(Disk Free, Linux/macOS): Shows disk space usage- Example:
df -h
displays disk usage in human-readable format
- Example:
systeminfo
(Windows): Displays detailed system information- Example:
systeminfo
shows hardware and software configuration
- Example:
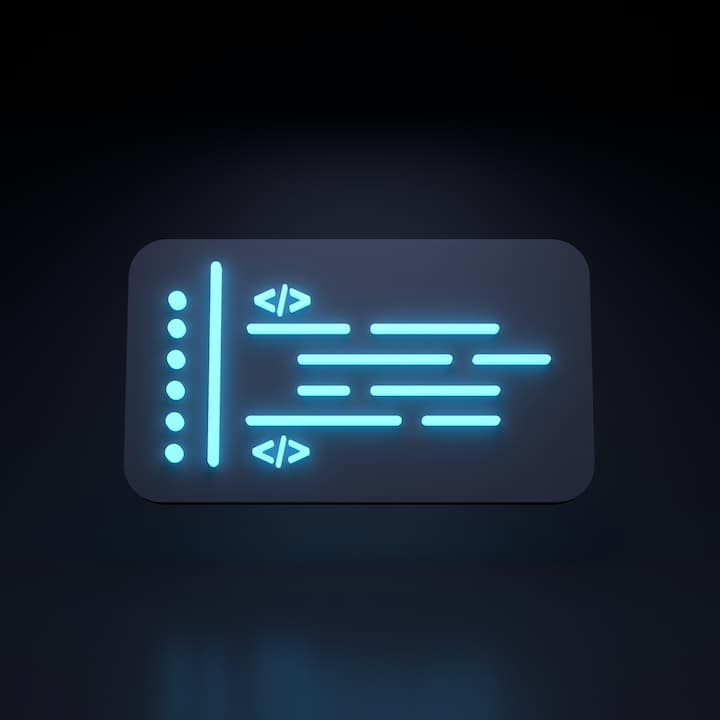
Understanding CLI Command Syntax
Basic Syntax Patterns
To effectively use what CLI commands are available, you need to understand their syntax:
- Most commands follow the pattern:
command -options arguments
- Options are often prefixed with
-
(single letter) or--
(word) - Example:
ls -l --color=auto
lists files in long format with colored output
Command Chaining
CLI commands can be combined for more powerful operations:
- Using semicolons (
;
) to run multiple commands sequentially- Example:
mkdir project; cd project; touch readme.md
- Example:
- Using
&&
to run subsequent commands only if the previous command succeeds- Example:
cd project && ls -la
only lists files if changing directory succeeds
- Example:
Input/Output Redirection
Understanding what CLI commands are for redirecting input and output is crucial:
>
redirects output to a file (overwrites existing content)- Example:
echo "Hello" > greeting.txt
- Example:
>>
appends output to a file- Example:
echo "Additional text" >> notes.txt
- Example:
|
(pipe) sends output from one command as input to another- Example:
ls -la | grep "May"
lists files and then filters for those containing “May”
- Example:
Operating System-Specific CLI Commands
What Are CLI Commands in Windows?
Windows Command Prompt has its own set of commands:
dir
: Lists directory contentscls
: Clears the screencopy
andxcopy
: Copies files and directoriesdel
andrmdir
: Removes files and directoriesipconfig
: Displays network configurationtaskkill
: Terminates processes
What Are CLI Commands in Linux/macOS?
Unix-based systems share many common commands:
ls
: Lists directory contentsclear
: Clears the terminal screencp
andmv
: Copies and moves filesrm
andrmdir
: Removes files and directoriesifconfig
orip
: Displays network configurationkill
: Terminates processes
Cross-Platform CLI Usage
Modern developers often need to work across different operating systems. Tools like Windows Subsystem for Linux (WSL) allow running Linux commands on Windows, and understanding what CLI commands are available across platforms is increasingly important for cross-platform development.
Advanced CLI Concepts
Shell Scripting
Once you understand what CLI commands are, you can combine them into scripts:
#!/bin/bash
# Simple backup script
BACKUP_DIR="/backup/$(date +%Y%m%d)"
mkdir -p $BACKUP_DIR
cp -r /important/files/* $BACKUP_DIR
echo "Backup completed to $BACKUP_DIR"
Environment Variables
Environment variables store configuration information for CLI commands:
- Setting an environment variable:
export PATH=$PATH:/new/path
(Linux/macOS) orset PATH=%PATH%;C:\new\path
(Windows) - Accessing environment variables:
echo $PATH
(Linux/macOS) orecho %PATH%
(Windows)
Package Management
Many CLI commands are available through package managers:
apt
orapt-get
(Debian/Ubuntu):apt install package-name
yum
ordnf
(Fedora/CentOS):yum install package-name
brew
(macOS):brew install package-name
choco
(Windows):choco install package-name
Troubleshooting Common CLI Issues
Command Not Found
If you get “command not found” errors:
- Check your spelling
- Ensure the command is installed
- Verify the command is in your PATH
Permission Denied
For “permission denied” errors:
- Use
sudo
(Linux/macOS) to run commands with administrator privileges - Check file permissions with
ls -l
(Linux/macOS) oricacls
(Windows)
Getting Help
Most CLI commands include built-in help:
command --help
orcommand -h
: Shows brief help informationman command
(Linux/macOS): Displays the manual page for the command
Tips for Mastering CLI Commands
Practice Regularly
The best way to learn what CLI commands are and how to use them is through regular practice. Try replacing GUI actions with CLI commands whenever possible.
Learn Keyboard Shortcuts
Keyboard shortcuts can make CLI usage even faster:
Tab
: Auto-completes commands and file namesCtrl+C
: Interrupts the current commandCtrl+L
orclear
: Clears the screenUp/Down
arrows: Navigates command history
Create Aliases
Create shortcuts for frequently used commands:
- Linux/macOS:
alias ll='ls -la'
lists all files with details - Windows:
doskey ls=dir
creates an alias for thedir
command
Use Online Resources
Continue learning what CLI commands are through:
- Interactive tutorials on platforms like Codecademy or freeCodeCamp
- Command reference websites like SS64.com
- Community forums like Stack Overflow
Conclusion
Understanding what are CLI commands and how to use them effectively is a powerful skill that enhances your computing capabilities. Whether you’re a developer, system administrator, or power user, mastering CLI commands allows you to work more efficiently, automate repetitive tasks, and gain deeper control over your operating system.
As you continue your journey exploring CLI commands, remember that practice is key. Start with basic commands and gradually incorporate more complex ones into your workflow. Before long, you’ll find yourself preferring the command line for many tasks you previously performed through a graphical interface.
The command line might seem intimidating at first, but the investment in learning what CLI commands are available to you will pay dividends throughout your computing career.
FAQs
What are CLI commands?
- CLI (Command Line Interface) commands are text-based instructions used to interact with a computer system, often for tasks like managing files or running programs.
How do I use CLI commands?
- You use a terminal or command prompt to type commands, which are executed by the system to perform various tasks like navigating directories or installing software.
Are CLI commands the same for all operating systems?
- No, CLI commands differ between operating systems. For example, Linux and macOS use bash or zsh, while Windows uses Command Prompt or PowerShell.
What are some common CLI commands?
- Common commands include
cd
(change directory),ls
(list files),mkdir
(make directory), andrm
(remove file).
Why should I learn CLI commands?
- Learning CLI commands can improve your efficiency, provide more control over your system, and is essential for tasks like software development and server management.