Git and GitHub have become indispensable tools in modern software development. They empower developers to collaborate seamlessly, track changes over time, and maintain an organized workflow. However, despite their power and widespread use, GitHub issues can be challenging for beginners and even experienced developers.
Making a single mistake—such as committing to the wrong branch or mishandling a merge conflict—can lead to confusion, downtime, or messy repositories. This article highlights six common GitHub issues developers encounter and provides practical solutions to resolve them efficiently.
TL;DR:
- GitHub is a platform for version control and collaboration, enabling teams to manage code, track changes, and streamline workflows.
- Developers often face challenges like committing to the wrong branch, forgetting to
gitignore
, and dealing with merge conflicts or unsynced repositories. - Practical fixes include cherry-picking commits, updating
.gitignore
, using Git LFS for large files, and syncing local and remote repositories. - Understanding how to resolve Git and GitHub issues is important for maximizing productivity and maintaining a seamless development process.
- By addressing these GitHub issues, developers can maintain cleaner repositories and collaborate more effectively.
Understanding GitHub
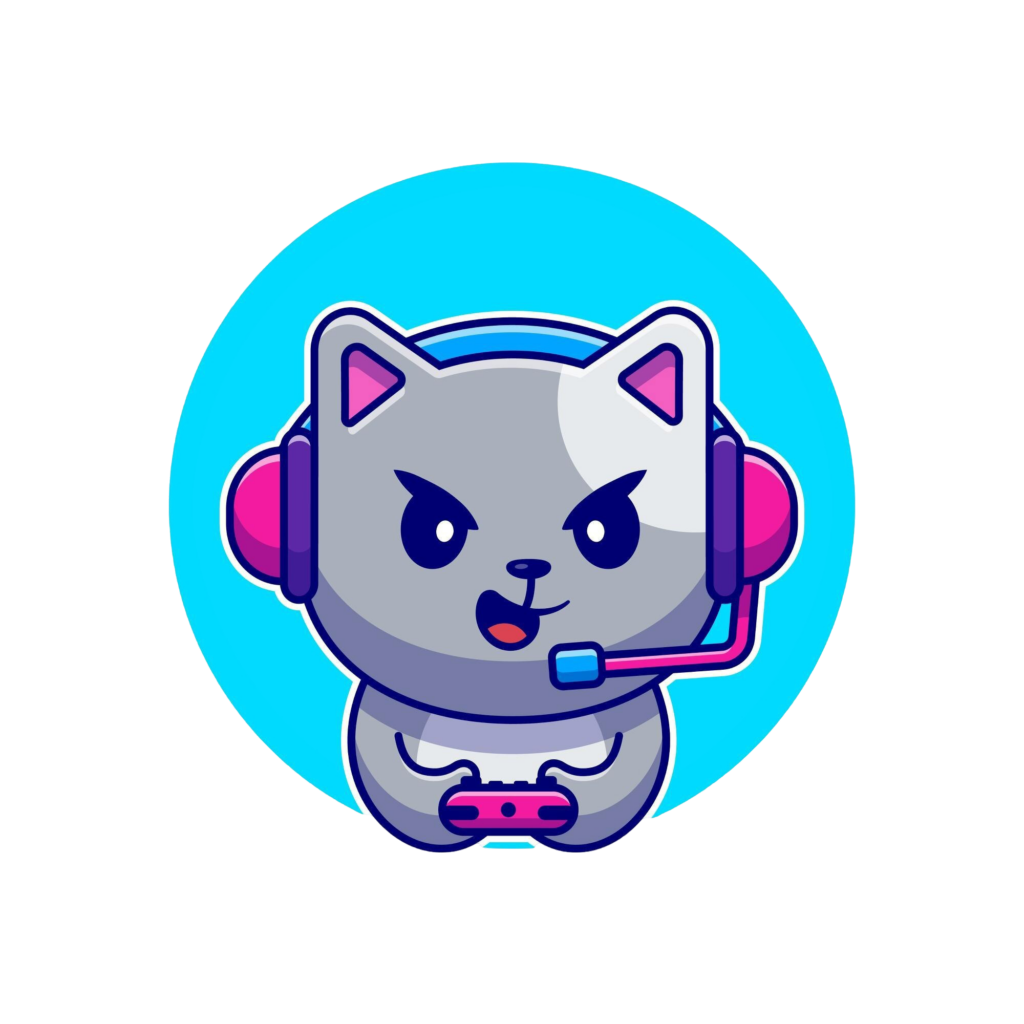
GitHub is a web-based platform built around the Git version control system that helps developers collaborate on code more effectively. By hosting projects on GitHub, users gain access to features like pull requests for code reviews, issues for tracking bugs, and GitHub Actions for automating tests and deployments. This streamlined workflow not only enhances productivity and accountability but also fosters a vibrant community where individuals and organizations can openly share insights and contribute to each other’s codebases.
Common Issues on GitHub
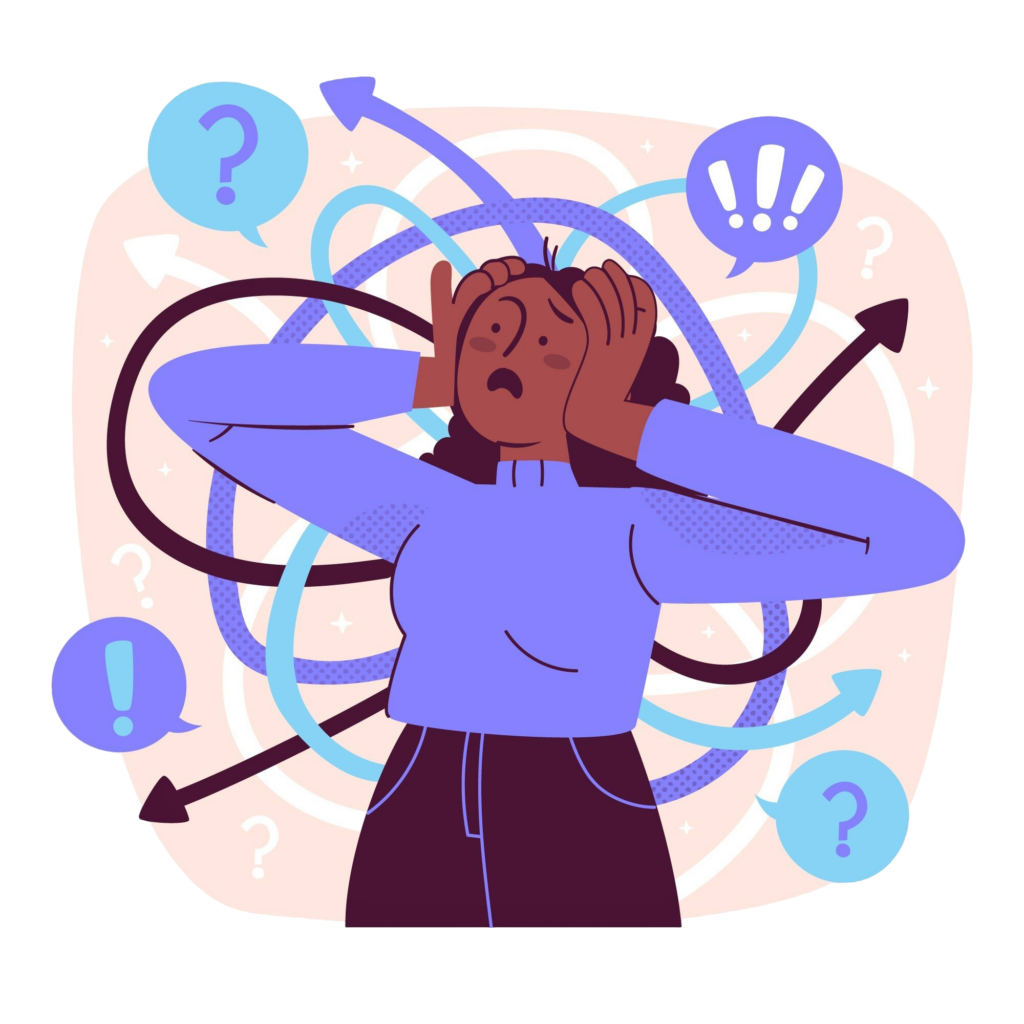
Despite its many advantages, using GitHub can sometimes be challenging, especially for developers new to version control best practices. Understanding how to troubleshoot problems powers up developers to fully harness GitHub’s capabilities which leads to smoother collaborations and more robust software.
1. Committing to the Wrong Branch
One of the most common Git mistakes is realizing that you’ve just committed to the wrong branch. Perhaps you intended to commit your changes to a feature
branch but accidentally committed them to main
. This can lead to a lot of issues, especially if those changes are not ready for production.
So how exactly could you fix this issue,
- Step1 – Identify your latest commit
- Run
git log
to identify and confirm the commit hash of the wrong commit.
- Run
- Step 2 – Create or switch to the correct branch
- If you haven’t already created the branch you intended to work on, do so with
git checkout -b <correct-branch>
- If the branch already exists, just switch to it by running the command
git checkout <correct-branch>
- If you haven’t already created the branch you intended to work on, do so with
- Step 3 – Cherry-pick the commit
- Cherry-picking means copying a specific commit from one branch to another. Run the command
git cherry-pick <commit-hash>
to cherry pick the commit and apply it to the correct branch.
- Cherry-picking means copying a specific commit from one branch to another. Run the command
- Step 4 -Remove the commit from the wrong branch
- Now go back to the original (wrong) branch and remove the wrong commit. You could do this by running the command
git revert <commit-hash>
on the wrong branch.
- Now go back to the original (wrong) branch and remove the wrong commit. You could do this by running the command
To avoid repeating this mistake, regularly verify your current branch by running git branch
or checking your terminal prompt if it displays the branch name. This practice makes sure that your changes are made to the correct branch and are managed effectively.
2. Forgetting to gitignore Files
A .gitignore
file specifies which files and directories Git should ignore in a project. This is extremely important for preventing sensitive information and unnecessary files from cluttering a repository.
However, it’s easy to forget to add entries to your .gitignore
at the start of a project, which can result in committing files that don’t belong in version control.
So how exactly could you fix this issue,
- Step 1 – Update your
.gitignore
- Include the necessary entries in your
.gitignore
file to make sure that all unwanted files or directories are excluded from version control.
- Include the necessary entries in your
- Step 2 – Remove the unwanted files from Git
- Simply adding an entry to
.gitignore
won’t remove files that have already been committed to the repository. To exclude them properly, you need to remove the files from Git’s tracking by running the commandgit rm -r --cached <path-to-file-or-folder>
- Simply adding an entry to
- Step 3 – Commit the changes
- Once you have removed all unwanted files from Git, you can go ahead and push your changes to the correct branch. After this these files will no longer be available in future commits although they will be available in history.
To avoid repeating this mistake, always set up your .gitignore
file as one of the very first steps when you create a new repository. You can take advantage of GitHub’s curated .gitignore
templates for popular programming languages and frameworks. These templates provide a great starting point and help you include the right exclusions.
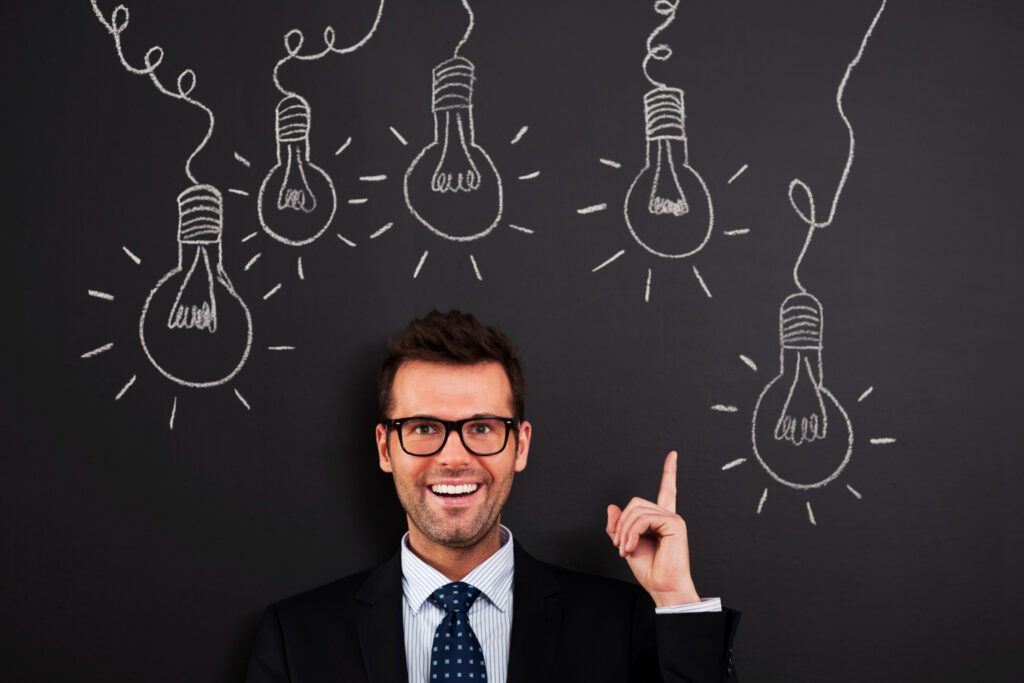
3. Accidentally Pushing Large Unwanted Files to a Repo
Sometimes you might commit and push large files such as high-resolution images, video files, or compiled binaries to the repository by mistake. This can quickly balloon the size of your repository and make cloning or pulling unnecessarily time-consuming. Removing these large files from the commit history can be tricky.
So how exactly could you fix this issue,
- Step 1 – Remove those files from Git tracking
- If you catch the issue early, you can prevent the large file from being included in future commits by removing it from the index. Use the command
git rm --cached <large-file>
to untrack the file while keeping it locally, then push the changes to GitHub to update the repository.
- If you catch the issue early, you can prevent the large file from being included in future commits by removing it from the index. Use the command
- Step 2 – Rewrite history
- If the large file has already been committed and needs to be completely removed from the repository, including all past commits, you can rewrite the Git history using tools like
git filter-branch
orgit filter-repo
to erase all traces of the file.
- If the large file has already been committed and needs to be completely removed from the repository, including all past commits, you can rewrite the Git history using tools like
If your project regularly needs large files, consider using Git Large File Storage. It stores large files on a remote server optimized for binary data, preventing your Git history from becoming huge.
4. Working on the Wrong Branch
Working on the wrong branch is a variation of making a commit to the wrong branch but can lead to more extensive issues. Perhaps you began coding on main
or a release branch instead of your feature branch, or you accidentally continued adding commits to a branch that was meant for a different task.
So how exactly could you fix this issue,
- Step 1 – Identify all the relevant commits
- Use
git log --oneline
to find the commit on the wrong branch.
- Use
- Step 2 – Create a new branch
- Checkout to a valid branch and create a new branch by running the command
git checkout -b <new-branch>
- Checkout to a valid branch and create a new branch by running the command
- Step 3 – Cherry-pick or merge commit
- Move the commits you made on the wrong branch into the new one. You can cherry pick them by running the command
git cherry-pick <commit-hash>
or use a merge if that makes more sense in your workflow.
- Move the commits you made on the wrong branch into the new one. You can cherry pick them by running the command
- Step 4 – Clean up the old branch
- If the old branch now contains extraneous changes, revert or reset them as needed
To avoid this mistake being repeated, create or switch to your feature branch immediately after you start your development session. A lot of developers use prompts or specialized Git GUI clients that highlight the current branch to prevent confusion.
5. Files Missing on a Pull Request but Available on the Branch
Occasionally, you might open a pull request and find out that certain files are missing or that GitHub shows fewer changes than you expected, even though the files are present in your local branch. This scenario often occurs due to local commits not being pushed, or because of merges that have gone wrong.
So how exactly could you fix this issue,
- Step 1 – Make sure that all local commits are pushed
- First, verify that all your local commits have been pushed to GitHub. Run
git status
to check the current state of your branch and confirm if it’s up-to-date with the remote branch.
- First, verify that all your local commits have been pushed to GitHub. Run
- Step 2 – Check for untracked files
- Look for any untracked files that might be missing from your PR. If there are any, stage them using
git add .
, commit them with a meaningful message, and push them to GitHub.
- Look for any untracked files that might be missing from your PR. If there are any, stage them using
- Step 3 – Validate the pull request’s base and compare branches
- Double check that the pull request is set to merge the correct source branch into the correct target branch. If the base branch is incorrect, GitHub may not display the expected files.
- Step 4 – Re-open or recreate the PR
- If the above steps fail, you can close the original PR and open a new one after confirming your local branch is up to date with remote changes.
To avoid repeating this mistake make sure you always run git status
or use a Git GUI to confirm all intended changes have been staged and committed. Push regularly to make sure that your remote branch matches what you have locally.
6. Local Repo Is Not Updated with the Remote or Vice Versa
Sometimes your local repository is out of sync with the remote one. This discrepancy can lead to merge conflicts, missing commits, or outdated code. In other instances, you might have commits that aren’t appearing on GitHub, or changes from collaborators that aren’t showing up in your local environment.
So how exactly could you fix this issue,
- Step 1 – Fetch and merge or rebase
- Regularly update your local branch by pulling the latest changes from the remote repository using
git pull
(for merging) orgit pull --rebase
(for rebasing). This helps avoid conflicts and ensures you’re working with the latest code.
- Regularly update your local branch by pulling the latest changes from the remote repository using
- Step 2 – Push your local changes
- Make sure that all your local commits are pushed to GitHub using
git push
. This keeps the remote repository up-to-date and in sync with your work.
- Make sure that all your local commits are pushed to GitHub using
- Step 3 – Resolve merge conflict
- If conflicts arise during a merge or rebase, Git will pause the process and highlight the conflicting files. Manually resolve the conflicts, stage the changes, and complete the process with
git merge --continue
orgit rebase --continue
.
- If conflicts arise during a merge or rebase, Git will pause the process and highlight the conflicting files. Manually resolve the conflicts, stage the changes, and complete the process with
- Step 4 – Check remote tracking
- Check if your local branch is correctly tracking the intended remote branch using
git branch -vv
. If needed, set the correct upstream branch withgit branch --set-upstream-to=origin/branch-name
.
- Check if your local branch is correctly tracking the intended remote branch using
Best Practices for Git and GitHub Workflow
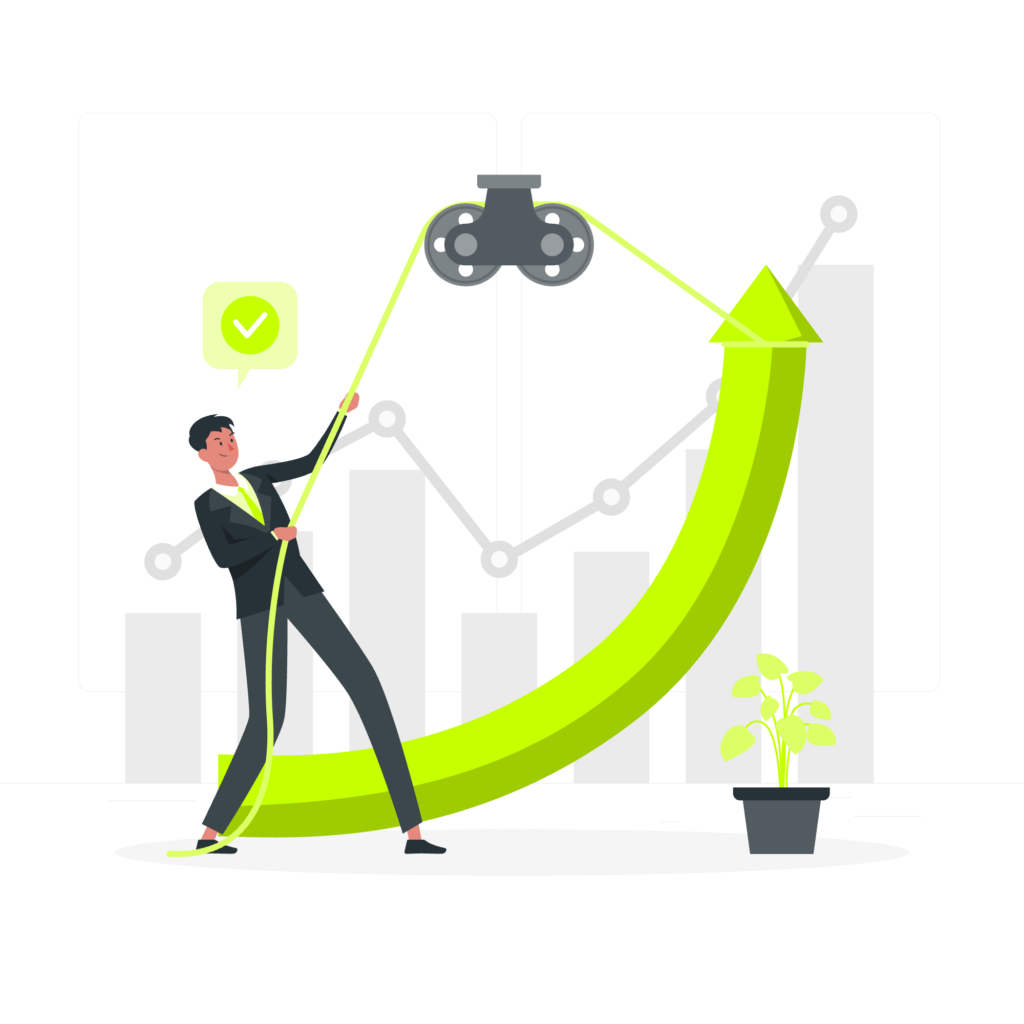
To make the most of Git and GitHub, it’s essential to follow best practices that promote efficiency, collaboration, and a clean codebase. By adopting these practices, teams can reduce errors and streamline the development process.
Commit Early and Often | Regular, small commits make it easier to track changes and revert specific commits if necessary. Each commit should focus on one logical change, this clarity makes reviewing and debugging simpler. |
Write Meaningful Commit Messages | A good commit message includes a short summary of the change, any relevant issue numbers, and a more detailed explanation if needed. This practice makes it easier to understand why a change was made. |
Set Up .gitignore Properly | Make sure your .gitignore is well-structured from the start. This prevents sensitive or unnecessary files from ever entering version control. |
Adopt a Clear Branching Strategy | Whether you choose GitFlow, GitHub Flow, or a simpler branching model, define your strategy clearly for your entire team. Use feature branches to isolate new work, and merge or rebase them into the main branch only when ready. |
Use Descriptive Branch Names | Instead of naming your branch fix-branch , consider something more informative, like fix/user-login-bug . This helps teammates and your future self understand what’s happening in each branch at a glance. |
Maintain Clear Documentation | Document your Git workflows, branching strategy, and common commands in a README or Wiki. This helps new team members come up to speed quickly and reduces friction. |
Conclusion
Git and GitHub are essential tools for modern software development, offering powerful features for version control and collaboration. While challenges can arise and disrupt workflows, understanding how to address these issues enables a smoother development and better outcomes. By following best practices and learning to troubleshoot effectively, developers can fully harness GitHub’s potential to create, manage, and deliver high-quality projects with confidence.
FAQs
What should I do if I accidentally commit sensitive information to GitHub?
- If you have committed secrets such as passwords or API keys, immediately invalidate or change them to mitigate risk. Then, remove them from the Git history using tools like
git filter-repo
orgit filter-branch
, and force-push the changes. Remember that once data is pushed to GitHub, there is a chance it was cloned or cached. Revoking the compromised credentials is paramount.
How can I recover deleted commits?
- If you have not garbage-collected yet, you can often recover deleted commits via the
git reflog
. The reflog keeps track of where your HEAD has been, allowing you to find the commit’s hash and merge or cherry-pick it back into a branch.
What is the best way to handle binary files in Git?
- The best approach is to avoid committing large binary files directly. Instead, use Git LFS for large assets or store them externally if possible. This keeps your repository size manageable and prevents performance bottlenecks.
How do I avoid merge conflicts?
- While it’s impossible to completely eliminate merge conflicts, you can minimize their occurrence by following a few key practices. First, work on small, focused branches to avoid overlapping changes. Regularly pull or fetch updates to keep your local branches in sync with the remote repository.
- Additionally, communicate with your team about any significant code changes before implementing them, making sure everyone is aligned and reducing the chance of conflicts arising.
How do I handle multiple pull requests that change the same files?
- Coordinate with the team to merge PRs in a logical order. If a PR merges first, the subsequent PR should rebase or merge those changes to avoid conflicts. Good communication and a clear workflow plan reduce clashes in the same files.