For developers, Git is the ultimate companion. It acts as a version control system, meticulously tracking changes made to your codebase. But even the most seasoned Git users encounter errors from time to time. Fear not, for this guide will equip you with the knowledge to tackle these errors head-on and keep your development workflow smooth.
Understanding Git
Imagine a project timeline. You start with a basic codebase (version 1), then add new features (version 2), fix bugs (version 3), and so on. Git keeps track of all these changes, allowing you to revert to any previous version if needed. It also facilitates collaboration, enabling multiple developers to work on the same codebase simultaneously without conflicts.
Understanding Git Errors
Git errors typically arise due to misunderstandings of commands, incorrect configurations, or conflicts during collaboration. These errors often manifest as cryptic messages on your terminal, leaving you scratching your head. But worry not, the error message usually holds the key to resolving the issue.
Common Git Errors
Now, let’s dive into some of the most frequent Git errors you might encounter:
1. Refusing to merge unrelated histories:
This error pops up when you try to merge two projects that haven’t worked together before, like trying to shove square pegs into round holes. It happens because the histories of their changes and any labels don’t line up with the code you’re trying to add or download.
Solution:
- One of the solutions to fix this issue is using the following git flag:
–allow-unrelated-histories.
- Another solution is a two-step approach. First, use git fetch to download the latest changes from the remote repository (where your master branch resides). Then, use
git merge master
to merge those changes into your feature branch (Branch A).
2. Remote origin already exists:
This error indicates that you’re trying to create a new remote named “origin” (the default name for the remote repository) when one already exists.
Solution:
- There are two ways to handle this. You can either rename the new remote using
git remote rename <old-name> <new-name>
, or you can simply use the existing “origin” remote for pushing and pulling code.
3. Failed to push some refs to <remote-name>
This error signifies an issue during the push operation. Reasons could be various, such as:
- Unauthenticated Push: You might not be authenticated to push code to the remote repository. Check your credentials and ensure you have the necessary permissions.
- Unpushed Local Commits: You might have unpushed commits in your local branch. Use git push to push them to the remote repository.
- Remote Branch Updates: The remote branch might have received updates since your last pull. Resolve any conflicts before pushing again.
4. not a git repository:
This error clearly states that Git is unable to recognize the directory you’re working in as a Git repository.
Solution:
- Make sure you’re indeed working within a Git repository. If you haven’t initialized one yet, use git init to create a new Git repository in the desired directory.
5. repository not found:
This error pops up when Git cannot locate the remote repository you’re trying to connect to. Double-check the URL you’ve provided for the remote repository and ensure it’s accurate.
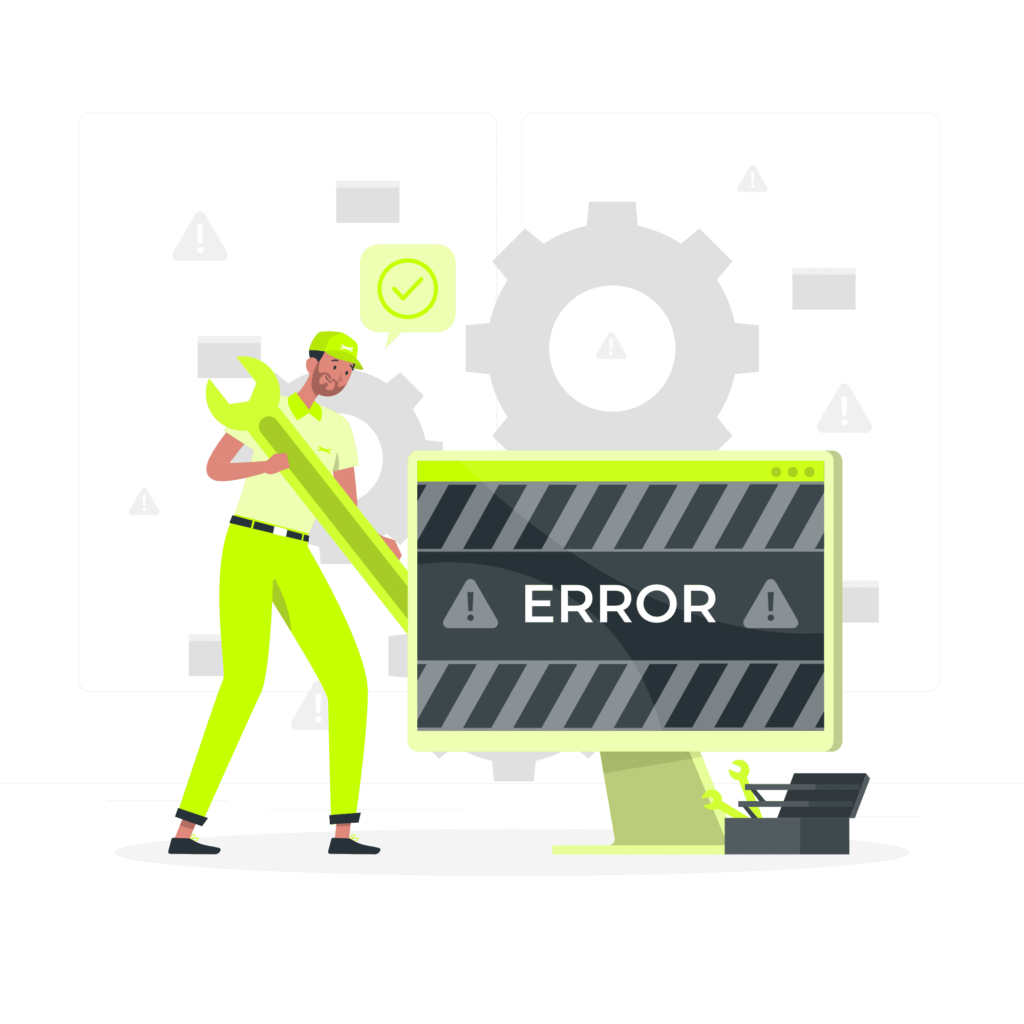
Common Git Challenges and Solutions
While errors are one aspect, there are also common challenges you might face while using Git. Here are some solutions to navigate these hurdles:
- Editing a Commit Message: You’ve made a commit, but the message isn’t clear. No worries! Use
git commit --amend
to open the previous commit message for editing. - Cleaning Local Commits Before Pushing: Sometimes, you might have a messy local history with unnecessary commits. Use
git rebase -i <branch-name
> to squash, combine, or even delete commits before pushing to the remote repository. - Reverting Pushed Commits: Made a mistake in a pushed commit? Fear not! Use
git revert <commit-hash>
to create a new commit that effectively reverses the changes introduced in the problematic commit. - Removing a File from Git Without Removing from the File System: Want to remove a file from Git’s version control but keep it in your working directory? Use
git rm --cached <filename>
. This removes the file from Git’s tracking but keeps it on your local system. - Avoiding Repeated Merge Conflicts: Merge conflicts occur when changes made in different branches affect the same lines of code. To avoid these, ensure clear communication and frequent merges between collaborating developers. Additionally, utilizing tools like visual merge editors can significantly simplify the conflict resolution process.
- Finding a Commit that Broke Something After a Merge: After a merge, if you encounter unexpected issues, you might need to identify the culprit commit. Use
git log
to view the commit history. Look for commits introduced around the time the problems started. Optionally you can usegit bisect
to find the commit that broke something after a merge. You can then usegit checkout <commit-hash>
to revert to a stable state before the problematic commit
Here is how to use git bisect :- First, tell
git bisect start
that you’re starting the process. - Then, use
git bisect bad
to mark the current commit (with the issue) as “bad.” - Point
git bisect good
to the last known commit that was definitely working (“good”). git bisect
will then automatically check out a commit roughly halfway between “good” and “bad.”- Test this commit to see if the issue persists.
- If it’s still there, mark it as “bad” with
git bisect bad
. Otherwise, mark it as “good” withgit bisect good
. git bisect
will keep iterating, narrowing down the search based on your feedback until it pinpoints the exact problematic commit.
- First, tell
Practice Makes Perfect
While these solutions provide a solid foundation, the best way to master Git is through practice. Don’t hesitate to experiment and explore different Git commands. There are also numerous online resources and tutorials available to deepen your understanding. Additionally, consider using a graphical Git client. These tools offer a visual interface for managing your Git repository, making it easier to understand complex workflows.
Embrace the Power of Collaboration
Git is a powerful tool that thrives on collaboration. Here are some tips to ensure a smooth workflow when working with others:
- Maintain a Clean Branch History: Regularly rebase your branch to keep your commit history clean and linear. This makes it easier for others to understand the code evolution.
- Communicate Effectively: Discuss changes and features with your team before starting work on a new branch. This helps to avoid conflicts and ensures everyone is on the same page.
- Utilize Pull Requests: Before merging your changes into the main branch, submit a pull request. This allows other developers to review your code and provide feedback before integration.
- Resolve Conflicts Promptly: If merge conflicts arise, address them promptly to avoid delaying the development process. Use clear communication and collaborative tools to streamline conflict resolution.
Conclusion
By understanding common Git errors, challenges, and best practices, you’ll be well-equipped to navigate the world of version control with confidence. Remember, Git is a powerful tool that empowers you to collaborate effectively, track changes meticulously, and ultimately, build better software. So, keep practicing, explore its functionalities, and embrace the power of Git for a smoother and more efficient development journey.
FAQs:
What is a ‘detached head’ in Git and how can I fix it?
- A ‘detached head’ occurs when you check out a commit that isn’t attached to any branch. To fix it, create a new branch from that commit or check out an existing branch.
How do I resolve a merge conflict in Git?
- To resolve a merge conflict, edit the files to remove the conflicts, mark the conflicted files as resolved using
git add
, and then complete the merge withgit commit
.
What causes a ‘failed to push some refs to remote’ error in Git?
- This error typically occurs if there are new commits on the remote that you don’t have locally. Fix it by pulling the changes first with
git pull
and then pushing again.
Why do I see ‘permission denied (publickey)’ when using Git?
- This happens if your SSH key isn’t added to your Git server or is misconfigured. Ensure your SSH key is correctly setup and added to your Git server account.
How can I undo a ‘git add’ before a commit?
- To undo
git add
, usegit reset <file>
to unstage a file, orgit reset
to unstage all changes.
What is Git and why is it important for developers?
- Git is a version control system that lets developers manage and track changes to their codebase, making it essential for collaborative and individual projects.
How can I improve my Git skills?
- Practice using Git regularly, explore advanced features, and consider contributing to open-source projects to gain more experience.
What are the best practices for using Git in a team environment?
- Use feature branches, frequent commits, meaningful commit messages, and always pull before you push to minimize conflicts.
Can Git be used for projects other than software development?
- Yes, Git is versatile and can manage any set of files, making it useful for collaboration on various types of projects, not just software development.
What tools integrate well with Git for project management?
- Tools like GitHub, GitLab, and Bitbucket enhance Git workflows with features like issue tracking, code reviews, and CI/CD pipelines.