JavaScript is one of the most popular programming languages used today, and understanding how to work with data types is fundamental to mastering it. One of the most common tasks involves converting JavaScript string to number. This is especially important because JavaScript is dynamically typed, meaning variables can hold values of any type, but sometimes we need to explicitly convert them for calculations or other operations. In this article, we’ll explore the various ways to convert a JavaScript string into a number and understand the best practices, use cases, and potential pitfalls.
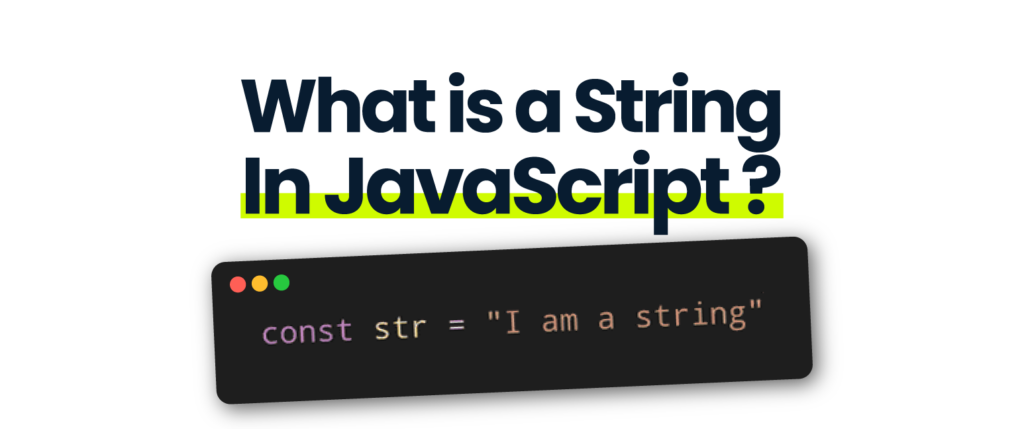
1. Introduction to Data Types in JavaScript
JavaScript, as a dynamically typed language, allows variables to hold multiple types of data. The most commonly used types include:
- String: A sequence of characters used to represent text, e.g.,
"Hello, World!"
. - Number: Represents both integer and floating-point numbers, e.g.,
10
or10.5
.
Often, especially in scenarios like receiving input from a user or fetching data from a server, we deal with numbers stored as strings. While strings are excellent for representing text, they can’t be used for mathematical operations like addition or multiplication unless converted into numbers.
Example Scenario:
// Example
let numberString = "42";
let result = numberString + 10; // Result: "4210" instead of 52
In this example, JavaScript treats numberString
as a string, concatenating instead of adding, which leads to unexpected behavior. This is why converting strings to numbers is essential.
2. Why Convert Strings to Numbers?
There are several reasons why you might need to convert a JavaScript string to a number:
- Mathematical Operations: If you want to perform any calculation on a string that represents a number, it must first be converted to a number.
- Data Validation: When receiving input from users, forms, or APIs, the input may arrive as strings even though they are supposed to be numeric.
- Performance: Operations on numbers are faster than on strings when performing repeated mathematical computations.
By properly converting strings to numbers, you ensure accurate calculations, better data handling, and improved code readability.
3. Methods for Converting JavaScript Strings to Numbers
JavaScript provides several ways to convert strings to numbers. Let’s explore the most commonly used methods.
a) Using Number()
The simplest way to convert a string to a number is by using the built-in Number()
function. This method works for both integers and floating-point numbers.
// Syntax
Number(string);
// Example
let str = "123";
let num = Number(str); // Output: 123
This method is straightforward, but it has limitations. If the string cannot be converted to a valid number, it returns NaN
(Not-a-Number).
b) Using parseInt()
If you want to convert a string to an integer, parseInt()
is another commonly used function. It parses a string and returns an integer of the specified radix (base), which defaults to 10 (decimal).
// Syntax
parseInt(string, radix);
// Example
let str = "456";
let num = parseInt(str, 10); // Output: 45
Important Note: If the string contains non-numeric characters, parseInt()
stops parsing when it encounters them.
// Example
let str = "456abc";
let num = parseInt(str); // Output: 456
parseInt()
is particularly useful when you only want the integer part of a floating-point number or are dealing with hexadecimal values.
c) Using parseFloat()
If you need to convert a string that contains decimal values, parseFloat()
is the best method. It parses a string and returns a floating-point number.
// Syntax
parseFloat(string);
// Example
let str = "123.45";
let num = parseFloat(str); // Output: 123.45
Like parseInt()
, parseFloat()
stops parsing when it encounters a character that is not part of a valid floating-point number.
d) Using the Unary +
Operator
Another shorthand method for converting a string to a number is by using the unary +
operator. This is a clean and fast way to perform the conversion.
// Syntax
+string;
// Example
let str = "789";
let num = +str; // Output: 789
This method is concise, but similar to Number()
, it returns NaN
if the string is not a valid number.
e) Using Math.floor()
, Math.ceil()
, and Math.round()
While these methods are typically used for rounding numbers, they can also implicitly convert strings to numbers.
// Example
let str = "123.9";
let rounded = Math.round(str); // Output: 124
These functions first convert the string to a number, then apply their respective rounding operation.
4. Edge Cases and Common Pitfalls
While converting strings to numbers is a common task, there are a few edge cases and pitfalls you need to watch out for:
- Non-Numeric Characters: If your string contains characters that are not numeric, functions like
Number()
will returnNaN
.parseInt()
andparseFloat()
will parse until they hit an invalid character, which can be misleading.
// Example
let str = "123abc";
let num = Number(str); // Output: NaN
let num2 = parseInt(str); // Output: 123
- Empty Strings: When an empty string is passed,
Number("")
returns0
, whileparseInt("")
andparseFloat("")
returnNaN
.
// Example
let empty = "";
let num = Number(empty); // Output: 0
let num2 = parseInt(empty); // Output: NaN
- Whitespace: Leading and trailing whitespaces are ignored by
Number()
,parseInt()
, andparseFloat().
// Example
let str = " 456 ";
let num = Number(str); // Output: 456
5. Best Practices for String to Number Conversion
To ensure your code is efficient and error-free, follow these best practices when converting strings to numbers in JavaScript:
- Choose the Right Method: Use
parseInt()
for integers,parseFloat()
for floating-point numbers, andNumber()
when you need to handle both. - Check for
NaN
: Always check forNaN
to avoid unexpected behavior. You can do this using theisNaN()
function.
// Example
let num = Number("abc");
if (isNaN(num)) {
console.log("Invalid number");
}
- Specify the Radix for
parseInt()
: Always specify the radix when usingparseInt()
to avoid bugs when dealing with numbers in bases other than 10.
// Example
let num = parseInt("1010", 2); // Output: 10 (binary to decimal conversion)
- Be Mindful of Edge Cases: Handle cases like empty strings and non-numeric characters to prevent unexpected results.
6. Real-World Applications
The ability to convert JavaScript string to number has many real-world applications, especially in web development. Some common use cases include:
- Form Validation: User inputs in forms often come as strings, even if they are numbers (like age, price, etc.). You’ll need to convert these inputs to numbers to perform validation or calculations. Example:
// Example let price = document.getElementById('price').value; // price is a string let numPrice = Number(price); // Convert to number for further operations
- API Data Handling: When receiving data from an API, numbers might come as strings, so conversion is required before processing.
Example:
fetch('/api/data')
.then(response => response.json())
.then(data => {
let age = Number(data.age);
// Now you can use the numeric value of age
});
Conclusion: Converting JavaScript String to Number
Converting a JavaScript string to a number is a fundamental task that you’ll encounter frequently in web development. Understanding the different methods availableNumber()
, parseInt()
, parseFloat()
, the unary +
operator, and others can help you write cleaner, more efficient code. Always be mindful of edge cases, and remember to validate your conversions using functions like isNaN()
.
What’s the easiest way to convert a string to a number in JavaScript?
- Use
Number()
for both integers and floating-point numbers, orparseInt()
for integers andparseFloat()
for decimals.
What happens if the string doesn’t contain a number?
- If invalid,
Number()
and+
returnNaN
, whileparseInt()
andparseFloat()
parse up to the first non-numeric character.
How do I check if the conversion was successful?
- Use
isNaN()
to check if the result isNaN
, indicating the conversion failed.
Can parseInt()
handle decimal numbers?
- No,
parseInt()
only parses integers. UseparseFloat()
for decimals.
Why specify a radix with parseInt()
?
- Specifying the radix (usually 10) ensures the string is interpreted as a decimal, avoiding confusion with octal or hexadecimal numbers.
How does JavaScript treat empty strings during conversion?
Number("")
returns0
, whileparseInt("")
andparseFloat("")
returnNaN
.
Is the unary +
operator the same as Number()
?
- Yes, both convert strings to numbers, but
+
is more concise.