Delegatecall
is a powerful but intricate feature in Solidity that allows contracts to delegate execution to other contracts. Understanding delegatecalls
is crucial for building advanced smart contract functionalities like upgradeable contracts and proxy patterns. This article dives into the world of delegatecalls, exploring their inner workings, potential pitfalls, and best practices.
Understanding delegatecall
delegatecall
in Solidity is a low-level function, that enables a contract (the Caller) to execute code from another contract (the Callee) but using the storage, sender, and value of the Caller. Essentially, delegatecall
preserves the context of the Caller while running the logic of the Callee. This feature is particularly useful for creating upgradeable and modular smart contracts.
Practical Use Cases
- Upgradeable Contracts: One of the primary uses of
delegatecall
is to create upgradeable smart contracts. By separating the contract logic from the storage, developers can update the contract’s logic without altering its state. This is achieved by deploying a new contract with the updated logic and directing calls from the proxy contract (which holds the state) to this new logic contract usingdelegatecall
.
- Modular Contract Systems:
delegatecall
allows for modular contract design, where different functionalities are split into separate contracts. This modularity enhances the manageability and scalability of the system. Each module can be upgraded independently without affecting the overall system state.
Implementing delegatecall
in Solidity
Here’s a basic example demonstrating how to use delegatecall
:
solidityCopy code<code>pragma solidity ^0.8.0;
contract Callee {
uint public num;
function setNum(uint _num) public {
num = _num;
}
}
contract Caller {
uint public num;
address public calleeAddress;
function setCalleeAddress(address _calleeAddress) public {
calleeAddress = _calleeAddress;
}
function delegateSetNum(uint _num) public {
(bool success, bytes memory data) = calleeAddress.delegatecall(
abi.encodeWithSignature("setNum(uint256)", _num)
);
require(success, "delegatecall failed");
}
}
In this example, the Caller
contract uses delegatecall
to invoke the setNum
function from the Callee
contract. However, the state changes (updating the num
variable) occur within the Caller
contract’s storage.
Detailed Example: Proxy Pattern
A common pattern utilizing delegatecall
is the proxy pattern. In this pattern, a proxy contract holds the storage and delegates function calls to an implementation contract. Here’s an example:
solidityCopy code<code>pragma solidity ^0.8.0;
// Implementation contract
contract Implementation {
uint public x;
address public owner;
function setX(uint _x) public {
x = _x;
}
}
// Proxy contract
contract Proxy {
address public implementation;
address public owner;
constructor(address _implementation) {
implementation = _implementation;
owner = msg.sender;
}
function setImplementation(address _implementation) public {
require(msg.sender == owner, "Only owner can set implementation");
implementation = _implementation;
}
fallback() external payable {
(bool success, bytes memory data) = implementation.delegatecall(msg.data);
require(success, "delegatecall failed");
}
}
In this pattern, the Proxy
contract can update its implementation by changing the implementation
address, thereby enabling contract upgrades without changing the storage.
Key Considerations and Risks
- Security Risks: Improper use of
delegatecall
can lead to significant vulnerabilities, such as unexpected state changes or reentrancy attacks. Always validate the called function and handle the return values carefully. Ensure the called contract is trusted and verified to avoid security breaches. - Storage Layout: Both the Caller and Callee contracts must share the same storage layout. Any mismatch can lead to unpredictable behavior or security vulnerabilities. Developers must carefully design and document the storage structure to prevent issues.
- Gas Consumption: Using
delegatecall
can be more gas-intensive than direct calls due to the additional overhead. Consider this factor when designing your contract architecture to optimize for efficiency. - Complexity:
delegatecall
adds complexity to the contract logic. Thorough testing and auditing are essential to ensure the system works as intended and remains secure.
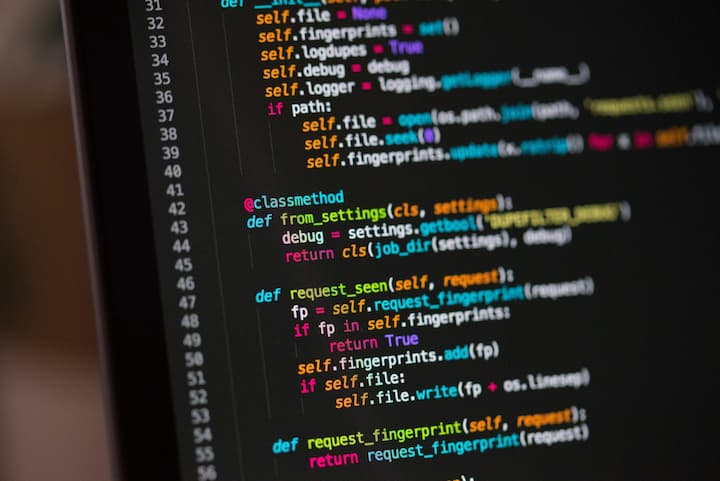
Conclusion
delegatecall
is a potent tool in Solidity, offering flexibility for creating upgradeable and modular contracts. However, it requires a deep understanding and cautious implementation to avoid potential pitfalls. By mastering delegatecall
, you can leverage its benefits while ensuring the security and efficiency of your smart contracts.
FAQs:
What is delegatecall in Solidity?
- delegatecall is a low-level function in Solidity that allows a contract to execute code from another contract while maintaining its own context (storage, msg.sender, etc.).
Why use delegatecall in Solidity?
- delegatecall is used for code reuse and to enable upgradable contracts by delegating function calls to different contract versions.
How does delegatecall differ from call in Solidity?
- While call changes the context to the target contract, delegatecall keeps the context of the calling contract, allowing access to its storage and state.
What are the risks associated with delegatecall?
- delegatecall can introduce security vulnerabilities if the called contract has malicious code or if storage layouts are not aligned properly.
How can you ensure delegatecall is secure?
- Ensure that the called contract is trusted, carefully manage storage layouts, and perform thorough testing and auditing to mitigate security risks.
Can delegatecall be used for contract upgrades?
- Yes, delegatecall is often used in proxy patterns to enable contract upgrades by directing calls to new implementations while preserving state.
What is the difference between staticcall and delegatecall?
- staticcall is used to call functions without modifying state, while delegatecall allows for state modification within the caller’s context.
What is a proxy contract in Solidity?
- A proxy contract delegates calls to another contract (implementation) using delegatecall, enabling contract logic upgrades while keeping the same address.
How do you test delegatecall functionality in Solidity?
- Testing delegatecall involves writing unit tests to check the correct execution of functions, ensuring storage layout alignment, and verifying expected behavior.
What is the significance of the fallback function in Solidity?
- The fallback function is a default function that handles calls to non-existent functions in a contract, often used in conjunction with delegatecall for proxies.