The lifeblood of Web3 applications often lies in tokens, and ERC token standards provide a common language for creating and interacting with these digital assets on the Ethereum blockchain. These standards, like the widely used ERC-20 for fungible tokens and ERC-721 for non-fungible tokens (NFTs), define a set of rules for token creation, transfer, and ownership. Understanding these standards is crucial for developers to ensure interoperability between their applications and different tokens within the Web3 ecosystem.
Understanding ERC Token Standards
Ethereum Request for Comments (ERC) token standards are protocols that define how tokens should function on the Ethereum blockchain. They ensure that tokens created by different developers adhere to the same rules, making them compatible with various applications, wallets, and exchanges. Let’s explore some of the key ERC standards:
ERC-20: The Fungible Token Standard
ERC-20 is the most widely adopted token standard on Ethereum. It defines a set of rules for creating fungible tokens, which are interchangeable with each other. For example, each ERC-20 token has the same value and properties as another token of the same type.
Key Functions of ERC-20 Tokens:
- totalSupply: Returns the total supply of tokens.
- balanceOf: Returns the balance of tokens for a specific address.
- transfer: Transfers tokens from the sender’s address to another address.
- approve: Approves another address to spend tokens on behalf of the sender.
- transferFrom: Transfers tokens from one address to another using the allowance mechanism.
- allowance: Returns the amount of tokens that an address is allowed to spend on behalf of another address.
Example Code:
pragma solidity ^0.8.0;
interface ERC20 {
function totalSupply() external view returns (uint256);
function balanceOf(address account) external view returns (uint256);
function transfer(address recipient, uint256 amount) external returns (bool);
function allowance(address owner, address spender) external view returns (uint256);
function approve(address spender, uint256 amount) external returns (bool);
function transferFrom(address sender, address recipient, uint256 amount) external returns (bool);
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed owner, address indexed spender, uint256 value);
}
contract MyToken is ERC20 {
string public constant name = "MyToken";
string public constant symbol = "MTK";
uint8 public constant decimals = 18;
uint256 private _totalSupply = 1000000 * 10 ** uint256(decimals);
mapping(address => uint256) private _balances;
mapping(address => mapping(address => uint256)) private _allowances;
constructor() {
_balances[msg.sender] = _totalSupply;
emit Transfer(address(0), msg.sender, _totalSupply);
}
function totalSupply() public view override returns (uint256) {
return _totalSupply;
}
function balanceOf(address account) public view override returns (uint256) {
return _balances[account];
}
function transfer(address recipient, uint256 amount) public override returns (bool) {
_balances[msg.sender] -= amount;
_balances[recipient] += amount;
emit Transfer(msg.sender, recipient, amount);
return true;
}
function allowance(address owner, address spender) public view override returns (uint256) {
return _allowances[owner][spender];
}
function approve(address spender, uint256 amount) public override returns (bool) {
_allowances[msg.sender][spender] = amount;
emit Approval(msg.sender, spender, amount);
return true;
}
function transferFrom(address sender, address recipient, uint256 amount) public override returns (bool) {
_balances[sender] -= amount;
_balances[recipient] += amount;
_allowances[sender][msg.sender] -= amount;
emit Transfer(sender, recipient, amount);
return true;
}
}
ERC-721: The Non-Fungible Token Standard
ERC-721 is the standard for creating non-fungible tokens (NFTs), which are unique and cannot be exchanged on a one-to-one basis with other tokens. Each ERC-721 token represents a distinct asset, such as digital art, collectibles, or real estate.
Key Functions of ERC-721 Tokens:
- balanceOf: Returns the number of NFTs owned by a specific address.
- ownerOf: Returns the owner of a specific NFT.
- safeTransferFrom: Safely transfers ownership of an NFT from one address to another.
- transferFrom: Transfers ownership of an NFT from one address to another.
- approve: Approves another address to transfer a specific NFT.
- setApprovalForAll: Approves or removes another address as an operator for the caller’s NFTs.
- getApproved: Returns the approved address for a specific NFT.
- isApprovedForAll: Returns whether an operator is approved for all of the owner’s NFTs.
Example Code:
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/utils/Counters.sol";
contract MyNFT is ERC721 {
using Counters for Counters.Counter;
Counters.Counter private _tokenIds;
constructor() ERC721("MyNFT", "MNFT") {}
function mintNFT(address recipient, string memory tokenURI) public returns (uint256) {
_tokenIds.increment();
uint256 newItemId = _tokenIds.current();
_mint(recipient, newItemId);
_setTokenURI(newItemId, tokenURI);
return newItemId;
}
}
ERC-1155: The Multi-Token Standard
ERC-1155 is a multi-token standard that allows for the creation of both fungible and non-fungible tokens within a single contract. This standard is highly efficient for applications requiring multiple token types, such as gaming platforms.
Key Features of ERC-1155:
- Batch Transfers: Enables batch transfers of multiple token types, reducing transaction costs.
- Atomic Swaps: Supports atomic swaps, ensuring that multiple operations are executed in a single transaction.
- Efficient Storage: Uses a single smart contract to manage multiple token types, saving storage space.
Example Code:
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol";
contract MyMultiToken is ERC1155 {
uint256 public constant GOLD = 0;
uint256 public constant SILVER = 1;
uint256 public constant THORS_HAMMER = 2;
constructor() ERC1155("<https://game.example/api/item/{id}.json>") {
_mint(msg.sender, GOLD, 1000, "");
_mint(msg.sender, SILVER, 1000, "");
_mint(msg.sender, THORS_HAMMER, 1, "");
}
}
ERC-20 Issues
The ERC-20 token standard has become the backbone of Web3 development, enabling the creation of fungible tokens on the Ethereum blockchain. However, while ERC-20 offers a foundation for innovation, it’s not without its limitations and potential issues. Let’s dive into some of the key ERC-20 Issues Web3 developers need to be aware of:
- Limited Functionality ERC-20 is designed for basic token transfers and lacks built-in features for functionalities like burning (permanent removal) or pausing token transfers. Developers need to implement custom logic or additional smart contracts to achieve these functionalities.
- Inconsistent Implementations While the standard defines core functionalities, there’s room for interpretation and variations in how different token contracts handle edge cases (e.g., receiving tokens to a contract not designed to hold them). This can lead to unexpected behavior and potential security vulnerabilities.
- The ‘Pull’ vs. ‘Push’ Conundrum ERC-20 relies on a “pull” mechanism, where the recipient initiates the transfer. This can be inefficient for scenarios requiring real-time updates or automated workflows.
- The Specter of Spam Transactions Low transaction fees can incentivize spam attacks, flooding the network with unnecessary token transfers and clogging the blockchain.
Real-World Examples
Here’s how these issues have manifested in the wild:
- The Parity Wallet Hack (2017): A flaw in how a wallet contract handled ERC-20 tokens with custom logic led to a vulnerability that attackers exploited to steal millions of dollars worth of tokens.
- Spam Attacks on Popular DEXes: Decentralized Exchanges (DEXes) have faced challenges with spam transactions from users attempting to manipulate token prices or disrupt trading activity.
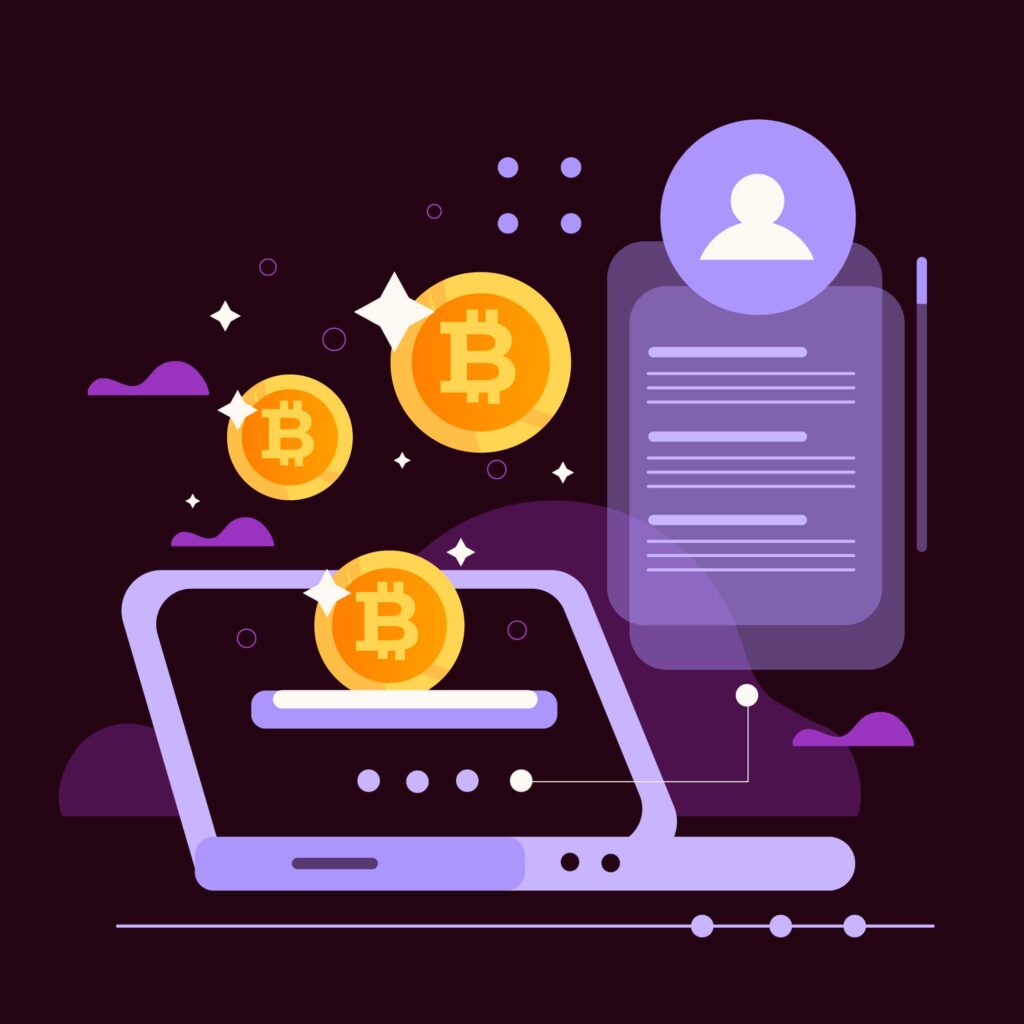
Mitigating the Risks
Web3 developers can address these issues through various strategies:
- Utilize Wrapper Contracts: For functionalities beyond basic transfers, consider creating wrapper contracts that interact with the ERC-20 token and provide additional features.
- Thorough Testing: Rigorously test smart contracts to identify potential inconsistencies or vulnerabilities arising from ERC-20 limitations.
- Exploring Alternatives: For specific use cases, evaluate alternative token standards (like ERC-777) that offer features like burning or “push” functionality.
- Community Collaboration: Advocate for improvements to the ERC-20 standard itself to address limitations and enhance its functionality for future use cases.
Conclusion: ERC Token Standards
Understanding ERC token standards is crucial for any developer working within the Ethereum ecosystem. These standards provide a consistent framework for creating and managing tokens, ensuring interoperability and ease of integration across different platforms and applications. While ERC-20 remains the most popular standard for fungible tokens, developers should be aware of its limitations and consider alternative standards like ERC-721 for NFTs and ERC-1155 for multi-token use cases.
By adhering to these standards and staying informed about their nuances and potential issues, developers can build robust and secure Web3 applications that leverage the full potential of blockchain technology. Whether you’re creating the next big NFT marketplace or a decentralized finance platform, a solid understanding of ERC token standards will be an invaluable asset in your developer toolkit.
FAQs:
What are ERC token standards?
- ERC token standards are predefined rules that Ethereum-based tokens follow to ensure compatibility and functionality within the Ethereum ecosystem.
What is the ERC-20 token standard?
- The ERC-20 token standard is a set of guidelines for creating fungible tokens that can be exchanged or traded on the Ethereum blockchain.
What distinguishes ERC-721 from ERC-20?
- Unlike ERC-20, which is for fungible tokens, ERC-721 is used for non-fungible tokens (NFTs), representing unique assets.
What is ERC-1155, and how is it different from other ERC standards?
- ERC-1155 is a multi-token standard allowing the creation of both fungible and non-fungible tokens within a single contract.
Why are ERC token standards important?
- ERC token standards ensure interoperability, security, and ease of development, making it simpler to create and manage tokens on the Ethereum blockchain.
How do ERC tokens impact the Ethereum ecosystem?
- ERC tokens enhance the functionality and versatility of the Ethereum network by enabling a wide range of applications, from decentralized finance (DeFi) to gaming.
What are the common uses of ERC-20 tokens?
- ERC-20 tokens are commonly used for Initial Coin Offerings (ICOs), DeFi applications, and as a means of transferring value on the Ethereum network.
Can ERC-721 tokens be used in gaming?
- Yes, ERC-721 tokens are popular in gaming for representing unique in-game items, characters, and collectibles.
How does ERC-1155 benefit developers?
- ERC-1155 offers developers flexibility and efficiency by allowing multiple token types in a single contract, reducing transaction costs and complexity.
What is tokenization, and how do ERC tokens facilitate it?
- Tokenization is the process of converting assets into digital tokens on a blockchain. ERC tokens provide the framework for securely creating and managing these digital assets.