In the realm of Web3, the concept of decentralization is not just a buzzword—it’s a fundamental principle. Decentralized Autonomous Organizations (DAOs) epitomize this ethos, where token holders collectively make decisions through voting. This setup empowers the community but also introduces a significant vulnerability: Governance Attacks.
Imagine a scenario where a group manipulates a democratic system for their own benefit. That’s essentially what a governance attack entails. Let’s delve into the mechanics, real-world examples, and strategies to safeguard against these threats.
Understanding Governance Attacks
Governance attacks are attempts to seize control of a DAO by manipulating its governance mechanisms. Here’s a step-by-step breakdown of how these attacks unfold:
The Power Grab
Attackers aim to amass voting power within a DAO. They can achieve this through legitimate means, such as buying a large number of tokens, or through malicious tactics, such as flash loan attacks. Flash loans allow attackers to borrow large sums of tokens without collateral, giving them temporary voting power.
Proposal Perfidy
Once the attackers have sufficient voting power, they can submit and pass malicious proposals designed to benefit themselves at the expense of the project. These proposals can take various forms:
- Draining the Treasury: Attackers siphon funds from the DAO’s treasury for personal gain.
- Self-Serving Parameter Changes: They alter protocol parameters to their advantage, which can harm the long-term health of the project.
- Rug Pull: After seizing control, attackers may completely dismantle the project, leaving investors empty-handed.
Real-World Wreckage
Governance attacks are not just theoretical—they have caused significant damage in the real world. Here are two notable examples:
The Beanstalk Fiasco (2022)
In 2022, the Beanstalk protocol fell victim to a flash loan attack. The attacker gained a majority stake and was able to siphon off $181 million. This incident highlighted the vulnerabilities in DAO governance and the need for more robust security measures.
The DAO Hack (2016)
One of the earliest and most infamous governance attacks occurred in 2016 with The DAO. A flaw in its code allowed a hacker to divert funds, causing a major setback for the early DAO movement. This attack underscored the importance of thorough code audits and security practices in decentralized projects.
Building a More Resilient DAO
To combat governance attacks, a multi-pronged approach is essential. Here are some strategies to enhance the resilience of DAOs:
- Tokenomics Design Carefully designing token distribution is crucial to prevent excessive concentration of power. This involves ensuring that no single entity or small group can easily amass enough tokens to control the DAO.
- Voting Mechanisms Exploring alternative voting mechanisms can help balance power within the DAO. One such mechanism is quadratic voting, which gives more weight to smaller token holders’ votes. This can prevent whales (large token holders) from dominating the decision-making process.
- Community Engagement An active and vigilant community is a strong defense against governance attacks. Encouraging community members to scrutinize proposals and flag suspicious activity can help detect and prevent malicious actions.
Multi-Sig Wallets
Implementing multi-signature wallets for critical actions adds an extra layer of security. These wallets require multiple parties to authorize transactions, making it more difficult for a single entity to execute a governance attack.
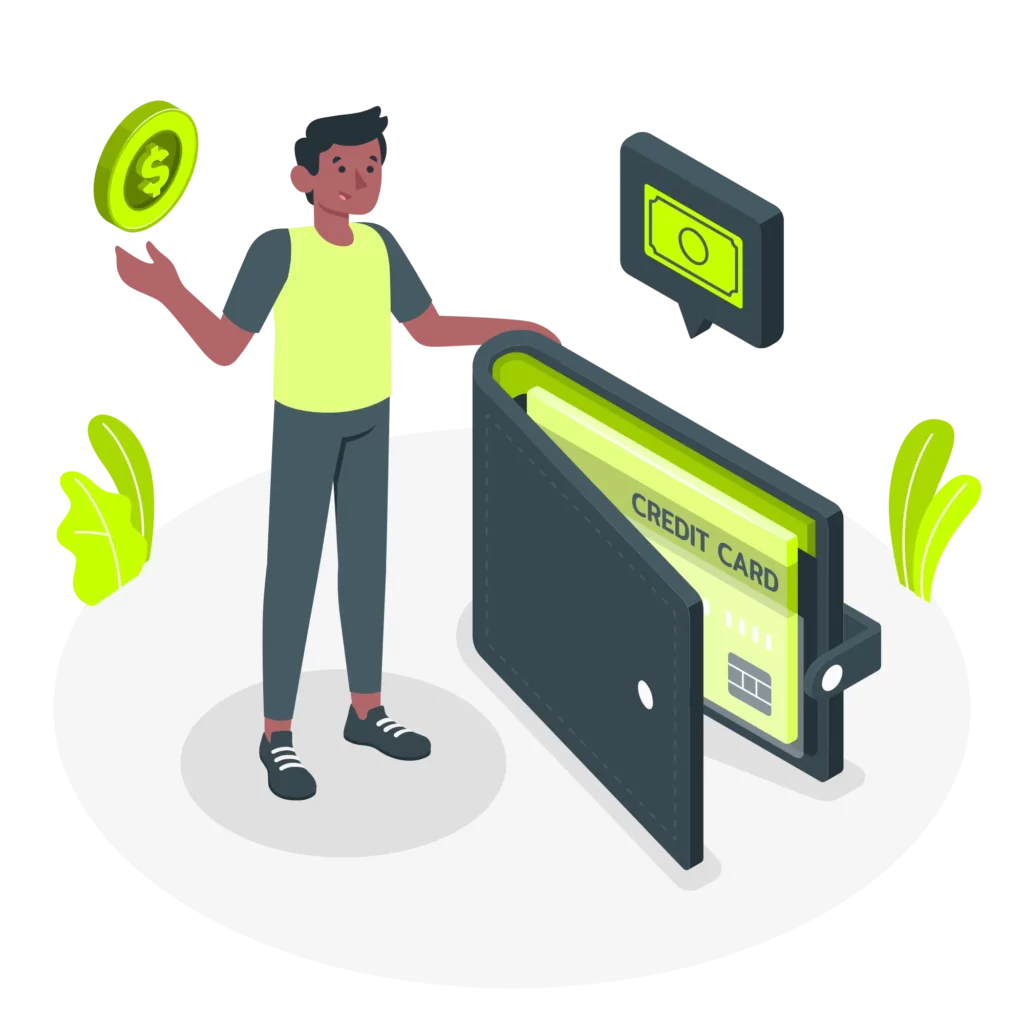
Example Code
To illustrate how some of these strategies can be implemented, let’s look at a basic example of a multi-signature wallet in Solidity. This example demonstrates how multiple parties can be required to approve a transaction before it is executed.
pragma solidity ^0.8.0;
We begin by setting the Solidity compiler version to 0.8.0 or higher.
contract MultiSigWallet {
address[] public owners;
mapping(address => bool) public isOwner;
uint public required;
struct Transaction {
address to;
uint value;
bytes data;
bool executed;
uint numConfirmations;
}
mapping(uint => mapping(address => bool)) public isConfirmed;
Transaction[] public transactions;
Here, we define the MultiSigWallet
contract with several key components:
owners
: An array of addresses that are authorized owners of the wallet.isOwner
: A mapping to check if an address is an owner.required
: The number of confirmations required to execute a transaction.Transaction
: A struct to represent a transaction, including the recipient (to
), the amount (value
), data to be sent (data
), a flag indicating execution status (executed
), and the number of confirmations (numConfirmations
).isConfirmed
: A nested mapping to track which owners have confirmed a transaction.transactions
: An array to store all proposed transactions.
modifier onlyOwner() {
require(isOwner[msg.sender], "Not an owner");
_;
}
modifier txExists(uint _txIndex) {
require(_txIndex < transactions.length, "Transaction does not exist");
_;
}
modifier notExecuted(uint _txIndex) {
require(!transactions[_txIndex].executed, "Transaction already executed");
_;
}
modifier notConfirmed(uint _txIndex) {
require(!isConfirmed[_txIndex][msg.sender], "Transaction already confirmed");
_;
}
Modifiers are used to apply common checks:
onlyOwner
: Ensures the function caller is an owner.txExists
: Ensures the transaction exists.notExecuted
: Ensures the transaction has not already been executed.notConfirmed
: Ensures the transaction has not already been confirmed by the caller.
event Deposit(address indexed sender, uint amount);
event SubmitTransaction(
address indexed owner,
uint indexed txIndex,
address indexed to,
uint value,
bytes data
);
event ConfirmTransaction(address indexed owner, uint indexed txIndex);
event RevokeConfirmation(address indexed owner, uint indexed txIndex);
event ExecuteTransaction(address indexed owner, uint indexed txIndex);
Events are declared to log activities on the blockchain:
Deposit
: Logs when ether is deposited into the wallet.SubmitTransaction
: Logs when a transaction is submitted.ConfirmTransaction
: Logs when a transaction is confirmed.RevokeConfirmation
: Logs when a confirmation is revoked.ExecuteTransaction
: Logs when a transaction is executed.
constructor(address[] memory _owners, uint _required) {
require(_owners.length > 0, "Owners required");
require(
_required > 0 && _required <= _owners.length,
"Invalid required number of owners"
);
for (uint i = 0; i < _owners.length; i++) {
address owner = _owners[i];
require(owner != address(0), "Invalid owner");
require(!isOwner[owner], "Owner not unique");
isOwner[owner] = true;
owners.push(owner);
}
required = _required;
}
The constructor initializes the contract:
- It takes an array of owner addresses and the required number of confirmations.
- It ensures there are owners and the required number is valid.
- It sets the owner addresses and marks them as valid owners.
receive() external payable {
emit Deposit(msg.sender, msg.value);
}
The receive
function allows the contract to receive ether and logs the deposit.
function submitTransaction(
address _to,
uint _value,
bytes memory _data
)
public
onlyOwner
{
uint txIndex = transactions.length;
transactions.push(Transaction({
to: _to,
value: _value,
data: _data,
executed: false,
numConfirmations: 0
}));
emit SubmitTransaction(msg.sender, txIndex, _to, _value, _data);
}
The submitTransaction
function allows an owner to propose a new transaction:
- It takes the recipient address (
_to
), amount (_value
), and data (_data
). - It creates a new transaction and adds it to the transactions array.
- It logs the submission event.
function confirmTransaction(uint _txIndex)
public
onlyOwner
txExists(_txIndex)
notExecuted(_txIndex)
notConfirmed(_txIndex)
{
Transaction storage transaction = transactions[_txIndex];
transaction.numConfirmations += 1;
isConfirmed[_txIndex][msg.sender] = true;
emit ConfirmTransaction(msg.sender, _txIndex);
}
The confirmTransaction
function allows an owner to confirm a proposed transaction:
- It checks that the transaction exists, has not been executed, and has not been confirmed by the caller.
- It increments the number of confirmations and marks the transaction as confirmed by the caller.
- It logs the confirmation event.
function executeTransaction(uint _txIndex)
public
onlyOwner
txExists(_txIndex)
notExecuted(_txIndex)
{
Transaction storage transaction = transactions[_txIndex];
require(
transaction.numConfirmations >= required,
"Cannot execute transaction"
);
transaction.executed = true;
(bool success, ) = transaction.to.call{value: transaction.value}(
transaction.data
);
require(success, "Transaction failed");
emit ExecuteTransaction(msg.sender, _txIndex);
}
The executeTransaction
function allows an owner to execute a confirmed transaction:
- It checks that the transaction exists, has not been executed, and has enough confirmations.
- It sets the transaction as executed and attempts to send the funds and data.
- It logs the execution event.
function revokeConfirmation(uint _txIndex)
public
onlyOwner
txExists(_txIndex)
notExecuted(_txIndex)
{
Transaction storage transaction = transactions[_txIndex];
require(isConfirmed[_txIndex][msg.sender], "Transaction not confirmed");
transaction.numConfirmations -= 1;
isConfirmed[_txIndex][msg.sender] = false;
emit RevokeConfirmation(msg.sender, _txIndex);
}
}
The revokeConfirmation
function allows an owner to revoke their confirmation of a transaction:
- It checks that the transaction exists, has not been executed, and has been confirmed by the caller.
- It decrements the number of confirmations and unmarks the transaction as confirmed by the caller.
- It logs the revocation event.
Conclusion: Governance Attack
Governance attacks represent a significant threat to the integrity and success of DAOs. By understanding how these attacks work and implementing robust defenses, the Web3 community can build more resilient decentralized systems. Tokenomics design, innovative voting mechanisms, active community engagement, and multi-signature wallets are all vital components in this defense strategy. As the space continues to evolve, staying vigilant and adaptive will be key to ensuring the security and longevity of decentralized governance.
FAQs:
What are governance attacks in smart contracts?
- Governance attacks exploit vulnerabilities in the decision-making processes of decentralized systems, leading to unauthorized control or manipulation.
How do governance attacks impact blockchain security?
- These attacks can compromise the integrity and trust of a blockchain network, resulting in financial losses and damaged reputations.
What are common methods of governance attacks?
- Common methods include exploiting voting mechanisms, manipulating consensus protocols, and taking advantage of poorly designed governance frameworks.
How can governance attacks be prevented?
- Implementing robust security measures, regular audits, decentralized voting systems, and thorough testing of governance frameworks can help prevent these attacks.
What role do smart contracts play in governance attacks?
- Smart contracts automate governance processes, and if they have vulnerabilities, they can be exploited to execute malicious activities within the blockchain.
What are some examples of governance attacks in DeFi?
- Notable examples include the DAO attack and the Beanstalk governance attack, both of which resulted in significant financial losses.
How can blockchain communities improve governance security?
- By adopting transparent governance models, conducting frequent security audits, and fostering community involvement in decision-making.
What is the significance of decentralized voting in preventing governance attacks?
- Decentralized voting ensures that no single entity can easily manipulate the governance process, promoting fairness and security.
Why is regular auditing important for smart contract governance?
- Regular auditing helps identify and fix vulnerabilities before they can be exploited, maintaining the integrity of the governance system.
How do malicious actors exploit smart contract vulnerabilities?
- Malicious actors may use tactics like code injection, Sybil attacks, or exploiting logic flaws within the smart contract to gain unauthorized control or access.