Web3 security relies heavily on digital signatures to verify the authenticity and authorization of transactions. However, a vulnerability known as signature malleability can pose a significant threat. This allows attackers to manipulate signatures in subtle ways, potentially bypassing security checks. These malleable signatures could be reused for unauthorized transactions or even entirely forged, jeopardizing user funds and eroding trust in the Web3 ecosystem. Developers must prioritize robust signature verification mechanisms and utilize libraries and practices that address these malleability risks.
Let’s explore how Signatures and Malleability impact Web3 security.
Improper Signature Verification
Web3 applications rely heavily on cryptography to ensure secure transactions and user authentication. A critical aspect of this security is Signature Verification. When done incorrectly, it can lead to devastating consequences, allowing unauthorized access to funds and eroding trust in the entire ecosystem. Let’s dive into the risks of improper signature verification and how developers can safeguard their projects.
The Guardian at the Gate (or Not)
Imagine a user interacting with a Web3 wallet. To approve a transaction, they sign a message with their private key. This signature acts as a digital fingerprint, proving their ownership and authorization. The onus falls on the Web3 application to verify this signature before executing the transaction.
Improper signature verification can occur in several ways:
- Incomplete Verification: The application might only verify a portion of the signature, leaving it vulnerable to manipulation by attackers who can forge a valid-looking signature for their malicious purposes.
- Improper Key Management: If the application stores or transmits private keys insecurely, attackers who gain access can forge signatures and steal user funds.
- Custom Verification Logic: In an attempt to optimize performance, developers might implement their own signature verification logic instead of relying on established cryptographic libraries. This introduces the risk of errors or vulnerabilities in the custom code.
Real-World Reputations Tarnished:
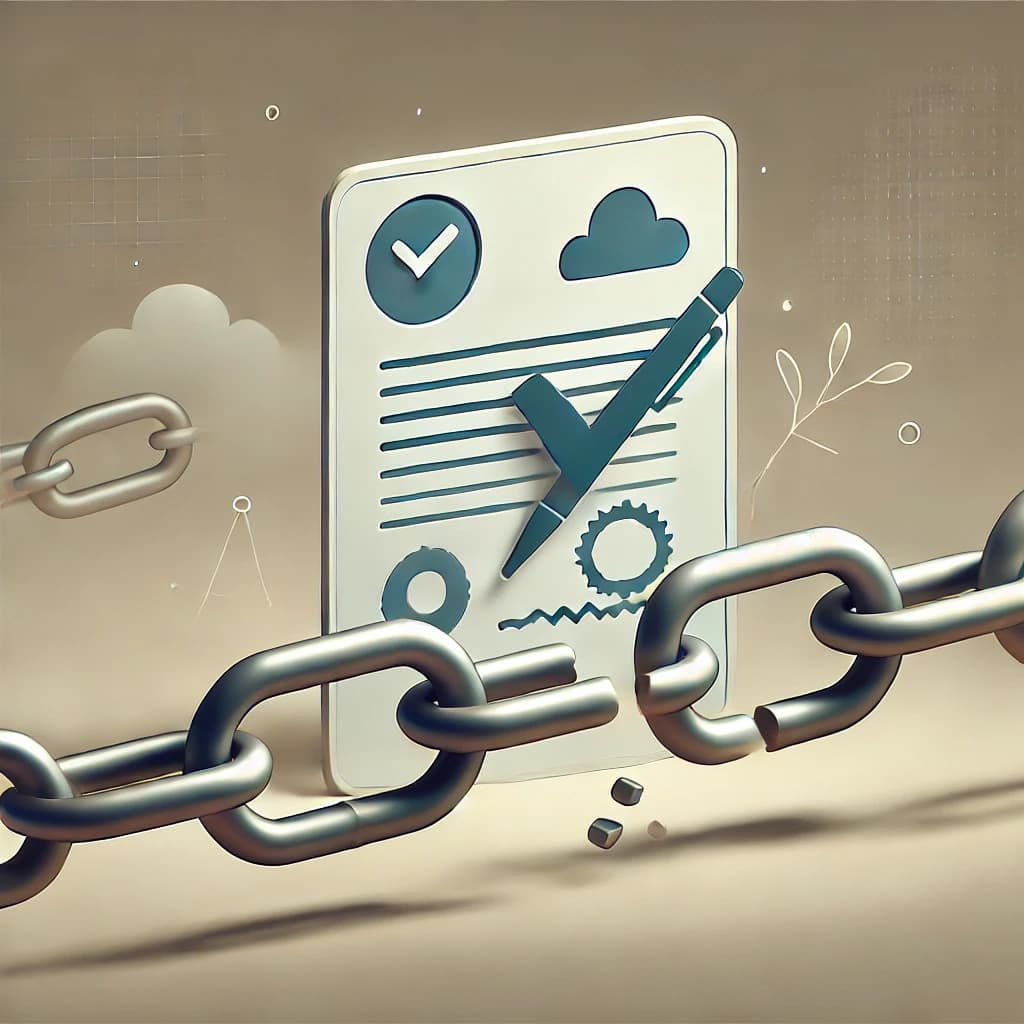
Improper signature verification has led to serious breaches in Web3:
- The Parity Multisig Hack (2017): A flaw in the signature verification process of a popular multi-signature wallet contract allowed attackers to steal millions of dollars worth of Ether from user accounts.
- The Lazarus Group Attacks (2020): A hacking group targeted DeFi protocols with vulnerabilities in their signature verification, enabling them to steal cryptocurrency from users’ wallets.
Building a Wall of Defense:
Web3 developers can fortify their applications against these threats through:
- Leveraging Cryptographic Libraries: Utilize well-tested and secure cryptographic libraries for signature generation and verification. These libraries handle the complex cryptographic operations and offer robust implementations.
- Thorough Code Audits: Conduct rigorous security audits that specifically scrutinize the signature verification process to identify and address potential vulnerabilities.
- Secure Key Management: Implement secure practices for private key storage and transmission. This might involve hardware security modules or multi-party computation techniques.
Signature Malleability
Web3 thrives on the power of cryptography, and digital signatures play a crucial role in ensuring the authenticity and integrity of transactions. However, a lesser-known vulnerability lurks within – Signature Malleability. This technical quirk allows attackers to manipulate signatures in specific ways, potentially bypassing security checks and compromising user funds. Let’s explore how signature malleability works and how developers can mitigate its risks.
The Art of Deception
Imagine a user signing a transaction on a Web3 platform. The platform generates a digital signature based on the transaction details and the user’s private key. This signature acts as a cryptographic seal of approval. But what if this signature could be subtly altered without affecting its validity?
Signature malleability allows attackers to do just that:
- Reusing Signatures: An attacker might exploit a malleable signature to “replay” it for a different transaction with a slightly modified value. This could enable them to steal funds by tricking the platform into processing a transaction the user never intended.
- Bypassing Verification: In some cases, malleability can be used to create new signatures that validate as authentic even though they weren’t generated with the intended private key. This could allow attackers to forge signatures entirely and steal user funds.
Real-World Robberies
Signature malleability has been exploited in real-world attacks:
- The Ethereum Parity Wallet Hack (2017): A vulnerability in the Parity wallet software allowed attackers to leverage signature malleability to steal millions of dollars worth of Ether from user accounts.
- The Bitcoin Transaction Malleability Debate (2011-2014): The Bitcoin community grappled with the implications of signature malleability, leading to protocol updates to mitigate these risks.
Securing the Signature Realm
Web3 developers can safeguard their applications against signature malleability through several strategies:
- Enforce Canonical Encoding: Ensure consistent encoding of transaction data across all parts of the application. This reduces the chances of attackers generating unintended malleable variants of the signature.
- Upgrade Cryptographic Libraries: Stay updated with the latest cryptographic libraries that address known signature malleability vulnerabilities.
- Digital Signature Algorithms with Fixed Length: Utilize digital signature algorithms that produce signatures with a fixed length, making them more resistant to manipulation.
Example Code: Handling Signatures and Malleability
To give a more practical understanding, let’s dive into some example code using JavaScript and Solidity to handle signatures and address malleability.
JavaScript Example: Using Ethers.js for Signature Verification
First, we’ll use the ethers.js
library to demonstrate proper signature generation and verification in JavaScript.
Installing Ethers.js
You can install the ethers.js library using npm:
npm install ethers
Signature Generation and Verification
Here’s a complete example demonstrating how to sign a message and verify the signature using ethers.js:
const { ethers } = require("ethers");
// Generate a random wallet (private key and public address)
const wallet = ethers.Wallet.createRandom();
// Message to be signed
const message = "This is a message to be signed";
// Hash the message
const messageHash = ethers.utils.id(message);
// Sign the message
const signature = wallet.signMessage(ethers.utils.arrayify(messageHash));
// Verify the signature
const recoveredAddress = ethers.utils.verifyMessage(message, signature);
// Check if the recovered address matches the signer's address
if (recoveredAddress === wallet.address) {
console.log("Signature is valid and matches the signer's address");
} else {
console.log("Signature is invalid or does not match the signer's address");
}
Solidity Example: Signature Verification on Ethereum
In Solidity, verifying a signature requires using the ecrecover
function. Here’s an example of a smart contract that verifies a signature:
pragma solidity ^0.8.0;
contract SignatureVerifier {
function getMessageHash(string memory message) public pure returns (bytes32) {
return keccak256(abi.encodePacked(message));
}
function verify(
address signer,
string memory message,
bytes memory signature
) public pure returns (bool) {
bytes32 messageHash = getMessageHash(message);
bytes32 ethSignedMessageHash = getEthSignedMessageHash(messageHash);
return recoverSigner(ethSignedMessageHash, signature) == signer;
}
function getEthSignedMessageHash(bytes32 messageHash) public pure returns (bytes32) {
// Ethereum Signed Message: "\\\\x19Ethereum Signed Message:\\\\n32" + messageHash
return keccak256(abi.encodePacked("\\\\x19Ethereum Signed Message:\\\\n32", messageHash));
}
function recoverSigner(bytes32 ethSignedMessageHash, bytes memory signature) public pure returns (address) {
(bytes32 r, bytes32 s, uint8 v) = splitSignature(signature);
return ecrecover(ethSignedMessageHash, v, r, s);
}
function splitSignature(bytes memory sig)
public
pure
returns (bytes32 r, bytes32 s, uint8 v)
{
require(sig.length == 65, "invalid signature length");
assembly {
r := mload(add(sig, 32))
s := mload(add(sig, 64))
v := byte(0, mload(add(sig, 96)))
}
}
}
The above example comprises several functions to handle signature verification: getMessageHash computes the keccak256 hash of the message; verify checks that the signature was generated by the signer’s address; getEthSignedMessageHash prefixes the message hash with the Ethereum Signed Message prefix and hashes it again to prevent signature replay attacks across different networks; recoverSigner uses ecrecover
to retrieve the signer’s address from the signature; and splitSignature divides the signature into r
, s
, and v
components, essential for the ecrecover
function.
Conclusion
Proper signature verification and handling of signature malleability are crucial for the security of Web3 applications. Developers must use well-tested cryptographic libraries, conduct thorough code audits, and implement secure key management practices. By understanding and mitigating the risks associated with improper signature verification and signature malleability, developers can protect user funds and maintain trust in the Web3 ecosystem. The provided examples in JavaScript and Solidity demonstrate practical implementations of these concepts, helping developers to build more secure applications.
FAQs
What are digital signatures in Web3?
- Digital signatures in Web3 are cryptographic proofs that verify the authenticity and integrity of blockchain transactions.
How does malleability affect blockchain transactions?
- Malleability allows an attacker to alter the transaction ID without changing its content, potentially causing issues like double-spending.
What is transaction malleability?
- Transaction malleability is the ability to modify the unique identifier (hash) of a blockchain transaction after it has been signed.
How can signatures enhance Web3 security?
- Signatures verify that transactions are authorized by the owner of the corresponding private key, ensuring authenticity and preventing fraud.
What measures can prevent transaction malleability?
- Using Segregated Witness (SegWit), upgrading to malleability-resistant signature schemes, and implementing strong protocol rules can prevent transaction malleability.
Why is transaction integrity crucial in blockchain security?
- Maintaining transaction integrity ensures that data has not been tampered with, preserving trust and security in the blockchain network.
What is Segregated Witness (SegWit)?
- SegWit is a protocol upgrade that reduces transaction malleability by separating signature data from transaction data, enhancing blockchain efficiency and security.
How do cryptographic signatures work?
- Cryptographic signatures use algorithms to create a unique digital fingerprint of a transaction, which can be verified but not forged.
Can transaction malleability be completely eliminated?
- While difficult to eliminate completely, implementing robust security practices and protocol upgrades can significantly reduce the risk of transaction malleability.
What are the risks of ignoring transaction malleability in Web3?
- Ignoring transaction malleability can lead to security vulnerabilities like double-spending attacks, loss of funds, and decreased trust in the blockchain network.