JavaScript and Solidity are both high-level programming languages widely used in their respective domains. While JavaScript dominates the web development world, Solidity is the most prominent language for writing smart contracts on the Ethereum blockchain. Despite their distinct purposes, these two languages share several similarities that make learning one advantageous for understanding the other.
How similar are JavaScript and Solidity? This article provides an in-depth exploration of the similarities between JavaScript and Solidity and highlights how learning one can aid in understanding the other. However, it’s crucial to note that while these languages share certain features, their underlying purposes differ significantly.
Understand Javascript and Solidity
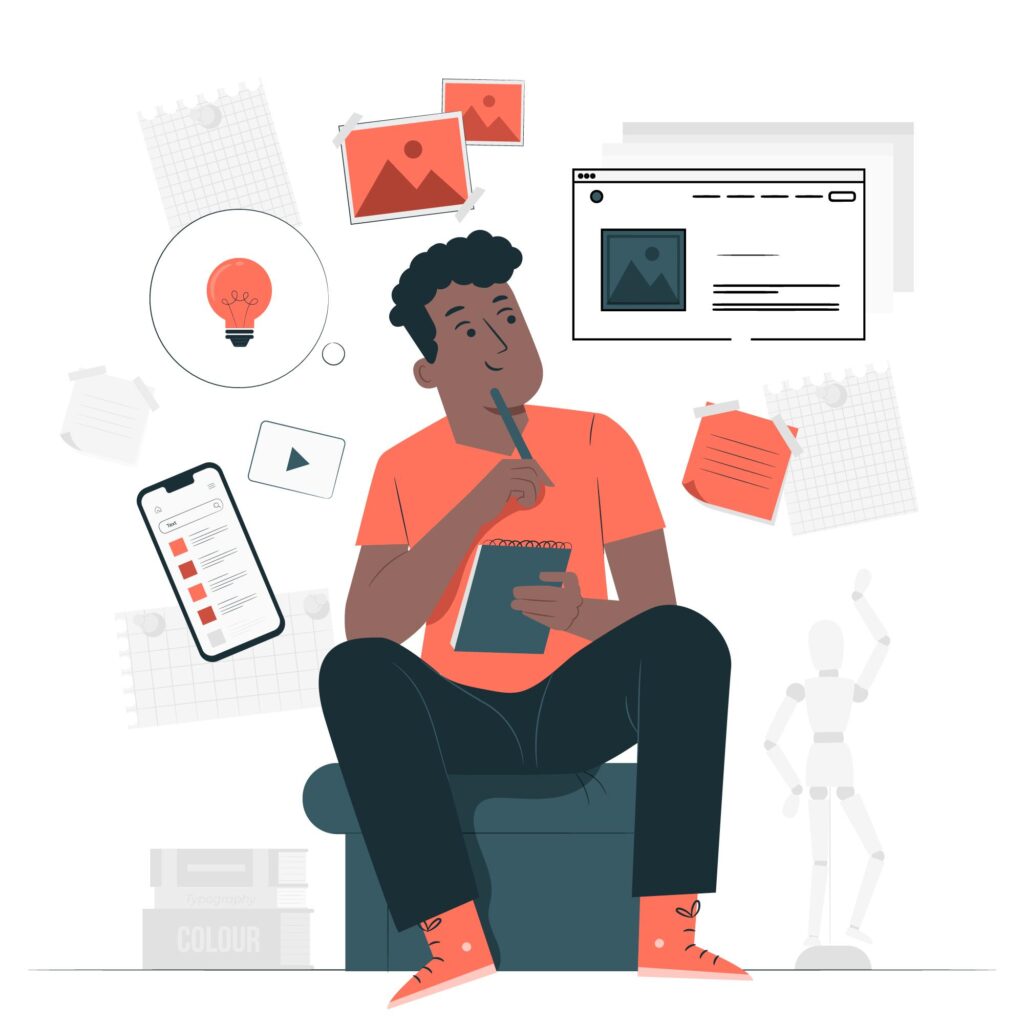
Before diving into their similarities, it’s important to first understand what each language is and its core purpose within its respective field.
What is JavaScript ?
JavaScript is a high-level, dynamic programming language primarily used to create interactive and dynamic elements on websites. Originally developed in 1995 by Brendan Eich at Netscape, JavaScript has evolved significantly. It is one of the core technologies of web development. It enables developers to build rich, engaging user interfaces by adding functionality like animations, form validation, content updates without reloading the page, and handling user interactions.
What is Solidity ?
Solidity is a high-level, statically-typed programming language designed specifically for writing smart contracts on blockchain platforms, most notably Ethereum. Solidity was developed by Gavin Wood, Christian Reitwiessner, and others as part of the Ethereum project in 2014. It is influenced by several other programming languages like JavaScript, Python, and C++, making it relatively easy to learn for developers familiar with these languages.
Similarities between Javascript and Solidity
Now that we have a foundational understanding of what these languages are and their primary uses, let’s explore the similarities between them. These similarities will help illustrate how learning one can facilitate understanding the other more easily.
1. Syntax and Structure
Both JavaScript and Solidity share a similar syntax, which makes the code visually familiar for developers experienced in either language. This will assist developers in learning one language more efficiently if they are already experienced with the other. Below are some elements where the syntax appears similar.
- Curly Braces
{}
- Used for block definitions.
- Functions
- Functions are defined in a similar manner on both languages.
- Semicolons
;
- The semicolon is optional in Javascript but required in Solidity to terminate statements.
- Variable Declaration
- Declaring variables use similar syntax in both languages. However, Solidity required type declaration as well.
Example Code in Javascript
function sayHello() {
let greeting = "Hello";
console.log(greeting);
}
Example Code in Solidity
function sayHello() public {
string greeting = "Hello";
// logic to print the greeting
}
2. Data Types
Data types are classifications of data that tell the compiler or interpreter how to interpret and manipulate the data. Different programming languages have different sets of data types, but they generally fall into several broad categories. The following primitive data types are similar in Javascript and Solidity.
- string
- Represented as
string
in both.
- Represented as
- bool
- Represented as
bool
in Solidity andboolean
in
Javascript.
- Represented as
- Integers
- In JavaScript, integers are a subtype of
Number
, while Solidity has more specific types likeuint
(unsigned integers) andint
(signed integers) of different sizes (e.g.,uint256
,int128
).
- In JavaScript, integers are a subtype of
Example Code in Javascript
let count = 42;
let isTrue = true;
let greeting = "Hello";
Example Code in Solidity
uint count = 42;
bool isTrue = true;
string greeting = "Hello";
3. Control Structures
Solidity and Javascript have similar control structures for conditional statements and loops. The shared fundamental programming concepts between both languages facilitate a deeper understanding of each when experienced with the other.
Example Code in Javascript
if (x > 10) {
// Javascript Logic
}
for(let i = 0; i < 10; i++) {
// Javascript Logic
}
Example Code in Solidity
if (x > 10) {
// Solidity logic
}
for (uint256 i = 0; i < 10; i++) {
// Solidity Logic
}
4. Functions
Functions in JavaScript and Solidity share similarities in their syntax. Both languages allow defining functions that can accept parameters and return values. However in Solidity, functions can have more explicit visibility modifiers such as public
, private
, external
, and internal
.
Example Code in Javascript
function count(number) {
// Javascript Logic
}
Example Code in Solidity
function count(uint256 number) public {
// Solidity Logic
}
5. Object Oriented Features
Both JavaScript and Solidity support object-oriented programming principles. While JavaScript achieves OOP through prototypes and the class
syntax, Solidity employs OOP more traditionally, enabling developers to define custom types and encapsulate behavior within smart contracts.
- JavaScript
- Classes allow you to define blueprints for creating objects.
- Solidity
- Contracts are similar to classes, where you define state variables and functions to manage and manipulate those variables.
Example Code in Javascript
// JavaScript Class
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
getDetails() {
return `${this.make} ${this.model}`;
}
}
Example Code in Solidity
// Solidity Contract
contract Car {
string public make;
string public model;
constructor(string memory _make, string memory _model) {
make = _make;
model = _model;
}
function getDetails() public view returns (string memory) {
return string(abi.encodePacked(make, " ", model));
}
}
6. Event Handling
While JavaScript handles DOM events like clicks and keypresses, Solidity also has an event system used to trigger logging and communicate with external applications. Solidity’s events are similar in concept to event emitters in JavaScript.
Example Code in Javascript
document.addEventListener("click", function() {
console.log("Clicked!");
});
Example Code in Solidity
event DataStored(uint indexed data);
function storeData(uint data) public {
emit DataStored(data);
}
7. Error Handling
Error handling is a crucial aspect of development, and both Solidity and JavaScript offer robust mechanisms for managing errors effectively. In JavaScript, try-catch
is used to handle exceptions that occur during code execution while in Solidity the require
, assert
, and revert
functions are used to handle errors and revert transactions if something goes wrong.
Example Code in Javascript
try {
// Code that may throw an error
} catch (error) {
console.log(error.message);
}
Example Code in Solidity
function withdraw(uint amount) public {
// require(code that may throw an error, Error message)
require(amount <= balance, "Insufficient balance");
// decrement balance only when balance is sufficient.
balance -= amount;
}
8. Comments
Comments are essential for enhancing the development experience, as they provide clarity and documentation within the code. Both JavaScript and Solidity support single-line and multi-line comments using the same syntax, making it easier for developers familiar with one language to apply similar practices in the other.
Example Code in Javascript
// This is a single-line comment
/* This is a
multi-line comment */
Example Code in Solidity
// This is a single-line comment
/* This is a
multi-line comment */
What are the Differences ? Javascript vs Solidity
While Solidity and JavaScript share several similarities in their syntax and structure, their core purposes are quite different. The following table will elucidate the key differences between JavaScript and Solidity.
Javascript | Solidity | |
Primary Use Case | Primarily designed for web development, powering dynamic websites and server-side applications. | Primarily designed for writing smart contracts that run on blockchain platforms like Ethereum |
Execution Environment | Runs on browsers or in a server environments using Node.js | Runs on the Ethereum Virtual Machine (EVM) executing code on the decentralized blockchain. |
State Management | Has state variables that are managed on local state in-memory while the code runs. Data persists only during execution. | Has state variables that are permanently stored on the blockchain. These variables are accessed and modified by functions in smart contracts |
Transaction Costs | Code execution is free with no additional costs except for computing resources. Users don’t have to pay for every function call. | Executing smart contracts on the blockchain required gas costs. |
Immutability | Code is mutable, and easy to update. It allows version updates without deploying a completely new application. | After deployment, smart contracts are immutable, which means they cannot be changed unless specific mechanisms are in place. |
Type Safety | Dynamically Typed language which means variables can hold any type of data and their types are determined at runtime. | Statically Typed language which means the variables must have declared types at compile time. |
Conclusion
JavaScript and Solidity share several similarities in syntax and structure, which can ease the learning process for developers transitioning between the two. However, they differ significantly in terms of their primary use cases. If you’re a JavaScript developer looking to explore blockchain development, learning Solidity may feel familiar and intuitive. Although Solidity introduces blockchain-specific concepts such as smart contracts, Ethereum addresses, and gas fees, your existing knowledge of JavaScript will make the transition smoother. Understanding these similarities will allow you to leverage your skills effectively across both domains, expanding your capabilities in both web and blockchain development.
FAQs:
What are the main similarities between JavaScript and Solidity?
- Both JavaScript and Solidity have similar syntax, especially in control structures like loops and conditionals, making them easier to learn for developers familiar with JavaScript.
What is Solidity mainly used for?
- Solidity is primarily used for writing smart contracts on the Ethereum blockchain, enabling decentralized applications (dApps).
Can JavaScript be used for blockchain development like Solidity?
- JavaScript can interact with blockchain networks but cannot create smart contracts. Solidity is specifically designed for Ethereum smart contracts.
Which language is easier to learn: JavaScript or Solidity?
- JavaScript is more accessible due to its widespread use and simpler environment setup, while Solidity requires knowledge of blockchain technology and smart contracts.
Is Solidity based on JavaScript?
- Solidity is inspired by JavaScript in terms of syntax but also draws from other languages like C++ and Python, with a focus on blockchain use cases.