When working with callback in JavaScript, is one of the most common challenges developers face is understanding the behavior of this, especially inside callbacks. Misusing or misinterpreting this can lead to bugs that are both difficult to find and fix. This comprehensive guide will demystify the concept of this in callbacks, showing you how to ensure that this always refers to the object or context you expect.
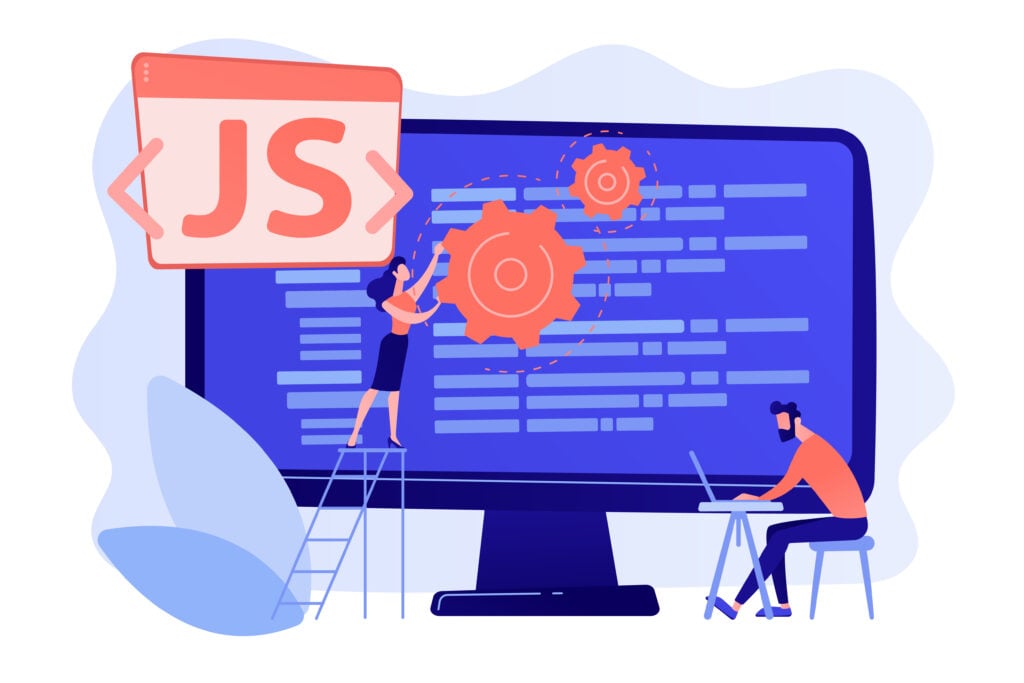
TL;DR
Mastering this in callbacks is key to avoiding bugs. The value of this depends on how and where a function is invoked. Common issues include losing context in callbacks or nested functions. Solutions include:
- Arrow functions: Inherit this from the surrounding scope.
- bind(): Explicitly bind this to a function.
- call/apply: Invoke functions with a specific this.
- self/that: Store this in a variable for reference.
Best practices favor modern solutions like arrow functions and a solid understanding of execution context. By mastering this, you’ll write cleaner, more reliable code. FAQs cover bind, call, apply differences, and debugging tips. reliable code. FAQs cover bind
, call
, apply
differences, and debugging tips.
What is this
in JavaScript?
In JavaScript, this
refers to the context in which the current code is executing, and its value depends on where and how a function is invoked. For instance, in the global context, this
points to the window
object in browsers. Within an object method, this
refers to the object that owns the method. Inside a function, strict mode causes this
to be undefined
, while non-strict mode makes it default to the global object. However, understanding this in callbacks is essential, as the behavior of this often depends on the function’s invocation style.
Common Issues with this
in Callbacks
Problem 1: Loss of Context
When you pass a method as a callback in JavaScript, the this reference inside that method might not refer to the original object anymore:
const person = {
name: "John",
greet() {
console.log(`Hello, my name is ${this.name}`);
}
};
setTimeout(person.greet, 1000); // Output: Hello, my name is undefined
In this example, the this
inside greet
no longer refers to the person
object but instead defaults to the global object (window
in browsers or undefined
in strict mode).
Problem 2: Nested Functions
When using nested functions, this
in the inner function doesn’t inherit the context of the outer function.
const person = {
name: "John",
greet() {
setTimeout(function() {
console.log(`Hello, my name is ${this.name}`);
}, 1000);
}
};
person.greet(); // Output: Hello, my name is undefined
Solutions to Access the Correct this
1. Use Arrow Functions
Arrow functions don’t have their own this
. Instead, they inherit this
from the surrounding lexical scope.
const person = {
name: "John",
greet() {
setTimeout(() => {
console.log(`Hello, my name is ${this.name}`);
}, 1000);
}
};
person.greet(); // Output: Hello, my name is John
This is the most common and straightforward solution for callbacks.
2. Bind this
Explicitly
You can use the bind
method to explicitly set the value of this
when passing a function as a callback.
const person = {
name: "John",
greet() {
setTimeout(this.greet.bind(this), 1000);
}
};
person.greet(); // Output: Hello, my name is John
The bind
method creates a new function with this
permanently set to the specified value.
3. Use self
or that
Variables
Before ES6 introduced arrow functions, a common workaround was to store the value of this
in a variable like self
or that
.
const person = {
name: "John",
greet() {
const self = this;
setTimeout(function() {
console.log(`Hello, my name is ${self.name}`);
}, 1000);
}
};
person.greet(); // Output: Hello, my name is John
While this method works, it’s generally considered less elegant compared to arrow functions.
4. Use call
or apply
Methods
You can use call
or apply
to invoke the callback immediately with a specific this
value.
const person = {
name: "John",
greet() {
setTimeout(function() {
console.log(`Hello, my name is ${this.name}`);
}.call(this), 1000);
}
};
person.greet(); // Output: Hello, my name is John
Note: This method doesn’t allow for asynchronous execution in the same way as bind
or arrow functions
5. Using Classes and Instance Methods
When working with classes, ensure you use instance methods and bind them correctly.
class Person {
constructor(name) {
this.name = name;
}
greet() {
setTimeout(() => {
console.log(`Hello, my name is ${this.name}`);
}, 1000);
}
}
const person = new Person("John");
person.greet(); // Output: Hello, my name is John
Best Practices for Managing this
- Prefer Arrow Functions: They provide a clean and modern solution for maintaining the correct
this
. - Use
bind
Sparingly: While effective, creating new bound functions can have memory and performance implications. - Understand the Execution Context: These are considered outdated solutions.
- Test in Strict Mode: Always test your code in strict mode to catch issues with
this
early. - Understand the Execution Context: Familiarize yourself with how
this
behaves in different contexts to avoid unexpected results.
By understanding and applying these solutions, you can confidently handle this in callbacks and write cleaner, more reliable JavaScript code. Mastering these techniques will help you avoid common pitfalls and make debugging easier.
FAQs
1. What is the difference between bind
, call
, and apply
?
bind
: Returns a new function withthis
permanently set to the provided value.call
: Invokes a function with a specifiedthis
value and arguments passed individually.apply
: Similar tocall
, but arguments are passed as an array.
2. Why does this
behave differently in arrow functions?
Arrow functions don’t have their own this
. They inherit this
from their surrounding lexical scope, making them ideal for callbacks.
3. Can I use arrow functions everywhere to fix this
?
Not always. Arrow functions aren’t suitable for object methods or constructors, as they don’t have their own this
or arguments
object.
4. What happens if I don’t bind this
in a callback?
If this
isn’t bound explicitly, its value depends on how the callback is invoked. In most cases, it defaults to the global object (window
in browsers or undefined
in strict mode).
5. How can I debug this
in JavaScript?
Use tools like console.log(this)
or browser developer tools to inspect the value of this
at different points in your code.