In the dynamic and evolving landscape of Web3, randomness plays a crucial role in ensuring the fairness and unpredictability of various applications. From provably fair gaming to the generation of unique tokens, the integrity of randomness is paramount. However, when this randomness is compromised, it opens the door to a range of vulnerabilities collectively known as Insecure Randomness Attacks. Understanding and mitigating these risks is essential for anyone developing smart contracts.
The Source of the Trouble
At the heart of many Web3 applications lies the pseudorandom number generator (PRNG). While PRNGs are designed to produce sequences of numbers that appear random, their output is determined by an initial value known as the seed. The security of these generators hinges on the unpredictability of the seed. If attackers can predict or manipulate the seed, they can predict the PRNG’s output, compromising the randomness and security of the application.
Why Predictable Seeds Are Dangerous
- Predictable Seeds: If the seed value is derived from easily obtainable data, such as timestamps, block hashes, or user addresses, it becomes predictable. Attackers can use this predictability to their advantage, undermining the randomness.
- Off-chain Bias: When randomness is generated off-chain (e.g., on a user’s computer), it can be tampered with before being submitted to the blockchain. This allows attackers to manipulate the input to their benefit.
Example of Predictable Seed in Solidity
Consider a simple example in Solidity where a seed is generated using the current block timestamp:
pragma solidity ^0.8.0;
contract PredictableRandom {
function getRandomNumber() public view returns (uint256) {
uint256 seed = uint256(keccak256(abi.encodePacked(block.timestamp)));
return seed;
}
}
In this example, the seed is derived from the current block timestamp, making it predictable. An attacker can easily guess the seed by knowing the block time.
When Lady Luck Frowns
The consequences of insecure randomness can be severe and far-reaching. Here are a few scenarios where insecure randomness can wreak havoc:
- Unfair Games In provably fair games, the fairness relies on the unpredictability of the outcomes. If attackers can predict the random numbers used in the game, they can unfairly win bets, draining funds from the contract and eroding trust in the platform.
- RNG-Based Token Exploits Randomness is often used in token generation to ensure a fair distribution. Predictable randomness allows attackers to manipulate the system, acquiring a disproportionate share of valuable tokens.
- Reentrancy Vulnerability Insecure randomness can also exacerbate other vulnerabilities, such as reentrancy attacks. An attacker can exploit predictable randomness to repeatedly exploit the contract within a single transaction.
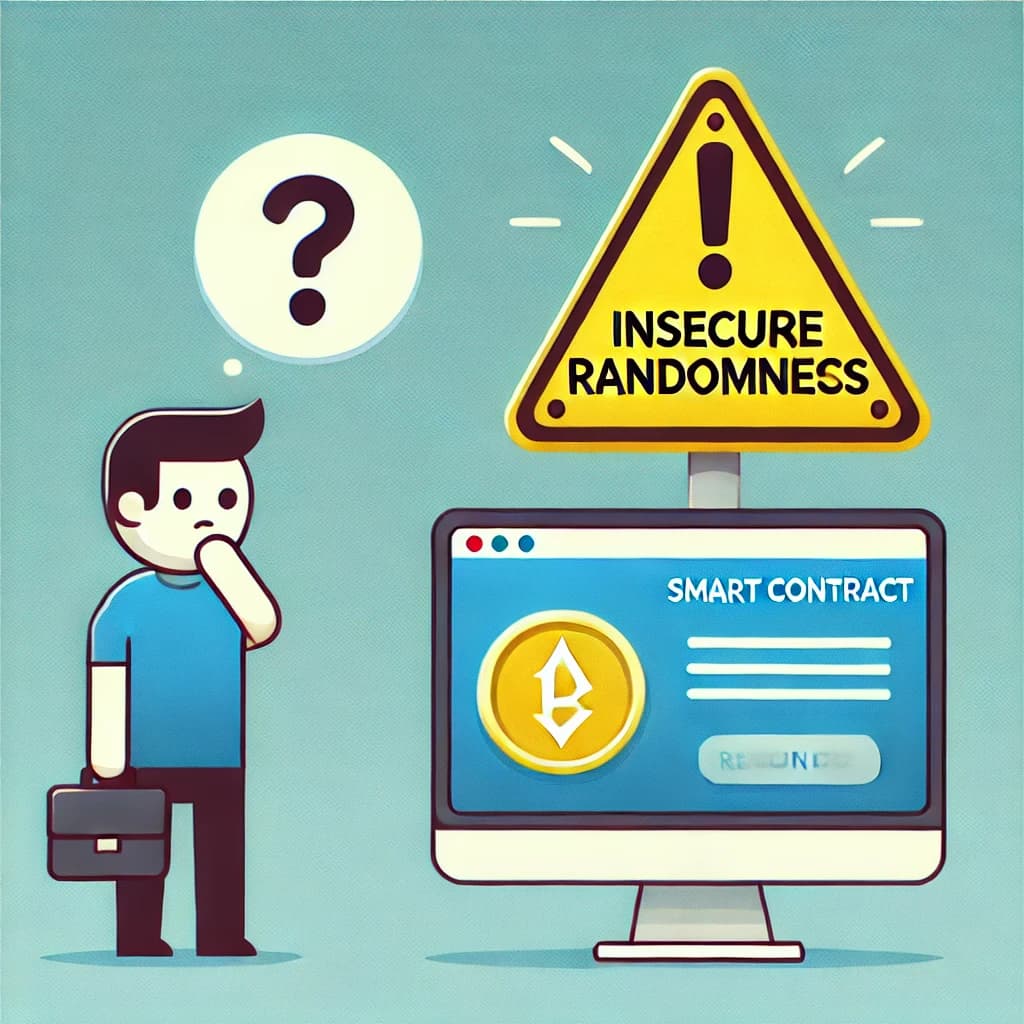
Real-World Incidents
History has shown that insecure randomness can lead to devastating exploits. Here are a couple of notable incidents:
The Etherroll Exploit (2016)
Etherroll, a decentralized dice game, suffered an exploit due to a flaw in its PRNG. Attackers were able to predict the winning numbers, resulting in significant financial losses for the platform.
The DAO Roulette (2016)
The DAO’s use of an off-chain source of randomness made it vulnerable to manipulation. This contributed to the infamous DAO hack, which led to the loss of millions of dollars worth of Ether.
Securing Your Random Acts
To safeguard your smart contracts from insecure randomness, consider the following best practices:
Leverage Secure Oracles
Decentralized oracles can provide verifiable randomness on-chain, ensuring that the random numbers used in your contract are truly unpredictable. Oracles like Chainlink VRF (Verifiable Random Function) are designed specifically for this purpose.
Example of Using Chainlink VRF
Here is an example of how to use Chainlink VRF to generate secure random numbers in a Solidity contract:
pragma solidity ^0.8.0;
import "@chainlink/contracts/src/v0.8/VRFConsumerBase.sol";
contract SecureRandom is VRFConsumerBase {
bytes32 internal keyHash;
uint256 internal fee;
uint256 public randomResult;
constructor(address _vrfCoordinator, address _linkToken, bytes32 _keyHash, uint256 _fee)
VRFConsumerBase(_vrfCoordinator, _linkToken)
{
keyHash = _keyHash;
fee = _fee;
}
function getRandomNumber() public returns (bytes32 requestId) {
require(LINK.balanceOf(address(this)) >= fee, "Not enough LINK");
return requestRandomness(keyHash, fee);
}
function fulfillRandomness(bytes32 requestId, uint256 randomness) internal override {
randomResult = randomness;
}
}
In this example, Chainlink VRF is used to generate a secure random number. The random number is provided by a decentralized oracle, ensuring its unpredictability.
Cryptographically Secure PRNGs
Using cryptographically secure PRNGs with unpredictable seeds is another effective way to ensure the security of your randomness. These PRNGs are designed to withstand attacks and produce truly random outputs.
Example of Cryptographically Secure PRNG in Solidity
Here’s an example of using a more secure method for generating random numbers in Solidity:
pragma solidity ^0.8.0;
contract SecureRandom {
function getSecureRandomNumber() public view returns (uint256) {
uint256 seed = uint256(keccak256(abi.encodePacked(block.timestamp, block.difficulty, msg.sender)));
return seed;
}
}
In this example, the seed is derived from a combination of the current block timestamp, block difficulty, and the caller’s address, making it significantly harder to predict.
Community Audits
Encouraging community code reviews and audits can help identify potential randomness vulnerabilities. Peer review by experienced developers can uncover flaws that might otherwise go unnoticed.
Conclusion: Insecure Randomness
Insecure randomness is a critical vulnerability that can compromise the integrity and security of Web3 applications. By understanding the risks and implementing best practices, developers can protect their smart contracts from these attacks. Leveraging secure oracles, using cryptographically secure PRNGs, and encouraging community audits are essential steps in ensuring the security of randomness in your smart contracts.
Understanding and addressing insecure randomness is not just about protecting your code; it’s about safeguarding the trust and fairness that underpin the entire Web3 ecosystem. By taking these precautions, you can help ensure that Lady Luck remains impartial and that your smart contracts remain secure.
FAQs:
What is insecure randomness in smart contracts?
- Insecure randomness refers to the use of predictable or easily manipulated random number generation in smart contracts, making them vulnerable to exploitation.
How does insecure randomness affect blockchain security?
- It can lead to predictable outcomes, allowing attackers to manipulate contract behavior, compromise fairness, and exploit vulnerabilities for financial gain.
What are common sources of insecure randomness in smart contracts?
- Common sources include block hashes, timestamps, and other on-chain data that can be influenced or predicted by malicious actors.
How can insecure randomness be prevented in smart contracts?
- Utilizing secure randomness techniques such as off-chain randomness, cryptographic functions, and decentralized oracle networks can prevent vulnerabilities.
Why is secure randomness crucial in decentralized applications?
- Secure randomness ensures fair and unpredictable outcomes, which are essential for applications like lotteries, gaming, and consensus mechanisms.
What are some examples of attacks due to insecure randomness?
- Notable examples include lottery manipulation in early Ethereum contracts and gaming applications where outcomes were predictably influenced.
How do decentralized oracles enhance randomness security?
- Decentralized oracles provide unbiased and tamper-proof external data, reducing the risk of predictable or manipulated random number generation.
What are the best practices for implementing secure randomness in smart contracts?
- Best practices include using verifiable random functions (VRFs), combining multiple sources of entropy, and regularly updating randomness algorithms.
How can regular audits help in addressing insecure randomness?
- Regular audits can identify and correct weaknesses in randomness implementation, ensuring that smart contracts remain secure against manipulation.
What role do developers play in securing randomness in smart contracts?
- Developers must implement robust random number generation methods, stay updated with security practices, and conduct thorough testing to ensure contract integrity.