The JavaScript array is a fundamental building block for storing and manipulating collections of data. But what if you need to edit that data – add new elements, remove unwanted ones, or even replace existing items? That’s where the JavaScript splice()
method comes in, your tool for modifying arrays. This guide delves into the world of splice()
, making it easy to understand and use for both beginners and seasoned developers.
What is splice()
and Why Do You Need It?
Imagine you have a shopping list as an array: ["milk", "bread", "eggs", "cookies"]
. You realize you forgot bananas, so you need to add them to the list. Or maybe you’ve already bought the eggs and want to remove them. splice()
empowers you to handle these situations with ease.
In essence, splice()
is a built-in function specifically designed for manipulating the content of arrays. It allows you to perform three key actions:
- Removing Elements: You can take out unwanted items from any position within the array.
- Adding Elements: Insert new elements at a specific location in the array.
- Replacing Elements: Remove existing elements and simultaneously add new ones in their place.
What truly sets splice()
apart is its ability to do all of this in a single method call, streamlining your code and making modifications efficient.
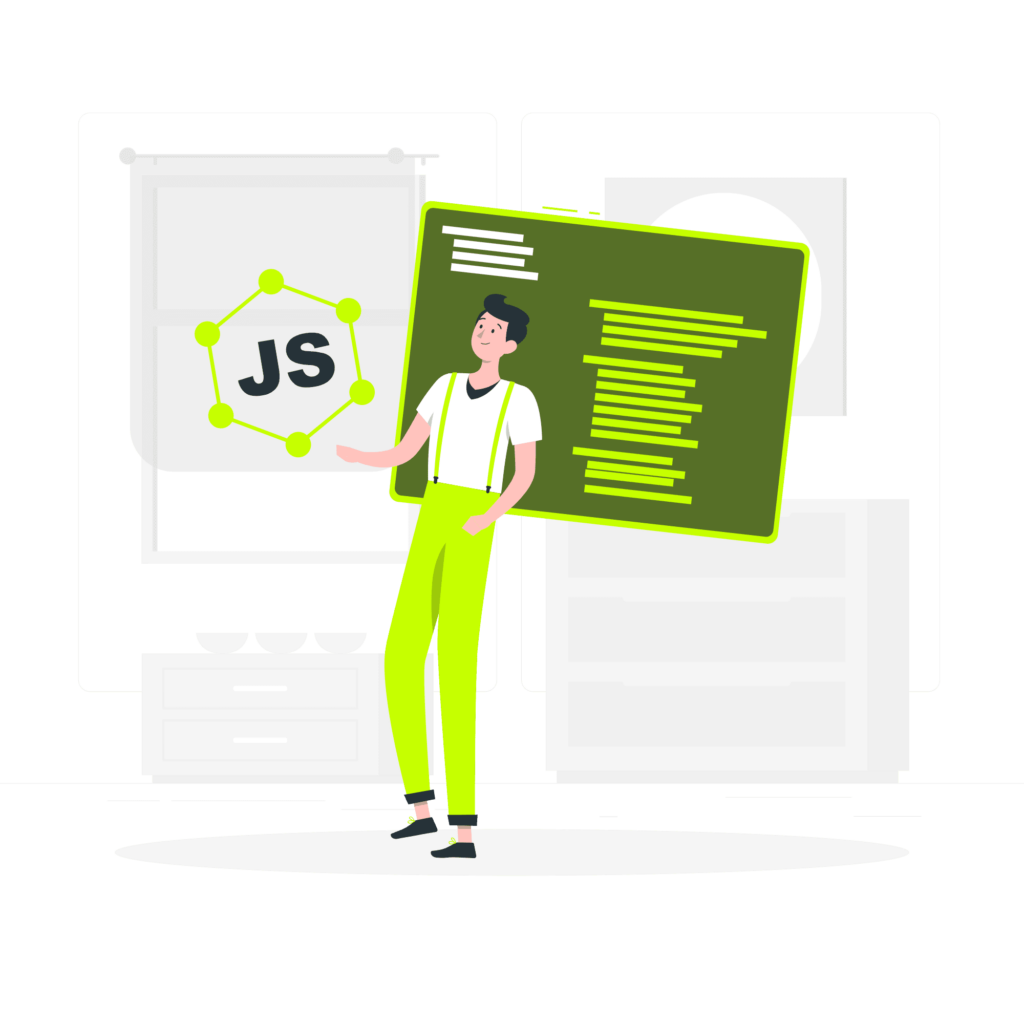
How Does JavaScript splice()
Work?
Understanding the syntax of splice()
is the first step to mastering its power. Here’s the basic structure:
array.splice(startIndex, deleteCount, item1, item2, ... itemN);
Let’s break down each part:
- array: This represents the array you want to modify.
- startIndex: This is the position at which you want to start making changes. It’s a zero-based index, meaning the first element is at index 0, the second at index 1, and so on. You can even use negative values to count backward from the end of the array.
- deleteCount (Optional): This specifies the number of elements you want to remove starting from the startIndex. If omitted, no elements are removed.
- item1, item2, … itemN (Optional): These are the new elements you want to insert at the startIndex. Any number of items can be added here.
Important Note: splice()
modifies the original array directly. It doesn’t create a new copy. This makes it efficient, but be mindful of unintended side effects if you need to preserve the original array.
Taking Out What You Don’t Need
Let’s revisit our shopping list example. You realize you don’t need cookies after all. Here’s how to remove them using splice()
:
const shoppingList = ["milk", "bread", "eggs", "cookies"];
shoppingList.splice(3, 1); // Remove 1 element starting from index 3 (cookies)
console.log(shoppingList); // Output: ["milk", "bread", "eggs"]
In this case, we specify 3
as the startIndex
, which points to the index of “cookies” (remember zero-based indexing). We set deleteCount
to 1
to remove just one element.
What if you want to remove everything from a certain point onwards? Simply omit the deleteCount
parameter:
shoppingList.splice(2); // Remove everything from index 2 onwards
console.log(shoppingList); // Output: ["milk", "bread"]
Adding Elements with Precision
Now imagine you forgot bananas. Let’s add them to the shopping list at the end:
shoppingList.splice(shoppingList.length, 0, "bananas"); // Add "bananas" at the end
console.log(shoppingList); // Output: ["milk", "bread", "bananas"]
Here’s the breakdown:
- We use
shoppingList.length
to get the current length of the array, which points to the end. - We set
deleteCount
to0
because we don’t want to remove any elements. - Finally, we specify “bananas” as the new item to be added.
Want to insert an element at a specific position? Just adjust the startIndex
:
shoppingList.splice(1, 0, "cheese"); // Add "cheese" after "milk" at index 1
console.log(shoppingList); // Output: ["milk", "cheese", "bread", "bananas"]
Here’s the breakdown:
- We set
startIndex
to1
, which points to the index after “milk” (remember zero-based indexing). - We keep
deleteCount
at0
since we don’t want to remove any elements. - Finally, we specify “cheese” as the new item to insert at that position.
Advanced Techniques with splice()
We’ve explored removing and adding elements with splice()
. Now let’s delve into more advanced techniques:
Replacing Elements
Imagine you bought apples instead of eggs. Here’s how to replace “eggs” with “apples”:
shoppingList.splice(2, 1, "apples"); // Replace 1 element at index 2 with "apples"
console.log(shoppingList); // Output: ["milk", "bread", "apples", "bananas"]
We kept deleteCount
at 1
to remove one element (“eggs”) and provided “apples” as the new item to insert at the same position.
Adding and Removing Simultaneously
You can combine removal and addition in a single splice()
call. Let’s say you decide to skip bread altogether and add juice instead:
shoppingList.splice(1, 1, "juice"); // Remove 1 element at index 1 and replace with "juice"
console.log(shoppingList); // Output: ["milk", "juice", "apples", "bananas"]
Negative Indices
Remember negative values for startIndex
? They let you count backwards from the end of the array. Here’s how to remove the last element (cheese) using a negative index:
shoppingList.splice(-1, 1); // Remove 1 element from the last index
console.log(shoppingList); // Output: ["milk", "juice", "apples", "bananas"]
Splicing with Caution
While splice()
is powerful, it’s crucial to use it carefully. Here are some things to keep in mind:
- Out-of-Bounds Indices: Using an invalid
startIndex
(like a negative value that goes beyond the array’s length) will result in unexpected behavior. - Accidental Overwrites: Be mindful of unintentionally overwriting elements if you’re not careful with
startIndex
anddeleteCount
.
Exploring Use Cases
Now that you’re equipped with splice()
, let’s explore some real-world scenarios where it shines:
- Building Interactive Lists: Imagine a to-do list application. You can use
splice()
to add new tasks, remove completed ones, or rearrange their order. - Filtering Data: Need to filter specific items from a larger dataset?
splice()
can help you remove unwanted elements based on certain criteria. - Creating Custom Data Structures: By combining
splice()
with other array methods, you can build your own custom data structures like stacks or queues.
Additional Tips and Tricks
- Practice Makes Perfect: Experiment with different
splice()
scenarios to solidify your understanding. - Read the Documentation: The official JavaScript documentation provides detailed information on
splice()
, including edge cases and advanced usage: MDN splice() - Consider Alternatives: For simple removals, consider using methods like
pop()
orshift()
. However,splice()
offers more flexibility for complex modifications.
Conclusion
By mastering splice()
, you’ll gain the power to manipulate arrays with ease, making your JavaScript code more efficient and versatile. So, the next time you need to modify an array, remember the art of the slice – splice()
is your trusty tool!
FAQs:
What is the JavaScript splice() method?
- The splice() method in JavaScript allows you to add, remove, or replace elements in an array.
How do you use splice() to remove elements from an array?
- Use array.splice(start, deleteCount) to remove elements, where ‘start’ is the index to start removing and ‘deleteCount’ is the number of elements to remove.
Can splice() be used to add elements to an array?
- Yes, array.splice(start, 0, item1, item2, …) adds elements without removing any, starting at the specified index.
How does splice() differ from slice()?
- Splice() modifies the original array, while slice() returns a shallow copy of a portion of the array without modifying it.
What are common use cases for the splice() method?
- Common use cases include removing elements, adding elements at a specific position, and replacing elements in an array.
Can splice() be used on strings in JavaScript?
- No, splice() is an array method and cannot be used on strings. Use string methods like substring() or slice() for string manipulation.
What does the splice() method return?
- The splice() method returns an array containing the deleted elements. If no elements are removed, it returns an empty array.
Is the original array altered when using splice()?
- Yes, splice() directly modifies the original array by adding, removing, or replacing elements.
What happens if deleteCount is 0 in splice()?
- If deleteCount is 0, no elements are removed from the array, and any additional arguments are inserted starting at the specified index.
How can splice() help in dynamic data manipulation?
- Splice() is useful for tasks like updating lists, managing data in dynamic applications, and efficiently handling array contents in JavaScript.