In a traditional transaction, one party sends funds to another in exchange for goods or services. This exchange typically follows a well-defined sequence of steps. However, in the realm of smart contracts, vulnerabilities can arise when this sequence is not properly enforced.
This vulnerability, known as a reentrancy attack, allows a malicious actor to exploit a weakness in a smart contract’s code. In this article, we’ll delve into the specifics of reentrancy attacks, exploring how they work, examining a real-world example, and discussing methods for prevention. We’ll also provide clear code examples to illustrate these concepts.
What is a Reentrancy Attack?
A reentrancy attack is a type of exploit that targets a vulnerability in smart contract functions involving external calls (like sending funds to another contract). This attack allows the malicious contract to repeatedly call the vulnerable function before it finishes executing, leading to multiple unauthorized executions of the function.
How It Works
Here’s a step-by-step breakdown of how a reentrancy attack unfolds:
- Attacker Initiates: An attacker sends money to a vulnerable smart contract function.
- The Bait is Set: The function performs its intended action, like transferring some of the attacker’s funds to another address.
- The Malicious Call: Before the function finishes, the attacker’s contract calls back into the original function again.
- Double Trouble: The original function, unaware it’s being re-entered, repeats the steps, sending more funds to the attacker before finally updating its internal state.
This process can be repeated multiple times, draining the contract’s funds before it realizes something is wrong.
Example of a Vulnerable Smart Contract
Let’s look at a simple example of a vulnerable smart contract in Solidity:
pragma solidity ^0.8.0;
contract VulnerableContract {
mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
// Transfer the requested amount to the caller
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
// Update the balance only after transferring
balances[msg.sender] -= _amount;
}
}
In this contract, the withdraw
function is vulnerable because it sends Ether to the caller before updating the balance.
The DAO Hack (2016)
One of the most infamous reentrancy attacks involved a Decentralized Autonomous Organization (DAO) on the Ethereum blockchain. The DAO’s smart contract had a critical reentrancy bug. An attacker exploited this by calling the DAO’s contribution function, essentially tricking the contract into sending them Ether multiple times. This resulted in a loss of over 60 million USD worth of Ether!
How the DAO Hack Worked
- The attacker created a malicious contract and called the
withdraw
function of the DAO. - Before the DAO could update the balance, the attacker’s contract called back into the
withdraw
function again. - This reentrancy allowed the attacker to drain funds repeatedly until the contract was empty.
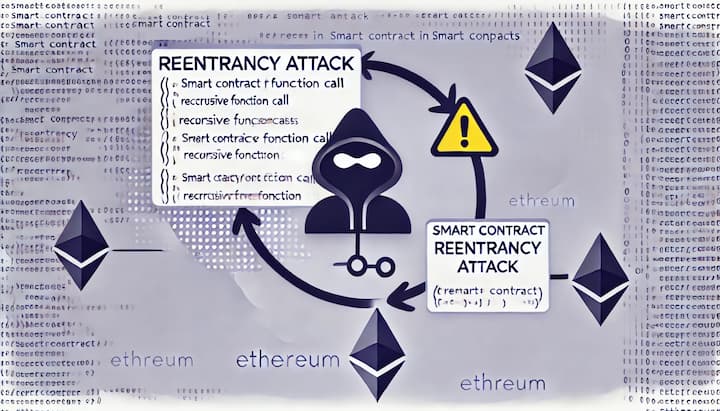
Preventing Reentrancy Attacks
Thankfully, there are ways to prevent reentrancy attacks. Here are some popular methods:
- Checks-Effects-Interactions Pattern (CEI) : This coding pattern ensures the contract updates its internal state (like recording a transfer) before making any external calls. By doing so, the contract can prevent reentrancy because the state is already updated when the external call is made.
pragma solidity ^0.8.0;
contract SecureContract {
mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
// Update the balance first
balances[msg.sender] -= _amount;
// Then transfer the requested amount
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
}
In this version, the balance is updated before the transfer, preventing reentrancy.
- Reentrancy Guards : Reentrancy guards are special functions that prevent a function from being re-entered by the same caller. One common way to implement this is by using a mutex, which locks the function during execution.
pragma solidity ^0.8.0;
contract SecureContractWithReentrancyGuard {
mapping(address => uint) public balances;
bool internal locked;
modifier noReentrancy() {
require(!locked, "No re-entrancy");
locked = true;
_;
locked = false;
}
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint _amount) public noReentrancy {
require(balances[msg.sender] >= _amount, "Insufficient balance");
// Update the balance first
balances[msg.sender] -= _amount;
// Then transfer the requested amount
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
}
The noReentrancy
modifier ensures that the function cannot be re-entered until it has finished executing.
- Solidity Safe Math Solidity offers functions for safe arithmetic operations that prevent overflow errors, which attackers can sometimes leverage in reentrancy attacks. Using the SafeMath library can add an extra layer of security.
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/utils/math/SafeMath.sol";
contract SecureContractWithSafeMath {
using SafeMath for uint;
mapping(address => uint) public balances;
bool internal locked;
modifier noReentrancy() {
require(!locked, "No re-entrancy");
locked = true;
_;
locked = false;
}
function deposit() public payable {
balances[msg.sender] = balances[msg.sender].add(msg.value);
}
function withdraw(uint _amount) public noReentrancy {
require(balances[msg.sender] >= _amount, "Insufficient balance");
// Update the balance first
balances[msg.sender] = balances[msg.sender].sub(_amount);
// Then transfer the requested amount
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
}
By using SafeMath, you ensure that your contract performs arithmetic operations safely.
Conclusion
Reentrancy attacks are a serious threat to smart contracts, but they can be effectively mitigated with proper coding practices. By understanding how these attacks work and implementing preventive measures like the Checks-Effects-Interactions pattern, reentrancy guards, and Solidity’s SafeMath library, developers can build more secure smart contracts that safeguard user funds.
FAQs
What is a reentrancy attack in smart contracts?
- A reentrancy attack exploits a vulnerability in smart contracts, allowing an attacker to repeatedly call a function before previous executions complete, leading to unexpected behaviors and potential loss of funds.
How can reentrancy attacks be prevented in smart contracts?
- Reentrancy attacks can be prevented by using proper coding practices such as the “Checks-Effects-Interactions” pattern, reentrancy guards, and thorough contract audits.
Why are reentrancy attacks a significant concern in DeFi?
- DeFi platforms often handle large amounts of assets, making them prime targets for reentrancy attacks. Exploits can result in substantial financial losses and damage to the platform’s reputation.
What are some famous examples of reentrancy attacks?
- One of the most notable examples is the DAO hack in 2016, where attackers exploited a reentrancy vulnerability, leading to the loss of around $60 million worth of Ether.
How does the “Checks-Effects-Interactions” pattern help prevent reentrancy attacks?
- This pattern ensures that state changes (checks and effects) are made before any external interactions, reducing the risk of reentrancy attacks by updating the contract state before calling external contracts.
Why is blockchain security important?
- Blockchain security is crucial because it protects users’ assets, maintains trust in the system, and ensures the integrity of decentralized applications and services.
What are some common vulnerabilities in smart contracts?
- Common vulnerabilities include reentrancy, integer overflow/underflow, improper access control, and unchecked external calls.
How can developers ensure their smart contracts are secure?
- Developers can ensure security by following best practices, conducting regular audits, using formal verification methods, and staying updated with the latest security research and tools.
What role do auditors play in blockchain security?
- Auditors review and analyze smart contract code to identify vulnerabilities, provide recommendations for improvements, and help ensure that the contracts function as intended without security flaws.