The Python return
statement is one of the most fundamental features of the language. Whether you’re writing a simple function to add two numbers or building complex software, understanding how and when to use return
is crucial for efficient programming.
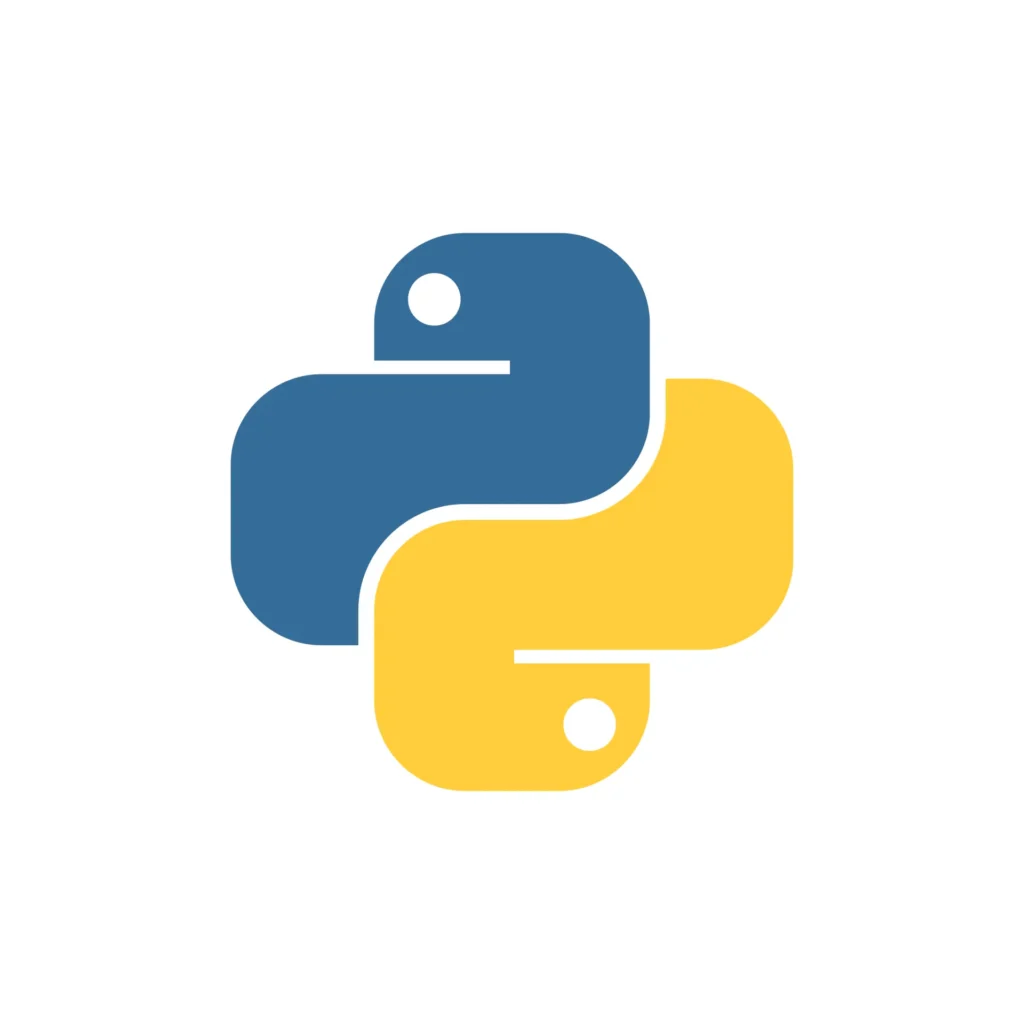
In this article, we’ll dive deep into the Python return
statement, explain its syntax, explore its use cases, highlight common mistakes, and discuss best practices. By the end, you’ll be equipped with the knowledge to use return
effectively in your projects.
What is the Return Statement in Python?
The return
statement in Python is used to exit a function and send a value back to the caller. It’s the mechanism through which a function communicates its result or output.
Here’s the basic syntax:
def function_name(your_parameters):
# your function body / code block
return value
value
: This is the data the function sends back to the caller. It can be any data type—an integer, string, list, dictionary, or even another function.- Optional: If no value is specified, the function returns
None
by default.
Why is the Return Statement Important?
The python return statement is what makes functions reusable and versatile. Without it, a function would perform its task but wouldn’t provide any output that could be used elsewhere in the program. To understand about python and return statement.
Key Benefits of the Return Statement
- Reusability: Functions with return statements can be called multiple times and used in different parts of a program.
- Flexibility: Return values can be used in other calculations, stored in variables, or passed to other functions.
- Readability: Clear return statements improve the readability and maintainability of your code.
Basic Usage of the Return Statement
Let’s start with a simple example to demonstrate how the return statement works:
Example 1: Returning a Value
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result) # The Output will be 8
- The function
add_numbers
takes two arguments (a
andb
). - It calculates the sum and sends the result back to the caller using the
return
statement. - The caller stores the result in the
result
variable and prints it.
Example 2: Returning Multiple Values
Python allows you to return multiple values as a tuple.
def calculate(a, b):
sum = a + b
product = a * b
return sum, product
s, p = calculate(4, 5)
print(f"Sum: {s}, Product: {p}") # Output: Sum: 9, Product: 20
This approach is handy when you need a function to provide multiple outputs.
Advanced Use Cases of the Return Statement
Returning Complex Data Structures
You can return lists, dictionaries, or even custom objects.
Example 3: Returning a List
def generate_numbers(n):
return [i for i in range(n)]
print(generate_numbers(5)) # Output: [0, 1, 2, 3, 4]
Example 4: Returning a Dictionary
def person_details(name, age):
return {"name": name, "age": age}
print(person_details("Alice", 30)) # Output: {'name': 'Alice', 'age': 30}
Using Return in Recursive Functions
Recursive functions rely heavily on the return
statement to break down problems into smaller subproblems.
Example 5: Calculating Factorial
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
Common Mistakes with the Return Statement
While the return
statement is straightforward, developers often make mistakes. Let’s explore some common pitfalls and how to avoid them.
Mistake 1: Forgetting to Return a Value
def greet(name):
print(f"Hello, {name}")
result = greet("Alice")
print(result) # Output: Hello, Alice and None
Solution: If you want the function to return a value, explicitly use the return
statement.
Mistake 2: Returning the Wrong Data Type
def add(a, b):
return str(a + b) # This str function converts sum into string
result = add(2, 3)
print(result + 2) # TypeError: can only concatenate str (not "int") to str
Solution: Ensure the return type matches the caller’s expectations.
Mistake 3: Using Return Outside a Function
The return
statement is only valid inside a function. Using it elsewhere will raise a SyntaxError
.
Best Practices for Using the Return Statement
- Be Explicit: Always specify what the function should return.
- Keep It Simple: Avoid overly complex return values that are hard to interpret.
- Document Your Functions: Use docstrings to explain what the function returns.
- Test Edge Cases: Ensure your return statement handles all possible inputs.
FAQs
Can a function have multiple return statements?
- Yes, a function can have multiple return statements. This is often used in conditional logic.
def check_number(num):
if num > 0:
return "Positive"
elif num < 0:
return "Negative"
return "Zero"
What happens if I don’t use a return statement?
- If you don’t use a return statement, the function will return
None
by default.
Can I return a function from another function?
- Yes, Python supports higher-order functions, which means you can return a function from another function.
def outer_function():
def inner_function():
return "Hello from inner function!"
return inner_function
func = outer_function()
print(func()) # Output: Hello from inner function!
Can I return multiple values without using a tuple?
- No. By default, multiple values are returned as a tuple.
How can I debug issues with the return statement?
- Use print statements or Python’s debugging tools to check what values your function returns at different stages.