TL;DR
Commit messages are critical for maintaining a clear and collaborative development process. They should be clear, concise, and consistent, adhering to a standard format with a descriptive subject line and optional detailed body.
Follow best practices like using the imperative tone, referencing related issues, and avoiding ambiguity. Avoid common pitfalls such as vague messages, combining multiple changes, or leaving messages blank. Tools like Git hooks, commit linting, and templates can help enforce standards and improve quality.
Commit messages are more than just a routine part of version control; they’re essential documentation that ensures clarity, accountability, and collaboration in software development. Despite their importance, commit messages are often undervalued and poorly written, leading to confusion and inefficiency. This guide delves into the proper use of commit messages, outlining best practices, the benefits of doing it right, and how to ensure your commit messages serve their purpose effectively.
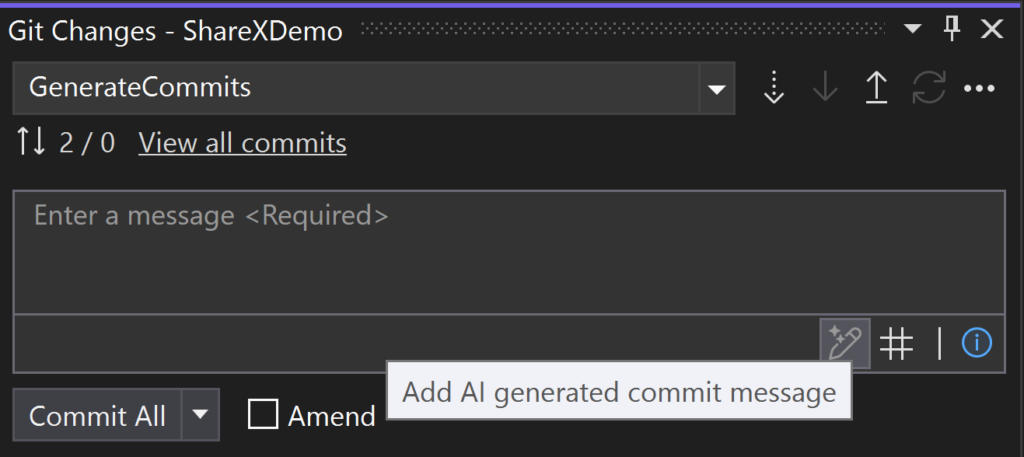
Why Commit Messages Matter
Commit messages provide context for changes made to a codebase. They act as a bridge between developers and the changes implemented over time. A well-written commit message can:
- Improve collaboration among team members.
- Simplify code reviews.
- Provide historical context for future debugging or feature expansion.
- Enhance transparency and accountability.
- Support automation tools in understanding changes.
Characteristics of a Good Commit Message
A good commit message is:
- Clear: Avoid vague descriptions. A clear message communicates the purpose of the change effectively.
- Concise: While providing enough detail, avoid unnecessary verbosity.
- Consistent: Adhere to a format or structure that your team agrees on.
- Actionable: Use imperative verbs to describe what the commit does (e.g., “Fix bug” instead of “Fixed bug”).
- Descriptive: Provide enough information to explain why a change was made, not just what was changed
Best Practices for Writing Commit Messages
Follow a Standard Format
A standard format makes sure of uniformity and readability. A commonly recommended structure is:
<Subject line: A short summary of the change (50 characters or less)>
<Body: A detailed explanation of the change (optional)>
<Footer: References to issues or pull requests (optional)>
Example –
Fix authentication bug in login API
The login API was returning a 500 error due to a null pointer exception. Added null checks to ensure the API handles missing parameters gracefully.
Closes #1234
Use Imperative Tone
Write commit messages as if you are commanding the code to perform an action. For instance:
- Good: “Add input validation to user forms.”
- Bad: “Added input validation to user forms.”
Keep the Subject Line Short and Informative
Aim for a subject line that is 50 characters or less. This ensures it’s easy to read and fits within most tools’ interface constraints.
- Good: “Refactor user authentication module.”
- Bad: “Refactored the entire user authentication module and added new security checks.”
Provide Context in the Body (When Needed)
If a commit introduces complex changes, use the body to explain:
- Why the change was necessary.
- How the change was implemented.
- Any potential implications or future considerations.
Reference Related Issues or Pull Requests
Link commits to relevant issue trackers or pull requests to create traceability. For example:
Resolve memory leak in database connection pooling
The connection pool was not properly releasing idle connections. Updated the configuration to enforce a timeout.
Fixes #987
Avoid Combining Multiple Changes in One Commit
Each commit should encapsulate a single logical change. This makes it easier to:
- Roll back specific changes.
- Understand the history of a codebase.
- Reduce merge conflicts.
Avoid Ambiguity and Jargon
Write commit messages with clarity and avoid technical jargon that might not be understood by all team members or future maintainers.
Spell-Check and Proofread
Typos or grammatical errors in commit messages can undermine professionalism and lead to miscommunication.
Common Mistakes to Avoid
- “WIP” Commits: Avoid vague messages like “WIP” (Work in Progress). Use descriptive messages that indicate what’s actually in progress.
- No Message at All: Never leave commit messages blank. It provides no context and adds confusion.
- Too Much Detail: Avoid turning commit messages into essays. The code should explain how the change was made; the commit message should explain why.
- Rewriting History Indiscriminately: Be cautious with tools like
git rebase
that allow you to rewrite commit history. Only rewrite history for private branches, not shared ones.
Tools and Techniques to Improve Commit Message Practices
Git Hooks
Git hooks are scripts that can be triggered by Git actions. For instance, you can use a commit-msg
hook to enforce a specific format or validate the content of commit messages.
Commit Linting Tools
Tools like CommitLint can automate the process of checking commit messages for compliance with your team’s standards.
Collaborative Reviews
Encourage team members to review commit messages during code reviews. This creates a culture of accountability and improves overall quality.
Templates
Using commit templates can help ensure consistency. For example:
# Subject (50 characters or less)
# Why this change was necessary
# What this change does
# Any implications of this change
Examples of Good Commit Messages
Feature Addition:
Add user profile page
Implemented a new user profile page that displays user details and recent activity. Added tests for the new page.
Bug Fix:
Fix null pointer exception in data processing module
Null values in the input data caused a crash. Added a check to handle nulls gracefully.
Refactor:
Refactor database query logic
Simplified query generation by using a query builder library. Improved maintainability and performance.
FAQs
Why should I use the imperative tone in commit messages?
- Using the imperative tone aligns with Git’s internal messages and provides consistency. It’s also more action-oriented, describing what the commit does.
How detailed should a commit message be?
- Include enough detail to explain why the change was made. If the change is complex, use the body to provide context. For simple changes, a concise subject line may suffice.
Can I edit commit messages after committing?
- Yes, you can use
git commit --amend
for the most recent commit or interactive rebase (git rebase -i
) for older commits. Be cautious when editing messages in shared branches.
What should I do if I forgot to write a message for a commit?
- Use
git commit --amend
to add or edit the commit message of the most recent commit.
Should I include code details in commit messages?
- No. Commit messages should explain why a change was made. The code itself explains how the change was implemented.
How can I enforce a standard for commit messages?
- Use tools like Git hooks or commit linting libraries to validate commit messages automatically.