TL;DR
- Bcrypt securely hashes passwords with salting, making them resistant to attacks.
- JWT enables stateless authentication by securely transmitting user data.
- Together, they improve security, scalability, and performance in modern web apps.
- Best practices include strong passwords, secure secret key management, and HTTPS usage.
- Implementing both reduces server load and enhances user authentication security.
In today’s digital world, security is paramount. As we increasingly rely on web applications to store sensitive information, it’s crucial to ensure that this data is protected from unauthorized access. Two essential technologies that play a vital role in application security are Bcrypt and JWT.
Securing Your Passwords with Bcrypt
Bcrypt, short for Blowfish crypt, is a powerful password hashing function. Unlike simply storing passwords in plain text, which makes them vulnerable to hacking, Bcrypt creates a unique and complex string of characters, called a hash, from a user’s password. This hash is then stored in the database instead of the actual password.
Here’s how Bcrypt works:
- Password Input: When a user creates an account or logs in, they provide a password.
- Salt Generation: Bcrypt generates a random string of characters, called a salt. This salt is unique for each user and adds an extra layer of security by making it infeasible to pre-compute a hash for a common password.
- Concatenation: The user’s password is then concatenated (combined) with the salt.
- Hashing: The combined password and salt are fed into the Bcrypt algorithm, which generates a unique hash.
- Storage: The generated hash is stored in the database.
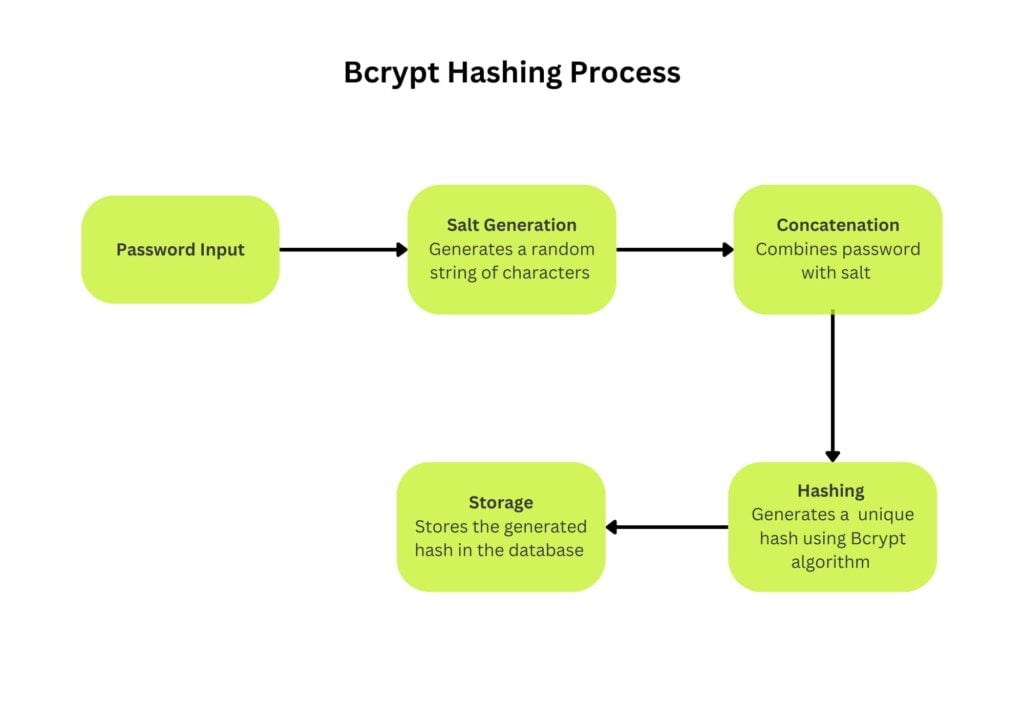
Here is an example of hashing using Bcrypt,
const bcrypt = require('bcrypt');
const saltRounds = 10; // You can adjust the number of salt rounds according to your security needs.
const bcrypt = require('bcrypt');
const plainPassword = 'mySuperSecretPassword';
// Hash the password
bcrypt.hash(plainPassword, saltRounds, function(err, hash) {
if (err) {
return console.error(err);
}
console.log('Hashed Password:', hash);
});
When a user attempts to log in, the following happens:
- Password Entry: The user enters their password during login.
- Retrieval: The corresponding hash stored for that user is retrieved from the database.
- Salting: The entered password is concatenated with the same salt used when the user’s account was created.
- Hashing: The combined password and salt are hashed using the Bcrypt algorithm.
- Comparison: The newly generated hash is compared to the stored hash. If they match, the login is successful; otherwise, it fails.
Here is an example of using Bcrypt to compare a password hashed using Bcrypt,
const bcrypt = require('bcrypt');
const plainPassword = 'mySuperSecretPassword';
const hashedPassword = '$2b$10$7QmZz/sEF/mJoERyUjEOuO.KmZuj3N/xIvAfANmZb0J9xFstIRYJu'; // Example hash
// Compare the plain password with the hashed password
bcrypt.compare(plainPassword, hashedPassword, function(err, result) {
if (err) {
return console.error(err);
}
if (result) {
console.log('Password is valid!');
} else {
console.log('Password is invalid!');
}
});
Benefits of Using Bcrypt:
- Security: Bcrypt makes it extremely difficult for hackers to crack passwords. Even if they manage to steal the hashed passwords from the database, they cannot easily reverse the hash to obtain the original password.
- Salt Protection: The use of a unique salt for each user prevents attackers from pre-computing rainbow tables, which are pre-calculated hashes for common passwords.
- Adaptability: Bcrypt is a customizable algorithm. The work factor, which determines the number of iterations required to generate the hash, can be adjusted to increase security as computing power advances.
Streamlining Authentication with JWT
JSON Web Token (JWT) is a compact and self-contained way of securely transmitting information between parties as a JSON object. It consists of three parts:
- Header: The header contains information about the type of token (JWT) and the signing algorithm used.
- Payload: The payload contains the claims, which are pieces of information about the user or the application. These claims can include user ID, username, email address, and any other relevant data.
- Signature: The signature is generated by signing the header and payload using a secret key. This ensures the integrity and authenticity of the JWT.
Here’s how JWT works in a typical authorization flow:
- Login: The user logs in to the application and provides their credentials.
- Authentication: The application verifies the user’s credentials and, if successful, generates a JWT.
- Authorization: The JWT is sent back to the user, typically in the HTTP response header or as a cookie.
- Access Requests: With subsequent requests to access resources within the application, the user sends the JWT in the Authorization header.
- Verification: The application receives the JWT and verifies the signature using its secret key. If the signature is valid, the payload is decoded to extract the user’s claims.
- Access Granting: Based on the verified claims, the application determines if the user has the necessary permissions to access the requested resource.
Benefits of Using JWT:
- Stateless Authentication: JWT eliminates the need for the application to maintain session state on the server. This improves scalability and simplifies application architecture.
- Security: JWTs are signed using a secret key, making them tamper-proof. Any modification to the header or payload will invalidate the signature.
- Flexibility: JWTs can be used for various authorization purposes, such as user authentication, API access control, and content authorization.
Here is an example of signing a JWT,
const jwt = require('jsonwebtoken');
jwt.sign({ id: user.id, username: user.username }, secretKey, { expiresIn: '1h' });
Here is an example of verifying a JWT,
const jwt = require(‘jsonwebtoken’);
jwt.verify(token, secretKey, (err, user) => {
if (err) {
Throw err; // Handle if token isn’t valid
}
req.user = user;
next(); // Handle if token is valid
});
Bcrypt and JWT Working Together for Enhanced Security
While Bcrypt and JWT address different aspects of security, they work together to create a robust security posture for modern applications. Here’s how:
- Secure Password Storage: Bcrypt ensures secure storage of user passwords. When a user creates an account, their password is hashed with Bcrypt, and only the hash is stored in the database. This makes it virtually impossible for attackers to steal and decrypt actual passwords, even in the event of a data breach.
- Stateless Authentication with JWT: JWTs facilitate stateless authentication. After successful login, the server generates a JWT containing user information (claims) and signs it with a secret key. The JWT is then sent back to the user. With subsequent requests, the user sends the JWT in the Authorization header. The server verifies the signature and extracts user claims from the payload to determine access permissions.
This eliminates the need for the server to maintain session state, which is typically stored in a database or in-memory cache. This streamlines application architecture and improves scalability. - Reduced Server Load: Since JWTs contain user claims, the server doesn’t need to query the database for user information on every request. This reduces the server load and improves application performance.
- Flexibility and Extensibility: JWTs can be customized to include additional claims besides user information. These claims can represent roles, permissions, or other context-specific data. This flexibility allows for fine-grained access control within the application.
- Single Sign-On (SSO): JWTs can be used to implement SSO across multiple applications. When a user logs in to one application, a JWT can be issued that can be used for authentication by other trusted applications. This eliminates the need for users to log in repeatedly across different systems.
Here’s an example of how Bcrypt and JWT work together in a typical login and authorization flow:
- User Login: The user enters their username and password during login.
- Bcrypt Verification: The server retrieves the stored hash for that username and uses Bcrypt to compare the hashed version of the entered password with the stored hash. If they match, authentication is successful.
- JWT Generation: Upon successful authentication, the server generates a JWT containing user claims (e.g., user ID, roles) and signs it with a secret key.
- JWT Issuance: The JWT is sent back to the user, typically stored in a cookie or returned in the response header.
- Access Requests: With subsequent requests to access application resources, the user’s browser sends the JWT in the Authorization header.
- JWT Verification: The server receives the JWT and verifies the signature using its secret key. If the signature is valid, the user claims are extracted from the payload.
- Authorization Decision: Based on the extracted claims (e.g., user ID and roles), the server determines if the user has the necessary permissions to access the requested resource. If authorized, access is granted; otherwise, access is denied.
Best Practices for Using Bcrypt and JWT
Here are some best practices to keep in mind when using Bcrypt and JWT:
- Strong Passwords: Encourage users to create strong passwords to further enhance security with Bcrypt.
- Secret Key Management: The secret key used for signing JWTs should be strong, random, and kept confidential. Never store it directly in your application code.
- JWT Expiration: Set an appropriate expiration time for JWTs to minimize the risk of unauthorized access even if a token is compromised.
- HTTPS Communication: Always use HTTPS for communication between the client and server to ensure data encryption and prevent man-in-the-middle attacks.
- Regular Updates: Keep Bcrypt and any JWT libraries used in your application up-to-date with the latest security patches.
By following these best practices and leveraging the combined strengths of Bcrypt and JWT, you can create a secure and efficient authentication system for your modern application.
Conclusion
In conclusion, Bcrypt and JWT are vital tools for building secure and scalable applications. By understanding their individual roles and utilizing them in conjunction, you can significantly enhance your application’s security posture and protect sensitive user data.
FAQs
What is Bcrypt and how does it work?
- Bcrypt is a password hashing function that incorporates a salt to protect against rainbow table attacks, ensuring secure password storage.
What is JWT and why is it used?
- JWT (JSON Web Token) is a compact token format used for securely transmitting information between parties, commonly used for authentication and authorization.
How do Bcrypt and JWT enhance web app security?
- Bcrypt secures passwords by hashing them, while JWT provides a secure way to transmit user authentication data, both essential for robust web app security.
Can Bcrypt and JWT be used together in a web application?
- Yes, Bcrypt can be used to hash passwords, and JWT can handle user authentication, offering a comprehensive security approach.
What are the advantages of using Bcrypt for password hashing?
- Bcrypt provides strong security through salting and a computationally intensive hashing process, making it difficult for attackers to crack passwords.
How do you implement JWT in a web application?
- Implement JWT by generating a token upon user login, sending it to the client, and verifying the token on subsequent requests to ensure secure access.
What are common use cases for JWT?
- JWT is commonly used for user authentication, API authentication, and secure information exchange between services.
Is Bcrypt resistant to brute-force attacks?
- Bcrypt is designed to be computationally expensive, making it resistant to brute-force attacks by significantly slowing down the hashing process.
How can you securely store JWTs?
- Securely store JWTs in HTTP-only cookies or secure storage mechanisms provided by the platform to prevent unauthorized access.
What are the potential security risks of using JWT?
- Risks include token theft, replay attacks, and insufficient expiration times, which can be mitigated through proper implementation and security practices.