JavaScript, the language that powers the web, offers a dynamic toolbox for creating interactive experiences. One of the essential tools in this toolbox is the setTimeout
function. This function allows you to schedule the execution of code after a specified delay, adding a layer of control and timing to your web applications.
This guide delves into the world of setTimeout
in JavaScript, making it accessible for both beginners and seasoned developers. We’ll explore its purpose, syntax, usage patterns, and best practices, equipping you to confidently incorporate timed actions into your projects.
Understanding the Need for Delay
Imagine a scenario where you want to display a success message after a user submits a form. Right after submission, it might feel abrupt to show the message. A slight delay can create a smoother user experience, mimicking a real-world confirmation process. This is where setTimeout
comes in handy.
Here are some common use cases for setTimeout
:
- Simulating real-world delays: Create a more natural flow by introducing pauses between events, like displaying a loading animation before fetching data.
- Fading out elements: Gradually reduce the opacity of an element over time to create a smooth fade-out animation.
- Timed messages: Display temporary notifications or alerts after a specific duration.
- Polling for data: Periodically check for updates from a server at set intervals.
How setTimeout
Works
The setTimeout
function takes two arguments:
- A callback function: This is the code you want to execute after the delay. It can be an anonymous function defined directly within the
setTimeout
call or a pre-defined function you’ve created elsewhere. - Delay in milliseconds: This specifies the amount of time to wait before executing the callback function. 1000 milliseconds equals 1 second.
Here’s the basic syntax:
setTimeout(function_to_execute, delay_in_milliseconds);
Example:
function showSuccessMessage() {
alert("Your form has been submitted successfully!");
}
setTimeout(showSuccessMessage, 3000); // Display message after 3 seconds
In this example, the showSuccessMessage
function is scheduled to be executed after a 3-second delay (3000 milliseconds).
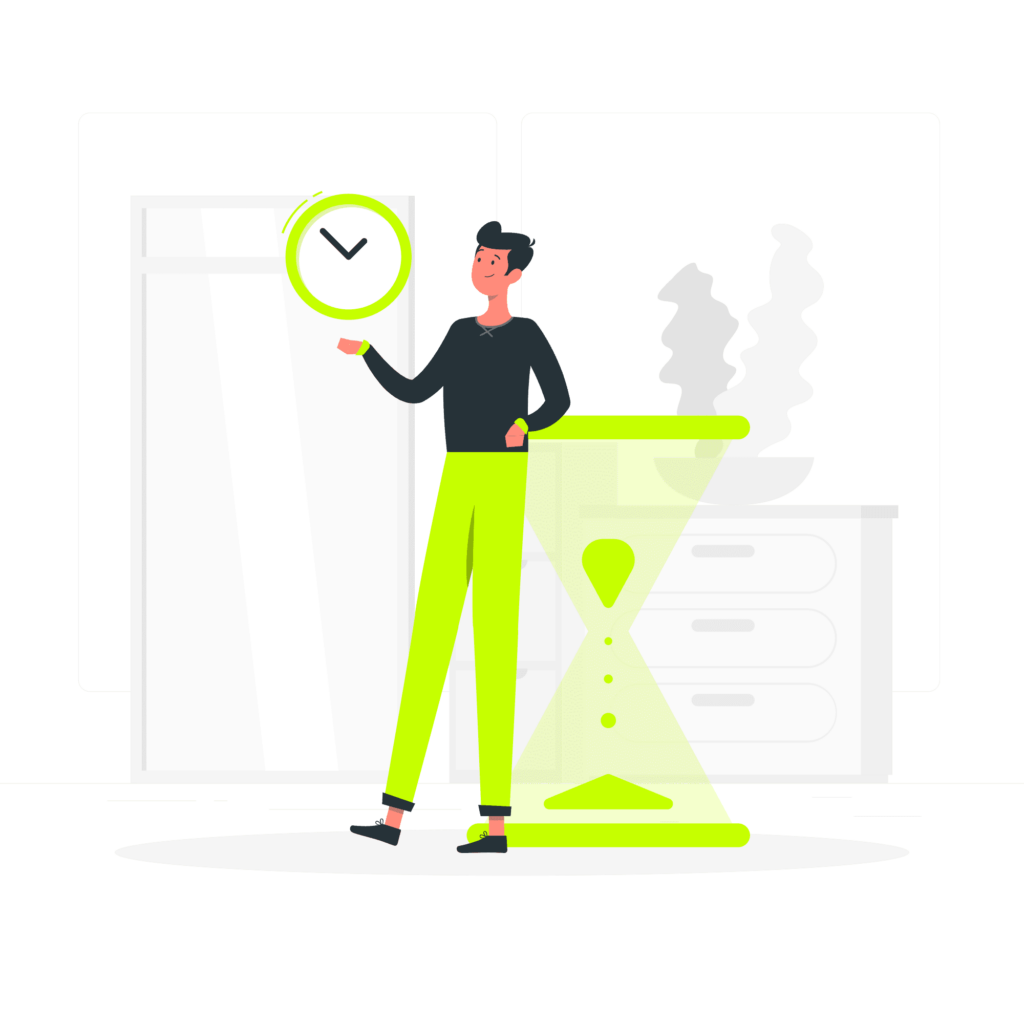
What Happens Behind the Scenes
JavaScript is an event-driven language, meaning it executes code in response to events like user interactions or browser actions. When you call setTimeout
, it doesn’t pause the execution of your program. Instead, it creates a timer in the background and continues running the rest of your code.
Once the specified delay elapses, the browser’s JavaScript engine adds the callback function to a queue of tasks waiting to be executed. This queue ensures that even if multiple setTimeout
calls are made, their execution happens in the order they were scheduled, maintaining proper timing.
Important Note: setTimeout
guarantees a minimum delay, not an exact one. Due to browser optimizations and multitasking, the actual delay might vary slightly. For more precise timing needs, consider advanced techniques like the requestAnimationFrame
API.
Exploring the Power of setTimeout
Now that you understand the core functionality, let’s explore some practical applications of setTimeout
:
Fading Out Elements
Enhance the visual appeal of your webpages by creating smooth fade-out animations. Here’s an example:
function fadeOutElement(elementId) {
let element = document.getElementById(elementId);
let opacity = 1;
function fadeStep() {
opacity -= 0.1; // Reduce opacity by 10% each step
element.style.opacity = opacity;
if (opacity > 0) {
setTimeout(fadeStep, 20); // Repeat every 20 milliseconds
}
}
fadeStep();
}
fadeOutElement("myElement"); // Fade out the element with ID "myElement"
This code defines a fadeOutElement
function that gradually reduces the opacity of an element with a specified ID. The setTimeout
function is used to schedule the fadeStep
function to run repeatedly at a set interval (20 milliseconds), effectively creating a smooth fading animation.
Polling for Data
In situations where data updates are crucial, you can use setTimeout
to periodically check for new information from a server:
function checkForUpdates() {
// Fetch data from the server
fetch("data.json")
.then(response => response.json())
.then(data => {
// Process the received data
console.log("Received data:", data);
// Update your UI or application logic based on the data
updateMyUI(data); // Replace with your function to update UI
// Schedule the next check for updates after a delay
setTimeout(checkForUpdates, 5000); // Check every 5 seconds
})
.catch(error => {
console.error("Error fetching data:", error);
// Handle any errors that might occur during the fetch request
});
}
// Start the initial check for updates
checkForUpdates();
Advanced Techniques with setTimeout
While the fundamental use of setTimeout
is straightforward, there are advanced techniques that unlock its full potential:
Chaining setTimeout
Calls for Sequential Actions
Need to execute multiple actions one after another with specific delays? You can chain setTimeout
calls to create a sequence:
function task1() {
console.log("Task 1 completed");
}
function task2() {
console.log("Task 2 completed");
}
setTimeout(task1, 1000); // Execute task1 after 1 second
setTimeout(task2, 3000); // Execute task2 after 3 seconds
Here, task1
executes after a 1-second delay, followed by task2
after another 2-second delay.
Combining setTimeout
with setInterval
for Repeated Actions
For scenarios requiring continuous or periodic execution of a task, setTimeout
can be combined with its cousin setInterval
. While setTimeout
executes a function only once after a delay, setInterval
repeatedly executes a function at a specified interval:
function updateClock() {
let currentTime = new Date();
console.log(currentTime.toLocaleTimeString());
}
let updateInterval = setInterval(updateClock, 1000); // Update clock every second
// To stop the updates:
clearInterval(updateInterval);
This code defines an updateClock
function that displays the current time. setInterval
is used to call this function every 1000 milliseconds (1 second), creating a continuously updating clock. The clearInterval
function allows you to stop the updates when needed.
Canceling Scheduled Delays with clearTimeout
Imagine you call setTimeout
to display a message, but the user performs an action that makes the message irrelevant. You can use clearTimeout
to cancel the scheduled delay:
let timeoutId;
function showWarningMessage() {
alert("Are you sure you want to proceed?");
}
timeoutId = setTimeout(showWarningMessage, 5000); // Schedule message after 5 seconds
// User clicks a button to confirm the action
clearTimeout(timeoutId); // Cancel the scheduled message
In this example, a warning message is set to appear after 5 seconds. However, if the user confirms the action before the delay, the clearTimeout
function cancels the scheduled message.
Remember: When using clearTimeout
, you need to store the returned ID from the setTimeout
call (assigned to timeoutId
in the example). This ID is used to reference the specific timer you want to cancel.
Best Practices for Using setTimeout
Effectively
To leverage JavaScript setTimeout
effectively in your projects, consider these best practices:
- Break Down Complex Tasks: If a task involves multiple steps, consider breaking it down into smaller functions and chaining
setTimeout
calls for a more readable and manageable code structure. - Handle Edge Cases: Account for scenarios where a user interaction might render a scheduled action unnecessary. Use
clearTimeout
to prevent irrelevant actions. - Consider Alternatives: For precise timing requirements, explore techniques like the
requestAnimationFrame
API, which synchronizes with the browser’s refresh rate for smoother animations. - Provide Clear Comments: Document your code with comments explaining the purpose of each
setTimeout
call and the delays used. This enhances code maintainability for yourself and others.
By following these practices, you can ensure that setTimeout
adds value to your code and creates a well-structured and efficient web application.
Conclusion: Javascript setTimeout()
setTimeout
is a powerful tool in your JavaScript arsenal. It allows you to introduce delays, create animations, and manage the flow of your application. By understanding its core functionality, exploring advanced techniques, and following best practices, you can confidently schedule actions and enhance the user experience of your web projects.
This guide has equipped you with the knowledge to leverage setTimeout
effectively. So go forth and create dynamic and engaging web experiences with the art of delayed actions!
FAQs:
How does the setTimeout() function work in JavaScript?
- The setTimeout() function executes a specified function after a designated delay, measured in milliseconds.
Can I cancel a setTimeout() once it has been set?
- Yes, you can use the clearTimeout() function with the identifier returned by setTimeout() to cancel the execution.
What is the syntax for using setTimeout() in JavaScript?
- The syntax is
setTimeout(function, delay)
, where function is the code to execute and delay is the time in milliseconds.
Can setTimeout() execute a function repeatedly?
- No, setTimeout() executes a function only once. Use setInterval() for repeated execution.
What are common use cases for setTimeout() in web development?
- Common use cases include creating pauses, delaying actions, and executing code after a delay for animations or user interactions.
How does setInterval() differ from setTimeout()?
- setInterval() repeatedly calls a function with a fixed delay between each call, whereas setTimeout() calls a function once after a delay.
Is the delay time guaranteed in setTimeout()?
- The delay time is not guaranteed due to potential browser and system performance variations; it represents the minimum delay.
Can I pass parameters to the function executed by setTimeout()?
- Yes, you can pass parameters by using an anonymous function or arrow function that calls the target function with arguments.
How do I handle errors inside a setTimeout() function?
- Errors inside setTimeout() can be handled using try-catch blocks within the function executed by setTimeout().
Does setTimeout() affect the performance of my JavaScript code?
- While setTimeout() is generally efficient, excessive use or very short delays can impact performance, so it should be used judiciously.