JavaScript, the popular language of the web, breathes life into interactive websites and dynamic applications. But even the most brilliant code can encounter errors, leaving your masterpiece malfunctioning. Fear not, fellow developer! This guide on JavaScript error handling equips you with the essential tools and techniques to tackle JavaScript errors with confidence, ensuring your code runs smoothly and delivers an exceptional user experience.
Common JavaScript Errors
Before we dive into solutions, let’s explore the battleground – the different types of errors you might encounter:
- Syntax Errors: These are grammatical errors in your code. A missing semicolon, a misspelled variable name, or a forgotten closing parenthesis can all trigger these. The browser throws a red flag, highlighting the line with the error, making them relatively easy to identify.
- Runtime Errors: These errors lurk in the shadows, only revealing themselves when your code executes. Examples include trying to access a non-existent variable, dividing by zero, or attempting to perform an operation on the wrong data type. These can be trickier to pinpoint.
- Logical Errors: The logic behind your code might be flawless, but the desired outcome isn’t achieved. This could be due to incorrect assumptions, faulty algorithms, or a misunderstanding of how JavaScript works. These require a deeper dive into your code’s reasoning.
Error Handling with try…catch
JavaScript offers a robust mechanism called try…catch to gracefully handle errors. Here’s how it works:
- try Block: This block encloses the code that might throw an error. It’s like a designated zone for potential troublemakers.
- catch Block: If an error occurs within the try block, control jumps to the catch block. This block allows you to handle the error, providing informative messages to the user and preventing your application from crashing.
Here’s an example:
try {
let result = undefined / 10; // This line might cause an error
console.log(result);
} catch (error) {
console.error("Oops, something went wrong! Please check your calculations.");
}
In this example, the try block attempts to divide undefined by 10, which would normally throw a runtime error. However, the catch block intercepts it, displaying a user-friendly message instead of a cryptic error code.
Pro Tip: The catch block receives an error object as an argument. This object contains valuable information about the error, such as its type and location in your code. You can use this information to provide more specific error messages.
Debugging Techniques
Even with error handling in place, pinpointing the exact cause of an error can be like finding a needle in a haystack. Here’s your debugging toolkit:
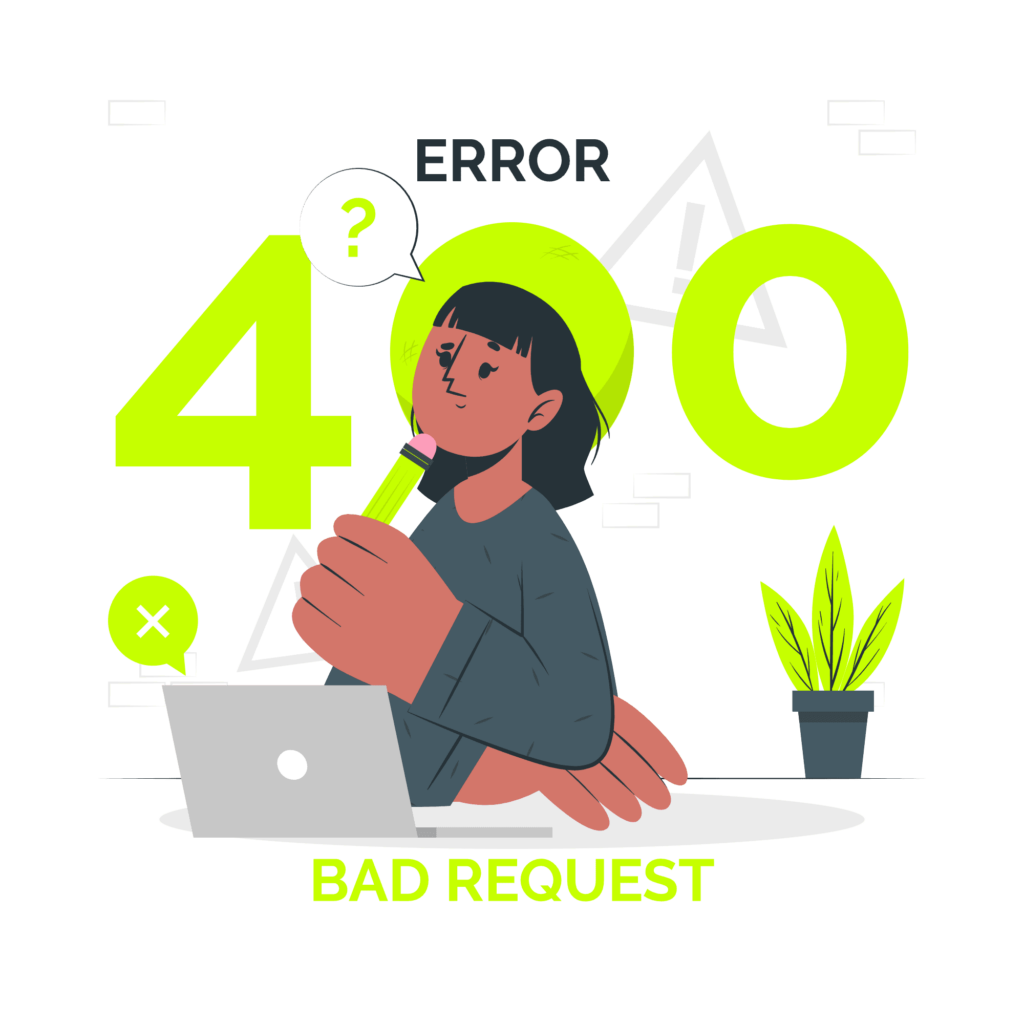
- Console Logging: Your browser’s console is your window into the soul of your code. Use console.log() statements to print variable values, messages, or the state of your application at different points. This helps you trace the flow of your code and identify where things go awry.
- Browser Developer Tools: Every modern browser offers built-in developer tools, a treasure trove for debugging. These tools allow you to:
- Set Breakpoints: Pause your code execution at specific lines, allowing you to inspect variables and the call stack (a record of function calls) to pinpoint the error’s origin.
- Inspect Variables: See the values of variables at any point in your code, helping you understand the data flow and identify unexpected changes.
- The Debugger: This advanced feature lets you step through your code line by line, examining variable values and the call stack after each step.
- Linters and Static Code Analysis Tools: These are third-party tools that analyze your code without running it. They can identify potential errors, syntax issues, and code smells (bad coding practices) even before you hit the run button.
Preventing Errors Before They Happen
While error handling and debugging are crucial, preventing errors in the first place is even better. Here are some defensive programming techniques:
- Input Validation: Always validate user input before using it in your code. This ensures you’re working with the expected data types and prevents errors caused by invalid inputs.
- Type Checking: Use JavaScript’s built-in type checking methods like typeof or instanceof to verify the data types of variables before performing operations on them. This can catch potential type mismatches that might lead to runtime errors.
- Error Throwing: If you encounter a situation where your code can’t proceed due to invalid data or unexpected conditions, use the throw keyword to throw a custom error. This allows you to handle errors gracefully within your code instead of relying on the browser to throw cryptic error messages. You can throw custom errors with informative messages explaining the issue, making debugging easier.
Advanced Error Handling Techniques
While try…catch is the foundation of error handling, JavaScript offers more advanced techniques to manage complex scenarios:
- Multiple catch Blocks: You can have multiple catch blocks within a single try block. Each catch block can handle a specific type of error using the error object’s type property. This allows for more granular error handling.
try {
let result = undefined / 10;
console.log(result);
} catch (TypeError) {
console.error("Looks like you're trying to perform an operation on an invalid data type.");
} catch (ReferenceError) {
console.error("Uh oh, you're referencing a variable that doesn't exist.");
}
- finally Block: The finally block is an optional block that always executes, regardless of whether an error occurs or not. It’s commonly used for cleanup tasks like closing files or releasing resources.
try {
const file = openFile("data.txt");
// Read data from the file
} catch (error) {
console.error("Error reading file:", error);
} finally {
if (file) {
file.close(); // Close the file even if an error occurred
}
}
- Promises and Async/Await: Asynchronous code, where operations take time to complete, introduces new challenges for error handling. Promises and async/await provide mechanisms to handle errors in asynchronous operations. You can use the catch() method on promises or a try…catch block with async/await to catch errors that occur during asynchronous execution.
Debugging Asynchronous Code
Debugging asynchronous code can be trickier than synchronous code because errors might not manifest immediately. Here are some tips:
- Use Debugging Tools: Leverage browser developer tools’ breakpoints and call stack inspection to track the flow of your asynchronous code and identify where errors occur.
- Console Logging: Strategically place console.log() statements to track the progress of asynchronous operations and the values of variables at different stages.
- Debuggers with Asynchronous Support: Some browser developer tools offer features specifically designed for debugging asynchronous code, such as the ability to pause execution while waiting for asynchronous operations to complete.
User-Friendly Error Messages
Error messages shouldn’t be cryptic codes that only developers understand. Strive to provide informative messages that guide users towards resolving the issue. Here are some tips:
- Explain the Problem: Clearly state what went wrong, avoiding technical jargon.
- Offer Solutions: If possible, suggest ways for the user to fix the problem or provide alternative paths within your application.
- Maintain a Positive Tone: Even when errors occur, a friendly and helpful tone can go a long way in user experience.
Conclusion
Error handling and debugging are essential skills for any JavaScript developer. By embracing these techniques, you can transform your code from a ticking time bomb into a robust and reliable application. Remember, errors are inevitable, but with the right tools and mindset, you can conquer them efficiently and ensure a smooth user experience.
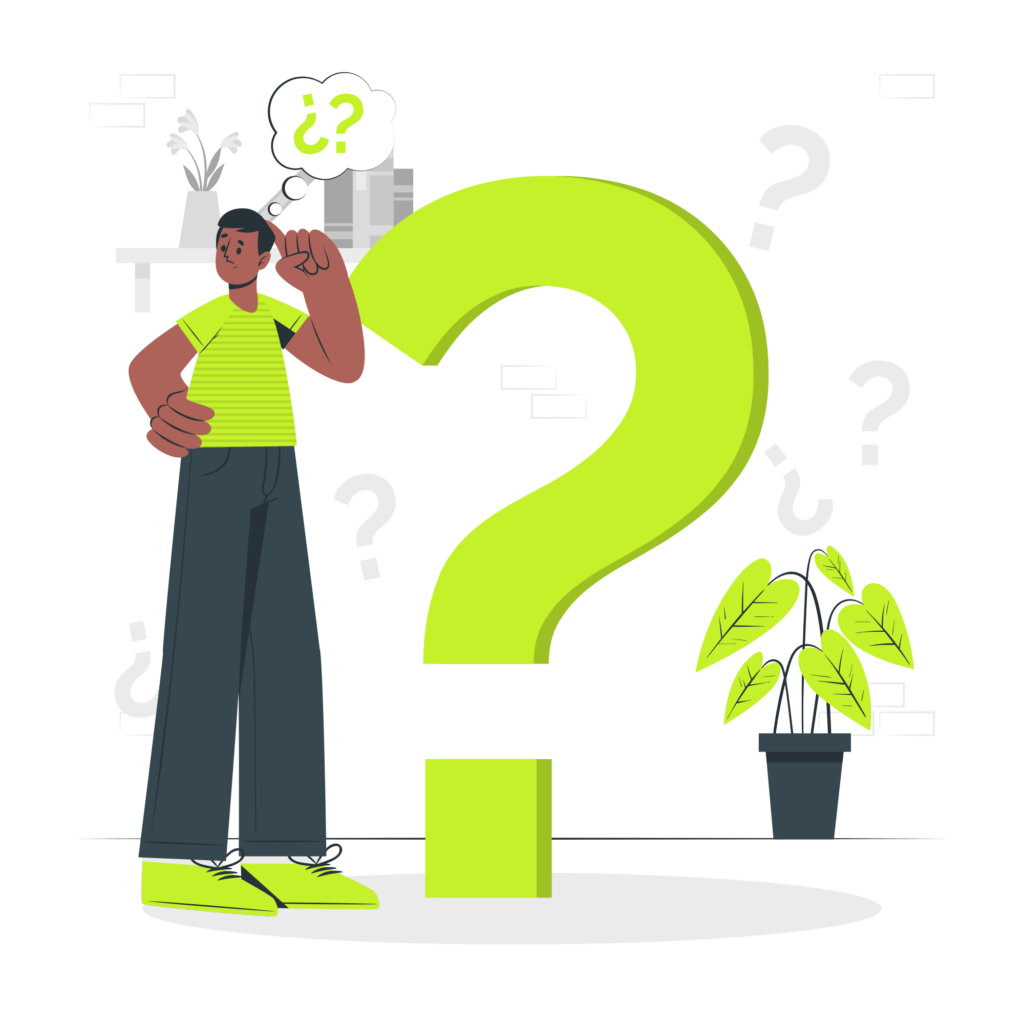
FAQs
What are common JavaScript error handling techniques?
- Common techniques include using try-catch blocks, throwing custom errors, and utilizing console logs for debugging.
How can I debug JavaScript code effectively?
- Utilize browser developer tools, apply breakpoints, leverage console.log statements, and conduct step-by-step code execution.
What tools are essential for debugging JavaScript?
- Essential tools include browser developer consoles, source maps, and debugging software like Node.js inspector or Chrome DevTools.
Why is error handling important in JavaScript?
- Proper error handling prevents crashes, improves user experience by managing exceptions gracefully, and maintains code flow.
How do I use try-catch in JavaScript?
- Enclose code that might throw an error in a
try
block and handle exceptions in thecatch
block to manage errors effectively.
What is the best way to learn JavaScript debugging?
- Practice by building projects, using debugging tools in IDEs and browsers, and reading comprehensive tutorials and documentation.
Can debugging techniques vary between browsers?
- Yes, debugging techniques and tools can vary slightly between browsers, but common principles like breakpoints and console use remain consistent.
What are the differences between syntax errors and runtime errors?
- Syntax errors occur during code parsing due to incorrect code structure, while runtime errors occur during execution, often due to logical errors.
How can I prevent common JavaScript errors?
- Follow best practices, use linters and code validators, write testable code, and consistently review and refactor your codebase.
Is it necessary to use third-party libraries for error handling?
- While not necessary, third-party libraries can simplify and enhance error handling and logging, especially in complex applications.