Imagine a website that’s just a static image, like a printed page. No clicking, no animations, no dynamic content – just… there. Boring, right? That’s where event handling in JavaScript comes in, like a spark that brings your web pages to life. It lets them respond to user interactions and events, creating a truly interactive experience.
What are Events ?
Think of events as signals sent by the user or the browser. Clicking a button, hovering over an image, even the page fully loading – these are all events. JavaScript’s Event Handling is about capturing these signals and making your web page react accordingly.
Here are some common events you might use:
- Click: When a user clicks on an element (button, image, etc.)
- Hover: When a user moves their mouse over an element
- Submit: When a user submits a form
- Load: When the entire page or an element (like an image) finishes loading
- Keypress: When a user presses a key on their keyboard
This is just a small list of the most commonly used events, there are many more events available!
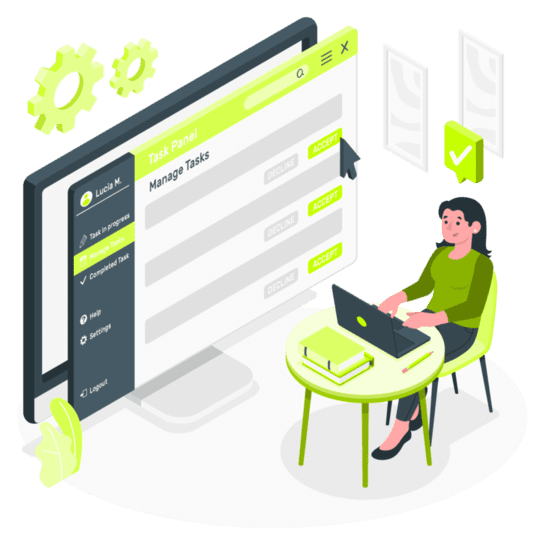
What are Event Handlers ?
Event handlers are like the backstage crew of your web page’s performance. They are JavaScript functions that wait for specific events to happen and then execute a block of code in response. This code can do anything you can imagine – change the content of an element, display a message, trigger an animation, or even send data to a server.
There are two main ways to assign event handlers in JavaScript:
- Using HTML Event Attributes: This is the simpler approach. You can directly assign a JavaScript function name to an HTML element’s on attribute, followed by the event name (e.g., onclick, onmouseover). Here’s an example:
<button onclick="changeColor()">Click Me!</button>
<script>
function changeColor() {
document.getElementById("myDiv").style.backgroundColor = "red";
}
</script>
In this example, clicking the button calls the changeColor() function, which changes the background color of the element with the ID “myDiv” to red.
- Using addEventListener() Method: This method offers more flexibility. You can attach multiple event handlers to the same element, specify different event types, and even remove event handlers later. Here’s how it works:
<button id="myButton">Click Me!</button>
<script>
const button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button Clicked!");
});
</script>
Here, we use addEventListener() on the button element. The first argument is the event type (“click” in this case), and the second is the function to be executed. Now, clicking the button displays an alert message.
Bringing Your Web Pages to Life with Event Handling
Let’s explore some real-world examples of how event handling can transform your web pages:
- Interactive Forms: Imagine a form where the error message appears only when the user tries to submit the form with an empty field. Event handlers on the submit event can validate the form data and display appropriate messages.
- Image Galleries: Create a dynamic image gallery where hovering over an image displays a caption or highlights the image border. Event handlers on the mouseover event can achieve this with ease.
- Accordions and Tabs: Build collapsable sections or tabbed content that reveals information upon user interaction. Event handlers on the click event can toggle the visibility of these sections.
- Interactive Games: Develop simple games where user actions like clicking or pressing keys trigger game logic. Event handlers on these events become the core of your game’s interactivity.
These are just a few examples. With event handling, the possibilities are endless!
Best Practices for Event Handling in JavaScript
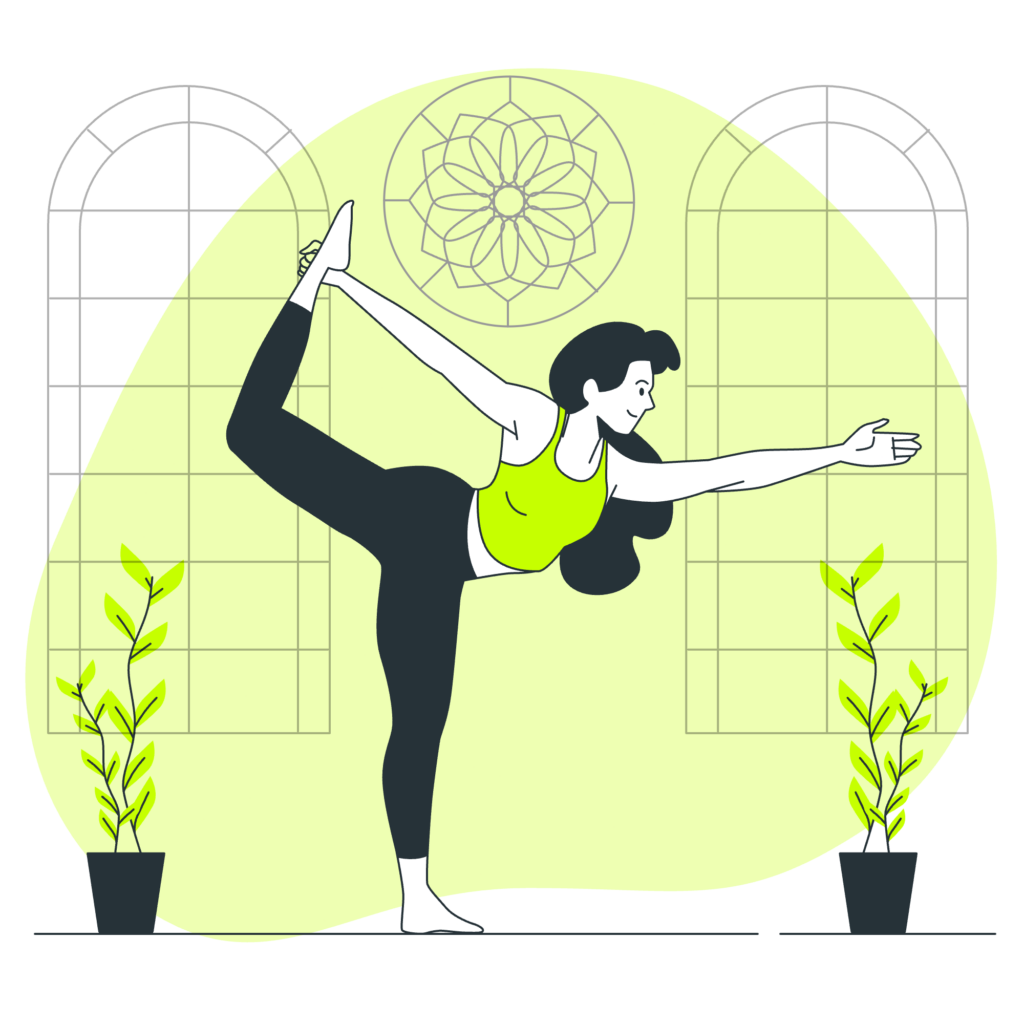
As you dive deeper into event handling, here are some tips to keep in mind:
- Separate Event Handlers from HTML: For better code organization, consider attaching event handlers using JavaScript instead of relying on inline HTML attributes.
- Use Meaningful Event Handler Names: Choose function names that clearly describe the action they perform (e.g., showDetails(), validateForm()).
- Prevent Default Behavior (When Necessary): In some cases, events like form submission might have default actions. Use event.preventDefault() to stop these defaults if your event handler wants to do something different.
- Handle Event Bubbling: When an event occurs on an element, it can “bubble up” to its parent elements. Be mindful of this behavior and use event.stopPropagation() if you only want the event to be handled by the element where it originated.
<div id="myDiv">
<button id="myButton">Click Me!</button>
</div>
Imagine attaching a click handler to both the button and the div. Clicking the button would trigger the click event on both elements. This might not be what you want. To prevent the event from bubbling up to the div, you can use event.stopPropagation() within the button’s click handler.
const button = document.getElementById("myButton");
button.addEventListener("click", function(event) {
// Perform some action specific to the button click
event.stopPropagation();
});
- Remove Event Listeners When Necessary: Event listeners can accumulate over time, especially on dynamic content. To prevent memory leaks and improve performance, consider removing event listeners when they are no longer needed. You can use the removeEventListener() method.
const button = document.getElementById("myButton");
const myHandler = function() {
// Event handler function
}
button.addEventListener("click", myHandler);
// Later, when you don't need the handler anymore
button.removeEventListener("click", myHandler);
- Use Event Delegation for Performance: As discussed earlier, delegation is a powerful technique for handling events on a group of elements with a single event listener attached to a parent element. This improves performance compared to attaching individual handlers to each element.
- Consider Cross-Browser Compatibility: While most event handling concepts work consistently across major browsers, there might be slight variations in behavior for certain events or properties. It’s always a good practice to test your code in different browsers to ensure a smooth user experience.
Earlier we explored the fundamentals of event handling in JavaScript. Now, let’s diver deeper into some advanced concepts to create even more refined web interactions.
Understanding Event Bubbling and Capturing
Remember event bubbling? When an event occurs on an element, it “bubbles up” to its parent elements in the HTML hierarchy. For example, clicking a button inside a div will trigger the click event on both the button and the div (unless stopped).
This can be useful, but sometimes you might want the opposite behavior – for the event to “capture” down the hierarchy instead. This is where event capturing comes in.
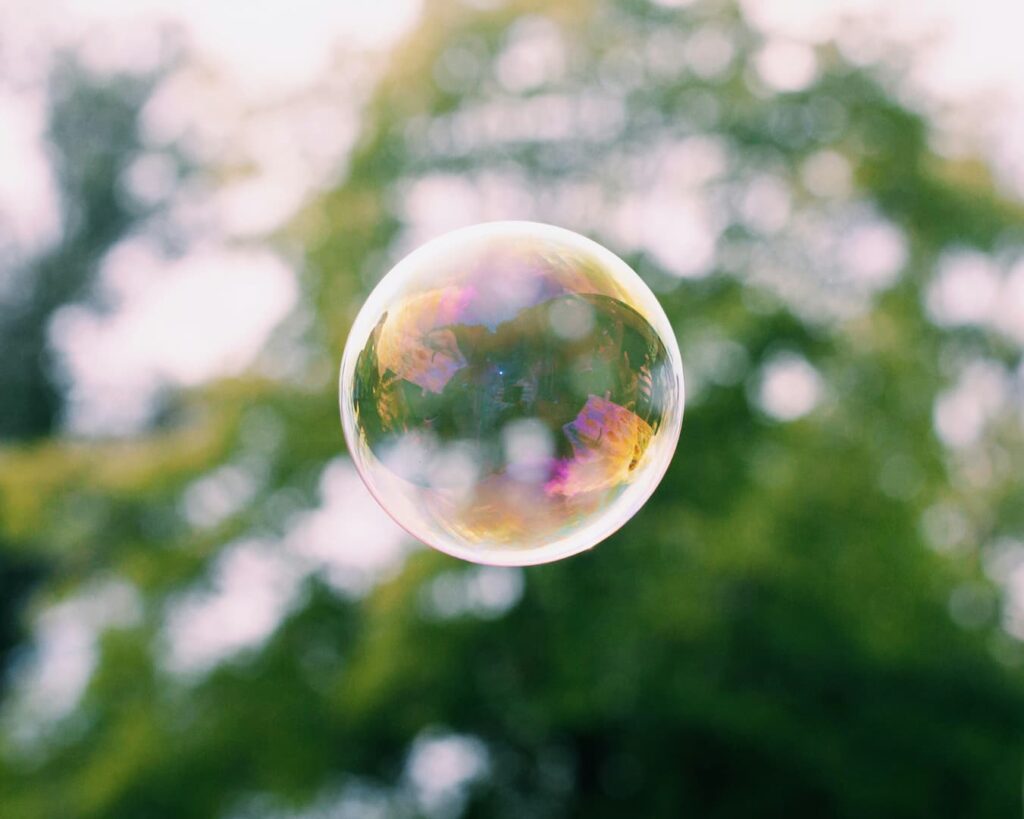
Here’s how to control bubbling and capturing:
- Default Behavior (Bubbling): This is the standard behavior. Events propagate up the DOM tree.
- event.stopPropagation(): This method stops the event from bubbling further up the hierarchy.
- addEventListener(event, handler, useCapture): The third argument, useCapture, is a boolean. Set it to true to make the event listener capture events instead of bubbling.
Imagine a nested menu structure. Clicking a sub-menu item might unintentionally trigger a click event on the main menu as well. Using event.stopPropagation() in the sub-menu’s click handler can prevent this unwanted behavior.
Handling Events Efficiently with Delegation
With a complex web page containing many elements, attaching individual event handlers to each one can become inconvenient and can slow down performance. This is where the event delegation comes to the rescue.
Delegation involves attaching an event handler to a parent element and then checking within the handler function to see which child element triggered the event. This way, you have a single handler for a group of elements.
Here’s how delegation works:
<div id="myList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</div>
<script>
const list = document.getElementById("myList");
list.addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
alert("You clicked item: " + event.target.textContent);
}
});
</script>
In this example, we attach a click handler to the myList element. Inside the handler, we check if the clicked element (event.target) is a list item (LI). If so, we display an alert message with the item’s text content.
Delegation is a powerful technique for handling events on dynamically generated content or large lists of elements.
Handling Keyboard Events
Event handling isn’t just about mouse interactions. JavaScript provides ways to capture keyboard events as well. This makes your web pages more accessible for users who rely on keyboards for navigation.
Here are some common keyboard events:
- keydown: Triggered when a key is pressed down
- keyup: Triggered when a key is released
- keypress: Triggered when a character is pressed (may not work in all browsers)
Imagine a website with a search bar. Using the keydown event, you can detect when the user presses the Enter key and trigger a search based on the entered text. This enhances the user experience for those who prefer keyboard navigation.
Event Objects
When an event occurs, JavaScript provides an event object as an argument to the event handler function. This object contains valuable information about the event, such as:
- event.type: The type of event that occurred (e.g., “click”, “keydown”)
- event.target: The element that triggered the event
- event.currentTarget: The element the event listener is attached to (can be different from event.target in some cases)
- event.clientX & event.clientY: Mouse cursor position coordinates (for mouse events)
- event.keyCode: Key code of the pressed key (for keyboard events)
By accessing these properties within your event handler, you can tailor your code’s response based on the specifics of the event.
Conclusion
Event handling in JavaScript is a powerful tool that forms the foundation for creating dynamic and interactive web pages. By understanding events, event handlers, and best practices, you can transform your web applications into engaging experiences for your users.
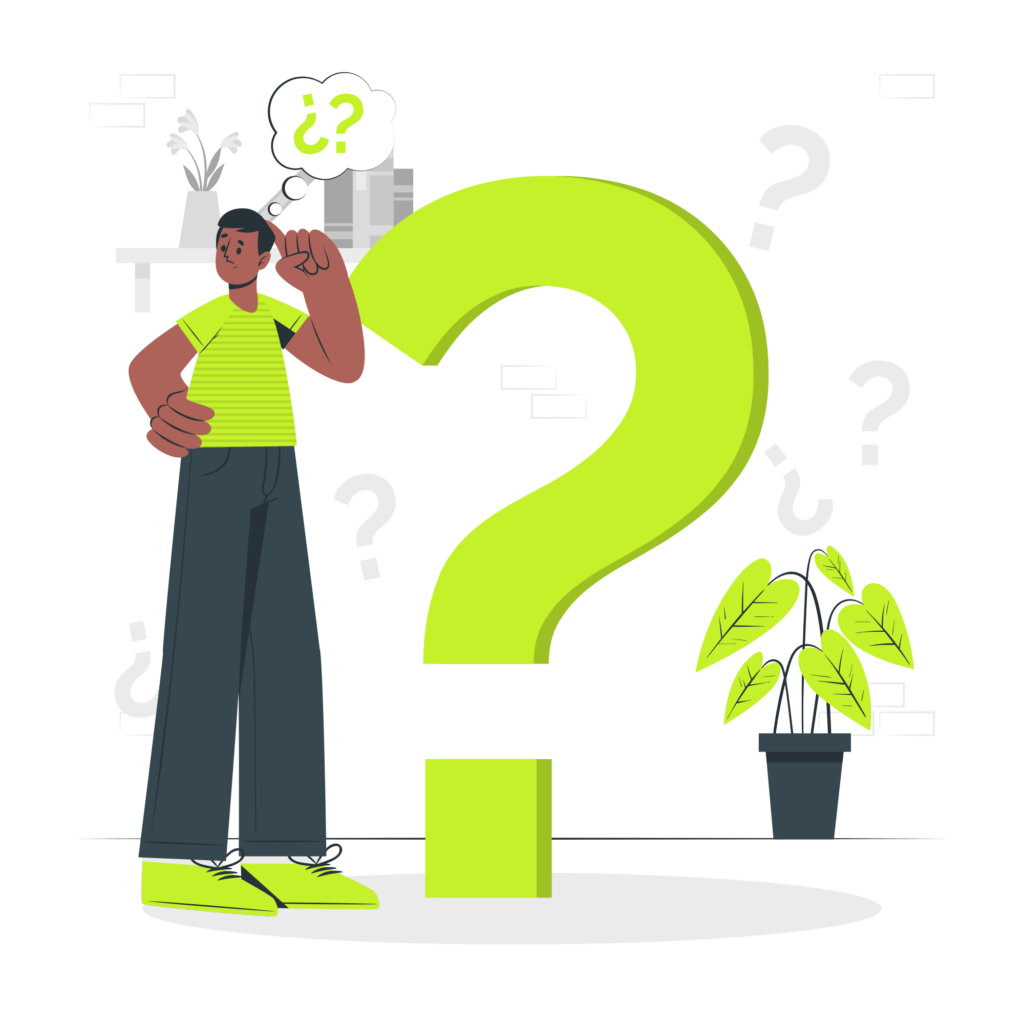
FAQs
What is event handling in JavaScript?
- Event handling involves responding to user actions like clicks, mouse movements, and keyboard inputs on a web page.
How do you add an event listener in JavaScript?
- Use the
addEventListener
method, specifying the event type and the function to be executed when the event occurs.
Can you remove an event listener?
- Yes, using the
removeEventListener
method, specifying the same event type and function used to add it.
What are common event types in JavaScript?
- Common events include
click
,mouseover
,mouseout
,keydown
,keyup
, andload
.
How does event propagation work in JavaScript?
- Event propagation in JavaScript can work in two ways: bubbling (default) and capturing, determining the order event handlers are executed.
What is JavaScript?
- JavaScript is a programming language used to create dynamic content on websites, enhancing user interfaces and experiences.
Why is JavaScript important for web development?
- It enables interactive elements on web pages, making sites more engaging and responsive to user actions.
How can JavaScript improve website usability?
- By allowing dynamic updates without reloading the page, handling form validations, and managing user interactions.
What are some best practices for JavaScript event handling?
- Use event delegation, avoid excessive
document
orwindow
events, and remove unneeded event listeners to improve performance.
Are there any tools to help debug event listeners in JavaScript?
- Yes, most modern browsers have built-in developer tools that can inspect and debug event listeners attached to page elements.