Modern JavaScript offers a wide range of functionalities that enhance the performance and efficiency of code. Among these features, the concept of a closure in JavaScript stands out as a powerful tool that enables better data encapsulation and modularity. This article explores the concept of closure in JS, how it works, and the benefits it provides in JavaScript development to improve code structure and functionality.
What is a Closure ?
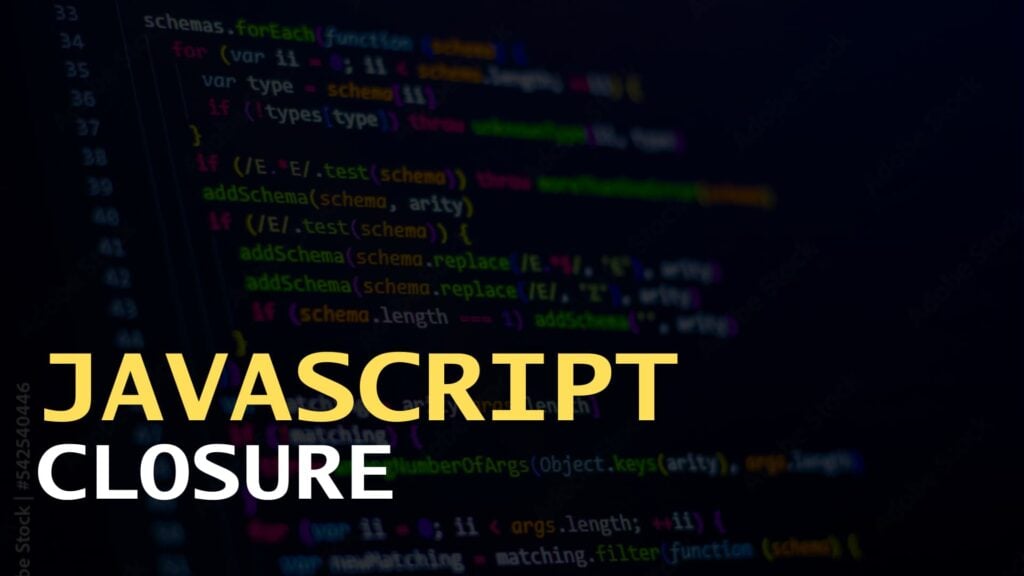
Before delving deep into the concept of closures, let’s first understand what closures are in the context of JavaScript. A closure is a feature in JavaScript where an inner function has access to the outer function’s variables even after the outer function has returned. The scope chain in a closure includes the variables from its own scope, the outer function’s scope, and the global scope. A closure is created when a function is defined inside another function and the inner function access variables from the outer function.
Key Components of Closures
- Inner Function – The function defined inside another function that has access to the outer functions variables.
- Outer Function – The function that defines the variable accessed by the inner function.
- Lexical Environment – The environment in which the function is defined together with all other functions and variables.
How Closures in JavaScript Work ?
When a function is created, it forms a closure with its lexical environment. This means the function retains a reference to the variables in its surrounding scope even after the outer function has finished executing. This enables the inner function to access the outer function’s variables whenever it is called, even if it is called outside the outer function’s scope. Here is an example to illustrate this
// outer function
function outerfunction() {
let outerVariable = "I am an outer Variable";
// inner function
function innerFunction() {
console.log(outerVariable)
}
return innerFunction();
}
let closureFunc = outerfunction();
closureFunc(); // output: I am an outer Variable
When outerFunction
is called, it returns innerFunction
. The returned innerFunction
now has a closure over the outerVariable
. Even though outerFunction
has finished executing, the innerFunction
still has access to outerVariable
. This is simply the concept of closure in Javascript Development.
Importance of Closure in Javascript Development
Closures are a fundamental concept in JavaScript that enable more versatile and powerful programming techniques. They play a crucial role in opening a world of possibilities in JavaScript development. Understanding closures is essential for any JavaScript developer aiming to write efficient and effective code. Here are some common benefits offered by closures
1. Data Privacy and Encapsulation
Encapsulation is a fundamental concept and principle of Object-Oriented Programming (OOP), and in JavaScript, this can be achieved using closures. Closures enable functions to have private variables that cannot be accessed from outside the function, providing a mechanism for creating secure and encapsulated code.
This is particularly useful for defining private methods within objects or modules that cannot be modified from the global scope, thereby reducing the risk of unintended side effects.
function Person() {
let age = 0;
return {
increment: function() {
age++;
console.log(age);
},
decrement: function() {
age--;
console.log(age);
},
getAge: function() {
return age;
}
}
};
let personAge = Person();
personAge.increment(); // output: 1
personAge.decrement(); // output: 0
console.log(personAge.getAge()); // output: 0
In the example provided, we have a function Person
that defines a private variable age
. This variable is not directly accessible from outside the Person
function. Instead, the Person
function returns an object that contains three methods: increment
, decrement
, and getAge
.
These methods form closures over the age
variable, allowing them to access and modify age
even after the Person
function has finished executing.
2. Function Factories that leads to Modular & Maintainable Code
Closures in Javascript enables function factories that generates other functions with preset configuration which enables enhanced modularity and code reusability in Javascript development.
function greetUser(message) {
function greet(name) {
console.log(message + ", " + name)
}
return name;
}
let SayHello = greetUser("Hello");
sayHello("Tom"); // output: Hello, Tom
let sayHi = greetUser("Hi");
sayHi("Alan"); //output: Hi, Alan
In the example provided, we have a function greetUser
that accepts a message
parameter that enables preset configuration resulting is modular and reusable code that can be easily maintained.
Conclusion
Closures are a powerful concept in JavaScript development that enable better modularity and enhances encapsulation in code. The benefits provided by closures facilitate easier programming, particularly when developing complex web applications. Mastering closures is essential for any Javascript developer, providing the tools necessary to solve complex programming challenges and create robust applications.
FAQs
What is a closure in JavaScript?
- A closure in JavaScript is a function that retains access to its outer scope, even after the outer function has finished executing.
Why are closures important in JavaScript?
- Closures are important because they allow functions to maintain references to variables from their outer scope, enabling powerful programming patterns like data encapsulation.
How do closures work in JavaScript?
- Closures work by keeping a reference to the lexical environment where the function was created, allowing it to access variables in its outer scope.
Can you provide an example of a closure in JavaScript?
- Sure! A basic example is a function inside another function that references a variable from the outer function, maintaining access to it even after the outer function completes.
What are common use cases for closures in JavaScript?
- Common use cases include creating private variables, callbacks, event handlers, and maintaining state in asynchronous functions.