In the ever-evolving world of blockchain development, Solidity stands out as the most popular programming language for Ethereum. However, like any other programming language, Solidity comes with its own challenges. When developing smart contracts in solidity, encountering errors is a common part of the coding process. One such error is the “identifier not found or not unique” error. Solving this error could get a lot complexed, particularly for those new to Solidity, as it may seem to arise without clear cause.
This article explores the typical scenarios that lead to this error, break down its root causes, and provide practical solutions to help you overcome it. Understanding the error itself and how to effectively solve it is crucial for ensuring your smart contracts run smoothly and securely on the Ethereum blockchain.
Understanding the Error
Before exploring possible solutions to solve the error, let’s first try to understand the root causes that could possibly lead us to this situation. Understanding the error itself could make the resolving process a lot easier. The “identifier not found or not unique” is a common error encountered by Solidity developers which gets a lot complexed to find a solution. This could happen for several reasons. By breaking down the various causes behind this error, you’ll be better equipped to identify the problem in your code and implement the necessary fixes.
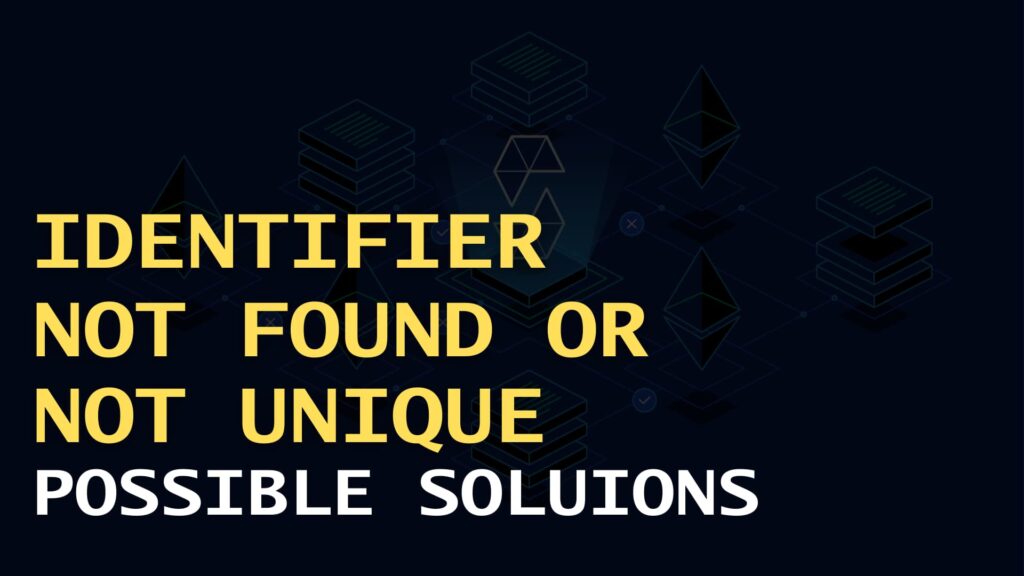
The “identifier not found or not unique” could arise in several scenarios. Following are some of the common causes that could produce this error.
1. Typographical Errors
In Solidity, an identifier name refers to the name given to variables, functions, contracts, and other entities within your code. A simple typo in the identifier name can cause the compiler to fail in recognizing the identifier. This could be one of the common causes that could lead to the error.
2. Scope Issues
When an identifier is defined on a different scope from where it’s being used, the compiler might not be able to find it. This could cause the error to be thrown because any used identifier will not be recognized by the compiler if it’s not defined or imported from another scope.
3. Missing Imports or Dependancies
While developing smart contracts, there are scenarios where a developer could use external libraries. If such libraries or contracts haven’t been imported correctly, the compiler won’t be able to find the identifier. This could cause the error to be thrown as the compiler is unable to recognize the library being used in the contract.
4. Naming Conflicts
While writing code in Solidity, we use identifiers for various situations. These identifiers are to be unique from each other. Multiple identifiers with the same name in overlapping scopes or inheritance hierarchies can lead to this error.
5. Incorrect Case Sensitivity
Solidity is case-sensitive, so even a small difference in case can cause the identifier to be unrecognized.
Possible Solutions
Encountering the “identifier not found or not unique” error could be a lot frustrating, but it’s usually a sign of a fixable issue within your code. There are several strategies you can employ to resolve it. In this section, we’ll walk you through a range of practical solutions that address the various root causes of this error that we discussed, helping you get your smart contract back on track.
1. Check for Typographical Errors
Do a proper check on the spelling of the identifier. Even a small typo can lead to the compiler being unable to find the correct identifier. Additionally, you could utilize the auto-completion features in your IDE to minimize the risk of typos. This features comes built in with almost every IDE available on the market.
uint public myVariable = 100;
function getMyVariable() public view returns (uint) {
return myVarible // incorrect: Typo in 'myVariable'
}
In the provided Solidity code, there’s a typo in the getMyVariable
function. Specifically, the variable name is misspelled as myVarible
instead of myVariable
. A simple way to solve this is to spell the identifier correctly.
2. Verify Identifier Scope
Ensure the identifier you are trying to access is defined within the correct scope. For instance, if you’re trying to access a variable or function inside a different function or contract, make sure it’s declared in a scope that is accessible. If you are working on complexed inheritance hierarchy, ensure that the identifiers are visible in the current scope.
contract MyContract {
// identifier defined inside scope.
uint private myVariable = 100;
function getMyVariable() public view returns (uint) {
return myVariable; // Correct usage within scope
}
function exampleFunction() public view returns (uint) {
return anotherVariable; // Error: anotherVariable is not defined in scope
}
}
In the provided Solidity code, myVariable
is correctly accessed because it is within the same scope where myVariable
was declared. However, in the exampleFunction
, there is an attempt to return the value of anotherVariable
, which has not been defined anywhere in the contract. Since anotherVariable
does not exist in the current scope. Since anotherVariable
does not exist in the current scope the compiler throws an error stating that the identifier is not recognized.
3. Ensure Proper Imports and Dependencies
Ensure that all necessary libraries are imported correctly into the smart contract and is accessable by the current scope. Missing paths in the import statement could result in errors where identifiers cannot be identified by the compiler.
import './MyContract.sol'
In the above import statement, we are trying to import a Solidity file into our contract. It is important to ensure that MyContract.sol
is available inside the working directory.
4. Resolve Name Conflicts
There could be scenarios where two identifiers could be defined using the same name. This could cause the Solidity compiler to throw an error. You could simply solve this issue by renaming one of them and ensuring they stay unique within their respective scopes.
uint private myVariable = 100; // Identifier defined with a unique name
// cannot use myVariable again since it is already defined within the scope
uint private myVariable = 100; // Compiler throws an error.
5. Fix Case Sensitivity Issues
Ensuring that you are consistent with the use of uppercase and lowercase letters in your identifiers could save you from encountering such issues. You could simply solve this by comparing the identifier usage with its declaration and ensuring there are no any case-sensitivity problems.
uint private myVariable = 100; // Variable declared
function getMyVariable() public view returns (uint) {
return MyVariable; // Error: MyVariable is not defined in scope
}
In this example, a variable myVariable
is declared and initialized with a value of 100
. The variable name is written in lowercase. However, in the getMyVariable
function, there’s an attempt to return a value using MyVariable
, which has an uppercase “M” at the beginning. This causes an error because Solidity is case-sensitive.
6. Use of Explicit References
If any of the above doesn’t seem to be an issue, then it could be something else that is causing the compiler to thrown an “identifier not found or not unique” error. This could be a case where there are multiple contracts with similar functions or variables. You could simply use explicit references to avoid the confusion.
// use explicit reference to call funcitons from another file.
ConttractName.FunctionName()
7. Check Compiler Versions
Sometimes the error might occur due to different compiler versions and the difference on how they handle certain cases. You can find possible solutions by ensuring that your code is compatible with the Solidity version you’re using. Use the pragma
directive to specify the Solidity version.
pragma solidity ^0.8.0; // ensure the version you use is compatitable
Conclusion
The “identifier not found or not unique” error in Solidity could be a lot frustrating to find a solution only until you could understand the exact cause of the issue. By methodically checking for common issues like typos, scope problems, name conflicts, and import errors, you can usually resolve it quickly. Understanding why this error occurs is the first step toward effectively troubleshooting and resolving it. As you gain experience, these errors will become easier to diagnose and fix, making you a more proficient Solidity developer.
FAQs
What does the “DeclarationError: Identifier not found or not unique” in Solidity mean?
- This error occurs when a variable, function, or type in Solidity is either not declared or has a name conflict, making it hard for the compiler to identify the correct one.
How can I fix the “Identifier not found or not unique” error in Solidity?
- Check for typos in variable names, ensure all identifiers are properly declared, and avoid naming conflicts by using unique identifiers.
Why does Solidity show “Identifier not found”?
- This happens when the compiler encounters a name that hasn’t been declared or imported, meaning it can’t find the associated definition in the current scope.
Can I prevent “Identifier not unique” errors in my Solidity code?
- Yes, by avoiding duplicate names within the same scope and being careful with scope management, you can prevent this error.
What tools can help debug Solidity errors like “Identifier not found”?
- Tools like Remix IDE, Truffle, and Solidity compiler warnings can help identify and resolve such errors by providing detailed feedback.