The Ethernaut Level 2 offers a fascinating glimpse into the vulnerabilities that can arise from seemingly minor oversights in smart contract development. This article takes you through the challenge, revealing the misstep in the contract’s construction and guiding you through the process of exploiting it. Furthermore, we explore the evolution of constructors in Solidity, providing a comprehensive understanding of how this aspect of Solidity has matured and how it impacts smart contract security and development.
The Fallout Challenge: A Case Study in Smart Contract Vulnerability
At the heart of the Ethernaut Challenge 2 is a smart contract named Fallout, which contains a critical flaw due to an incorrectly named constructor function. This vulnerability stems from a period in Solidity’s history when constructors were defined by naming a function after the contract. This method led to potential risks, especially if the contract was renamed without updating the constructor function’s name, thereby leaving the function open to being called by anyone.
// SPDX-License-Identifier: MIT
pragma solidity ^0.6.0;
import 'openzeppelin-contracts-06/math/SafeMath.sol';
contract Fallout {
using SafeMath for uint256;
mapping (address => uint) allocations;
address payable public owner;
/* constructor */
function Fal1out() public payable {
owner = msg.sender;
allocations[owner] = msg.value;
}
modifier onlyOwner {
require(
msg.sender == owner,
"caller is not the owner"
);
_;
}
function allocate() public payable {
allocations[msg.sender] = allocations[msg.sender].add(msg.value);
}
function sendAllocation(address payable allocator) public {
require(allocations[allocator] > 0);
allocator.transfer(allocations[allocator]);
}
function collectAllocations() public onlyOwner {
msg.sender.transfer(address(this).balance);
}
function allocatorBalance(address allocator) public view returns (uint) {
return allocations[allocator];
}
}
Exploiting the Misnamed Constructor
The challenge revolves around the fact that the Fal1out function, intended as the constructor, was misnamed. This oversight allowed any user to call this function, claim ownership of the contract, and expose a significant security vulnerability. The solution involves interacting with the contract to call this misnamed function, demonstrating a critical lesson in the importance of precise syntax and naming conventions in smart contract development.
The Evolution of Constructors in Solidity
The journey of constructors in Solidity from version-specific functions to the adoption of the constructor keyword reflects the language’s evolution toward enhanced safety, readability, and developer experience.
Early Versions (Before 0.4.22)
Originally, Solidity utilized a naming convention for constructors that required the function to share the name with the contract. This approach, while straightforward, introduced a vulnerability to renaming errors—a concern highlighted by our exploration of the Ethernaut Challenge 2.
// Example of an old-style constructor
contract MyContract {
uint public value;
function MyContract(uint _value) public {
value = _value;
}
}
Introduction of the constructor Keyword (0.4.22 and Later)
The introduction of the constructor keyword in Solidity 0.4.22 marked a significant leap forward, eliminating the risk associated with contract renaming and enhancing code clarity.
// Example with the `constructor` keyword
contract MyContract {
uint public value;
constructor(uint _value) public {
value = _value;
}
}
Current Best Practices
Today, the best practice involves using the constructor keyword without visibility specifiers, a testament to Solidity’s ongoing development aimed at improving security and developer experience.
// Current best practice example
contract MyContract {
uint public value;
constructor(uint _value) {
value = _value;
}
}
Implementing the Solution to the Challenge
Understanding the historical context and evolution of constructors in Solidity enhances our appreciation for the solution to the Ethernaut Challenge 2. By exploiting the misnamed constructor vulnerability, we not only navigate the challenge but also engage with a practical example of how Solidity’s development has sought to mitigate such risks.
Steps to Claim Ownership
- Identifying the misnamed constructor (Fal1out).
- Interacting with the contract to call this function. Setting the owner to be the
msg.sender
- Verifying ownership transfer through automated testing and interaction scripts, or also through the Ethernaut broswer console.
This approach, while specific to the challenge, offers broader lessons in smart contract security, attention to detail, and the importance of staying updated with Solidity’s best practices.
Conclusion: Lessons Beyond the Challenge
The Ethernaut Challenge 2, set against the backdrop of Solidity’s evolution, provides a rich learning experience that extends beyond the specifics of the challenge. It emphasizes the importance of understanding the fundamentals of smart contract development, the implications of language updates, and the continuous need for diligence and best practices in the blockchain development space. As Solidity and the Ethereum ecosystem evolve, staying informed and engaged with these developments remains crucial for developers aspiring to build secure, efficient, and reliable smart contracts.
If you are curious about the Fallback Challenge, don’t worry! we got your back. Go checkout our article about the Ethernaut Challenge 1 here.
Ready for the next Ethernaut challenge? Click to see what’s next in our series!
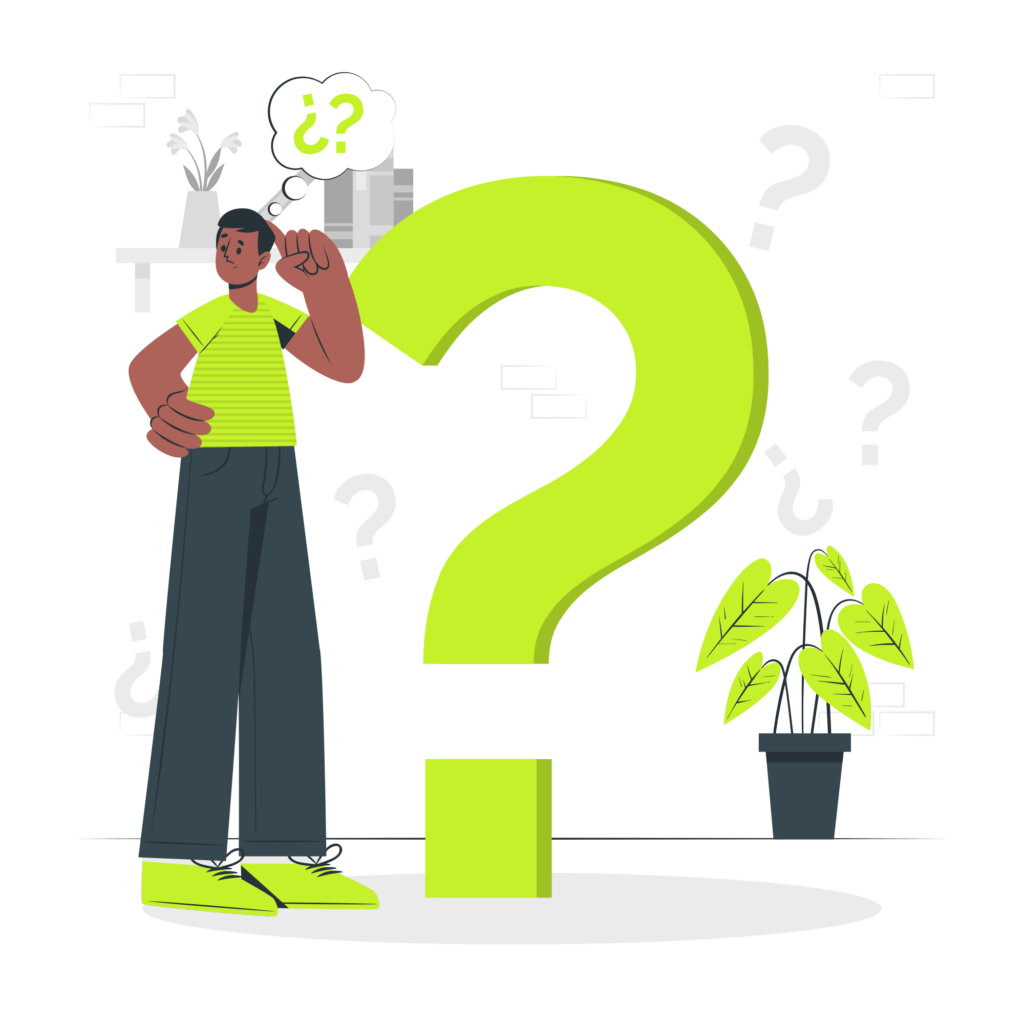
FAQs:
What is the Ethernaut Challenge 2 about?
- The Ethernaut Challenge 2, known as “Fallout,” is a smart contract puzzle that involves exploiting a vulnerability due to an incorrectly named constructor function. Participants are tasked with claiming ownership of the contract by calling this misnamed function.
Why does the misnamed constructor in the Fallout contract pose a vulnerability?
- In Solidity, constructors are meant to be called once upon contract deployment to initialize the contract’s state. The Fallout contract’s constructor was incorrectly named, making it a regular function callable by anyone. This allows an attacker to claim ownership of the contract by calling the misnamed function.
How did Solidity’s approach to constructors evolve over time?
- Initially, Solidity used a naming convention for constructors where the constructor function had the same name as the contract. This changed with Solidity 0.4.22, which introduced the
constructor
keyword, making contract initialization clearer and less prone to errors related to contract renaming.
What are the current best practices for defining constructors in Solidity?
- As of the latest Solidity versions, the best practice is to use the
constructor
keyword without visibility specifiers. This approach is more secure and intuitive, eliminating the risks associated with the old naming convention and making the code more readable.
Can constructors have visibility specifiers in Solidity?
- Initially, constructors could have visibility specifiers like
public
orinternal
. However, with the introduction of theconstructor
keyword and subsequent Solidity versions, setting visibility for constructors became unnecessary and is now disallowed. Constructors are implicitly internal and are called only once upon contract creation.
Why is understanding the evolution of Solidity important for developers?
- Keeping up with Solidity’s evolution helps developers write safer, more efficient, and up-to-date code. Understanding changes, such as the transition to the
constructor
keyword, allows developers to avoid common pitfalls and vulnerabilities in smart contract development.