In the world of blockchain and smart contracts, security is paramount. However, there’s a fine line between ensuring security and creating rigid, inflexible systems. One common pitfall in smart contract design is excessive function restriction. While well-intended, overly restrictive functions can limit a contract’s usability and adaptability. In this article, we’ll explore the concept of excessive function restriction, its implications, and how to find a balance between security and usability.
Understanding Excessive Function Restriction
Excessive function restriction refers to the practice of implementing overly strict limitations on the functionalities of a smart contract. This usually stems from a desire to enhance security by limiting who can execute certain functions and under what conditions. While this can protect against malicious actors, it can also create significant hurdles for legitimate users and future upgrades.
Consider a smart contract designed to send funds only to specific addresses on a whitelist. Here’s a simplified version of what such a contract might look like:
pragma solidity ^0.8.0;
contract WhitelistedTransfer {
address public owner;
mapping(address => bool) public whitelist;
modifier onlyOwner() {
require(msg.sender == owner, "Not the contract owner");
_;
}
modifier onlyWhitelisted(address _to) {
require(whitelist[_to], "Address not whitelisted");
_;
}
constructor() {
owner = msg.sender;
}
function addToWhitelist(address _addr) public onlyOwner {
whitelist[_addr] = true;
}
function removeFromWhitelist(address _addr) public onlyOwner {
whitelist[_addr] = false;
}
function sendFunds(address _to, uint256 _amount) public onlyOwner onlyWhitelisted(_to) {
require(address(this).balance >= _amount, "Insufficient balance");
payable(_to).transfer(_amount);
}
receive() external payable {}
}
In this example, the contract only allows the owner to send funds to addresses on a whitelist. While this enhances security, it also means that if a legitimate need arises to send funds to a new, unlisted address, the contract cannot accommodate it without modification.
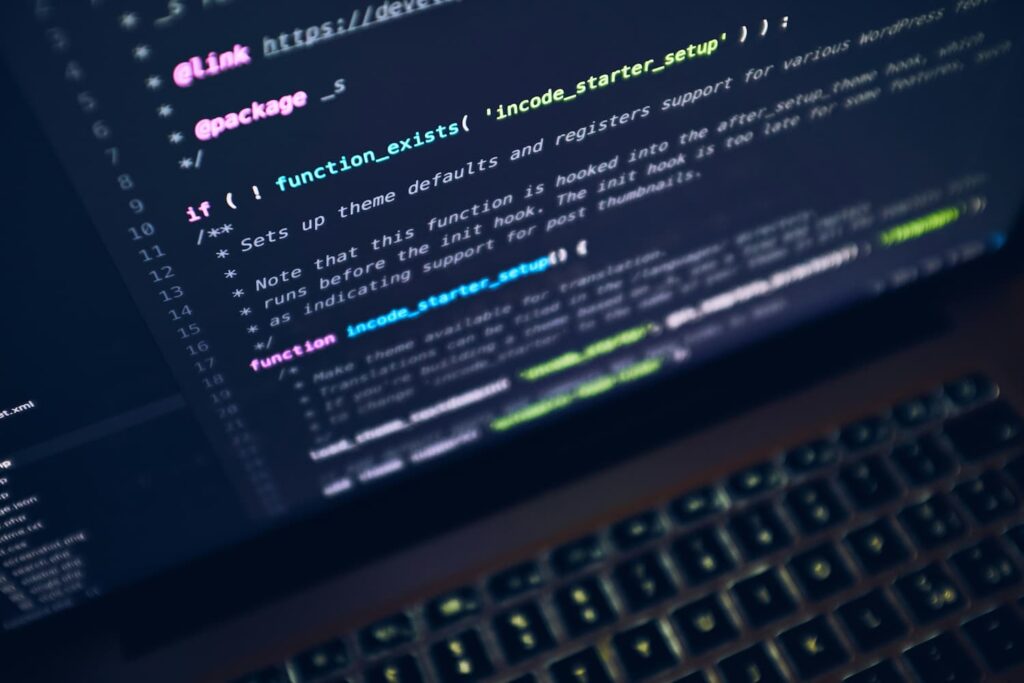
Decentralized Exchange (DEX) Limitations
Decentralized Exchanges (DEXs) are another area where excessive function restriction can have adverse effects. Many DEXs rely on smart contracts to facilitate token trading. Some early DEX implementations had overly restrictive functions, limiting the types of trades that could be executed. This rigidity hindered user experience and adoption compared to more flexible DEXs.
Here is an example. Imagine a DEX contract that only allows trading between specific token pairs:
pragma solidity ^0.8.0;
contract RigidDEX {
address public tokenA;
address public tokenB;
constructor(address _tokenA, address _tokenB) {
tokenA = _tokenA;
tokenB = _tokenB;
}
function tradeAForB(uint256 _amount) public {
// Logic to trade tokenA for tokenB
}
function tradeBForA(uint256 _amount) public {
// Logic to trade tokenB for tokenA
}
}
In this case, the contract only supports trades between tokenA and tokenB. If users want to trade other tokens, they’re out of luck unless the contract is modified or redeployed, which can be costly and inconvenient.
Finding the Balance
Achieving a balance between security and usability is crucial in smart contract design. Here are some strategies to avoid excessive function restriction:
Future-Proofing
When designing a smart contract, consider potential future needs and leave room for reasonable modifications. For example, instead of hardcoding specific addresses or token pairs, use a more flexible approach that can be updated as needed.
pragma solidity ^0.8.0;
contract FlexibleDEX {
mapping(address => mapping(address => bool)) public supportedPairs;
function addPair(address _tokenA, address _tokenB) public {
supportedPairs[_tokenA][_tokenB] = true;
}
function removePair(address _tokenA, address _tokenB) public {
supportedPairs[_tokenA][_tokenB] = false;
}
function trade(address _fromToken, address _toToken, uint256 _amount) public {
require(supportedPairs[_fromToken][_toToken], "Pair not supported");
// Logic to trade _fromToken for _toToken
}
}
In this example, the contract can support new token pairs without requiring a redeployment.
Configurable Options
Implement configuration options that allow for adjustments without requiring code changes. For instance, using a dynamic whitelist that the contract owner can update ensures that the contract can adapt to changing needs.
pragma solidity ^0.8.0;
contract ConfigurableWhitelist {
address public owner;
mapping(address => bool) public whitelist;
modifier onlyOwner() {
require(msg.sender == owner, "Not the contract owner");
_;
}
modifier onlyWhitelisted(address _to) {
require(whitelist[_to], "Address not whitelisted");
_;
}
constructor() {
owner = msg.sender;
}
function addToWhitelist(address _addr) public onlyOwner {
whitelist[_addr] = true;
}
function removeFromWhitelist(address _addr) public onlyOwner {
whitelist[_addr] = false;
}
function sendFunds(address _to, uint256 _amount) public onlyOwner onlyWhitelisted(_to) {
require(address(this).balance >= _amount, "Insufficient balance");
payable(_to).transfer(_amount);
}
receive() external payable {}
}
Here, the owner can dynamically update the whitelist, allowing for greater flexibility.
Emergency Shutdown
Including a secure emergency shutdown mechanism can help address critical situations without compromising the contract’s overall security. This allows for a quick response to unforeseen issues while maintaining the integrity of the contract.
pragma solidity ^0.8.0;
contract EmergencyShutdown {
address public owner;
bool public isShutdown;
modifier onlyOwner() {
require(msg.sender == owner, "Not the contract owner");
_;
}
modifier notShutdown() {
require(!isShutdown, "Contract is shutdown");
_;
}
constructor() {
owner = msg.sender;
isShutdown = false;
}
function shutdown() public onlyOwner {
isShutdown = true;
}
function restart() public onlyOwner {
isShutdown = false;
}
function criticalFunction() public notShutdown {
// Critical logic here
}
}
In this example, the owner can shut down or restart the contract as needed, providing a failsafe mechanism.
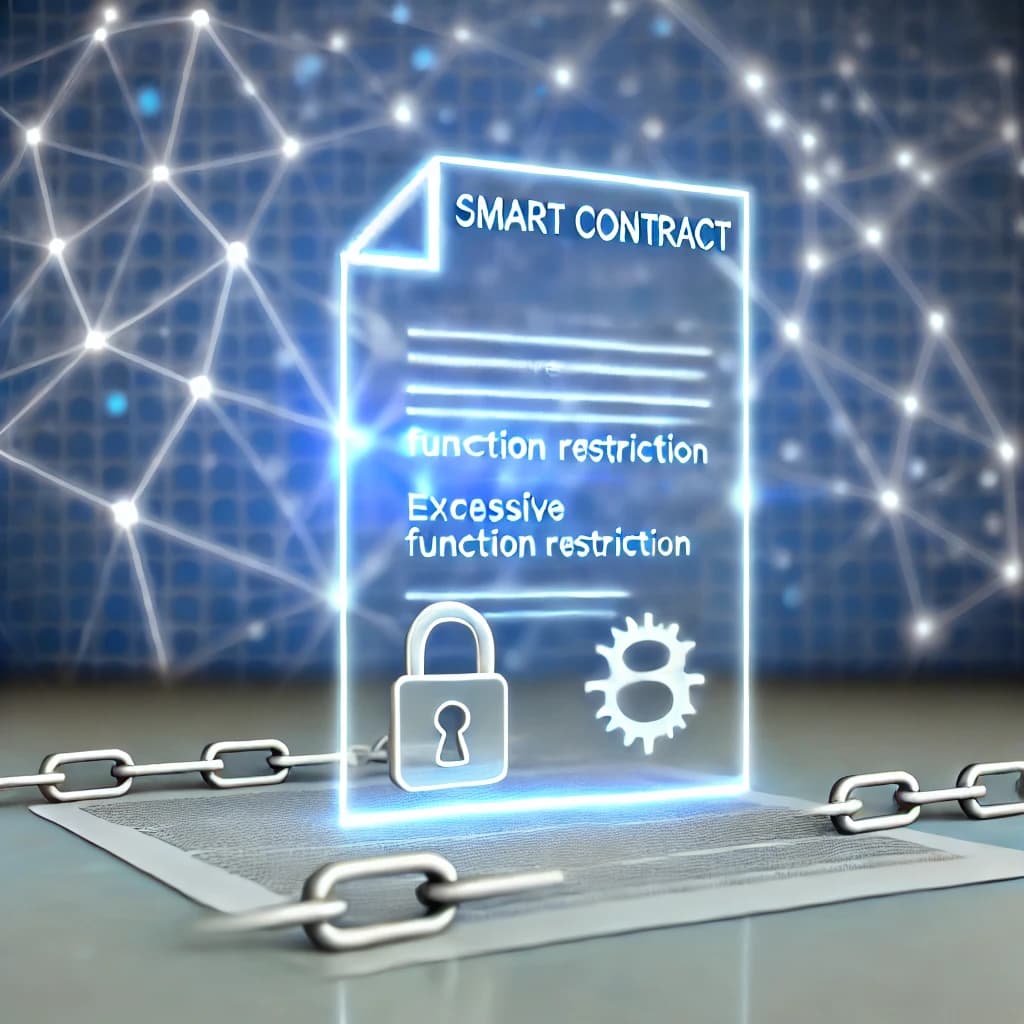
Conclusion
Excessive function restriction can cripple the usability and adaptability of a smart contract. While security is crucial, it should not come at the expense of flexibility and future-proofing. By adopting a balanced approach that prioritizes both security and usability, developers can create robust and user-friendly contracts that stand the test of time.
When designing smart contracts, it’s essential to consider potential future needs, implement configurable options, and include emergency shutdown mechanisms. By doing so, developers can avoid the pitfalls of excessive function restriction and create contracts that are both secure and adaptable.
In summary, achieving the right balance in smart contract design requires foresight, flexibility, and a willingness to adapt. By keeping these principles in mind, developers can ensure their contracts are both secure and user-friendly, paving the way for broader adoption and success in the blockchain ecosystem.
FAQs:
Why are excessive function restrictions problematic in smart contracts?
- They can limit the flexibility and functionality of smart contracts, leading to inefficiencies and increased complexity.
How do function restrictions impact blockchain efficiency?
- Excessive restrictions can lead to increased gas costs and slower transaction times, reducing overall blockchain performance.
What are some solutions to optimize smart contract performance?
- Solutions include simplifying contract logic, optimizing code, and implementing only necessary restrictions to ensure security and efficiency.
How can developers ensure their smart contracts are efficient?
- By conducting thorough testing, using optimization tools, and following best practices for smart contract development.
What role does blockchain security play in function restrictions?
- Security is crucial, but overly restrictive functions can hinder performance. Balancing security and functionality is key.
How do function restrictions affect decentralized applications (DApps)?
- They can limit the capabilities of DApps, making them less user-friendly and potentially reducing their adoption.
What best practices should be followed for smart contract optimization?
- Use modular design, avoid complex logic, and regularly audit contracts for potential improvements.
Can excessive restrictions lead to higher gas costs?
- Yes, more complex and restrictive functions can increase gas consumption, leading to higher costs for users.
What is the impact of function restrictions on user experience in blockchain applications?
- Restrictions can lead to slower, less responsive applications, negatively affecting the user experience.