What are Solidity Events?
Solidity events are a way for smart contracts to communicate with each other and with external applications. They allow developers to track all significant actions and transactions in a contract, providing crucial information about the state and interaction of the contract. They can also be used to promote transparency and accountability in the blockchain ecosystem.
The Beauty of Solidity Events
One of the most beautiful things about Solidity events is that they promote transparency and accountability in the blockchain ecosystem. Developers can emit events and declare events that anyone can access. This means that anyone can see what is happening in a contract, which helps to build trust with users.
What is Solidity emit
?
In Solidity, emit
is a keyword used to trigger events. These events are inheritable members of contracts. When you use emit
, followed by the event name and its parameters, it logs the event to the blockchain. This means that the event is stored on the blockchain and can be accessed and listened to externally.
Here’s an example of how Solidity emit might be used in a smart contract:
pragma solidity ^0.8.0;
contract MyContract {
event MyEvent(address indexed sender, uint256 value);
function myFunction(uint256 _value) public {
// Some logic
emit MyEvent(msg.sender, _value);
}
}
In this example, MyEvent
is emitted every time myFunction
is called, logging the sender’s address and the value passed to the function. External applications can listen to MyEvent
to track these calls.
What does Declaring or Emitting an event mean?
Declaring an event | Emitting an event |
---|---|
To declare an event, you use the event keyword, followed by the event name and input parameters. For example, the following code declares an event called Deposit: | To emit an event, you use the emit keyword, followed by the name of the event and the values of the input parameters. For example, the following code emits the Deposit event: |
#Code snippetevent Deposit(address indexed _from, bytes32 indexed _id, uint _value); | #Code snippetemit Deposit(msg.sender, _id, msg.value); |
The indexed keyword tells Solidity to store the value of the parameter in the event log. The address type is used to represent an Ethereum address. The bytes32 type is used to represent a 32-byte value. The uint type is used to represent an unsigned integer. | The msg. sender variable refers to the address of the sender of the transaction that emitted the event. The _id and _value variables are the values of the input parameters to the Deposit event. |
What are the various Solidity Event Types?
Smart contracts can communicate with one another and with external apps using solidity events. They give vital information about the status and interaction of the contract and enable developers to keep track of all major activities and transactions in a contract.
Solidity events come in two varieties: indexed events and non-indexed events.
- Indexed events are events that are indexed have parameter values that are stored in a form that makes them indexable. This implies that it is simple to run a query over the event log to retrieve all events of a specific category.
- Non-indexed events are events that are not easily indexable due to the manner the parameter values are stored are known as non-indexed events. This implies that it is difficult to search the event log for all events of a specific category.
Indexed events are generally used by developers since they make it simple to follow the occurrence of particular events. An indexed event, for instance, might be used to monitor the exchange of tokens between two accounts.
Here is an example of an indexed event:
- Code snippet
event Transfer(
address indexed _from,
address indexed _to,
uint256 _value
);
In this example, the Transfer event has three input parameters: _from
, _to
, and _value
. The indexed
keyword tells Solidity to store the value of the _from
and _to
parameters in a way that can be easily indexed. This means that you can easily query the event log to find all events of the Transfer type where the _from
and _to
parameters are equal to specific values.
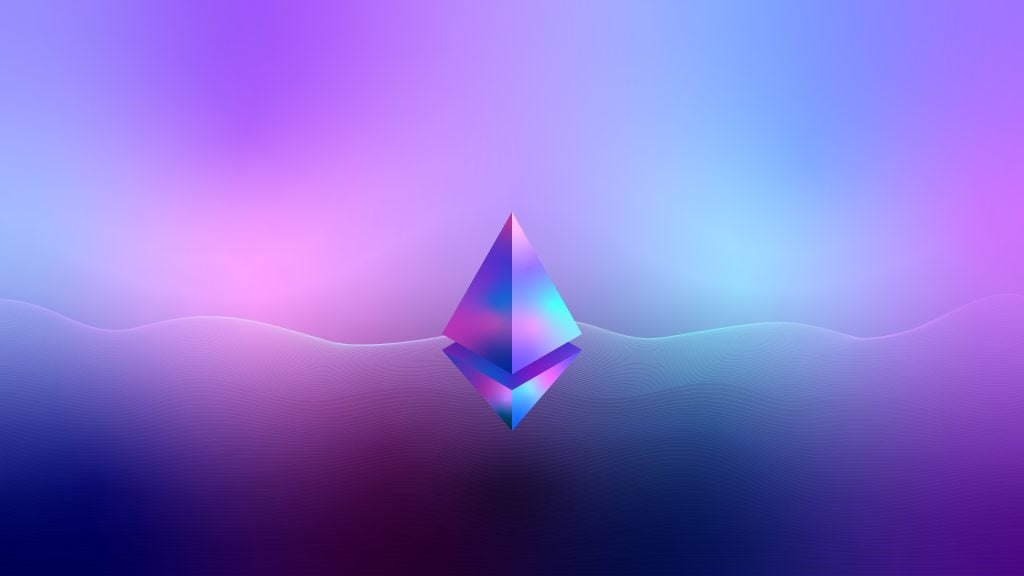
What are the differences between Functions and Events in Solidity?
Functions and events are two of the most important concepts in Solidity, the programming language used to create smart contracts. While they are both used to define the behavior of a smart contract, they serve different purposes.
Functions | Events |
---|---|
Functions define the behavior of a smart contract. They are used to perform actions such as transferring tokens, creating new accounts, and storing data. Functions can be called by other contracts or by external applications. | Events serve as signals that indicate to external interfaces and dApps when a particular action has occurred within the contract. Events are emitted by functions, and they contain information about the action that was performed. Events can be listened to by other contracts or by external applications. |
Here is an example to illustrate the difference between functions and events:
contract Example {
event Transfer(address indexed _from, address indexed _to, uint256 _value);
function transfer(address to, uint256 value) public {
// This is a function. It modifies the state of the contract by transferring tokens.
emit Transfer(msg.sender, to, value);
}
}
In this example, the transfer
function transfers tokens from the current address to the specified address. It also emits the Transfer
event, which logs the transfer.
The main difference between functions and events is that functions modify the state of the contract, while events only log information about what has happened. Functions can also return a value, while events cannot.
In this example, the transfer
function modifies the state of the contract by transferring tokens. The Transfer
event simply logs the transfer, it does not modify the state of the contract.
Here is a table summarizing the key differences between functions and events in Solidity:
Feature | Function | Event |
---|---|---|
Modifies state | Yes | No |
Returns value | Can | Cannot |
Logs information | No | Yes |
What are applications of Solidity Events?
Solidity events are a versatile tool that can be used in a variety of ways in smart contract development. Depending on the project’s needs, events can be used to track state changes, trigger notifications, log data, and more.
Here are a few examples:
- Audit Trails: Solidity events enable developers to create public audit trails of all contract interactions, making it possible to track the contract’s state and transactions.
- Notification Systems: Solidity events can trigger notifications to external interfaces or dApps, such as email or SMS alerts, letting them know when a certain activity has taken place within a contract.
- Token Transfers: Solidity developers often use events to track token transfers within contracts, a critical function for tracking ownership and flow.
- Automated Testing: Testing is an essential part of smart contract development. With events, developers can set up automated testing frameworks to verify their contracts’ correctness and maintainability.
Why Should You Use Solidity Events In A Smart Contract?
Solidity events have many functional advantages over other means of contract communication:
- Transparency: they promote transparency by publicly logging all contract interactions and transactions, which are easy to access and audit.
- Efficiency: they are lightweight ways of communicating between smart contracts and external interfaces or dApps. They reduce the number of unnecessary blockchain interactions required, which in turn increases efficiency.
- Flexibility: they allow developers to exchange data flexibly between smart contracts and external interfaces or dApps. They can include multiple input parameters and can occur at any point in contract execution.
To sum up, Solidity events are a flexible and effective mechanism that can be utilized to increase the effectiveness, accountability, and transparency of blockchain systems. There are numerous internet resources accessible if you’re interested in learning more about Solidity events. After gaining a foundational understanding, you can begin creating your own dApps and advancing the blockchain ecosystem.
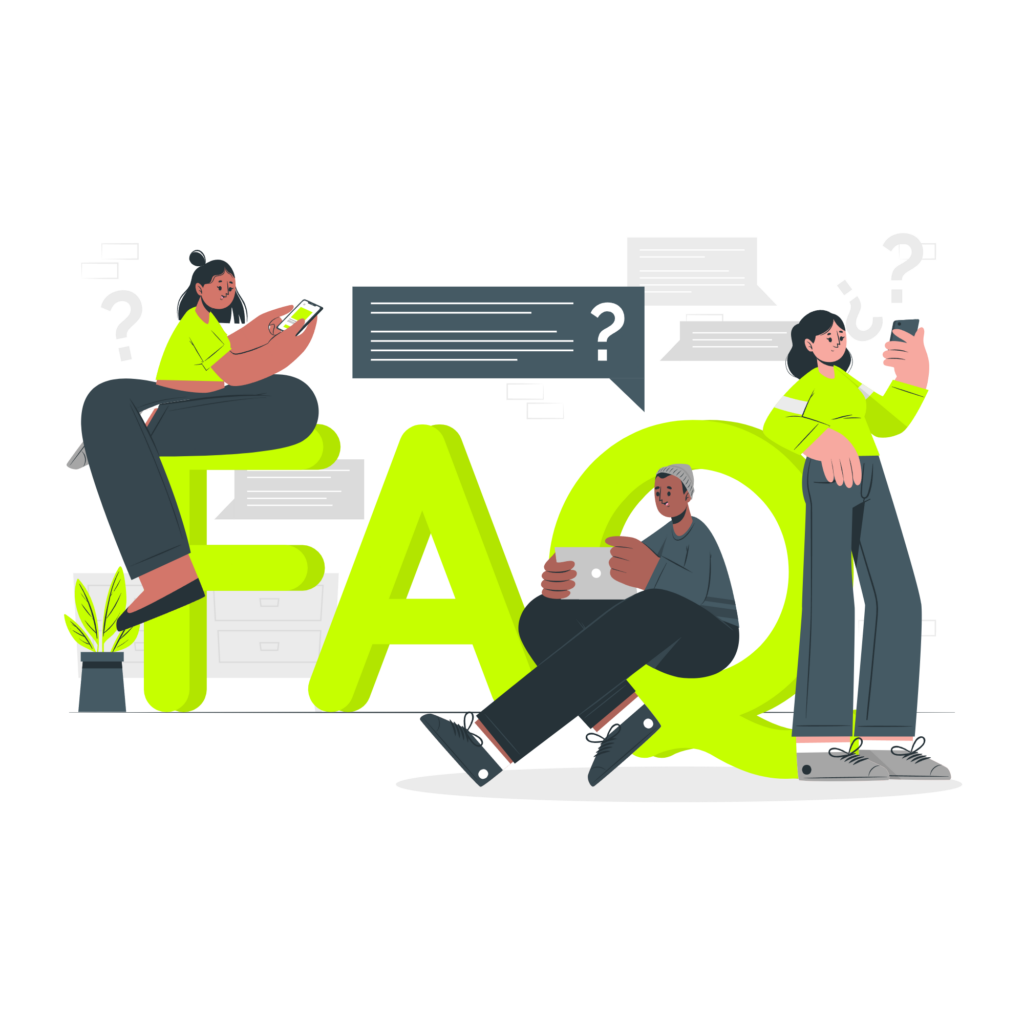
- What are Solidity events in ethers?
Solidity events in ethers are events that are emitted by smart contracts on the Ethereum blockchain. They can be used to track the state of the contract, such as when a token is transferred or a new account is created.
- What are events in Ethereum?
Events in Ethereum are similar to events in Solidity. They are emitted by smart contracts and can be used to track the state of the contract. However, events in Ethereum are also stored on the blockchain, which makes them accessible to anyone.
- What is the difference between function and event in Solidity?
The main difference between functions and events in Solidity is that functions modify the state of the contract, while events only log information about what has happened. Functions can also return a value, while events cannot.
- What are the parameters of Solidity event?
The parameters of a Solidity event are the values that are logged when the event is emitted. The parameters can be of any data type, including integers, floats, strings, and addresses.
- What is the size limit of Solidity event?
The size limit of a Solidity event is 320 bytes. This includes the name of the event, the parameters, and the data types of the parameters.
- What are data types in Solidity?
Data types in Solidity are used to define the type of data that can be stored in a variable. The main data types in Solidity are:
- Integers
- Floats
- Strings
- Booleans
- Addresses
- Arrays
- Structs
- Mappings
- What are the 4 main data types?
The 4 main data types in Solidity are:
- Integers: Integers are numbers without decimals.
- Floats: Floats are numbers with decimals.
- Strings: Strings are sequences of characters.
- Booleans: Booleans are values that can be either true or false.
- What are the 7 different data types?
The 7 different data types in Solidy are:
- Integers (int, uint)
- Floats (float, double)
- Strings (string)
- Booleans (bool)
- Addresses (address)
- Arrays (array)
- Structs (struct)
- Mappings (mapping)
- What are the 3 main types of data?
The 3 main types of data are:
- Primitive data types: Primitive data types are the basic building blocks of data. They are integers, floats, strings, and booleans.
- Structured data types: Structured data types are made up of primitive data types. They are arrays, structs, and mappings.
- Reference data types: Reference data types are pointers to other data types. They are contracts and functions.
- What are the 5 main data types in databases?
The 5 main data types in databases are:
- Text: Text is a string of characters.
- Numeric: Numeric is a number.
- Boolean: Boolean is a value that can be either true or false.
- Date: Date is a date and time.
- Time: Time is a time.