What is Smart Contract ABI?
A smart contract ABI, or Application Binary Interface, is a standard way to interact with smart contracts in the Ethereum ecosystem. It defines the functions and structures that are available in a smart contract, and how to encode and decode data to call those functions.
ABIs are used by both external applications and other smart contracts to interact with each other. For example, a user interface for a decentralized exchange would use the ABI of the exchange contract to call the functions that allow users to trade tokens.
To simplify things,
- Smart Contract ABI converts binary code into human-readable code.
- Smart Contract ABI helps smart contracts interact with external applications.
- Smart Contract ABI is like a pathway that helps developers understand smart contract code.
- Smart Contract ABI is important for blockchain development.
ABIs are represented in JSON format, and they are typically generated by the compiler when a smart contract is deployed.
Here is an example of a simple ABI for a smart contract with a single function:
[
{
"type": "function",
"name": "transfer",
"inputs": [
{
"type": "address",
"name": "_to",
"internalType": "address"
},
{
"type": "uint256",
"name": "_amount",
"internalType": "uint256"
}
],
"outputs": [],
"stateMutability": "nonpayable"
}
]
This ABI defines a single function called transfer()
, which takes two arguments: the address of the recipient and the amount of tokens to transfer. The function has no outputs, and it is non-payable, meaning that it does not require any ETH to be sent with the transaction.
To call the transfer()
function using this ABI, we would need to encode the arguments in a specific format. For example, to transfer 10 tokens to the address 0x1234567890abcdef1234567890abcdef12345678
, we would encode the following data:
0xa9059cbb0000000000000000000000000000000000000000000000001234567890abcdef1234567890abcdef12345678
000000000000000000000000000000000000000000000000000000000000000a
This encoded data can then be sent to the smart contract using a transaction.
ABIs are an essential part of the Ethereum ecosystem, and they allow developers to build powerful and complex smart contracts that can interact with each other and with external applications.
What are the Building Blocks of ABI?
If you’re new to smart contract development, you might be wondering what an ABI file is. Essentially, it’s a file that acts as a communication interface between your smart contract and external applications. The file consists of two main components: function definitions and event definitions.
So basically,
- Function definitions specify the name, inputs, outputs, and payment acceptance of smart contract functions.
- Event definitions specify the name, data, and interpretation of events that can be triggered by a smart contract.
Properly formatted function calls and event subscriptions are necessary for smart contract interoperability with external applications.
In smart contract development or building a dApp, it’s really important to understand ABI. It helps you ensure seamless communication and data transfer between smart contracts and external apps. By mastering it, you can properly format function calls and event subscriptions, resulting in interoperable smart contracts. So go ahead and add this essential skill to your development toolkit – you’ll be glad you did!
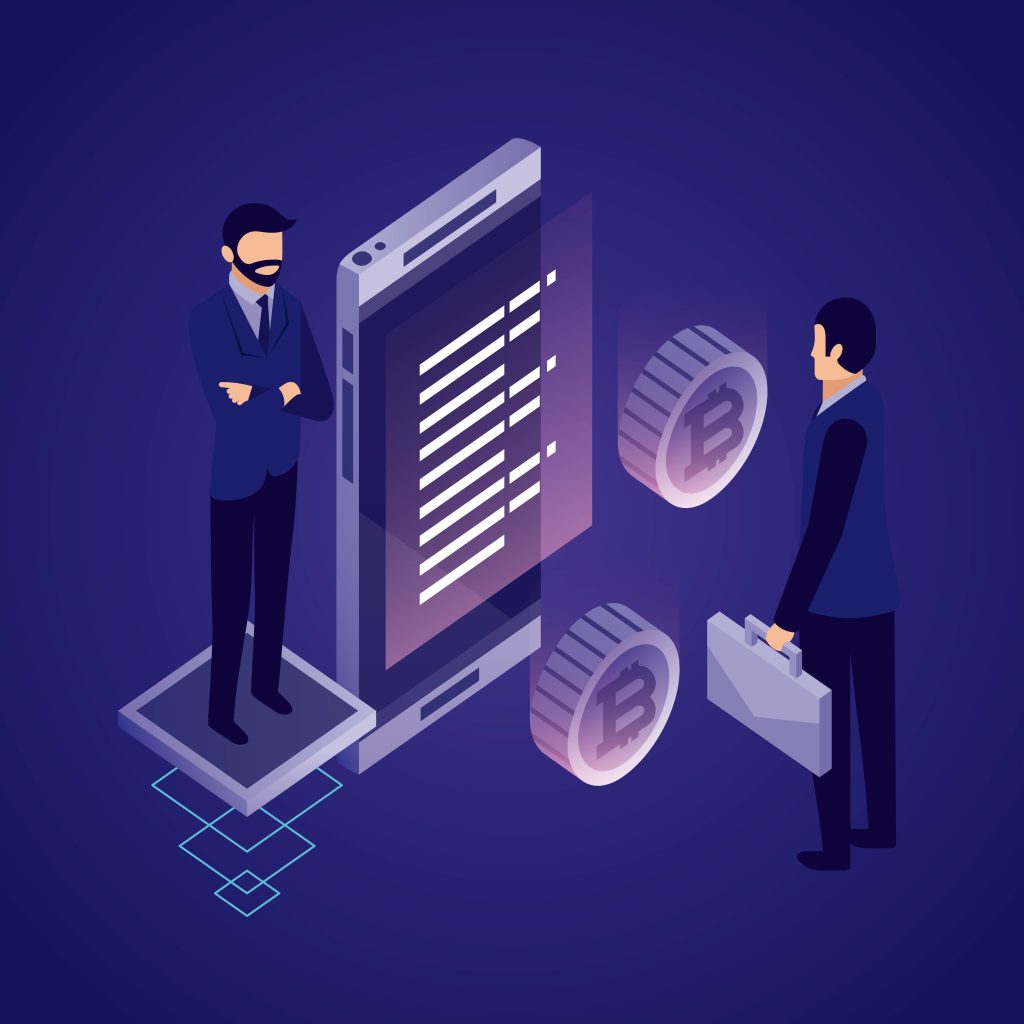
What do Encoding and Decoding in ABI mean?
If you’re interested in smart contract development, you’ll need to know about encoding and decoding. These two processes help smart contracts communicate and transfer data with the blockchain and external apps.
– Encoding is the process of converting data into a binary format, making it easy to store and transmit on the blockchain. Smart contract functions, events, and data require encoding in order to interact with the blockchain.
– Decoding, on the other hand, is the process of converting binary data back into a human-readable format, making it easy for smart contracts to read and interpret data from external applications.
If you’re working with smart contracts, it’s important to use the right encoding and decoding protocols. These processes ensure that data is transferred and stored correctly on the blockchain. To do this, you’ll want to use a standard encoding protocol, keep encoded data small to minimize gas costs, and thoroughly test encoding and decoding processes. With a solid understanding of encoding and decoding principles, you’ll be all set for successful smart contract development and blockchain interoperability!
What Are the Best Practices and Tools for ABI Interaction with Smart Contracts?
It is an essential part of smart contracts, as it enables external apps to call functions and receive events. Developers must encode the function’s name and parameters and send them as transactions to the smart contract’s address. The smart contract then executes the function and returns a result decoded using the same ABI rules.
To keep smart contracts efficient one must,
- Make it secure, and interoperable,
- Use a standard ABI format,
- Validate input parameters,
- Minimize gas costs.
Several tools and libraries are also available to help developers work with ABI and ensure successful smart contract development. These include the,
- Remix ID – Remix IDE simplifies smart contract development and testing, including ABI support.
- Truffle – Truffle is a development environment for building and deploying smart contracts
- Embark – Embark is a platform with integrated testing, deployment, and development for decentralized apps
- Web3.js & Ethers.js – Web3.js and Ethers.js are JavaScript libraries that provide APIs for working with smart contracts
By using these tools and libraries, developers can save time and effort while working with ABI, and ensure successful smart contract development.
How to Generate and Use ABI?
Certainly! Here are code snippets for generating, executing, and encoding a smart contract ABI:
- Generating Smart Contract ABI:
pragma solidity ^0.8.0;
contract MyContract {
function myFunction(uint256 param) public pure returns (uint256) {
// Function logic
return param * 2;
}
}
const solc = require('solc');
const contractSourceCode = `
pragma solidity ^0.8.0;
contract MyContract {
function myFunction(uint256 param) public pure returns (uint256) {
// Function logic
return param * 2;
}
}
`;
const input = {
language: 'Solidity',
sources: {
contractName: {
content: contractSourceCode,
},
},
settings: {
outputSelection: {
'*': {
'*': ['abi'],
},
},
},
};
const output = JSON.parse(solc.compile(JSON.stringify(input)));
const abi = output.contracts.contractName.MyContract.abi;
- The Solidity contract code defines a contract named
MyContract
with a function namedmyFunction
that takes auint256
parameter and returns auint256
value. The function simply doubles the input parameter. - The
solc
library is imported to compile the Solidity contract code and generate the ABI. - The
contractSourceCode
contains the Solidity contract code as a string. - The
input
object is defined with the Solidity code and compilation settings. - The
solc.compile()
function compiles the Solidity code and produces the output object. - The ABI is extracted from the output object and stored in the
abi
variable.
- Executing a Smart Contract using ABI:
const Web3 = require('web3');
const web3 = new Web3(provider);
const contractAddress = '0x123...'; // Address of the deployed contract
const contractInstance = new web3.eth.Contract(abi, contractAddress);
// Calling a contract function
const result = await contractInstance.methods.myFunction(123).call({ from: accounts[0] });
console.log(result);
- The
Web3
library is imported to interact with the Ethereum blockchain. - An instance of
Web3
is created with the appropriate provider, such as an HTTP provider or a WebSocket provider. contractAddress
is the address of the deployed smart contract where the contract instance will be created.- An instance of the smart contract is created using the
web3.eth.Contract(abi, contractAddress)
constructor, whereabi
is the previously generated ABI andcontractAddress
is the deployed contract’s address. contractInstance.methods.myFunction(123).call()
calls themyFunction
function of the smart contract on the blockchain, passing123
as the input parameter.- The
result
variable stores the returned value from the function call, which in this case would be the doubled value of123
. - The
console.log(result)
statement outputs the result to the console.
- Encoding Data with ABI:
const Web3 = require('web3');
const web3 = new Web3(provider);
const contractAddress = '0x123...'; // Address of the deployed contract
const contractInstance = new web3.eth.Contract(abi, contractAddress);
// Encoding function call with ABI
const encodedData = contractInstance.methods.myFunction(123).encodeABI();
console.log(encodedData);
- The
Web3
library is imported to interact with the Ethereum blockchain. - An instance of
Web3
is created with the appropriate provider, such as an HTTP provider or a WebSocket provider. contractAddress
is the address of the deployed smart contract where the contract instance will be created.- An instance of the smart contract is created using the
web3.eth.Contract(abi, contractAddress)
constructor, whereabi
is the previously generated ABI andcontractAddress
is the deployed contract’s address. contractInstance.methods.myFunction(123).encodeABI()
encodes the function call tomyFunction
with the input parameter123
using the contract’s ABI.- The encoded data is stored in the
encodedData
variable. - The
console.log(encodedData)
statement outputs the encoded data to the console
These code snippets provide an overview of generating a smart contract ABI, executing contract functions using ABI, and encoding data for contract interactions. Remember to replace the placeholders (contractAddress
, provider
, accounts[0]
) with the appropriate values in your specific scenario.
What does the future hold for ABI?
There’s no one-size-fits-all approach to working with ABI in the blockchain world – after all, every developer and every audience is unique.
However, what I can promise you is this: ABI is becoming increasingly important for ensuring smart contracts work seamlessly with the blockchain and external apps.
As the blockchain industry continues to evolve and grow, staying informed about the latest developments and best practices related to ABI is crucial for developers and businesses aiming to stay competitive.
So, if you’re starting in blockchain development, take some time to learn about it and keep up with its future growth and development. By staying focused on your business objectives and keeping up with best practices for working with contract ABI, you can gain a competitive advantage in the blockchain industry.
Remember to keep this strategic mindset in mind when working with contract ABI to save yourself time, effort, and plenty of heartaches.
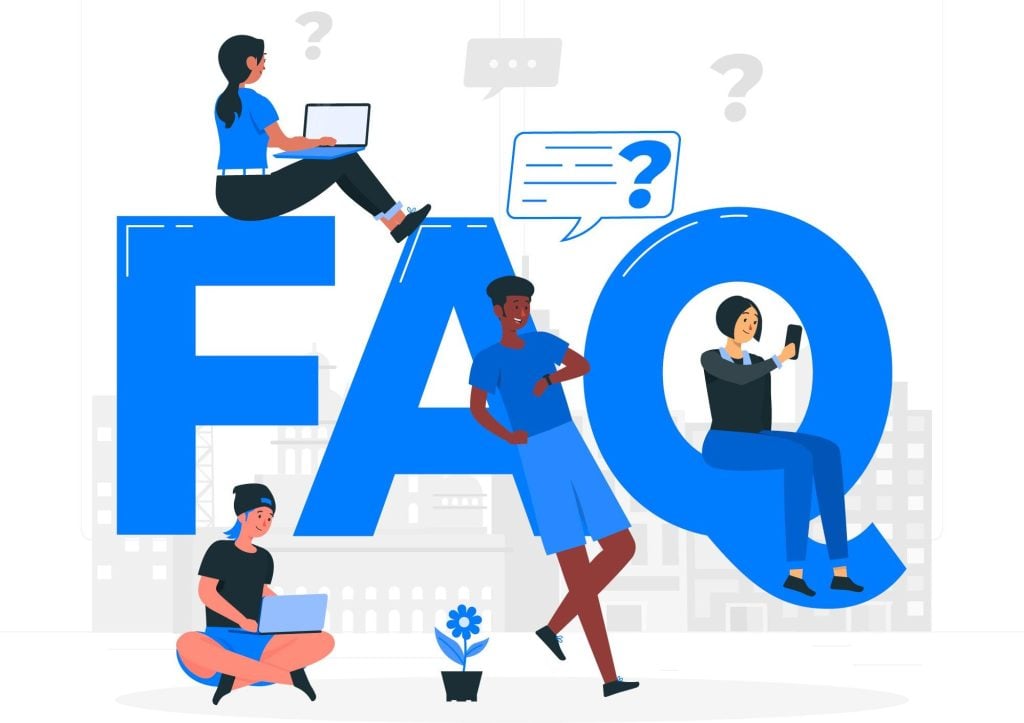
- What is the ABI in smart contract?
ABI stands for Application Binary Interface. It is a JSON (JavaScript Object Notation) file that defines the interface of a smart contract. This includes the names and types of the functions and events that are exposed by the contract.
- Where can I find the ABI of a smart contract?
The ABI of a smart contract can be found in the contract’s source code. It can also be found on the contract’s deployment address on a block explorer, such as Etherscan.
- How do you generate ABI for a contract?
There are a few ways to generate ABI for a contract. One way is to use the abi.encode function in Solidity. Another way is to use a tool like Remix or Truffle.
- What does ABI contain?
The ABI of a smart contract contains the following information:
- The names of the functions and events that are exposed by the contract.
- The types of the parameters and return values for each function and event.
- The signature of each function and event.
- Why is ABI used?
ABI is used to interact with smart contracts. It allows you to call functions on the contract and listen for events that are emitted by the contract.
- How does ABI work?
ABI is used to encode the data that is passed between a smart contract and an external application. The ABI defines the format of the data, so that the application knows how to interpret it.
- What is ABI in Dapps?
ABI is essential for Dapps (decentralized applications). Dapps are applications that run on the blockchain, and they need to be able to interact with smart contracts. ABI provides the interface that allows Dapps to interact with smart contracts.
- What is the ABI format?
The ABI format is a JSON (JavaScript Object Notation) file. It is a standard format that is used to define the interface of a smart contract.
- What is the use of ABI in blockchain?
ABI is used to interact with smart contracts on the blockchain. It allows you to call functions on the contract and listen for events that are emitted by the contract.
- Can I get ABI from Etherscan?
Yes, you can get ABI from Etherscan. To do this, go to the contract’s page on Etherscan and click on the “ABI” tab. This will show you the ABI of the contract in JSON format.