How Error Handling is Handled in Solidity Code?
Solidity, an object-oriented programming language used for implementing smart contracts on Ethereum and other blockchains, provides several functions to address potential errors during compile time and runtime. While syntax errors can be caught at compile time, runtime errors can be challenging to detect and often occur during contract execution. Examples of runtime errors include divide-by-zero, array-out-of-index, and so forth.
Solidity uses state-reverting exceptions to handle errors, effectively ensuring atomicity. When a smart contract call terminates with an error, all state changes made to variables, balances, and other aspects are reverted, all the way up the chain of contract calls. This error handling mechanism enables developers to interact directly with other contracts by declaring an interface.
It’s essential to note that Ethereum transactions are atomic, meaning they are either fully completed or have no effect on the state and are entirely reversed. Overall, Solidity provides a robust error handling mechanism that enables smart contract developers to write code that is reliable, efficient, and error-free.
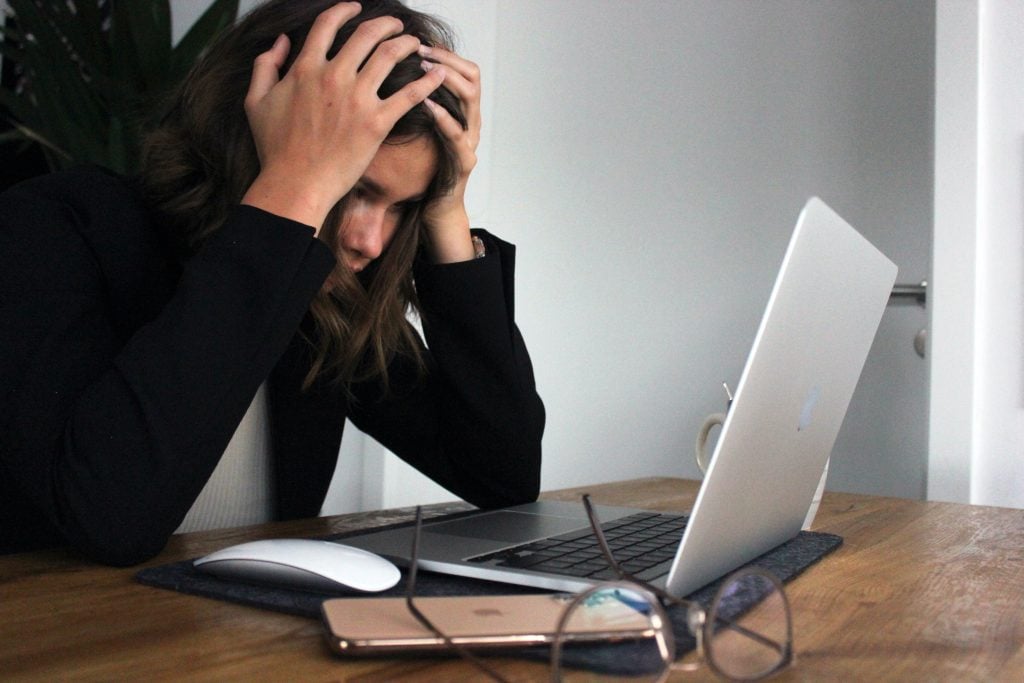
The 3 Main Solidity Error Handling Functions
Earlier versions of Solidity utilized a single throw statement for error handling, but this method proved suboptimal for gas efficiency and required additional test functions to check values and throw errors. To address this issue, Solidity version 4.10 introduced three special functions – assert, require, and revert – as new error handling constructs. With the throw statement made absolute, developers can now more effectively manage errors and optimize gas usage in their code.
The Require Function in Solidity
The require function plays a pivotal role in developing secure and reliable Solidity contracts. By employing the require function effectively, you can validate inputs, enforce conditions, and enhance the overall integrity of your contract’s logic. This article will provide valuable insights on how to utilize the require function in your Solidity contracts to ensure robustness and mitigate potential vulnerabilities
Here’s an example:
function transfer(address recipient, uint amount) public {
require(amount > 0, "Amount must be greater than 0");
balances[msg.sender] -= amount;
balances[recipient] += amount;}
In this example, the transfer function ensures that the amount being transferred is greater than zero before executing the rest of the function code. If the amount is not greater than zero, the function throws an exception and reverts the transaction.
The Assert Function in Solidity
The assert function is used to check for internal errors in the contract code. It takes a boolean expression as its argument and throws an exception and reverts the transaction if the expression evaluates to false. However, unlike the require function, the assert function should only be used to check for internal errors in the contract code that should never occur. Here’s an example:
function withdraw(uint amount) public {
assert(balances[msg.sender] >= amount);
balances[msg.sender] -= amount;
payable(msg.sender).transfer(amount);
}
In this example, the withdraw function checks if the user’s balance is greater than or equal to the withdrawal amount. If it’s not, the function throws an exception and reverts the transaction. However, since this should never occur if the contract code is working correctly, the assert function is used instead of the require function.
The Revert Function in Solidity
The revert function is similar to the require function in that it’s used to revert a transaction if a condition is not met. However, the revert function provides more flexibility in error handling and allows you to provide a reason string to explain why the transaction was reverted. Here’s an example:
function withdraw(uint amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
if (!msg.sender.send(amount)) {
revert("Failed to send funds");
}
}
In this example, the buyTokens function checks if there are enough tokens available for purchase before executing the rest of the function code. If there are not enough tokens, the function reverts the transaction and provides a reason string to explain why it was reverted.
To solve the EVM execution reverted error check our recent article here.
Conclusion
To sum up these functions, the require function should be used to validate user input or contract state before executing the rest of the contract code. The assert function should be used to check for internal errors in the contract code that should never occur. The revert function should be used to revert a transaction if a condition is not met and provides more flexibility in error handling. By understanding when to use these functions in your Solidity contracts, you can write safer and more secure smart contracts on the Ethereum blockchain
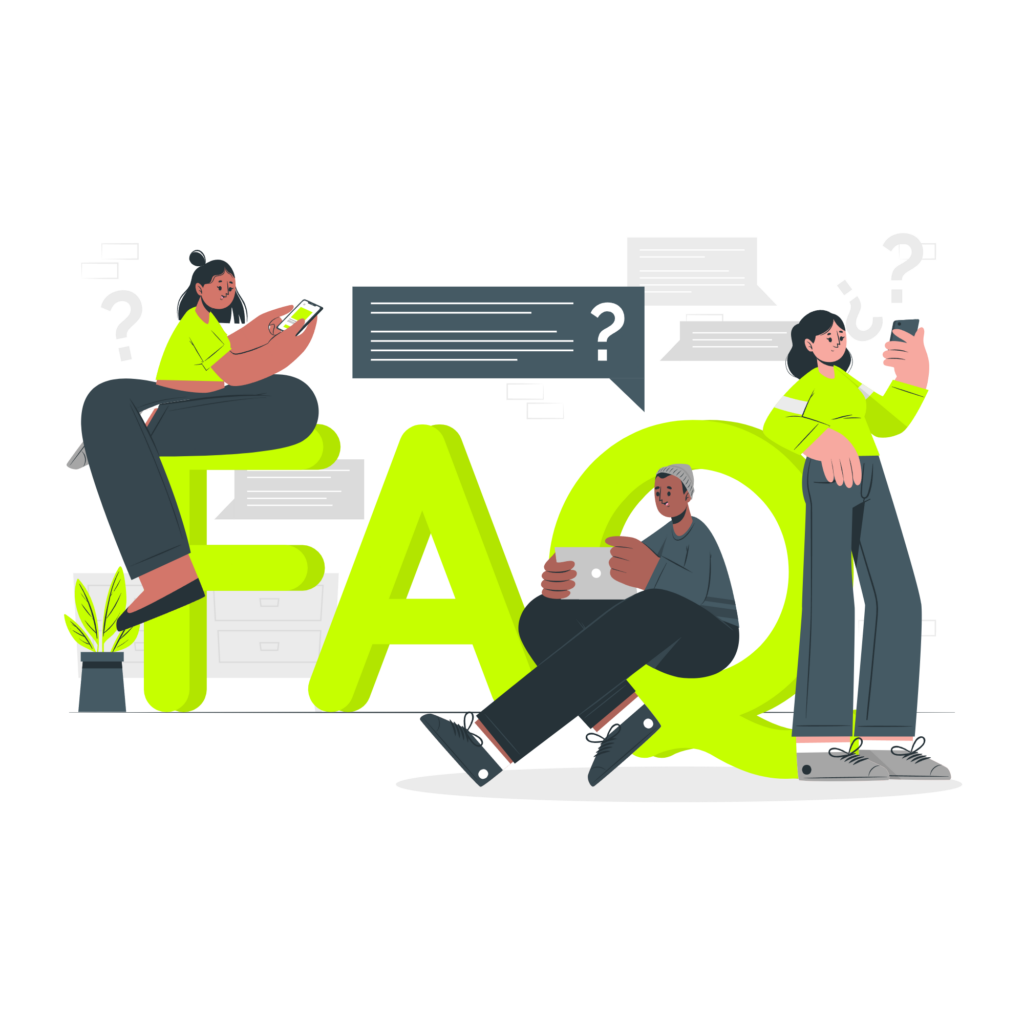
- What is the difference between require and assert and revert in Solidity?
The main difference is the behavior when the condition fails. require and revert revert the entire transaction and undo all changes, while assert is used for internal errors and will always revert the transaction.
- What are the error handling methods in Solidity?
Solidity provides three error handling methods: require, assert, and revert. These methods are used to validate conditions and handle exceptions in smart contracts.
- What is the difference between require and assert in Solidity?
The difference lies in the behavior when the condition fails. require and assert both check conditions, but require is used for input validation and will revert the transaction, while assert is used for internal errors and will always revert the transaction.
- What is the revert function in Solidity?
revert is a function in Solidity that is used to revert the entire transaction and undo all changes made so far. It is commonly used for error handling and to revert the state back to its original state.
- Why use require instead of assert?
Use require when you want to check for input validation and ensure that certain conditions are met before proceeding with the execution of the contract. If the condition fails, require will revert the transaction and undo all changes, providing a safer way to handle errors.
- Why do we use the require method?
The require method is used to validate conditions in smart contracts. It allows you to check for certain conditions and revert the transaction if the conditions are not met. This helps ensure that the contract functions correctly and prevents unwanted behavior.
- Which is better assert or verify?
In Solidity, assert is used for internal errors that should never occur, while require is used for input validation and to check conditions that should always be true. It is generally recommended to use require for most cases as it provides a safer way to handle errors and prevents unwanted behavior.
- When shouldn’t you use the assert statement?
The assert statement should not be used for input validation or checking conditions that might be false in certain cases. It is meant to be used for internal errors that should never occur. For input validation, it is recommended to use the require statement instead.
- Does assert raise an error?
Yes, when the condition provided to the assert statement evaluates to false, it will raise an error and revert the entire transaction.
- What happens if assert fails?
If an assert statement fails, there has likely been an internal programming issue. All modifications made during the course of the transaction are undone, and the contract’s execution is instantly suspended. This is done to safeguard the integrity of the contract’s state and to stop any additional unexpected activity.
11. What does require do in Solidity?
The require keyword is used in Solidity to ensure that certain conditions are met before proceeding with the execution of a function.
If the condition specified in the require statement is true, the function will continue to execute. However, if the condition is false, the function will stop executing and revert any changes made to the state of the contract. Reverting means that any state changes made during the execution of the function (such as changes to variables or storage) will be undone, and the contract will return to its previous state.
12. What is require vs assert in Solidity?
Require checks if inputs and conditions are valid, while assert checks if the code itself is behaving as expected, and throws an error if something unexpected or out of place happens.
13. Why use require instead of assert?
It’s important to use require when validating user input or external dependencies (such as whether a user’s balance is sufficient), and assert to validate internal conditions that should never fail. By using both of these keywords where appropriate, Solidity developers can create more robust and secure contracts that are less prone to error.