TL;DR
A Decentralized Exchange (DEX) allows peer-to-peer trading without intermediaries. To build a basic DEX using Solidity, key components include:
- Liquidity Pools: Users contribute tokens, enabling trades.
- AMM Formula: Ensures price balance in the pool (e.g., “x * y = k”).
- ERC20 Integration: Supports various tokens for seamless interaction.
Steps to build:
- Add Liquidity: Users deposit tokens and receive LP tokens.
- Swap Functionality: Facilitates token swaps using AMM formulas.
- Fee Mechanism: Deducts and distributes fees to liquidity providers.
Enhancements include LP token functionality, oracle integration, and governance for community control. Security is vital, with protection against reentrancy attacks and flash loans. Test and deploy using Truffle or Hardhat on Ethereum or a testnet like Rinkeby.
Decentralized finance (DeFi) is transforming the financial world, offering alternatives to traditional systems that are more transparent and don’t rely on middlemen. Central to DeFi are Decentralized Exchanges (DEXes), which allow direct peer-to-peer trading of digital tokens. Let’s explore how you can build your own DEX using Solidity, a popular programming language for creating smart contracts on the Ethereum blockchain. This guide will focus on creating a decentralized exchange, enabling you to actively participate in this innovative financial landscape.
Understanding DEX Basics
A DEX operates without a central authority through mechanisms known as Automated Market Makers (AMMs). Here’s a simplified look at the core concepts:
- Liquidity Pools: These are pools of tokens that users contribute to, enabling trading. The pool handles trades directly, replacing traditional buyer-seller matching.
- AMM Formulas: To determine trade prices, formulas like “x*y=k” ensure the product of the quantities remains constant, balancing prices as trades occur.
- Liquidity Providers: Contributors to the pool receive LP tokens as a receipt, which also entitle them to a share of the trading fees.
Building Your DEX: Key Components
Here’s what you need to build a basic DEX:
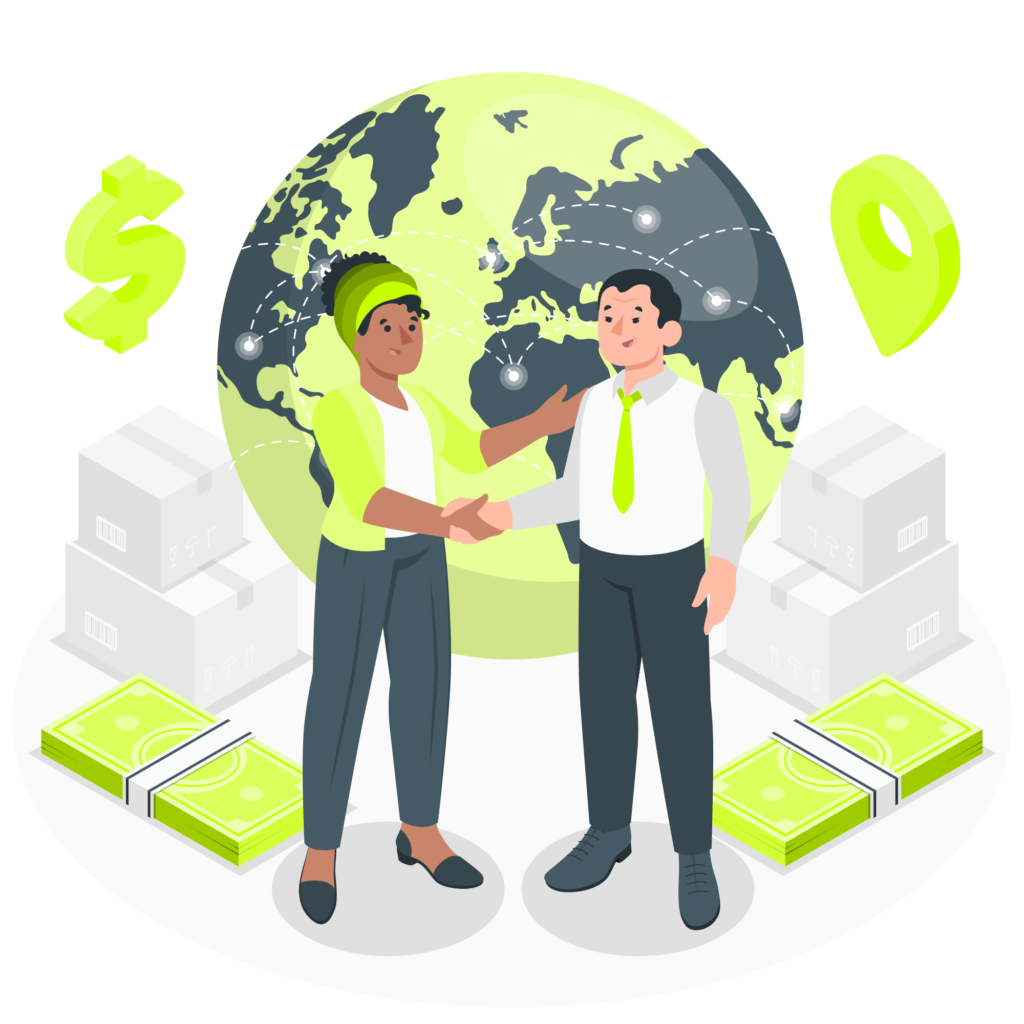
- ERC20 Integration: The ERC20 standard defines the functionalities for tokens on the Ethereum blockchain. By integrating ERC20, your DEX can interact with various tokens seamlessly.
- Liquidity Pool Management:
- Adding Liquidity: Users should be able to deposit tokens into the pool, receiving LP tokens in return.
- Removing Liquidity: LPs should be able to withdraw their share of the pool, burning their corresponding LP tokens.
- Swapping Functionality: The core of your DEX, this function facilitates token swaps between users based on the AMM formula.
- Fee Mechanism: Implement a fee structure that promotes liquidity provision and covers operational costs. Fees can be deducted from each swap and distributed proportionally to LPs.
Sample Solidity Code for a Basic DEX
Here’s a basic outline in Solidity:
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
contract MyDEX {
address public token1;
address public token2;
uint public reserve1;
uint public reserve2;
constructor(address _token1, address _token2) {
token1 = _token1;
token2 = _token2;
}
function addLiquidity(uint amount1, uint amount2) public {
IERC20(token1).transferFrom(msg.sender, address(this), amount1);
IERC20(token2).transferFrom(msg.sender, address(this), amount2);
// Logic to update reserves and mint LP tokens
}
function swap(address tokenIn, uint amountIn, address tokenOut, uint amountOutMin) public {
// Logic to handle swaps and charge fees
}
function getAmountOut(uint amountIn, uint reserveIn, uint reserveOut) public pure returns (uint) {
uint product = reserveIn * reserveOut;
return product / (reserveIn + amountIn); // AMM formula
}
}
Enhancing Your DEX
- Fee Implementation:
- Integrate a fee variable to define the percentage deducted from each swap.
- Modify the swap function to deduct the fee from the amountOut before transferring tokens.
- Distribute fees proportionally to LPs by accumulating them in a separate variable and updating LP token balances periodically.
- LP Token Functionality:
- Create an ERC20 compliant LP token contract to represent user contributions to the pool.
- Implement functions in the main DEX contract to mint LP tokens when users add liquidity and burn them when users withdraw liquidity.
- Ensure the total supply of LP tokens reflects the total value locked (TVL) in the pool.
- Advanced Features:
- Oracle Integration: Integrate an oracle service to provide reliable external price feeds for tokens, especially for non-ERC20 tokens. This can enhance the accuracy of AMM calculations.
- Order Matching: While AMMs offer a simpler approach, consider implementing on-chain order books for users who prefer setting specific buy or sell orders. This can cater to users seeking more control over their trades.
- Governance: Introduce a governance mechanism where LP token holders can vote on critical decisions, such as fee structure adjustments or new token listings. This fosters a community-driven approach to DEX development.
Security Considerations
- Reentrancy Attacks: Mitigate reentrancy attacks by employing techniques like checks-effects-interaction (CEI) patterns or utilizing libraries like OpenZeppelin’s ReentrancyGuard. These ensure transactions complete atomically, preventing attackers from manipulating your code during a swap.
- Flash Loan Attacks: Consider implementing flash loan protection mechanisms to prevent attackers from manipulating liquidity pools momentarily for their own gain.
- Smart Contract Audits: Thorough security audits by reputable firms are crucial before deploying your DEX on a live blockchain. These audits identify potential vulnerabilities and ensure the robustness of your code.
Deployment and Testing
- Local Testing: Utilize tools like Truffle or Hardhat to develop and test your DEX contract locally on a simulated blockchain environment. This allows you to identify and rectify bugs before deployment.
- Mainnet Deployment: Once thoroughly tested, deploy your DEX contract to the Ethereum mainnet or a compatible testnet like Rinkeby.
Conclusion: Creating a Decentralized Exchange
Building a DEX is a complex yet rewarding venture. This article has given you the fundamental knowledge how to create a decentralized exchange. Remember, consistent research, accurate testing, and a focus on security are very important for a successful DEX development journey. As you search deeper, apply the vast resources available online and within the blockchain developer community. By combining creativity and technical expertise, you can contribute to the ever-expanding field of Decentralized Finance.
FAQs
What is a decentralized exchange (DEX)?
- A DEX is a type of cryptocurrency exchange that allows for direct peer-to-peer cryptocurrency transactions to take place online securely and without the need for an intermediary.
How does Solidity function in creating a DEX?
- Solidity is used to write smart contracts that run on the Ethereum blockchain, crucial for handling the logic and transactions of a DEX.
What are the first steps to develop a DEX using Solidity?
- Start by learning Solidity basics, set up your development environment, and understand the Ethereum blockchain and its capabilities.
What are smart contracts?
- Smart contracts are self-executing contracts with the terms of the agreement directly written into lines of code, which run on the blockchain.
How important is security in building a DEX?
- Security is paramount in building a DEX, as vulnerabilities can lead to significant financial losses. Thorough testing and audits are essential.
What is blockchain technology?
- Blockchain is a distributed ledger technology that records transactions across multiple computers so that the record cannot be altered retroactively.
How does cryptocurrency work?
- Cryptocurrencies are digital currencies that use cryptography for security and operate independently of a central bank, typically using blockchain technology.
What is Ethereum?
- Ethereum is a decentralized, open-source blockchain system that features smart contract functionality.
What are the benefits of using a DEX over a centralized exchange?
- DEXs enhance security, reduce the risk of price manipulation, and offer users full control over their funds.
What skills are required to develop a DEX with Solidity?
- Solid proficiency in Solidity, understanding of Ethereum and blockchain, knowledge of cryptocurrency mechanics, and a strong grasp of security practices.