The world of blockchain technology has seen a increase in the development and use of tokens. These digital assets represent ownership, utility, or access within a blockchain ecosystem. Solidity, a high-level programming language specifically designed for writing smart contracts on the Ethereum Virtual Machine (EVM), plays a crucial role in creating these tokens. This article delves into the process of crafting your own token using Solidity, guiding you through the essential steps and considerations.
Understanding Tokens and the ERC-20 Standard
Before diving into Solidity code, it’s vital to grasp the concept of tokens and the standard used for creating them on Ethereum.
- Tokens: Tokens are units of value residing on a blockchain. They can represent various things, including fungible (interchangeable) currencies, unique digital collectibles, or access rights to specific services within a decentralized application (dApp).
- ERC-20 Standard: The ERC-20 standard is a set of guidelines established for creating tokens on the Ethereum blockchain. It defines six core functions that a token contract must implement to ensure compatibility with Ethereum wallets, exchanges, and other dApps. These functions include:
- totalSupply: Retrieves the total number of tokens ever created.
- balanceOf(address owner): Returns the balance of a specific token holder.
- transfer(address recipient, uint256 amount): Transfers tokens from the sender’s account to the recipient’s address.
- allowance(address owner, address spender): Checks the amount of tokens an owner has approved for a spender to transfer on their behalf.
- transferFrom(address sender, address recipient, uint256 amount): Allows a spender to transfer tokens from another account’s balance (as long as the allowance is sufficient).
- approve(address spender, uint256 amount): Grants permission for a spender to transfer a specified amount of tokens on the owner’s behalf.
By adhering to the ERC-20 standard, it can easily work with many different tools and services on the Ethereum network, like a piece of fitting perfectly into a puzzle making it more useful and convenient.
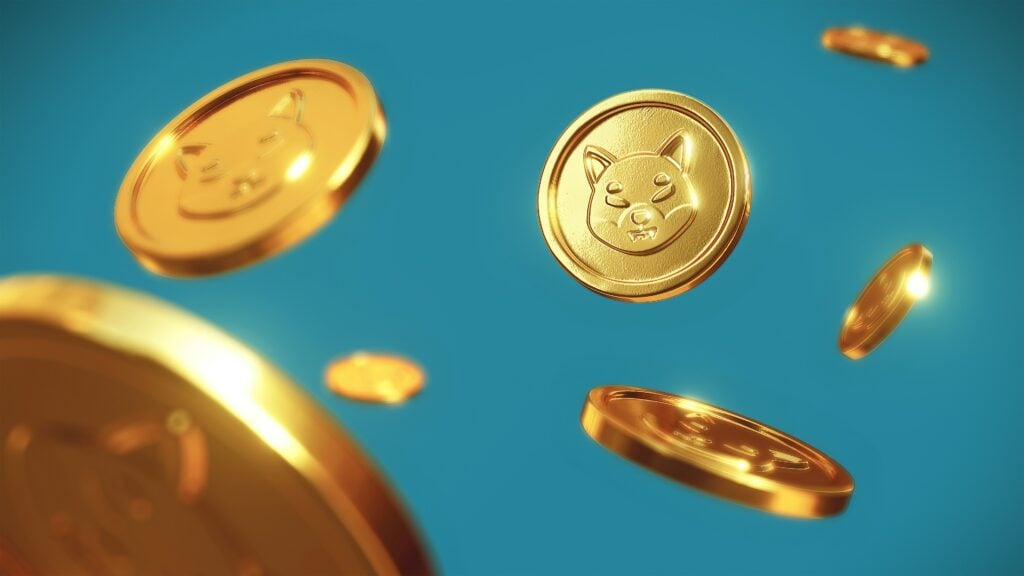
Setting Up Your Development Environment
To get started on your token creation journey, you’ll need a development environment equipped with the necessary tools:
- Solidity Compiler: This tool translates your human-readable Solidity code into bytecode, a format understandable by the EVM. You can install the command-line compiler using npm or include an online compiler like Remix.
- Solidity IDE (Optional): While you can write Solidity code in any text editor, an Integrated Development Environment (IDE) specifically designed for Solidity offers features like syntax highlighting, code completion, and debugging capabilities. Popular options include Remix (online), Visual Studio Code with Solidity extensions, or dedicated blockchain IDEs like Truffle or OpenZeppelin Defender.
- Blockchain Node (Optional): For local testing and deployment, you might consider setting up a local Ethereum node using tools like Geth or running a node on a test network like Rinkeby or Kovan.
Crafting Your ERC-20 Token Contract
Here comes the exciting part: writing the Solidity code for your token contract. Here’s a breakdown of the key components:
- Solidity Pragma: The first line typically specifies the Solidity version your code is compatible with. This ensures proper compilation and functionality. For instance:
pragma solidity ^0.8.0;
- Imports: You can import existing libraries or contracts to leverage functionality. Importing the OpenZeppelin ERC20 library simplifies the process by providing pre-built implementations of the ERC-20 standard functions.
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
- Contract Definition: Define your token contract using the contract keyword, followed by the chosen name for your token (e.g., MyToken). Inherit from the ERC20 contract to automatically implement the standard functions.
contract MyToken is ERC20 {
// ... rest of your contract code
}
- Token Properties:
- Name: Set the human-readable name of your token using the name variable.
- Symbol: Define a short abbreviation for your token using the symbol variable. This will be used for display purposes on exchanges and wallets.
- Decimals: Specify the number of decimal places your token represents. Most tokens use 18 decimals for divisibility.
constructor() ERC20("MyToken", "MTK") {
_mint(msg.sender, 1000000 * 10**uint256(decimals())); // Mint}
Let us explore additional functionalities you can incorporate into your token contract:
- Total Supply: Define the total number of tokens that will ever exist using a state variable of type uint256. You can either mint the entire supply during deployment or distribute it gradually.
uint256 public constant TOTAL_SUPPLY = 1000000 * 10**uint256(decimals()); - Minting: Implement a function with access control (e.g., only the contract owner) to create new tokens and add them to the total supply. Utilize the _mint function inherited from the ERC20 library.
function mint(address recipient, uint256 amount) public onlyOwner {
_mint(recipient, amount);
}
modifier onlyOwner() {
require(msg.sender == owner, "Only owner can mint tokens");
_;
}
- Burning: If desired, you can introduce a function to permanently remove tokens from circulation, reducing the total supply. Employ the _burn function from the ERC20 library.
function burn(uint256 amount) public {
_burn(msg.sender, amount);
}
- Ownership Transfer: Include functionality to transfer ownership of the contract to another address. This might be useful for future upgrades or maintenance.
address public owner;
constructor() ERC20("MyToken", "MTK") {
owner = msg.sender;
}
function transferOwnership(address newOwner) public onlyOwner {
owner = newOwner;
}
- Events: Utilize events to log information about token transfers and approvals on the blockchain. This allows external applications and users to track token activity.
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed owner, address indexed spender, uint256 value);
Example ERC-20 Contract
An ERC-20 contract with all the above mentioned functionalities would look like this,
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
// Token Properties
string public constant name = "MyToken";
string public constant symbol = "MTK";
uint8 public constant decimals = 18;
// Total Supply Definition
uint256 public constant TOTAL_SUPPLY = 1000000 * 10**uint256(decimals());
// Contract Owner
address public owner;
// Events
event Mint(address indexed to, uint256 amount);
event Burn(address indexed from, uint256 amount);
constructor() ERC20(name, symbol) {
owner = msg.sender;
_mint(msg.sender, TOTAL_SUPPLY); // Mint total supply to deployer
}
// Minting Function with Access Control (only owner)
function mint(address recipient, uint256 amount) public onlyOwner {
require(totalSupply() + amount <= TOTAL_SUPPLY, "Minting exceeds total supply");
_mint(recipient, amount);
emit Mint(recipient, amount);
}
// Burning Function
function burn(uint256 amount) public {
_burn(msg.sender, amount);
emit Burn(msg.sender, amount);
}
// Ownership Transfer Function
function transferOwnership(address newOwner) public onlyOwner {
owner = newOwner;
}
// Modifier to restrict functions to contract owner
modifier onlyOwner() {
require(msg.sender == owner, "Only owner can perform this action");
_;
}
}
Important Considerations:
- Security: Smart contract security is paramount. Always thoroughly test and audit your code before deployment to prevent vulnerabilities that could lead to loss of funds. Consider using established libraries like OpenZeppelin for secure implementations.
- Gas Fees: Executing transactions on the Ethereum blockchain incurs gas fees. Optimize your code for efficiency to minimize gas costs for users.
- Regulations: Depending on your token’s purpose and functionality, regulations might apply. Research relevant regulations in your jurisdiction to ensure compliance.
Testing and Deployment
Once you’ve written your token contract, it’s crucial to test it rigorously before deploying it on the Ethereum mainnet. Here’s how:
- Local Testing: Use a local Ethereum node or a test network like Rinkeby or Kovan to deploy your contract and simulate transactions. Tools like Truffle or Ganache can facilitate local testing.
- Unit Testing: Write unit tests to verify individual functions of your contract in isolation. This helps isolate potential bugs in specific areas of the code. Frameworks like Solidity Test Framework (STF) can aid in unit testing.
- Auditing: For critical projects, consider a professional security audit by a reputable blockchain security firm. This adds an extra layer of assurance and minimizes the risk of vulnerabilities.
Deployment:
After successful testing, you can deploy your contract to the Ethereum mainnet. The process involves interacting with your chosen blockchain node and paying the required transaction fees. Tools like Remix, Truffle, or dedicated blockchain IDEs offer deployment functionalities.
Additional Considerations:
- Wallet Integration: Ensure your token is compatible with popular Ethereum wallets and exchanges for wider adoption.
- Liquidity Provision: If you plan to enable trading of your token on decentralized exchanges (DEXs), you might need to provide initial liquidity for trading pairs.
Conclusion
Creating a token with Solidity empowers you to contribute to the ever-evolving world of blockchain technology. By following this guide and adhering to best practices, you can develop your own ERC-20 token and leverage its functionalities within the Ethereum ecosystem. Remember, continuous learning, security awareness, and thorough testing are key to a successful token creation journey.
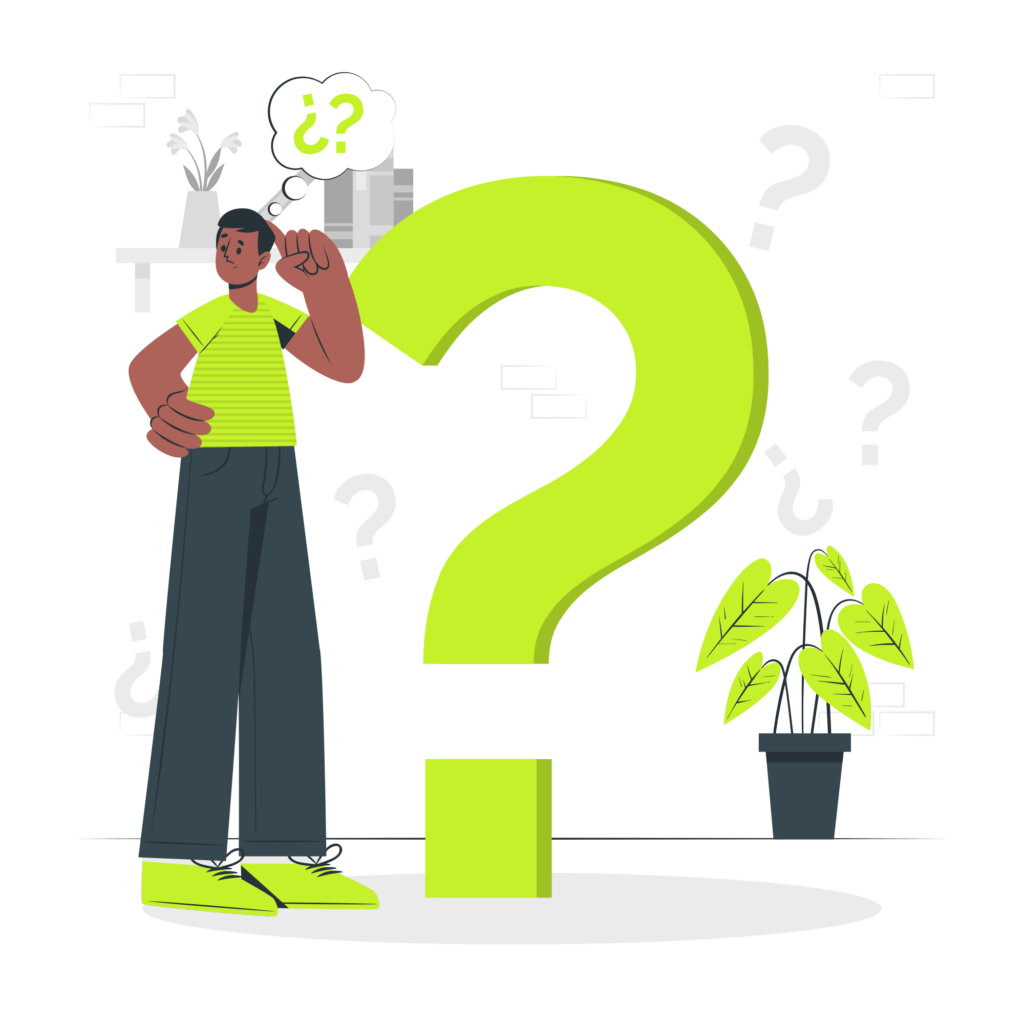
FAQs
What is Solidity?
- Solidity is a programming language designed for developing smart contracts that run on the Ethereum blockchain.
How do you create a token in Solidity?
- Creating a token in Solidity involves writing a smart contract that defines the token’s properties and behaviors, then deploying it to the Ethereum network.
What are the key components of a Solidity token contract?
- Key components include the contract declaration, state variables for tracking balances, and functions for transferring tokens and managing supply.
Can I create different types of tokens using Solidity?
- Yes, you can create various types of tokens, including fungible tokens (ERC-20), non-fungible tokens (ERC-721), and others.
What are the best practices for deploying a Solidity token?
- Best practices include thorough testing, code audits, optimizing gas usage, and ensuring contract security to prevent vulnerabilities.
What is Ethereum?
- Ethereum is a decentralized platform that enables developers to build and deploy smart contracts and decentralized applications (dApps).
What are smart contracts?
- Smart contracts are self-executing contracts with the terms of the agreement directly written into code, running on the blockchain.
How does blockchain technology work?
- Blockchain technology is a decentralized digital ledger that records all transactions across a network of computers securely and transparently.
What is the purpose of cryptocurrency tokens?
- Cryptocurrency tokens can represent various assets or utilities on a blockchain and are often used as part of dApps or as digital currency.
How can I learn Solidity programming?
- Learning Solidity can be approached through online tutorials, coding bootcamps, and comprehensive reading of the official Solidity documentation.