JavaScript arrays plays a crucial role in data organization in the language. They provide a flexible and dynamic way to store collections of elements, making them essential for various web development tasks, from managing user input to manipulating complex datasets. This article includes all necessary information on arrays in JavaScript that will be helpful for you to understand this powerful tool correctly.
Understanding Arrays
At its core, a JavaScript array is an ordered collection of elements. These elements can be of various data types, including numbers, strings, booleans, objects, and even other arrays. This versatility allows you to represent diverse kinds of information within a single variable. Unlike some data structures in other languages, JavaScript arrays are zero-indexed, meaning the first element resides at index 0, the second at index 1, and so on.
There are two primary ways to create arrays in JavaScript:
- Array Literal: This is the most common and concise method. You enclose a comma-separated list of elements within square brackets ([]). For example:
const fruits = ["apple", "banana", "orange"];
const numbers = [1, 2, 3, 4];
- Array Constructor: While less frequently used, the Array constructor allows you to specify the initial length of the array or provide individual elements as arguments. Here’s an example:
const emptyArray = new Array(); // Creates an empty array
const vegetables = new Array(3); // Creates an array with a length of 3 (initially filled with undefined)
const colors = new Array("red", "green", "blue");
Accessing Elements
Extracting elements from an array is straightforward. You use the array variable name followed by the element’s index within square brackets. Remember, indexing starts at 0.
const fruits = ["apple", "banana", "orange"];
const firstFruit = fruits[0]; // firstFruit will hold "apple"
const lastFruit = fruits[fruits.length - 1]; // Accessing the last element using length property
Modifying Elements
JavaScript arrays are mutable, meaning you can change their content after creation. You can assign a new value to an existing element using its index.
fruits[1] = "mango"; // Replaces "banana" with "mango"
Adding and Removing Elements
Arrays provide built-in methods for adding and removing elements:
push()
: Appends elements to the end of the array.
fruits.push("grapefruit"); // Adds "grapefruit" to the end
pop()
: Removes and returns the last element from the array.
const removedFruit = fruits.pop(); // removedFruit will hold "grapefruit" (assuming no further modifications)
unshift()
: Inserts elements at the beginning of the array.
fruits.unshift("kiwi"); // Adds "kiwi" to the beginning
shift()
: Removes and returns the first element from the array.
const firstRemovedFruit = fruits.shift(); // firstRemovedFruit will hold "kiwi"
splice()
: This versatile method allows you to insert, remove, or replace elements at a specified index. It takes three arguments: starting index, number of elements to remove (optional, defaults to 0), and elements to insert (optional).
fruits.splice(1, 2, "watermelon", "pineapple"); // Removes "mango" and "orange", inserts "watermelon" and "pineapple" at index 1
Iteration Techniques to Traverse an Array
Iterating through an array’s elements is crucial for processing and manipulating its contents. JavaScript offers several methods for achieving this:
for loop
: This classic approach provides a clear way to access each element using its index.
const fruits = ["apple", "banana", "orange"];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]); // Prints each fruit
}
for...of loop
: Introduced in ES6 (ECMAScript 2015), this loop iterates directly over the values of the array, making the syntax more concise.
for (const fruit of fruits) {
console.log(fruit); // Prints each fruit
}
forEach()
method: This higher-order function executes a provided function once for each element in the array. It takes a callback function as an argument that receives the element, its index, and the entire array.
fruits.forEach((fruit, index, array) => {
console.log(fruit, index, array
); // Prints details of each element
});
map()
method: This powerful method creates a new array with the results of calling a provided function on every element in the original array. It’s useful for transforming data.
const numbers = [1, 2, 3, 4];
const doubledNumbers = numbers.map(number => number * 2); // doubledNumbers will contain [2, 4, 6, 8]
filter()
method: This method creates a new array containing only the elements that pass a test implemented by the provided function.
const fruits = ["apple", "banana", "orange", "grape"];
const citrusFruits = fruits.filter(fruit => fruit.includes("cit")); // citrusFruits will contain ["citrus", "grapefruit"] (assuming "grapefruit" is added)
reduce()
method: This method applies a function against an accumulator and each element in the array to reduce it to a single value.
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((accumulator, number) => accumulator + number, 0); // sum will hold 10 (initial value of 0 is added)
Searching and Finding Elements in an Array
Finding specific elements within an array is a common task. JavaScript provides methods to locate elements based on their values or indexes:
indexOf()
: This method returns the first index at which a given element can be found in the array, or -1 if it’s not present.
const fruits = ["apple", "banana", "orange"];
const indexOfOrange = fruits.indexOf("orange"); // indexOfOrange will hold 2
lastIndexOf()
: Similar to indexOf(), this method returns the last index where the element is found, useful when duplicates exist.
fruits.push("orange"); // Add another "orange"
const lastIndexOfOrange = fruits.lastIndexOf("orange"); // lastIndexOfOrange will hold 3
includes()
: This method determines if an array contains a specific element, returning true if found, and false otherwise.
const fruits = ["apple", "banana", "orange"];
const hasMango = fruits.includes("mango"); // hasMango will hold false
find()
: This method returns the first element in the array that satisfies a test implemented by the provided function.
const fruits = ["apple", "banana", "orange"];
const firstCitrusFruit = fruits.find(fruit => fruit.includes("cit")); // firstCitrusFruit will hold "citrus" (assuming "citrus" is added)
findIndex()
: Similar to find(), this method returns the index of the first element that passes the test, or -1 if not found.
const fruits = ["apple", "banana", "orange"];
const indexOfCitrusFruit = fruits.findIndex(fruit => fruit.includes("cit")); // indexOfCitrusFruit will hold 0 (assuming "citrus" is at index 0)
Advanced Array Operations
JavaScript arrays offer a rich set of built-in methods for advanced operations:
concat()
: This method merges two or more arrays into a new array.
const fruits = ["apple", "banana"];
const vegetables = ["carrot", "potato"];
const combinedArray = fruits.concat(vegetables); // combinedArray will contain ["apple", "banana", "carrot", "potato"]
slice()
: This method extracts a section of an array and returns a new array. It takes two arguments (optional): starting index (inclusive) and ending index (exclusive).
const fruits = ["apple", "banana", "orange", "mango"];
const citrusFruits = fruits.slice(1, 3); // citrusFruits will contain ["banana", "orange"]
join()
: This method combines all elements of an array into a single string, separated by a specified delimiter (comma by default).
const fruits = ["apple", "banana", "orange"];
const fruitString = fruits.join(", "); // fruitString
sort()
: This method sorts the elements of an array in place. By default, it sorts alphabetically (for strings) or numerically. You can provide a comparison function for custom sorting logic.
const numbers = [3, 1, 4, 2];
numbers.sort(); // numbers will be modified to [1, 2, 3, 4]
const fruits
= ["apple", "banana", "orange"];
fruits.sort((a, b) => b.length - a.length); // Sorts fruits by length (descending)
reverse()
: This method reverses the order of elements in the array in place.
const fruits = ["apple", "banana", "orange"];
fruits.reverse(); // fruits will be modified to ["orange", "banana", "apple"]
every()
: This method determines whether all elements in the array pass a test implemented by the provided function.
const numbers = [1, 2, 3];
const allPositive = numbers.every(number => number > 0); // allPositive will hold false (since 1 is not greater than 0)
some()
: This method checks if at least one element in the array passes the test implemented by the provided function.
const fruits = ["apple", "banana", "grape"];
const hasCitrus = fruits.some(fruit => fruit.includes("cit")); // hasCitrus will hold true (assuming "grapefruit" is added)
Best Practices for Effective Array Usage
To become an array maestro, consider these best practices:
- Choose the right data structure: While arrays are versatile, for specific use cases, other data structures like Sets or Maps might be more efficient. Evaluate your needs before blindly opting for arrays.
- Be mindful of mutability: Accidental modifications can lead to unexpected behavior. Consider using methods that create new arrays (like slice()) when necessary to avoid unintended side effects.
- Utilize helper functions: Break down complex array operations into smaller, reusable functions for better code organization and readability.
- Handle edge cases: Ensure your code gracefully handles empty arrays, missing elements, or invalid data types.
- Leverage modern features: Take advantage of ES6+ features like for…of loops, arrow functions, and destructuring for cleaner and more concise array manipulation.
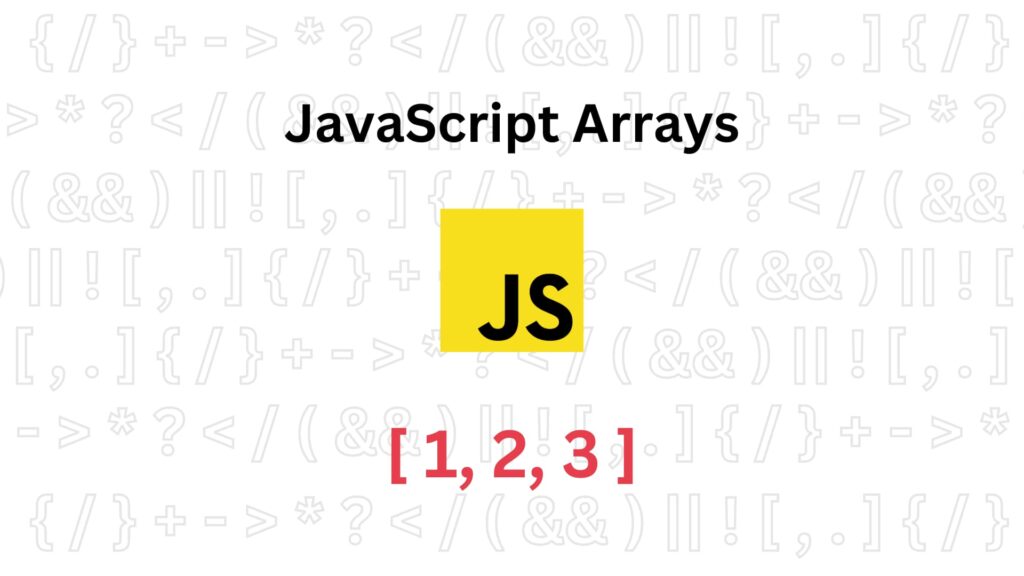
Exploring Advanced Concepts
As you delve deeper into JavaScript, you’ll encounter more advanced array concepts:
- Multidimensional Arrays: While not native JavaScript arrays, you can create arrays that hold other arrays, creating a grid-like structure for representing two-dimensional data (like matrices or tables).
- Flattening Arrays: Sometimes you might need to combine nested arrays into a single-level array. Methods like reduce() or recursion can be employed for this purpose.
- Custom Array Methods: JavaScript allows you to extend the functionality of arrays by creating your own methods using prototypes. This enables tailored solutions for specific use cases.
Conclusion: JavaScript Arrays
JavaScript arrays are fundamental to web development. Once you master creating and manipulating arrays and their associated iteration techniques, you will be prepared to address a variety of data-related problems. This guide covered nearly everything you needed to know about arrays. Make sure to practice playing with arrays, and you will soon become a whiz!
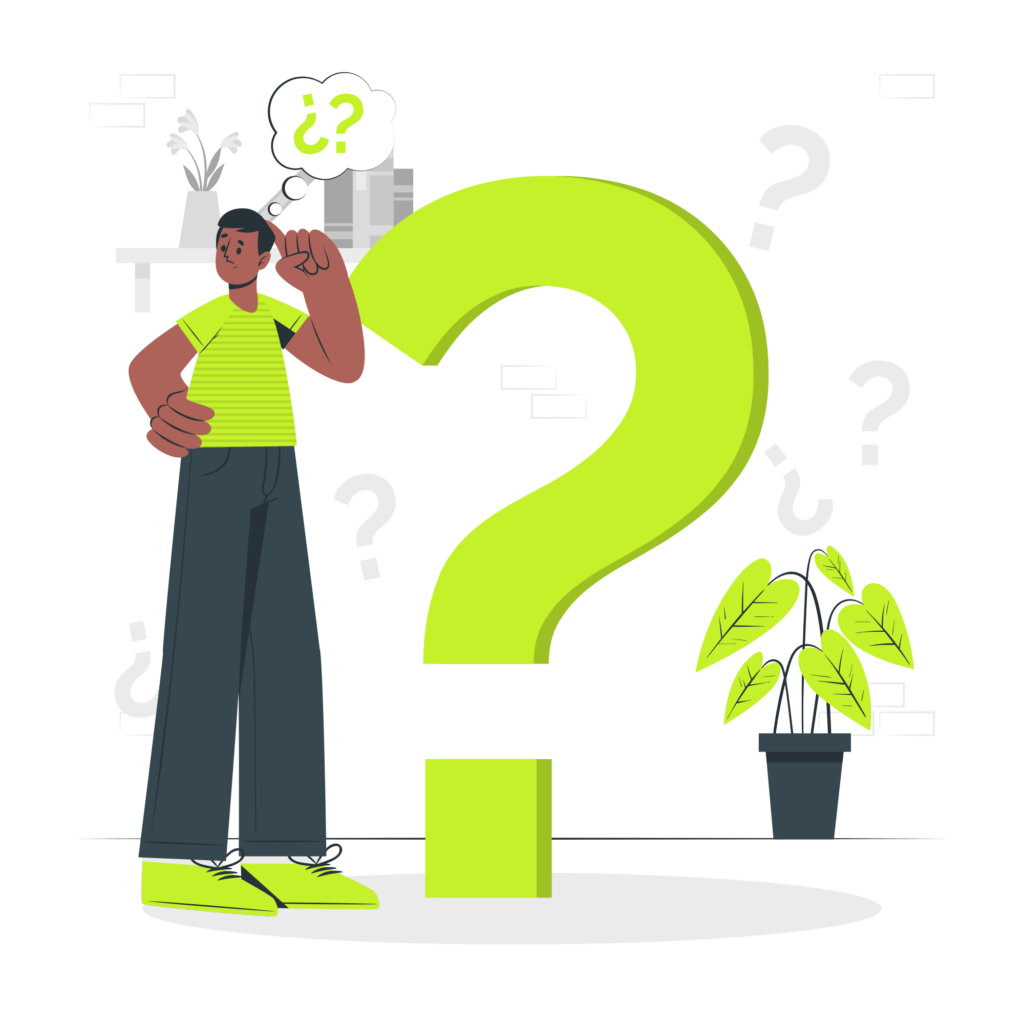
FAQs
What are JavaScript arrays and how are they used?
- JavaScript arrays are data structures that store multiple values in a single variable, used for storing lists of data like numbers, strings, or objects.
How can you create and initialize a JavaScript array?
- Arrays can be created using the Array constructor or by assigning values in square brackets, e.g.,
let myArray = [1, 2, 3];
.
What are some common methods to manipulate JavaScript arrays?
- Common methods include
.push()
,.pop()
,.shift()
,.unshift()
, and.splice()
for adding or removing elements.
How do you loop through a JavaScript array?
- You can loop through an array using
for
,forEach
,for...of
, ormap()
methods to perform operations on each element.
What is the difference between .slice()
and .splice()
in JavaScript arrays?
.slice()
returns a new array copy of a portion of the original array, while.splice()
changes the original array by adding or removing elements.
What is the importance of JavaScript in web development?
- JavaScript is crucial for creating interactive and dynamic websites, enabling functionalities like handling events, validating forms, and updating content without reloading the page.
How can JavaScript improve website performance?
- Efficient JavaScript code can enhance website speed and responsiveness, leading to improved user experience and better SEO rankings.
What are the best practices for coding with JavaScript?
- Best practices include using semantic variable names, keeping code DRY (Don’t Repeat Yourself), and regularly refactoring to improve readability and performance.
Can JavaScript be used for backend development?
- Yes, JavaScript can be used on the server side with Node.js, allowing developers to build and manage server-side applications with JavaScript.
What are some resources for learning advanced JavaScript?
- Advanced learners can explore books like “You Don’t Know JS” and online courses from platforms like Udemy, Coursera, or freeCodeCamp.