Imagine a web page as a magnificent castle. The blueprints outlining the structure and layout are similar to HTML (HyperText Markup Language). But a static blueprint doesn’t make a lively castle, right? That’s where the DOM (Document Object Model) comes in. It’s the dynamic 3D version of the blueprint where elements come alive, interact, and make the web page functional and engaging. When comparing HTML vs DOM, it’s clear that while HTML provides the essential structure, the DOM brings that structure to life, making the web experience interactive and functional.
What is HTML?
Think of HTML as the foundation and basic structure of your web page. It’s a simple language that uses tags written in angle brackets (<>
) to define the content and layout. Here’s a breakdown:
- Tags: These are instructions for the browser like
<p>
for paragraph or<h1>
for heading. They come in pairs with an opening tag (e.g.<p>
) and a closing tag (e.g.</p>
) to define the element’s scope. - Content: The text, images, videos, and other elements you see on the page go between the opening and closing tags.
- Attributes: These provide additional information about an element, like giving a heading an
id
or an image asrc
(source) attribute.
For instance, the following HTML code creates a simple heading and paragraph:
<h1>Welcome to My Castle!</h1>
<p>This is the main hall.</p>
This code instructs the browser to display “Welcome to My Castle!” as a heading (likely bigger and bolder) and “This is the main hall.” as a paragraph.
While HTML provides the structure, it’s like a static image. It can’t change or respond to user interaction. That’s where the DOM steps in.
About the DOM
The DOM is a tree-like representation of the web page created by the browser after it reads the HTML code. It’s a dynamic model where each HTML element becomes an object, and the tags define the object’s properties and relationships.
Here’s how the magic unfolds:
- Browser Parses the HTML: When you load a web page, the browser reads the HTML code line by line.
- Creating the DOM Tree: Each element in the HTML code becomes a node in the DOM tree. The
<html>
element is the root node, and all other elements branch out from it, mimicking the parent-child relationships defined in the HTML. - Adding Functionality: The DOM doesn’t just represent the structure; it also adds properties and methods to each element. These allow you to manipulate the elements using programming languages like JavaScript.
Imagine our previous HTML code. In the DOM, the heading “Welcome to My Castle!” becomes an object with properties like text content, style information (font size, color), and a reference to its position in the tree (child of the main document object).
Power of DOM Manipulation
With JavaScript, you can leverage the DOM to make your web page interactive and dynamic. Here are some ways you can use DOM manipulation:
- Changing Content: Update text, images, or any element’s content on the fly without reloading the page. Imagine a button click that changes the text in a paragraph.
- Adding or Removing Elements: Dynamically add new elements (like pop-up windows) or remove existing ones based on user interaction.
- Styling Elements: Change the style (color, font size, etc.) of elements after the page loads. This lets you create hover effects, animations, and more.
- Responding to Events: Capture user interactions like clicks, scrolls, and key presses, and trigger actions based on them. This allows for forms, interactive games, and dynamic web experiences.
The possibilities with DOM manipulation are vast. It’s like having a toolbox to control every aspect of your web page after it’s loaded, making it truly interactive and engaging.
A Real-world Example
Let’s revisit our castle example and see how DOM manipulation can enhance it. We can add a button that, when clicked, changes the text in the main hall paragraph to “Welcome to the Throne Room!” using JavaScript:
<button id="throneRoomButton">Enter Throne Room</button>
<p id="mainHallText">This is the main hall.</p>
const button = document.getElementById("throneRoomButton");
const paragraph = document.getElementById("mainHallText");
button.addEventListener("click", function() {
paragraph.textContent = "Welcome to the Throne Room!";
});
Further Adventures with HTML and DOM
In the previous section, we explored the basic concepts of HTML and DOM. Now, let’s dive deeper and discover some advanced techniques that truly bring your webpages to life.
Peek into DOM Nodes
Remember the DOM tree? Each element in the HTML becomes a node in this tree. There are different types of nodes that play specific roles:
- Element Nodes: These represent the HTML elements like headings, paragraphs, buttons, images, etc. They are the workhorses of the DOM, containing content, attributes, and properties.
- Attribute Nodes: These are attached to element nodes and provide additional information about them. For example, an
id
attribute or aclass
attribute associated with an element node. - Text Nodes: These contain the actual text content displayed on the webpage. They reside within element nodes and define what the user sees.
- Comment Nodes: These are used to include comments within the HTML code that are not displayed on the webpage but are helpful for developers.
Understanding these node types is crucial for effectively manipulating the DOM using JavaScript.
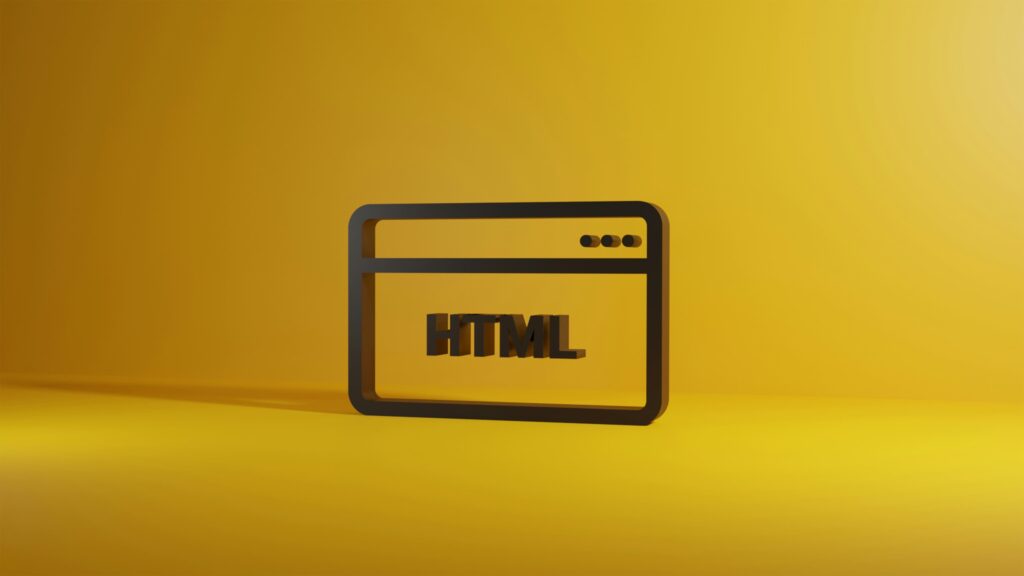
What are Selectors?
Imagine a vast castle with countless rooms. To interact with specific elements (like the throne room button), you need a way to find them. That’s where selectors come in.
Selectors are patterns used in JavaScript to target specific nodes in the DOM tree. Here are some common types:
- ID Selectors: These target elements with a unique
id
attribute. They are the most precise way to find an element, likedocument.getElementById("throneRoomButton")
. - Tag Selectors: These target all elements of a specific HTML tag, like
document.getElementsByTagName("p")
to find all paragraph elements. - Class Selectors: These target elements with a specific CSS class applied, like
document.getElementsByClassName("redButton")
to find all elements with the class “redButton”. - CSS Selectors: You can leverage the power of CSS selectors to target elements based on a combination of attributes, tags, and class names. This offers more flexibility, like selecting all paragraphs with a class “important” within a specific
div
element.
By mastering selectors, you can efficiently navigate the DOM tree and interact with the desired elements.
DOM Manipulation and CSS
Cascading Style Sheets (CSS) define the visual appearance of your web page. But DOM manipulation can work hand-in-hand with CSS to create dynamic styles.
Here’s how:
- Changing CSS Classes: You can add, remove, or toggle CSS classes on elements using JavaScript. This allows you to create effects like hover effects, where an element changes color or style when the user hovers over it.
- Modifying Styles Directly: Using JavaScript, you can directly modify the style properties of elements. Imagine dimming the lights in a room by changing the
opacity
property of an element representing a light source.
This interplay between DOM manipulation and CSS unlocks a whole new level of dynamic and interactive web design.
What are Events?
Events are user interactions like clicks, scrolls, key presses, or mouse movements. The DOM provides a way to capture these events and trigger actions in response.
Here’s the magic:
- Event Listeners: You can attach event listeners to elements using JavaScript. These listeners wait for a specific event to occur and then execute a function you define.
- Event Handlers: The function you define in the event listener is called the event handler. This code determines what happens when the event occurs. For example, an event handler for a click event on a button could change the content of a paragraph.
Events are the heart of interactivity. They allow users to control the webpage and create dynamic experiences.
Exploring Advanced DOM Techniques
As you dive deeper into web development, you’ll encounter even more powerful DOM manipulation techniques:
- DOM Traversal: Methods to navigate the DOM tree and access related elements, like moving from a parent element to its child nodes.
- Creating and Deleting Elements: Dynamically add new elements to the DOM or remove existing ones based on user interaction or other conditions.
- AJAX (Asynchronous JavaScript and XML): Techniques to update parts of a web page without reloading the entire page, creating a seamless user experience.
These advanced concepts allow you to build complex web applications with dynamic features and user interactions.
Conclusion: HTML vs DOM
By understanding HTML and DOM, you gain the power to create web pages that are not just informative but truly interactive and engaging. Imagine a castle that responds to your clicks, unveils hidden rooms, and provides a dynamic experience.
The journey of mastering HTML and DOM is like building a magnificent web presence brick by brick. You start with the solid foundation of HTML, defining the structure and content. Then the DOM breathes life into it, allowing you to manipulate elements, add interactivity, and create dynamic experiences.
FAQs
What is HTML?
- HTML (HyperText Markup Language) is the standard language used to create and structure content on the web.
What is the DOM?
- The DOM (Document Object Model) is a programming interface that allows scripts to update the content, structure, and style of a website dynamically.
How do HTML and DOM work together?
- HTML defines the structure and content of a webpage, while the DOM represents this content as objects that can be manipulated with JavaScript.
Why is understanding HTML and DOM important for web development?
- Understanding both is crucial for creating dynamic, interactive, and responsive web applications.
Can you modify HTML using the DOM?
- Yes, using JavaScript, developers can manipulate the DOM to change the HTML structure, content, and style in real-time.
What are some common uses of the DOM in web development?
- The DOM is used for tasks such as form validation, dynamic content updates, and handling user interactions.
How does JavaScript interact with the DOM?
- JavaScript can select, modify, add, or remove elements within the DOM, allowing for dynamic updates to the webpage.
What tools can help with HTML and DOM manipulation?
- Tools like Chrome DevTools, Firefox Developer Tools, and libraries like jQuery and React can aid in manipulating HTML and the DOM.
Are HTML and DOM standards maintained by the same organization?
- Yes, both HTML and DOM standards are maintained by the World Wide Web Consortium (W3C).
Can you use the DOM without HTML?
- No, the DOM is an abstraction of the HTML document, so HTML is necessary for the DOM to exist and be manipulated.