Imagine creating a sophisticated website that showcases your favorite musicians, complete with their names, genres, and intriguing facts. To effectively manage and display this information, a robust and efficient data handling approach is essential. This is where the powerful combination of JavaScript (JS) and JSON (JavaScript Object Notation) comes into play.
In this article, we will delve into the synergistic relationship between JavaScript and JSON, demonstrating how they can be used to store, manipulate, and present data seamlessly on your web pages. Whether you are a budding web developer or a seasoned professional looking to refine your skills, mastering the use of JS and JSON is crucial for creating dynamic, data-driven websites.
JavaScript
JavaScript is a versatile programming language capable of performing a wide range of tasks, from adding interactivity to web pages to manipulating complex data structures. Its true power is evident when working with JSON, as it seamlessly handles this data format with ease and efficiency.
JSON
JSON is a lightweight, human-readable format for storing and exchanging information. It uses a simple structure based on key-value pairs, similar to how you might organize information in a notebook. Here’s an example of JSON representing a musician:
{
"name": "The Beatles",
"genre": "Rock",
"funFact": "They hold the record for most number-one hits on the Billboard Hot 100 chart"
}
In this example, “name,” “genre,” and “funFact” are the keys, and “The Beatles,” “Rock,” and the provided text are the corresponding values. The beauty of JSON is its universality. It’s language-independent, meaning different programming languages, including JavaScript, can understand and work with JSON data.
From JSON to JavaScript and Back
While JSON might look like plain text, JavaScript can’t directly use it. Here’s where the magic happens! JavaScript provides built-in methods to convert between JSON and JavaScript objects.
- Deserialization: Transforming JSON into a JavaScript object. Imagine unpacking a box of data (JSON) and organizing it into a neat structure (JavaScript object) for easy access.
- Serialization: Converting a JavaScript object back into a JSON string. Think of packing your organized data (JavaScript object) back into a box (JSON string) for storage or transmission.
JavaScript’s JSON.parse()
method performs deserialization, taking a JSON string and returning a corresponding JavaScript object. Here’s how to use it with our musician example:
const musicianData = '{ "name": "The Beatles", "genre": "Rock", "funFact": "They hold the record for most number-one hits on the Billboard Hot 100 chart" }';
const musicianObject = JSON.parse(musicianData);
console.log(musicianObject.name); // Outputs: "The Beatles"
For serialization, JavaScript offers the JSON.stringify()
method. It takes a JavaScript object and returns a JSON string representation. Let’s say you want to store the musician data in a file:
const musicianObject = {
name: "The Beatles",
genre: "Rock",
funFact: "They hold the record for most number-one hits on the Billboard Hot 100 chart"
};
const jsonData = JSON.stringify(musicianObject);
console.log(jsonData); // Outputs: {"name":"The Beatles","genre":"Rock","funFact":"They hold the record for most number-one hits on the Billboard Hot 100 chart"}
Why Use JSON ?
JSON has several advantages that make it a perfect partner for JavaScript:
- Lightweight: It’s smaller compared to other data formats, making transmission faster.
- Human-Readable: Unlike some binary formats, JSON is easy for humans to understand and edit if needed.
- Language-Independent: Different programming languages can interpret and work with JSON data.
- Flexibility: JSON can represent various data types like strings, numbers, booleans, arrays (ordered lists), and even nested objects (objects within objects).
These features make JSON ideal for various scenarios in web development:
- Fetching Data from Servers: Websites often retrieve information from servers. JSON’s lightweight nature and ease of use make it a popular choice for data exchange. Imagine fetching a list of products from a server for your online store; JSON would be a perfect fit.
- Exchanging Data between Applications: JavaScript applications can leverage JSON to share data seamlessly, even if they’re developed using different technologies.
- Storing Application Data: JSON files can store configuration settings or user preferences for your web application.
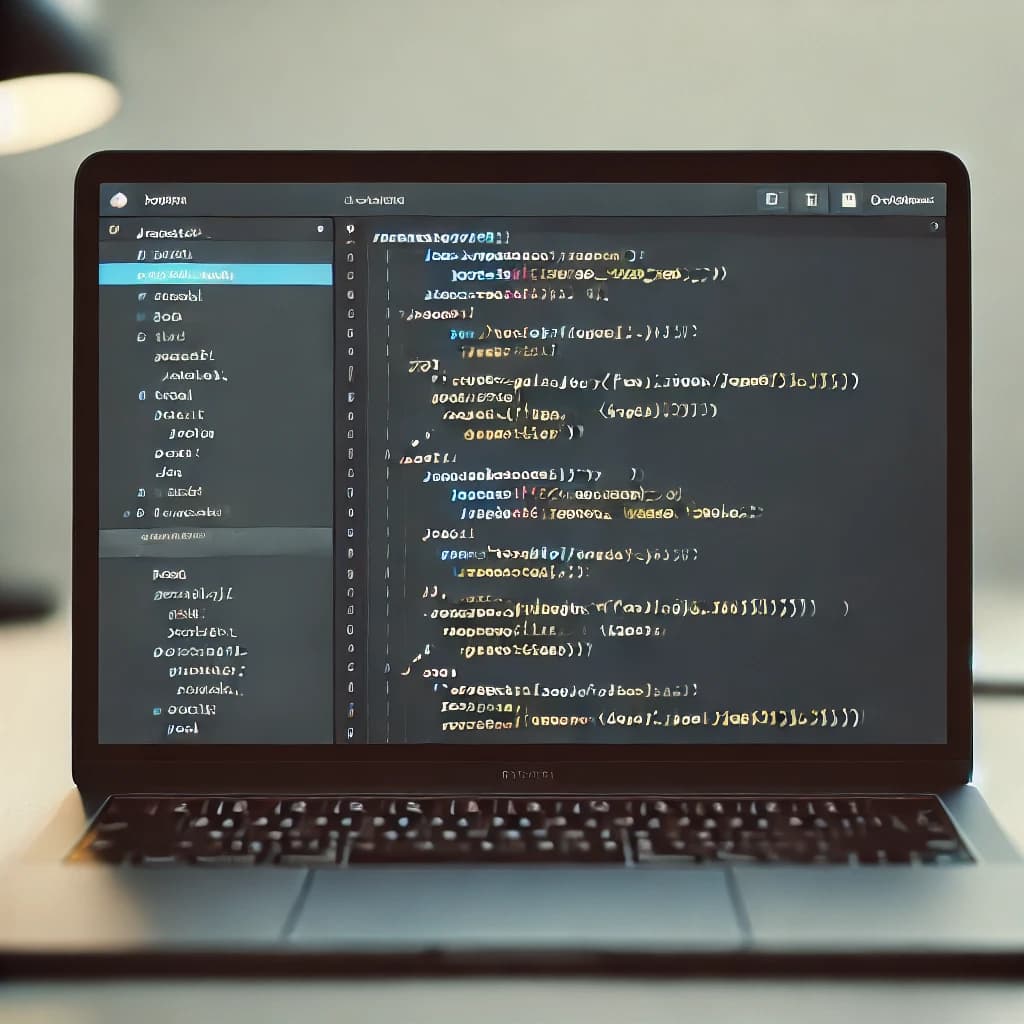
A Match Made in Web Development Heaven
By combining the power of JavaScript with the simplicity of JSON, you can create dynamic and data-driven web applications. Here’s a glimpse into what you can achieve:
- Interactive Web Pages: Update content on your webpage without full page reloads by manipulating the Document Object Model (DOM) based on your JSON data.
- Data Visualization: Present your data in an engaging and informative way using charting libraries like Chart.js or D3.js. These libraries work seamlessly with JavaScript objects (derived from JSON data) to create interactive charts, graphs, and other visual representations.
- Single-Page Applications (SPAs): Develop modern web applications that feel more like desktop applications. SPAs load a single HTML page initially and then dynamically update the content using JavaScript and AJAX. This creates a faster and more responsive user experience. JSON plays a crucial role in managing data within these applications.
- Offline Functionality: Provide a limited set of features even when users are offline. By storing essential data locally (often in JSON format) and implementing caching mechanisms, you can enhance the user experience even in situations with limited internet connectivity.
Accessing and Modifying JSON Data
Once you have a JavaScript object representing your JSON data (through deserialization), you can access and modify its properties using familiar dot notation or bracket notation:
const musicianObject = {
name: "The Beatles",
genre: "Rock",
funFact: "They hold the record for most number-one hits on the Billboard Hot 100 chart"
};
console.log(musicianObject.name); // Outputs: "The Beatles"
console.log(musicianObject["genre"]); // Outputs: "Rock" (using bracket notation)
musicianObject.genre = "Rock and Pop"; // Modifying a property
console.log(musicianObject.genre); // Outputs: "Rock and Pop" (updated value)
Looping Through JSON Arrays
JSON arrays, represented by square brackets []
, hold ordered collections of values. You can leverage JavaScript’s looping constructs (like for
loops or forEach
) to iterate through each element in an array:
const instruments = ["Guitar", "Drums", "Bass"];
for (let i = 0; i < instruments.length; i++) {
console.log(instruments[i]); // Outputs: "Guitar", "Drums", "Bass"
}
instruments.forEach(instrument => console.log(instrument)); // Using forEach loop
This allows you to dynamically display information or create interactive elements based on the data within the array.
Building Dynamic Content with DOM Manipulation
The Document Object Model (DOM) represents the structure of your web page. JavaScript allows you to manipulate the DOM, adding, removing, and modifying elements based on your JSON data. Here’s a simplified example:
<div id="musician-info"></div>
const musicianObject = {
name: "The Beatles",
genre: "Rock and Pop"
};
const infoDiv = document.getElementById("musician-info");
infoDiv.innerHTML = `<h2>${musicianObject.name}</h2><p>Genre: ${musicianObject.genre}</p>`;
This code retrieves the element with the ID “musician-info” and uses string interpolation (backticks
) to dynamically insert the musician’s name and genre within the element.
Fetching Data from External Sources with AJAX
AJAX (Asynchronous JavaScript and XML) allows you to retrieve data from a server without reloading the entire page. This is a powerful technique for keeping your web page dynamic and responsive. Here’s a simplified breakdown:
- Create an XMLHttpRequest object: This object handles communication with the server.
- Open a connection: Specify the URL of the resource you want to fetch (often containing JSON data).
- Define a callback function: This function executes when the data is successfully retrieved.
- Parse the JSON data: Use
JSON.parse()
to convert the received text into a JavaScript object. - Use the data: Now you have a JavaScript object representing the fetched data, and you can manipulate it as needed to update your web page.
A Practical Example
Imagine building a simple music player application. You can store song information (title, artist, genre) in a JSON file. Your JavaScript code can then:
- Fetch the JSON data using AJAX.
- Parse the data and create JavaScript objects representing each song.
- Use DOM manipulation to dynamically create a list of songs on your webpage.
- Add event listeners to each song item, allowing users to play the corresponding audio file.
This is just a basic example, but it demonstrates how JavaScript and JSON can work together to create a more engaging and interactive user experience.
Beyond the Basics
As you become more proficient, you can explore advanced techniques to further enhance your data-driven web applications:
- Error Handling: Implement mechanisms to handle potential errors during data fetching or parsing.
- Data Validation: Ensure the integrity of your data by validating it before using it in your application.
- Templating Engines: Utilize templating engines like Handlebars or Mustache.js to separate your data logic from the HTML structure for cleaner and more maintainable code.
- Client-Side Libraries: Explore libraries like Axios or Fetch API for simplified and streamlined data fetching capabilities.
Conclusion: Javascript and JSON
JavaScript and JSON form a formidable duo for managing data in web development. Their versatility and ease of use make them ideal for building dynamic and interactive web applications. From simple content updates to complex data visualizations and real-time interactions, this powerful partnership empowers you to create engaging user experiences.
As you dive deeper into web development, mastering JavaScript and JSON will equip you with the tools to build robust and user-centric applications. Remember, this dynamic duo is constantly evolving, with new features and libraries emerging to push the boundaries of what’s possible on the web. So, keep exploring, keep learning, and keep creating amazing web experiences!
FAQs:
How do you parse JSON in JavaScript?
- Use
JSON.parse()
to convert a JSON string into a JavaScript object.
How do you convert a JavaScript object to JSON?
- Use
JSON.stringify()
to convert a JavaScript object into a JSON string.
What are common uses of JSON in web development?
- JSON is commonly used for data exchange between a server and a web application, configuration files, and APIs.
Can JSON store arrays and nested objects?
- Yes, JSON can store arrays and nested objects, making it a versatile format for representing complex data structures.
What are the differences between JSON and XML?
- JSON is easier to read and write, more compact, and has better performance compared to XML, which is more verbose and requires parsing.
Is JSON language-dependent?
- No, JSON is language-independent, though it is based on JavaScript syntax. It is supported by many programming languages.
How do you handle errors when parsing JSON in JavaScript?
- Use
try...catch
blocks to handle errors that may occur during JSON parsing.
What is the significance of keys in JSON objects?
- Keys in JSON objects are always strings and must be unique within the same object.
Can you modify JSON data after parsing it in JavaScript?
- Yes, once JSON data is parsed into a JavaScript object, you can modify it like any other object.