JavaScript (JS) is the most common language used to make websites interactive. From the tiny animations that grab your attention to the complex applications running seamlessly in your browser, JavaScript plays a huge role in modern web development. But how JavaScript works might seem like magic. his article delves into the inner workings of what is JavaScript, breaking down JavaScript code to explore the key components and mechanisms that bring websites to life. We’ll uncover the secrets behind how JavaScript works and let you to understand the magic behind those interactive experiences.
What is JavaScript?
When you open a website, your computer and the tiny programs inside (like the browser) work together to make it work. JavaScript is like a special set of instructions hidden in the website that tells the browser what to do, kind of like following a recipe. Here’s a simplified overview of the process:
- The Download: When you request a web page, the browser fetches the HTML content along with any associated JavaScript files.
- Parsing and Interpretation: The browser’s JavaScript engine parses the code, turning it into a format the computer can understand. This often involves breaking down the code into an Abstract Syntax Tree (AST), a structured representation of the code’s elements.
- Execution: The parsed code is then executed line by line within the JavaScript engine.
- Interaction with the Web Page: The code can manipulate the Document Object Model (DOM), the browser’s internal representation of the web page, to update content, add animations, handle user interactions, and more.
This is a high-level view, but it highlights the core steps involved in bringing JavaScript code to life. Let’s delve deeper into the essential components that make this process possible.
About The JavaScript Engine
The JavaScript engine is the software program embedded within the browser responsible for interpreting and executing JavaScript code. Different browsers use different engines, with some of the most popular ones being:
-
- Google Chrome: V8 Engine
- Mozilla Firefox: SpiderMonkey Engine
- Safari: JavaScriptCore Engine
- Microsoft Edge: V8 Engine
These engines are responsible for several key tasks:
-
- Parsing: The engine reads the JavaScript code and breaks it down into a format the computer can understand.
- Code Execution: The engine interprets the parsed code and executes the instructions line by line.
- Memory Management: The engine allocates and manages memory for variables, objects, and other data structures used within the code.
- Interaction with the Browser: The engine interacts with the browser’s environment, allowing the code to access and manipulate the web page.
Modern JavaScript engines are highly optimized, often employing techniques like Just-in-Time (JIT) compilation to improve the speed of code execution. JIT compilation involves dynamically translating the code into machine code specific to the underlying hardware for faster processing.
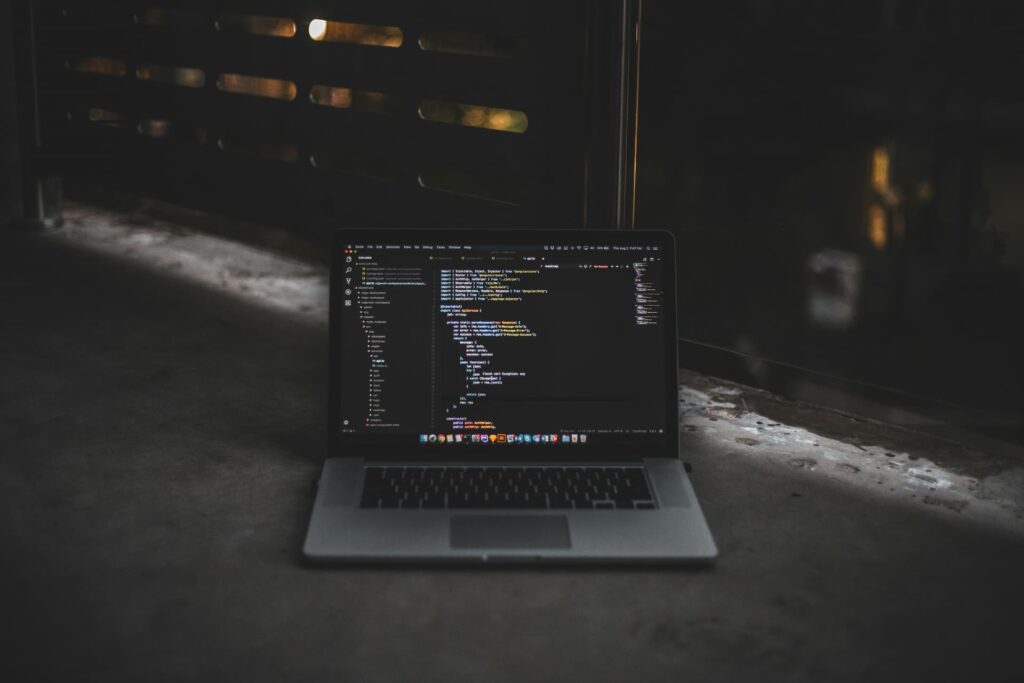
Understanding the Code Execution Environment
When JavaScript code is executed, it doesn’t operate in a vacuum. The engine creates a specific environment for each piece of code to run in. Two crucial components of this environment are:
-
- Call Stack: The call stack is a data structure that keeps track of the functions currently being executed. Each time a function is called, a new frame is pushed onto the stack. This frame holds information about the function’s arguments, local variables, and the place where execution should resume after the function finishes. When the function returns, its frame is popped off the stack. Breaking down JavaScript code involves understanding how the call stack manages the order of function execution.
-
- Heap: The heap is a memory space where the engine stores objects and dynamically allocated data. Unlike the call stack, which has a predefined structure, the heap is a more flexible memory pool that grows and shrinks as needed during program execution.
The call stack and heap work together to manage the execution of your code. The call stack dictates the order of function execution, while the heap stores the data used by those functions.
JavaScript’s Asynchronous Nature
One of JavaScript’s defining characteristics is its single-threaded nature. This means it can only execute one task at a time. However, this doesn’t prevent JavaScript from handling multiple events or performing long-running operations. This is achieved through a combination of the Event Loop and Callbacks:
-
- Event Loop: The event loop is a core mechanism within the JavaScript engine that continuously monitors two main sources:
-
-
- The call stack: The event loop checks if there are any functions waiting to be executed on the call stack.
-
- The task queue: This queue holds callbacks, functions that are scheduled to be executed later.
- Callbacks: Callbacks are functions that are passed as arguments to other functions. These functions are then invoked at a later point in time, typically when an asynchronous operation (like fetching data from a server) completes.
When the event loop finds an empty call stack, it checks the task queue. If there are any callbacks waiting, it removes one from the queue, pushes it onto the call stack, and executes it. This cycle continues, ensuring that even while the main thread is busy, other tasks can be queued and executed when possible. This asynchronous approach allows JavaScript to remain responsive to user interactions while handling long-running operations in the background.
Core JavaScript Concepts
JavaScript provides a rich set of features that allow you to build complex and interactive web applications. Here’s a look at some of the fundamental building blocks:
-
- Variables and Data Types: Variables are named containers that store data. JavaScript is dynamically typed, meaning the data type of a variable is determined at runtime. Common data types include numbers, strings, booleans, objects, and arrays.
-
- Operators: Operators perform operations on data. These include arithmetic operators (+, -, *, /), comparison operators (==, !=, <, >), logical operators (&&, ||, !), and more.
-
- Control Flow Statements: These statements control the flow of execution within your code. Examples include conditional statements (if, else), loops (for, while), and switch statements.
-
- Functions: Functions are reusable blocks of code that perform a specific task. They can take arguments and return values. Functions are essential for modularizing your code and promoting code reusability.
-
- Objects: Objects are fundamental data structures that store key-value pairs. They allow you to group related data and functionality together. Objects form the backbone of many web development frameworks and libraries.
-
- Arrays: Arrays are ordered collections of items that can hold various data types. They are essential for storing lists, sequences, and collections of data within your program.
Understanding these core concepts is fundamental for writing effective JavaScript code.
Advanced Features for Powerful Applications
JavaScript offers a vast array of features that enable the development of sophisticated web applications. Here are some key highlights:
-
- Closures: Closures are a powerful concept in JavaScript that allows functions to access variables from their outer (enclosing) scope even after the outer function has returned. This allows for data privacy and encapsulation within functions.
function createGreeter(greeting) {
// Inner function that stores the greeting
function greet(name) {
return `${greeting}, ${name}!`;
}
// Return the inner function with access to the greeting variable
return greet;
}
// Create a greeter function with a specific greeting
const morningGreeter = createGreeter("Good Morning");
// Call the returned function (greet) using the morningGreeter variable
console.log(morningGreeter("Alice")); // Output: Good Morning, Alice!
In this example, the greet function has access to the greeting variable even after the createGreeter function has finished executing. This closure allows us to create reusable functions with private data.
-
- Prototypes and Inheritance: Prototypes are a mechanism for creating objects that inherit properties and methods from other objects. This promotes code reusability and simplifies object-oriented programming in JavaScript.
// Define a base object (prototype) for Person
function Person(name) {
this.name = name;
}
// Add a greet method to the Person prototype
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}`);
}
// Create a new object (Developer) inheriting from Person
function Developer(name, skills) {
this.skills = skills;
// Call the Person constructor using call() to inherit properties
Person.call(this, name);
}
// Inherit the greet method from Person
Developer.prototype = Object.create(Person.prototype);
// Create a new Developer object
const alice = new Developer("Alice", ["JavaScript", "Python"]);
// Use the inherited greet method
alice.greet(); // Output: Hello, my name is Alice
This example demonstrates how the Developer object inherits properties and methods from the Person prototype, promoting code reuse and organization.
-
- DOM Manipulation: As mentioned earlier, JavaScript can interact with the Document Object Model (DOM), the browser’s internal representation of the web page. This allows you to dynamically change content, add and remove elements, and respond to user interactions.
// Select the paragraph element with ID "myParagraph"
const paragraph = document.getElementById("myParagraph");
// Change the text content of the paragraph
paragraph.textContent = "This paragraph content has been changed by JavaScript!";
// Create a new button element
const newButton = document.createElement("button");
newButton.textContent = "Click Me!";
// Add the button element to the body of the page
document.body.appendChild(newButton);
This example shows how to select an existing element, modify its content, and even create and add new elements to the DOM using JavaScript.
-
- Event Handling: JavaScript can listen for events triggered by user interactions (clicks, scrolls, etc.) and browser events (page load, resize, etc.). This allows your application to react to user actions and adapt to changes in the browser environment.
// Select the button element with ID "myButton"
const button = document.getElementById("myButton");
// Add a click event listener to the button
button.addEventListener("click", function() {
alert("You clicked the button!");
});
This example shows how to listen for a click event on a button and execute a function (showing an alert) when the click occurs.
-
- Asynchronous Programming (Promises, Async/Await): Asynchronous programming techniques like Promises and Async/Await allow you to handle long-running operations without blocking the main thread. This keeps your application responsive and improves user experience.
These advanced features unlock the full potential of JavaScript for building complex and interactive web applications.
JavaScript Beyond the Browser
While JavaScript’s primary domain is web development, its reach extends beyond the browser. Here are some additional environments where JavaScript plays a crucial role:
-
- Node.js: Node.js is a runtime environment that allows you to run JavaScript code outside of the browser. This opens doors for server-side development, building command-line tools, and creating real-time applications.
- Desktop Applications: Frameworks like Electron enable you to build desktop applications using web technologies like HTML, CSS, and JavaScript. This allows for cross-platform development and a unified codebase for web and desktop applications.
-
- Mobile Development: Frameworks like React Native allow you to build native mobile applications using JavaScript. This streamlines mobile development by leveraging existing web development skills and codebases.
The versatility of JavaScript makes it a valuable tool for developers across various domains.
Conclusion: How JavaScript Works?
JavaScript’s ability to breathe life into web pages and power interactive applications lies in its well-defined execution process, efficient engines, and powerful built-in features. By understanding the core concepts(what is javascript?, how does it work), advanced features, and broader ecosystem, you can harness the true potential of JavaScript to create dynamic and engaging web experiences.
Wanna Learn more about JavaScript? Check our articles on JavaScript 👇
-
- How JavaScript Works?
- JavaScript Varialbles
- JavaScript Operators
- JavaScript Functions
- JavaScript Arrays
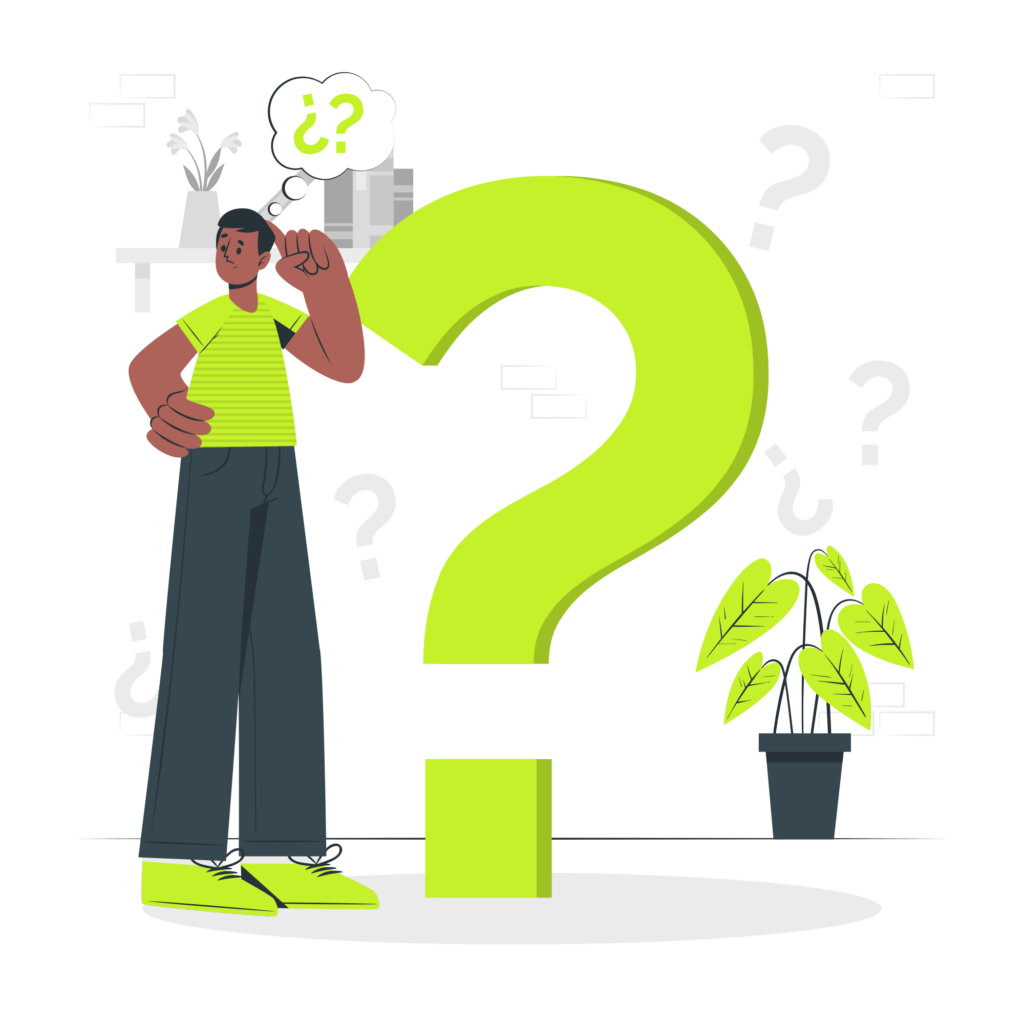
FAQs
How does JavaScript work in a web browser?
-
- JavaScript is executed by the browser’s JavaScript engine, interacting with the HTML and CSS to manipulate the DOM and make web pages interactive.
What are JavaScript events and how do they function?
-
- JavaScript events are actions that occur within the browser, which JavaScript can respond to, like user clicks or keypresses, to trigger specific functions.
How does JavaScript handle asynchronous operations?
-
- JavaScript handles asynchronous operations using callbacks, promises, and async/await, allowing non-blocking operations like server requests.
Can JavaScript run outside a web browser?
-
- Yes, JavaScript can run on servers or even on local machines, mainly through environments like Node.js, extending its capabilities beyond web browsers.
What is the execution context in JavaScript?
-
- The execution context is the environment in which JavaScript code is executed and evaluated, including global and function-level contexts.
What is the significance of JavaScript in web development?
-
- JavaScript is crucial for adding interactivity, handling user input, and creating dynamic content in web applications, enhancing user experience.
How do web browsers parse JavaScript code?
-
- Browsers parse JavaScript code by first converting it into an abstract syntax tree (AST) and then executing it, often compiling it to bytecode or machine code.
What are some common use cases for JavaScript?
-
- Common use cases include form validation, dynamic page updates, animations, and building web applications with frameworks like React or Angular.
How does JavaScript interact with HTML and CSS?
-
- JavaScript interacts with HTML and CSS by manipulating the DOM, allowing it to change elements, styles, and respond to user interactions dynamically.
What is the Document Object Model (DOM) in JavaScript?
-
- The DOM is a programming interface for web documents, representing the page structure as a tree, which JavaScript can manipulate to change document content and structure.