JavaScript operators are the building blocks that bring your code to life. They perform actions on values, compare data, control program flow, and manipulate data structures. Understanding these operators is fundamental to mastering JavaScript and creating dynamic and interactive web experiences. This comprehensive guide delves into the various types of JS operators, their functionalities, and how to use them effectively in your code.
What are the Types of Javascript Operators?
JavaScript boasts a rich set of operators, each with a specific purpose. Let’s explore the most commonly used categories:
01. Assignment Operators
These operators assign values to variables. The most basic is the single equal sign (=
), which assigns the value on the right to the variable on the left. For example:
let age = 25;
JavaScript also offers compound assignment operators that perform an operation and then assign the result. These include:
+=
: Adds and assigns (e.g.,age += 5
is equivalent toage = age + 5
)=
: Subtracts and assigns=
: Multiplies and assigns/=
: Divides and assigns%=
: Computes the remainder and assigns
02. Arithmetic Operators
These operators perform mathematical calculations on numbers.
+
: Addition (e.g.,10 + 5
evaluates to 15)-
: Subtraction (e.g.,20 - 3
evaluates to 17)*
: Multiplication (e.g.,4 * 6
evaluates to 24)/
: Division (e.g.,30 / 2
evaluates to 15)%
: Modulus (remainder after division) (e.g.,11 % 3
evaluates to 2)*
: Exponentiation (raising a number to a power) (e.g.,2 ** 3
evaluates to 8)
03. Comparison Operators
These operators compare values and return a boolean (true or false) result. They include:
==
: Loose equality (checks for equal value, can perform type coercion) (e.g.,1 == "1"
evaluates to true)===
: Strict equality (checks for equal value and type) (e.g.,1 === "1"
evaluates to false)!=
: Not equal (opposite of==
)!==
: Strict not equal (opposite of===
)<
: Less than<=
: Less than or equal to>
: Greater than>=
: Greater than or equal to
04. Logical Operators
These operators combine boolean expressions to create more complex logical conditions.
&&
: AND operator (both operands must be true for the result to be true) (e.g.,(age > 18) && (isCitizen === true)
evaluates to true if age is greater than 18 and isCitizen is true)||
: OR operator (at least one operand must be true for the result to be true) (e.g.,(loggedIn === true) || (hasAdminRights === true)
evaluates to true if either loggedIn or hasAdminRights is true)!
: NOT operator (inverts the boolean value) (e.g.,!(age < 13)
evaluates to true if age is not less than 13).
05. Conditional (Ternary) Operator
This operator provides a concise way to write an if-else statement in a single line. The syntax is:
condition ? expression_if_true : expression_if_false
For example:
let message = (age >= 18) ? "You are eligible to vote." : "You are not eligible to vote.";
06. Type Operators
These operators check the data type of a value. The most common ones are:
typeof
: Returns the data type of a value (e.g.,typeof(10)
evaluates to “number”)instanceof
: Checks if an object is an instance of a specific constructor (e.g.,object instanceof Array
checks if the object is an array)
07. Bitwise Operators
These operators perform bit-level operations on numbers. They are less commonly used in everyday JavaScript but can be helpful for low-level manipulation. Some examples include:
&
: Bitwise AND|
: Bitwise OR^
: Bitwise XOR<<
: Left shift>>
: Right shift~
: Bitwise NOT
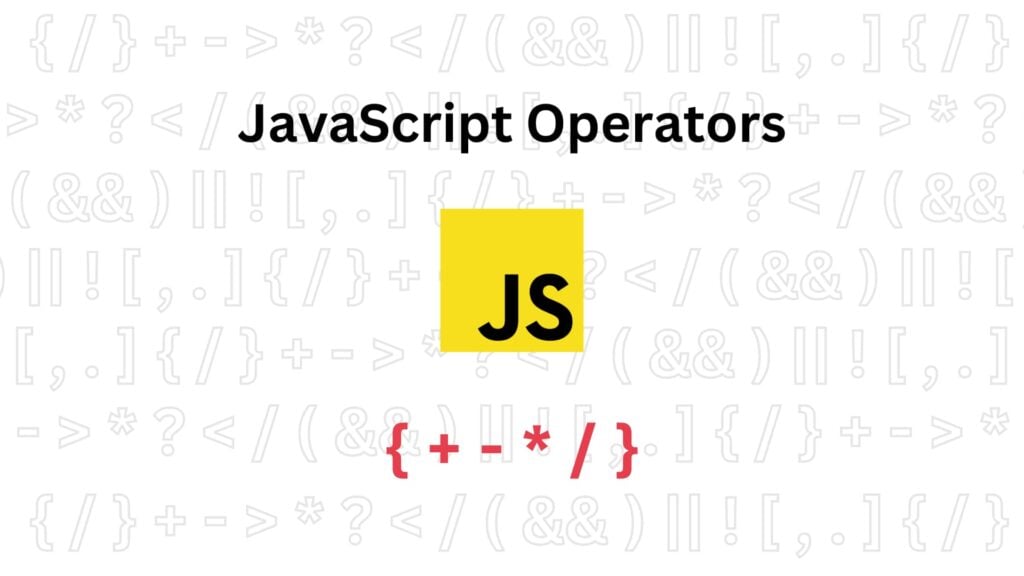
08. String Operators
JavaScript primarily uses the +
operator for string concatenation. However, there are a few nuances:
- +: When used with strings, it concatenates them (e.g.,
"Hello" + " World"
evaluates to “Hello World”) - When used with at least one numeric operand, it converts the other operand to a string and then concatenates (e.g.,
"Age: " + age
evaluates to a string like “Age: 25” if age is 25)
09. Increment and Decrement Operators
These operators are used to increment (increase) or decrement (decrease) the value of a variable by 1. They come in two forms:
- Pre-increment (
++x
): Increments the value and then returns the new value (e.g.,let count = 5; count++
evaluates to 5 but increments count to 6) - Post-increment (
x++
): Returns the current value and then increments (e.g.,let count = 5; let oldCount = count++
assigns 5 to oldCount and then increments count to 6)
Decrement operators (--x
and x--
) work similarly.
10. Comma Operator
This operator allows you to group multiple expressions into a single statement. However, only the rightmost expression’s value is returned. It’s often used for side effects (actions that don’t necessarily return a value). For example:
let x = 10, y = 20;
console.log(x, y++); // This logs 10 20 (y is incremented after the log)
11. Special Operators
JavaScript offers a few special operators for specific functionalities:
delete
: Deletes a property from an objectvoid
: Evaluates an expression but discards the result (often used for side effects)in
: Checks if a property exists in an object
Let’s Take a Look at Some Examples
Now that you’ve explored the different types of operators, let’s delve into some practical examples to solidify your understanding:
Example 1: Using Arithmetic Operators
let radius = 10;
let area = Math.PI * radius * radius; // Calculates the area of a circle
console.log("Area of the circle:", area);
Example 2: Utilizing Comparison Operators with Logical Operators
let grade = 85;
let hasAttendedAllClasses = true;
let isEligibleForHonors = grade >= 90 && hasAttendedAllClasses;
console.log("Eligible for honors:", isEligibleForHonors);
Example 3: Conditional (Ternary) Operator in Action
let age = 16;
let accessMessage = (age >= 18) ? "Granted access." : "Access denied.";
console.log(accessMessage);
Example 4: String Concatenation with the Plus Operator
let firstName = "John";
let lastName = "Doe";
let fullName = firstName + " " + lastName;
console.log("Full Name:", fullName);
Example 5: Incrementing a Counter Variable
let count = 0;
count++; // Pre-increment, logs 0 but increments to 1
console.log(count);
let visits = count++; // Post-increment, assigns 0 to visits and then increments to 1
console.log(visits); // This logs 1
Advanced Operator Usage
As you become more proficient in JavaScript, you can explore advanced operator usage scenarios:
- Operator Precedence: Understand the order in which operators are evaluated within an expression. Use parentheses to control the order if needed.
- Type Coercion: Be aware of how JavaScript implicitly converts values from one type to another during operations. This can sometimes lead to unexpected results.
- Bitwise Operators for Complex Manipulations: While less common, bitwise operators can be powerful for low-level bit manipulation tasks.
Conclusion
By mastering JavaScript operators, you unlock the true potential of the language. From performing calculations to manipulating data and controlling program flow, operators are the foundation upon which all JavaScript programs are built. This comprehensive guide has equipped you with the knowledge of various operators, their functionalities, and practical examples. Remember, consistent practice and exploration are key to solidifying your understanding.
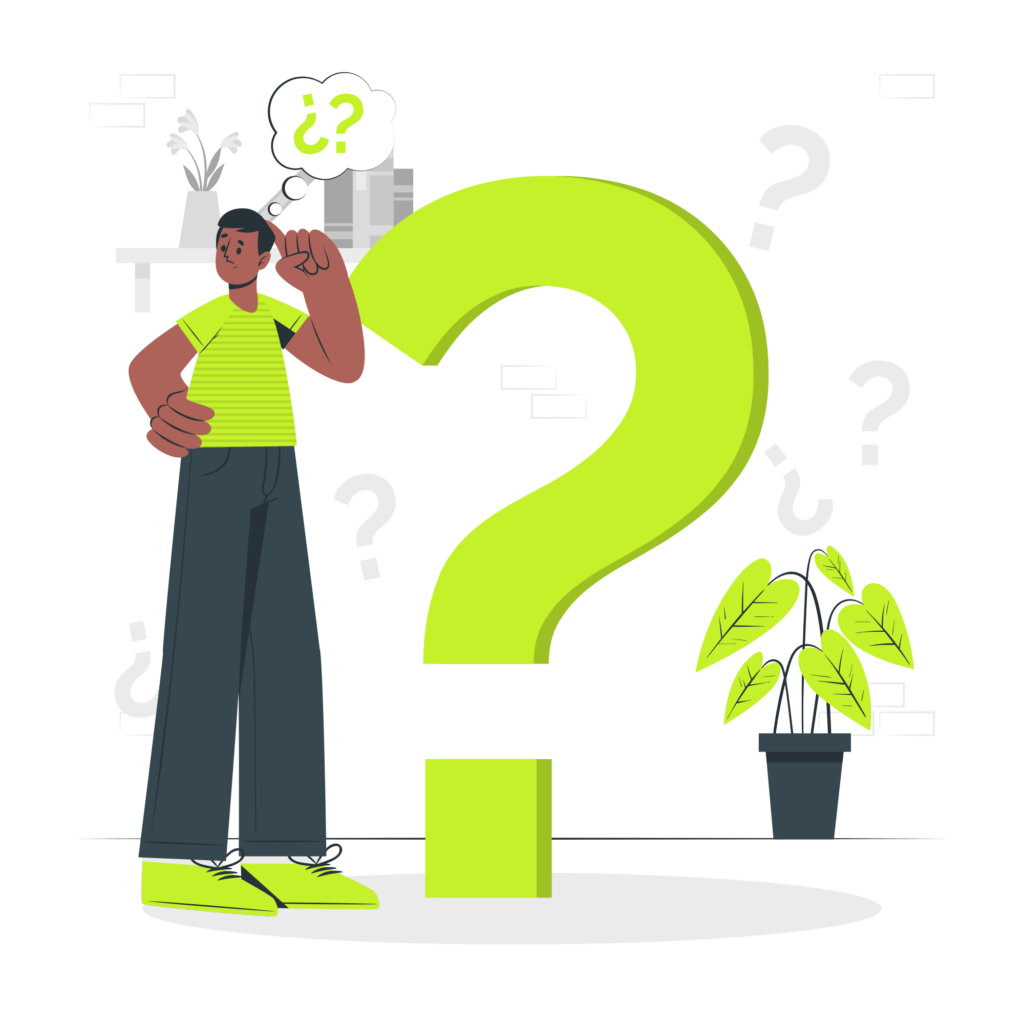
FAQs
What are JavaScript operators?
- JavaScript operators are symbols or keywords used to perform operations on variables and values, like addition, comparison, or logical operations.
How do JavaScript arithmetic operators work?
- Arithmetic operators in JavaScript perform basic mathematical operations like addition (+), subtraction (-), multiplication (*), and division (/), among others.
What is the purpose of comparison operators in JavaScript?
- Comparison operators compare two values and return a boolean value (true or false) depending on the outcome of the comparison, such as equal to (==) or greater than (>).
What are logical operators in JavaScript?
- Logical operators are used to determine the logic between variables or values, including AND (&&), OR (||), and NOT (!), helping in decision-making in code.
How do assignment operators function in JavaScript?
- Assignment operators assign values to JavaScript variables. The basic assignment operator is =, but there are others like += and -= that combine assignment with arithmetic operations.
What is JavaScript used for in web development?
- JavaScript is primarily used to create dynamic and interactive web content, enabling functionalities like form validation, animations, and event handling on web pages.
How can I start learning JavaScript?
- Start with the basics of syntax and operators, practice simple programs, and progressively move to more complex topics. Online tutorials, courses, and coding communities can be great resources.
What are the benefits of understanding JavaScript operators?
- Knowing JavaScript operators is crucial for writing efficient and effective code, enabling developers to implement complex logic and algorithms in web development.
How does JavaScript interact with HTML and CSS?
- JavaScript interacts with HTML and CSS to manipulate web page content and styles dynamically, allowing for interactive and responsive design.
Are JavaScript operators similar to operators in other programming languages?
- Many JavaScript operators are similar to those in other languages, but it’s important to understand the nuances and specific behaviors in JavaScript’s context.