Solidity, the programming language for Ethereum smart contracts, excels at automating tasks and enforcing complex logic within the secure confines of the blockchain. However, smart contracts by themselves operate in a closed environment, unable to directly access external data sources. This limitation hinders their ability to interact with the real world and execute actions based on events beyond the blockchain. Here’s where oracles in solidity bridge the gap.
What are Oracles?
Oracles act as trusted intermediaries, fetching data from the external world (APIs, web servers, sensors) and delivering it securely to smart contracts. They essentially translate real-world information into a format interpretable by the blockchain, enabling smart contracts to react to and leverage real-world events.
Why Use Oracles in Solidity?
Solidity smart contracts find numerous applications when empowered by oracles. Here are some key use cases:
- Decentralized Finance (DeFi): Price feeds from oracles are crucial for DeFi applications like lending protocols and automated market makers (AMMs) to determine fair collateralization levels and accurate asset pricing.
- Supply Chain Management: Oracles can track the movement of goods throughout the supply chain by integrating with sensors and logistics APIs, ensuring transparency and immutability of data.
- Insurance: Real-time weather data from oracles can be used by parametric insurance policies to automatically trigger payouts in the event of natural disasters.
- Prediction Markets: Oracles can deliver event outcomes, like sports game results or election winners, enabling the settlement of prediction markets in a fair and verifiable manner.
- Random Number Generation (RNG): Blockchain by itself is deterministic, making true randomness generation a challenge. Oracles can provide unpredictable, verifiable random numbers vital for games, lotteries, and other applications relying on chance.
Implementing Oracles in Solidity
While Solidity itself doesn’t have built-in oracle functionality, integrating them into your smart contracts involves a few key steps:
01. Choosing an Oracle Network
Several decentralized oracle networks (DONs) exist, each with its own strengths and weaknesses. Popular choices include:
- Chainlink: A widely used, decentralized oracle network with a robust reputation system and various data providers.
- Band Protocol: Offers high throughput and scalability, ideal for fast-paced applications.
- Umami: Focuses on data privacy and utilizes a secure multi-party computation (MPC) approach.
Consider factors like reputation, security, data types supported, and cost when selecting an oracle network.
02. Understanding Oracle Request Workflow:
The interaction between a smart contract and an oracle network typically follows this workflow:
- Request: The smart contract initiates a request for data, specifying the desired information and potentially offering a bounty for fulfilling the request.
// Example function to request a price for ETH/USD from Chainlink
function requestEthPrice() public {
// Replace with your actual Chainlink Aggregator contract address
AggregatorV3Interface priceFeed = AggregatorV3Interface(0x8A753707A3e4DdCdB6fcbAC442c1A13845EBCD6A);
// Request latest ETH/USD price
priceFeed.requestPriceData();
}
- Fulfillment: Nodes in the oracle network retrieve the data from the external source and submit it along with cryptographic proof verifying its authenticity.
- Callback: The smart contract receives the data and proof, validates them using the oracle network’s verification mechanism, and then utilizes the data as programmed.
// Example callback function to receive and process the price data
function fulfillPriceRequest(
uint256 requestId,
uint256 price,
uint256 startedAt,
uint256 updatedAt,
uint8 v,
bytes32 r,
bytes32 s
) public {
// Verify the data and proofs using Chainlink's verification mechanism
require(msg.sender == address(priceFeed), "Only oracle can fulfill request");
// Use the retrieved price data in your smart contract logic
// ...
}
03. Solidity Integration with Oracle Network:
Most oracle networks provide Software Development Kits (SDKs) with libraries and tools for interaction with smart contracts. These SDKs often include:
- Chainlink Aggregator Contract: Facilitates access to price feeds on the Chainlink network.
- Off-chain Consumer: A separate program running off-chain that interacts with the oracle network and relays data back to the smart contract.
Example Oracle Implementation in Solidity
This example demonstrates a basic implementation using Chainlink to retrieve the latest ETH/USD price and store it in a state variable within your Solidity smart contract.
Setup:
- Ensure you have a development environment set up for Solidity smart contract development (e.g., Remix IDE, Truffle).
- Install the necessary Chainlink libraries for your chosen environment.
- Replace
<YourOracleAddress>
with the actual address of the Chainlink Aggregator contract for ETH/USD on the network you’re deploying to (e.g., Ethereum mainnet, testnet).
Code Example:
pragma solidity ^0.8.0;
// Import Chainlink AggregatorV3Interface
import "@chainlink/contracts/src/v0.8/interfaces/AggregatorV3Interface.sol";
contract EthPriceConsumer {
// State variable to store retrieved ETH/USD price
uint256 public ethPrice;
// Chainlink Aggregator address for ETH/USD price feed
AggregatorV3Interface private priceFeed;
constructor(address _oracleAddress) public {
priceFeed = AggregatorV3Interface(_oracleAddress);
}
// Function to request latest ETH/USD price
function requestEthPrice() public {
priceFeed.requestPriceData();
}
// Callback function to receive and process price data (triggered by Chainlink)
function fulfillPriceRequest(
uint256 requestId, // Unused for this example
uint256 price,
uint256 startedAt,
uint256 updatedAt,
uint8 v,
bytes32 r,
bytes32 s
) public {
require(msg.sender == address(priceFeed), "Only oracle can fulfill request");
ethPrice = price;
}
// Function to access the retrieved ETH/USD price (for informational purposes)
function getEthPrice() public view returns (uint256) {
return ethPrice;
}
}
What the above code does:
- We import the
AggregatorV3Interface
from the Chainlink library. - The
EthPriceConsumer
contract is defined with a state variableethPrice
to store the retrieved price. - The constructor takes the address of the Chainlink Aggregator contract for ETH/USD as an argument.
- The
requestEthPrice
function initiates a request for the latest price data using therequestPriceData
function of thepriceFeed
contract. - The
fulfillPriceRequest
function acts as the callback function. It verifies the sender (should be the Chainlink oracle) and then updates theethPrice
state variable with the retrieved price. - The
getEthPrice
function allows retrieving the storedethPrice
for informational purposes within your smart contract.
Note: This is a simplified example for demonstration purposes. In a real-world scenario, you might implement additional functionalities like error handling and security checks. Remember to deploy the Chainlink Aggregator contract and your smart contract to the desired network.
Integrating the SDK involves:
- Importing necessary libraries: Use the provided libraries from the oracle network’s SDK.
- Defining data request parameters: Specify the data you need and any additional requirements.
- Initiating the request: Trigger a function within your smart contract to send a request to the oracle network.
- Handling the callback: Implement a function within your smart contract to receive and validate the data returned by the oracle network.
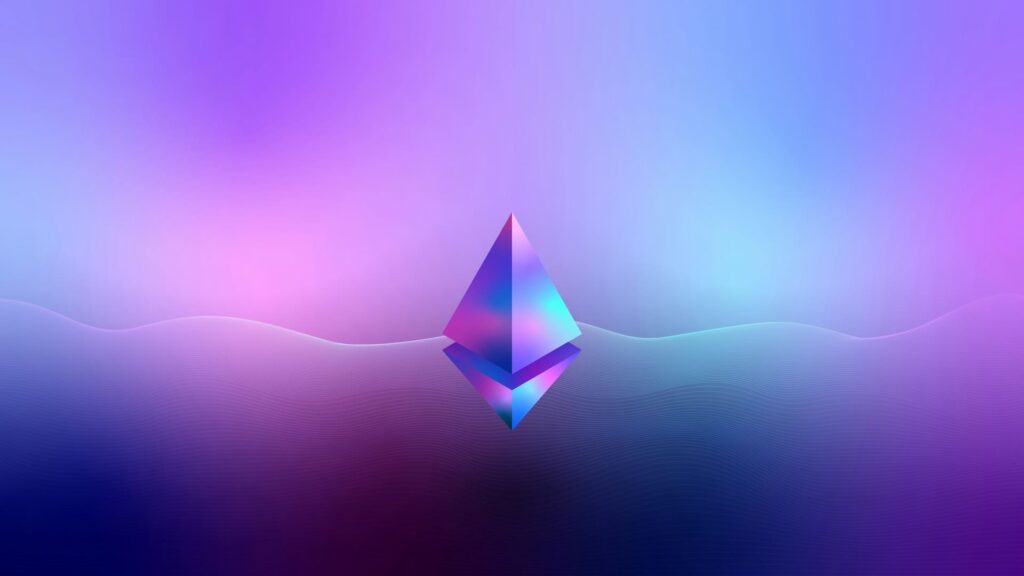
Security Considerations
Integrating oracles introduces additional security considerations for your Solidity smart contracts. Here are some key points to remember:
- Oracle Network Reputation: Choose a reputable and well-established oracle network to minimize the risk of malicious actors manipulating data feeds.
- Data Verification: Always implement robust mechanisms within your smart contract to validate the data received from the oracle network using the provided proofs.
- Off-chain Consumer Security: Securely manage the off-chain consumer program responsible for interacting with the oracle network. Mitigate potential vulnerabilities that could allow attackers to inject tampered data.
- Centralization Risks: While decentralized oracle networks aim to eliminate a single point of failure, some level of centralization might still exist within the network. Be aware of these potential risks and choose an oracle network with a strong decentralization philosophy.
Best Practices for Implementing Oracles
- Minimize Trust Assumptions: Design your smart contract to minimize trust assumptions on any single entity, including the oracle network itself. Implement multiple oracles for critical data points to enhance data reliability.
- Clear Requirements: Clearly define the data you require and the acceptable tolerance for potential delays or inconsistencies.
- Error Handling: Implement robust error handling mechanisms within your smart contract to gracefully handle situations like oracle network failures or invalid data returned.
- Gas Optimization: Be mindful of gas costs associated with oracle requests and data storage on the blockchain. Consider using techniques like off-chain computation for complex data manipulation to minimize on-chain costs.
- Regular Updates: Stay updated with the latest developments and security best practices within the oracle network you choose. Regularly review your smart contract code and update it as needed.
Advanced Topics in Oracle Integration
For more complex use cases, Solidity offers additional functionalities to enhance your interaction with oracles:
- Keepers: Automated background processes that can trigger specific smart contract functions based on pre-defined conditions. Keepers can be used to initiate oracle requests at regular intervals or when specific events occur on-chain.
- Decentralized Autonomous Services (DAOs): Use DAOs to govern oracle networks and manage data requests. This can be particularly useful for scenarios where community-based decisions are required for data validation or dispute resolution.
Real-World Examples of Oracles in Action
Here are some real-world examples showcasing the power of oracles in Solidity smart contracts:
- Augur: A decentralized prediction market platform that leverages Chainlink oracles to fetch event outcomes and settle prediction markets in a trustless manner.
- MakerDAO: A leading DeFi lending protocol that utilizes price feeds from oracles to determine collateralization ratios and maintain the stability of its Dai stablecoin.
- MarineChain: A blockchain platform for the maritime industry that integrates oracles with real-time vessel tracking data, enabling transparency and efficiency in global shipping operations.
Conclusion
Oracles play a critical role in unlocking the full potential of Solidity smart contracts. By enabling access to real-world data, they empower smart contracts to interact with the external world, opening doors to innovative and impactful decentralized applications. However, implementing oracles securely and efficiently requires careful consideration of security practices, best practices, and available tools. As the blockchain ecosystem continues to evolve, oracle technology will undoubtedly play a vital role in shaping the future of decentralized applications.
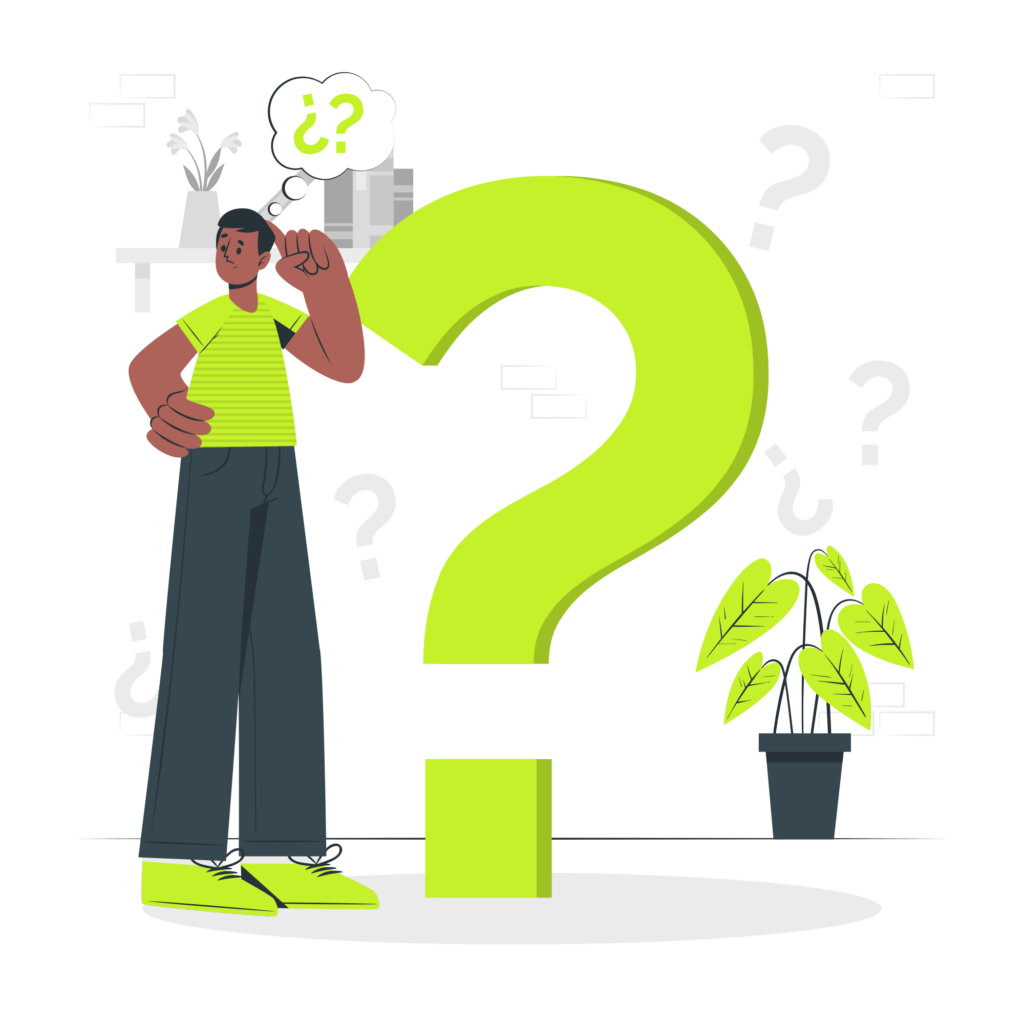
FAQs
What are oracles in the context of Solidity and smart contracts?
- Oracles in Solidity are external data feed providers that enable smart contracts to interact with data outside the blockchain.
How do you implement an oracle in Solidity?
- Implementing an oracle in Solidity involves creating a contract that communicates with an external service to fetch and process off-chain data.
Why are oracles important for blockchain applications?
- Oracles broaden the scope of smart contracts by allowing them to execute based on real-world events and data, making them more versatile and applicable.
What are some common use cases for oracles in blockchain?
- Oracles are used in finance for price feeds, in insurance for triggering claims, and in gaming for random number generation, among others.
How can you ensure the reliability of data from oracles in Solidity?
- Ensuring data reliability involves using multiple oracles, choosing reputable data sources, and implementing consensus mechanisms.
What is Solidity?
- Solidity is a programming language specifically designed for developing smart contracts on the Ethereum blockchain.
What are smart contracts?
- Smart contracts are self-executing contracts with the terms of the agreement directly written into lines of code, running on the blockchain.
How does blockchain technology work?
- Blockchain is a distributed database that maintains a continuously growing list of records, called blocks, secured from tampering and revision.
What are decentralized applications (dApps)?
- dApps are digital applications that run on a blockchain or peer-to-peer network of computers, outside the purview of a single authority.
What is Ethereum?
- Ethereum is a decentralized, open-source blockchain system that features smart contract functionality, widely used for building dApps.