TL;DR:
- Solidity is essential for building robust NFT marketplaces on the Ethereum blockchain.
- Key marketplace features include NFT listing, buying/selling, user management, security measures, and search functionalities.
- Developers should utilize the ERC-721 standard for NFT creation and management.
- Beyond Solidity, a full-stack approach involves front-end development with frameworks like React and back-end services for user authentication.
- Continuous development, security audits, and community engagement are vital for long-term marketplace success.
Solidity, the programming language specifically designed for Ethereum Virtual Machine (EVM), plays a pivotal role in building robust NFT marketplaces. This article explains, NFT marketplace development using Solidity step-by-step, giving you the knowledge to navigate this exciting field.
Core Functionalities of an NFT Marketplace
Before diving into the code, it’s crucial to grasp the fundamental functionalities an NFT marketplace should offer. Here’s a breakdown of the key features:
- NFT Listing and Management: Users (typically creators or owners) should be able to list their NFTs for sale on the marketplace. This involves specifying details like price, duration, and potentially a description. The marketplace facilitates the secure storage of these listing details.
- NFT Buying and Selling: Buyers can browse listed NFTs, filter based on various criteria, and seamlessly purchase them using their cryptocurrency wallets. The marketplace executes the transaction securely, transferring ownership of the NFT to the buyer and facilitating payment to the seller.
- User Management: The platform needs a user management system to differentiate between buyers, sellers, and potentially administrators. This can involve user registration, login functionality, and role-based access control.
- Security and Escrow: Security is paramount. The marketplace should leverage the inherent security of blockchain technology to ensure the safe transfer of NFTs and prevent fraud. Escrow functionalities can further enhance security by holding the NFT and funds until the transaction is complete.
- Marketplace Fees: Most marketplaces charge a commission fee on each transaction. The smart contract should handle the calculation and collection of these fees.
- Search and Discovery: A user-friendly search and discovery mechanism is essential for a thriving marketplace. This allows buyers to easily find NFTs that interest them, potentially based on filters like category, creator, or price range.
The Foundation in Solidity for an NFT Marketplace
Solidity is your tool for crafting the smart contracts that power the NFT marketplace. These contracts are self-executing programs deployed on the blockchain that govern the functionalities mentioned above.
Here’s a breakdown of the key steps involved in building the smart contract with Solidity:
- Setting Up the Environment:
- Install Node.js and a code editor like Visual Studio Code.
- Utilize tools like Hardhat or Truffle for development, testing, and deployment of the smart contract.
- Connect to a local blockchain instance (e.g., Ganache) for development and testing purposes.
- Defining Smart Contract Components:
- ERC-721 Standard: Leverage the ERC-721 standard, the foundational standard for NFTs on Ethereum. This standard defines core functionalities like ownership transfer, safe transfers, and approval mechanisms.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
contract MyNFTMarketplace is ERC721 {
// ... (other contract code)
}
This code snippet imports the ERC721 standard from OpenZeppelin. By inheriting from this standard, your marketplace contract automatically gains functionalities like ownership tracking, safe transfers, and approval mechanisms for NFTs.
- NFT Listing Structure: Create a data structure within the smart contract to store information about listed NFTs. This can include details like token ID, owner address, asking price, and potentially additional metadata.
struct Listing {
address seller;
uint256 tokenId;
uint256 price;
bool isListed;
}
mapping(uint256 => Listing) public listings;
This code defines a Listing structure to store information about listed NFTs. It includes details like the seller’s address, the NFT’s token ID, the asking price, and a boolean flag indicating if the NFT is currently listed for sale. A mappings data structure associates these listings with their corresponding NFT token IDs for easy retrieval.
- User Roles: Implement functionalities to differentiate between user roles (buyer, seller, admin) and control access to specific actions within the marketplace.
modifier onlyOwner() {
require(msg.sender == owner, "Only owner can perform this action");
_;
}
address public owner;
constructor() {
owner = msg.sender;
}
This simplified example demonstrates a basic access control mechanism using a modifier. The onlyOwner modifier restricts specific functions to the contract owner (set during deployment). You can further expand this to include roles like buyer and seller with specific permissions.
- Developing Core Functionalities:
- Listing and Delisting NFTs: Develop functions that allow sellers to list their NFTs with specified details and delist them when no longer available.
function listNFT(uint256 tokenId, uint256 price) public {
// Require checks for ownership and approval (if applicable)
listings[tokenId] = Listing(msg.sender, tokenId, price, true);
}
function delistNFT(uint256 tokenId) public {
// Require checks for ownership
listings[tokenId].isListed = false;
}
- The
listNFT
function allows sellers to list their NFTs with a specified price. Remember to include checks to ensure the seller owns the NFT and has properly approved the marketplace contract to transfer it (if applicable). The delistNFT function enables sellers to remove their NFTs from the marketplace.- Buying and Selling NFTs: Create secure functions for buyers to purchase listed NFTs using their wallets and for sellers to receive payment upon successful transactions.
function buyNFT(uint256 tokenId) public payable {
Listing memory listing = listings[tokenId];
require(listing.isListed, "NFT not listed");
require(msg.value >= listing.price, "Insufficient funds");
// Transfer NFT and funds (consider using payable address)
listings[tokenId].isListed = false;
}
- This simplified buyNFT function allows buyers to purchase listed NFTs. It retrieves the listing details, checks if the NFT is listed and if the buyer has sent enough funds. Upon successful purchase, the NFT is transferred to the buyer, and the seller receives the payment. Remember, real-world implementations will need to handle fee management and secure transfer of funds.
- Fee Management: Implement logic to calculate and collect marketplace fees on each transaction.
- Search and Filter: While the core functionalities reside within the smart contract, consider additional off-chain mechanisms (potentially using libraries like IPFS) to facilitate efficient search and filter functionalities for a user-friendly experience.
- Fee Management: Implement logic to calculate and collect marketplace fees on each transaction.
- Security Considerations:
- Thorough Testing: Rigorously test your smart contract using unit tests and integration tests to identify and eliminate vulnerabilities before deployment.
- Security Audits: Consider engaging blockchain security experts to conduct audits and identify potential security flaws in your smart contract code.
- Deployment and Interaction:
- Deploy your smart contract to your chosen blockchain network (e.g., Ethereum mainnet, a testnet).
- Develop a user interface (potentially using frameworks like React or Vue.js) that interacts with the deployed smart contract to provide a user-friendly experience for listing, buying, and managing NFTs within the marketplace.
NFT Marketplace Development
01. Setting Up ERC-721 Compliance (Basic Structure):
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/utils/Counters.sol";
contract MyNFT is ERC721 {
using Counters for Counters.Counter;
Counters.Counter private _tokenIdCounter;
constructor() ERC721("MyNFT", "MNFT") {}
function mintNFT() public {
uint256 tokenId = _tokenIdCounter.current();
_tokenIdCounter.increment();
_safeMint(msg.sender, tokenId);
}
}
This code snippet demonstrates a basic ERC-721 compliant NFT contract. It leverages OpenZeppelin libraries for functionalities like token ID generation, minting, and ownership management.
02. Creating an NFT Marketplace Contract (Simplified Example):
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "./MyNFT.sol";
contract NFTMarketplace {
// Structure to store listing details
struct Listing {
address seller;
uint256 tokenId;
uint256 price;
bool isListed;
}
// Mapping to store listings by NFT token ID
mapping(uint256 => Listing) public listings;
MyNFT public nftContract; // Instance of the NFT contract
constructor(address nftContractAddress) {
nftContract = MyNFT(nftContractAddress);
}
// Function to list an NFT for sale
function listNFT(uint256 tokenId, uint256 price) public {
require(nftContract.ownerOf(tokenId) == msg.sender, "Only owner can list");
listings[tokenId] = Listing(msg.sender, tokenId, price, true);
}
// Function to buy a listed NFT (simplified, excludes fee handling)
function buyNFT(uint256 tokenId) public payable {
Listing memory listing = listings[tokenId];
require(listing.isListed, "NFT not listed");
require(msg.value >= listing.price, "Insufficient funds");
nftContract.transferFrom(listing.seller, msg.sender, tokenId);
listings[tokenId].isListed = false;
// Pay seller (consider using payable address)
payable(listing.seller).transfer(msg.value);
}
}
This simplified example showcases a basic marketplace contract. It defines a data structure for storing listing details and utilizes mappings to associate them with NFT token IDs. Functions allow sellers to list their NFTs and buyers to purchase them, ensuring ownership transfer through the transferFrom function of the underlying ERC-721 contract.
- Additional Considerations:
- Remember, these are simplified examples. Real-world marketplaces will include functionalities like fee management, user roles, and security checks.
- Explore OpenZeppelin libraries for functionalities like Ownable (restricting specific functions to contract owner) and ReentrancyGuard (preventing reentrancy attacks).
- Consider using tools like Hardhat for testing and deployment of your smart contracts.
Advanced Features and Considerations for NFT Marketplaces
Building a robust NFT marketplace goes beyond the core functionalities. Here’s a look at some advanced features and considerations that can elevate your platform:
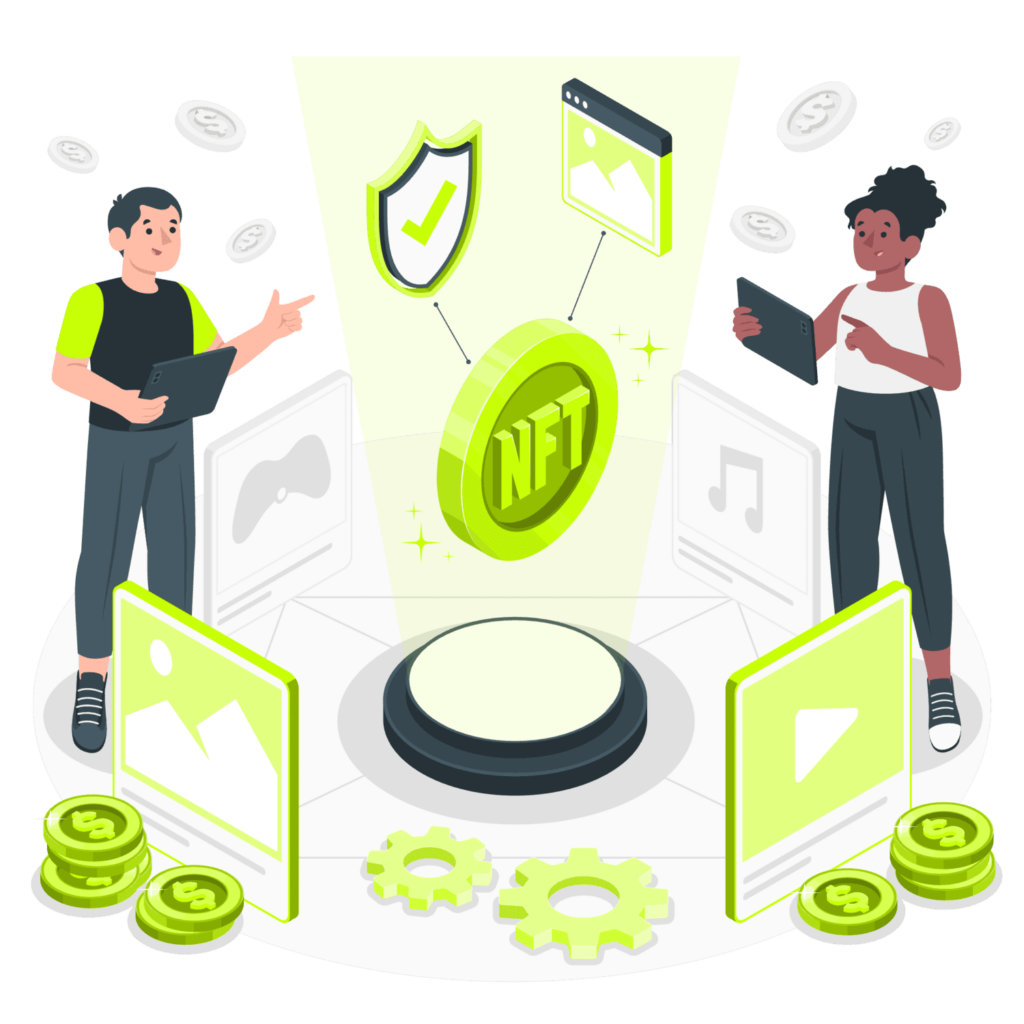
Enhanced User Experience:
- Auction Functionality: Implement an auction system where buyers can bid on NFTs, creating a dynamic pricing mechanism and potentially higher selling prices for creators.
- Offers and Counteroffers: Allow buyers to make offers on listed NFTs, enabling negotiation between buyers and sellers.
- Escrow Services: Integrate an escrow service within the smart contract to hold the NFT and funds until the transaction is complete, adding an extra layer of security for both parties.
- Royalty Management: Incorporate royalty structures into the smart contract, enabling creators to earn a percentage of future sales of their NFTs, even after the initial sale.
Advanced Functionality:
- Multi-Token Support: Expand beyond just Ethereum by allowing users to buy and sell NFTs using different cryptocurrencies.
- NFT Fractionalization: Enable the division of NFT ownership into smaller fractions, allowing for broader participation in ownership of high-value NFTs.
- Governance Mechanisms: Consider implementing a governance system where marketplace users can participate in decision-making processes related to fees, platform updates, and future features.
Integration and Interoperability:
- Off-Chain Storage: Utilize InterPlanetary File System (IPFS) or similar decentralized storage solutions to store large asset files (e.g., images, videos) associated with NFTs, while keeping core ownership and transfer logic on-chain for security.
- Marketplace Aggregators: Integrate with NFT marketplace aggregators that allow users to browse listings across multiple marketplaces, increasing the discoverability of NFTs on your platform.
Security and Scalability:
- Security Audits: Regularly conduct security audits by reputable blockchain security firms to identify and address potential vulnerabilities in your smart contracts.
- Layer-2 Scaling Solutions: Explore Layer-2 scaling solutions like Polygon or Immutable X to improve transaction speed and reduce gas fees, especially if you anticipate high transaction volume on your marketplace.
Additional Considerations:
- Compliance: Stay up-to-date with evolving regulations surrounding NFTs and cryptocurrencies to ensure your marketplace operates compliantly.
- Community Building: Foster a strong community around your marketplace by engaging with users, hosting events, and offering unique features that cater to their interests.
- Marketing and User Acquisition: Develop a robust marketing strategy to attract creators, collectors, and enthusiasts to your platform. Analyze user behavior and preferences to tailor your marketing efforts for maximum impact.
Beyond Solidity: The Full Stack Picture
While Solidity is crucial for building the smart contract foundation, a complete NFT marketplace requires a full-stack development approach. Here’s what you’ll need beyond Solidity:
- Front-end Development: Develop a user-friendly interface (UI) using frameworks like React or Vue.js that interacts with the deployed smart contract. This UI will allow users to browse listings, manage their accounts, and execute buying and selling actions.
- Back-end Development: Consider building a server-side component using languages like Node.js or Python to handle functionalities like user authentication, data storage for off-chain information, and communication with the deployed smart contract.
Conclusion
Developing a successful NFT marketplace requires a blend of technical expertise, a deep understanding of the NFT ecosystem, and a focus on user experience. By manioulating Solidity to craft secure and functional smart contracts, and incorporating the advanced features and considerations mentioned above, you can create a platform that caters to the evolving needs of the NFT community. Remember, continuous development, security audits, and a focus on community building are all crucial aspects for ensuring the long-term success of your NFT marketplace.
FAQs
What is NFT Marketplace Development with Solidity?
- NFT Marketplace Development with Solidity involves creating a blockchain-based platform for buying, selling, and trading non-fungible tokens (NFTs) using Solidity programming language.
How does Solidity enhance NFT Marketplace Development?
- Solidity, being the primary language for Ethereum smart contracts, offers robust and secure programming capabilities, making it ideal for developing reliable NFT marketplaces.
What are the benefits of creating an NFT Marketplace?
- An NFT marketplace allows creators to monetize their digital assets, provides a platform for unique collectibles trading, and offers transparency and security through blockchain technology.
What are smart contracts in the context of NFTs?
- Smart contracts are self-executing contracts with the terms directly written into code, enabling automatic and secure transactions in NFT marketplaces without intermediaries.
Can beginners learn to develop an NFT Marketplace using Solidity?
- Yes, beginners can learn to develop an NFT marketplace using Solidity, though it requires a foundational understanding of blockchain technology and programming.
What is Solidity?
- Solidity is a high-level, contract-oriented programming language used for implementing smart contracts on various blockchain platforms, predominantly Ethereum.
What is an NFT?
- A Non-Fungible Token (NFT) is a unique digital asset verified using blockchain technology, representing ownership of a specific item or piece of content.
How does blockchain technology support NFT marketplaces?
- Blockchain technology provides a decentralized, secure, and transparent platform, ensuring the authenticity and ownership of digital assets in NFT marketplaces.
What are the key components of an NFT marketplace?
- Key components include user interface, wallet integration, smart contracts for transaction processing, and a system for listing and categorizing NFTs.
What is the future of NFT Marketplace Development?
- The future looks promising with innovations in interoperability, enhanced security, and broader adoption across various industries, expanding the utility and value of NFTs.