TL;DR
- JavaScript has 7 main data types, Number, String, Boolean, Undefined, Null, Symbol, and BigInt.
- Data types are categorized into primitive (immutable) and reference (mutable) types.
- Type coercion allows JavaScript to automatically convert between different data types during operations.
- Understanding data types is important for writing efficient, bug-free code in JavaScript.
JavaScript is one of the most popular programming languages in the world today. It plays an important role in modern software development. One of the foundational concepts every developer must learn when working with JavaScript is understanding its data types.
In this article, we’ll explore the various JavaScript data types, what each type is, how to use them, and common mistakes to avoid. Whether you’re a beginner just getting started with JavaScript or an experienced developer looking to refresh your knowledge, this article will give you a thorough understanding of JavaScript data types.
What Are JavaScript Data Types?
In JavaScript, data types define the kind of values that variables can hold, helping developers structure and manage data effectively. Understanding these types is important, as it enables you to store, manipulate, and display information in your applications correctly.
JavaScript features a dynamic type system, meaning that a variable’s data type is determined at runtime rather than being explicitly declared. This flexibility allows variables to hold different types of data throughout a program’s execution, making JavaScript both powerful and versatile for handling various kinds of information.
JavaScript supports a variety of data types, ranging from simple values like numbers and strings to more complex structures like objects and arrays. These types can be divided into two categories such as primitive types and non-primitive types. Let’s look at these in more detail.
Primitive Data Types in JavaScript
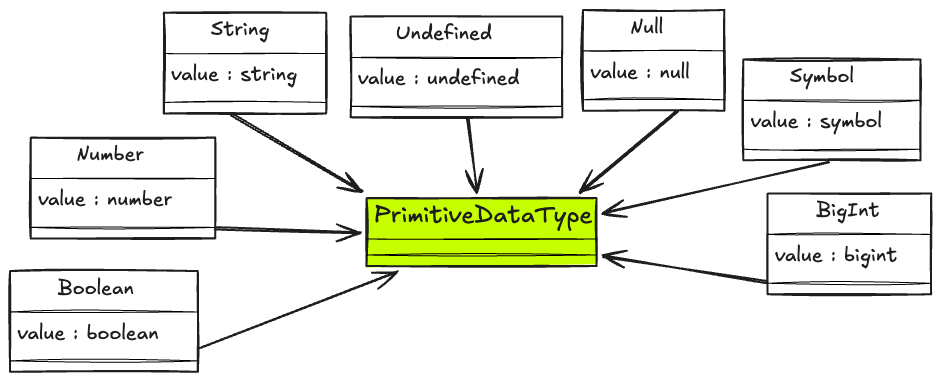
1. Number
The Number data type in JavaScript represents both integer and floating-point numbers. In JavaScript, numbers are always stored as floating-point values. This means that even whole numbers are technically floating-point numbers.
Example:
let age = 25;
let price = 19.99;
JavaScript includes a few special number values that serve unique purposes. Infinity represents positive infinity, while -Infinity represents negative infinity, both of which occur when a number exceeds the upper or lower limit of JavaScript’s number range.
Additionally, NaN (Not-a-Number) signifies an invalid numerical value, typically resulting from an undefined or erroneous mathematical operation, such as dividing zero by zero or attempting to parse a non-numeric string as a number.
2. String
The String data type is used to represent sequences of characters. Strings can be enclosed in single quotes ('
), double quotes ("
), or backticks (“`) for template literals.
Strings are immutable, meaning once a string is created, it cannot be altered. However, you can create new strings based on the old ones.
Example:
let greeting = "Hello, world!";
let name = 'John Doe';
let message = `Hello, ${name}!`;
3. Boolean
The Boolean data type has only two possible values, true or false. Booleans are commonly used for control flow in JavaScript, particularly in conditionals like if
statements and loops.
Example:
let isActive = true;
let hasPermission = false;
4. Undefined
A variable that has been declared but not assigned a value is automatically assigned the undefined value. It is also the default value for function arguments that are not provided.
Example:
let name;
console.log(name); // Output: undefined
5. Null
The null data type is used to represent the intentional absence of any object value. It is often used to indicate that a variable is empty or should be explicitly cleared.
Example:
let user = null
6. Symbol
Introduced in ECMAScript 6, the Symbol data type is used to create unique, immutable identifiers. Symbols are mainly used in object properties to avoid name collisions.
Example:
let id = Symbol('id');
let user = {
[id]: 'user123'
};
console.log(user[id]); // Output: 'user123'
7. BigInt
BigInt is a relatively new data type that allows for working with arbitrarily large integers. Unlike the Number type, which has a limit on the size of the number, BigInt can represent integers of arbitrary precision.
Example:
let bigNumber = 1234567890123456789012345678901234567890n;
Non-Primitive Data Types in JavaScript
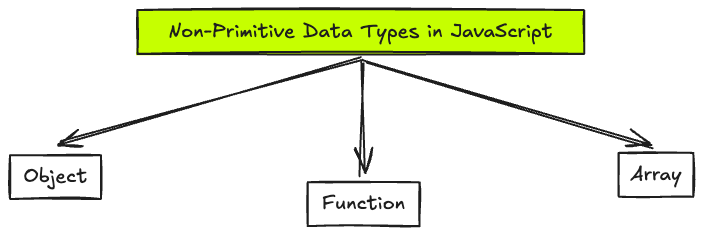
1. Object
The Object data type is one of the most powerful features of JavaScript. Objects are collections of key-value pairs, where the key is usually a string or symbol, and the value can be any data type, including other objects.
Example:
let person = {
name: 'John',
age: 30,
isEmployed: true
};
2. Array
An Array is a special type of object used to store ordered collections of data. Arrays in JavaScript can hold values of any type, and they are indexed by numbers. They come with many built-in methods like push()
, pop()
, shift()
, and unshift()
to manipulate the contents.
Example:
let fruits = ['Apple', 'Banana', 'Cherry'];
3. Function
In JavaScript, functions are first-class objects. This means that functions are treated as objects, and they can be assigned to variables, passed as arguments to other functions, and returned from other functions.
Example:
function greet(name) {
return `Hello, ${name}!`;
}
let greetUser = greet;
console.log(greetUser('Alice')); // Output: 'Hello, Alice!'
Converting Data Types in JavaScript
JavaScript is a dynamic typed language, meaning you don’t always have to explicitly convert types. JavaScript automatically converts one data type to another when necessary. This is known as type coercion.
For example, when you perform operations between a number and a string, JavaScript will convert the number to a string:
let result = 5 + '5';
console.log(result); // Output: '55'
On the other hand, you can also explicitly convert between types using functions like String()
, Number()
, or Boolean()
:
let num = Number('42'); // Converts string to number
let str = String(42); // Converts number to string
Common Mistakes to Avoid
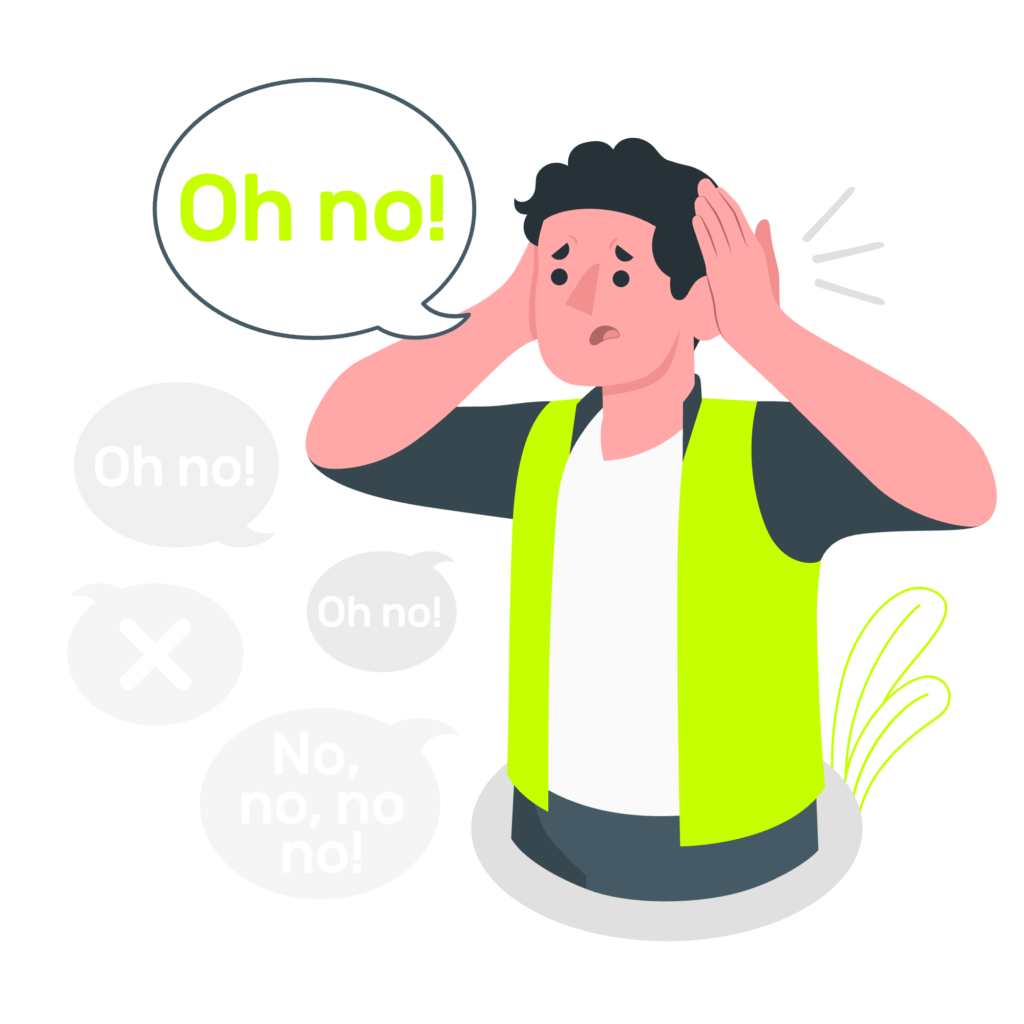
When working with JavaScript data types, certain mistakes can lead to unexpected behavior or bugs in your code. Understanding these common mistakes will help you write more reliable and efficient programs. Following are some key issues to watch out for when handling data types in JavaScript.
1. Confusing ==
with ===
In JavaScript, ==
is used for comparison and performs type coercion, while ===
is the strict equality operator and does not perform type coercion. It’s generally a good practice to use ===
to avoid unexpected results.
console.log(0 == false); // true
console.log(0 === false); // false
2. Misunderstanding null
and undefined
Both null
and undefined
represent the absence of value, but they are different in the context of JavaScript. null
is an object that is explicitly set, while undefined
is the default value for variables that haven’t been assigned a value.
console.log(null == undefined); // true
console.log(null === undefined); // false
3. Using Objects and Arrays Without Understanding Their Behavior
Since objects and arrays are reference types, it’s important to remember that they are assigned by reference, not by value. This means that if you assign one object or array to another, they both refer to the same memory location.
let arr1 = [1, 2, 3];
let arr2 = arr1;
arr2.push(4);
console.log(arr1); // Output: [1, 2, 3, 4]
4. Avoiding Implicit Type Coercion
Sometimes, JavaScript will coerce values to different types behind the scenes. While this can be convenient, it can also lead to unexpected results. Be aware of how type coercion works, especially with operators like +
, ==
, and &&
.
Conclusion
Understanding JavaScript data types is fundamental for any developer working with this language. Whether dealing with primitive types or more complex reference types like objects and arrays, each type plays an important role in how you store, manipulate, and process data in your programs.
By learning these data types and recognizing their unique behaviors, you’ll be able to write cleaner, more efficient, and bug-free code. As you continue your JavaScript journey, always keep in mind how different data types interact, as this knowledge will greatly enhance your ability to solve complex programming challenges.
FAQs
What is the difference between null
and undefined
?
- In JavaScript, null is an object that represents the intentional absence of any value, meaning a variable has been explicitly set to have no value. On the other hand, undefined is the default value assigned to variables that have been declared but not yet initialized. While both indicate the absence of a meaningful value, they serve different purposes and should not be used interchangeably.
How do you check the data type of a variable in JavaScript?
- You can use the
typeof
operator to check the data type of a variable.
What are reference types in JavaScript?
- Reference types in JavaScript include objects, arrays, and functions. These types store references to memory locations rather than the actual data, meaning changes to one variable will affect others if they share the same reference.
Can I change the data type of a variable in JavaScript?
- Yes, JavaScript is a dynamically-typed language, meaning the data type of a variable can be changed during runtime. For example, you can assign a string value to a variable, and later assign a number to the same variable without causing any errors.
Are arrays considered objects in JavaScript?
- Yes, arrays are a type of object in JavaScript. However, arrays come with special behavior and built-in methods that allow you to work with ordered collections of data. Despite this, they are still technically objects and can be manipulated as such.