In modern web development, providing a seamless and interactive user experience is crucial. A common challenge for a developer is handling user interactions without disrupting the user flow. This situation needs serious attention because the user experience is what leads your users to continue navigating on your website.
This article delves deep into the concept of Javascript events , explaining the importance of those concepts and providing real-world examples on how they can be adopted to enhance user experience on your web application. Understanding these concepts will enable you to build responsive and user-friendly web experience for your users.
What are Javascript Events ?
Events are things that happen in your program, which the system tells you about so your code can react to them. They can be triggered by various actions such as clicking a button, submitting a form, or moving the mouse. Understanding how to handle these events effectively is crucial for creating a seamless user experience in your web application.
JavaScript events are fundamental to creating interactive web applications. These events are actions or occurrences that happen in the browser, such as user interactions, browser actions (loading a page, resizing a window), or even script-triggered events. Handling these events allows developers to execute specific code in response, making web pages dynamic and responsive.
element.addEventListener('event type', **function()** {
// code that is executed when the event is triggered
});
The above code snippet demonstrates how to create an event listener in JavaScript. The element
represents the HTML element to which you want to attach the event listener. This element should be selected or created in your HTML document. The event type
is a string representing the type of event to listen for (e.g., ‘click’, ‘mouseover’, ‘keydown’). The function()
is the callback function that will be executed when the event is triggered.
Importance of Events in JavaScript
In the context of web development, an event is an action or occurrence recognized by software that may be handled by the software. JavaScript events are crucial for enhancing the usability and functionality of your web application. They open the door to a lot of opportunities to enhance the user experience. They enable real-time updates, allowing web pages to respond immediately to user input without the need to reload the entire page. This leads to a more seamless and engaging user experience.
For Instance, Imagine a scenario where a user clicks a button and the entire page should reload to reflect that change. This approach can be disruptive, slow, and inefficient. Instead, leveraging JavaScript events can lead to a more fluid and responsive experience.
The Problem with Full Page Reloads
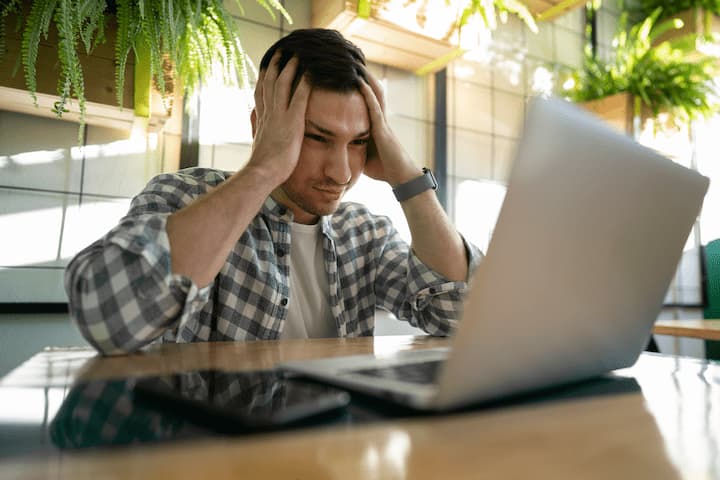
Imagine a user navigating an e-commerce site, adding items to their shopping cart. If every time the “Add to Cart” button is clicked and the page reloads, that would result in several issues that would significantly affect the user experience of your application.
- Flow Interruptions: The user’s flow is constantly interrupted.
- Performance: Full Page reloads could consume a lot of time which would affect the user experience significantly.
- Bandwidth: Full Page reloads could consume a lot of unnecessary bandwidth especially when the page is loaded with heavy content. (Images, videos etc…)
Now let’s enhance this scenario by leveraging JavaScript events. JavaScript events provide a more efficient way to handle user interactions without requiring full page reloads, which can improve the overall performance and user experience of a website. By using the click
event developers can update the web page dynamically.
document.getElementById('addToCart').addEventListener('click', function() {
// function to add product to cart
console.log('Item added to cart!');
});
The above code snippet allows the web application to update the cart items in a shopping cart instead of a full page reload.
Types of Events in JavaScript
Events in JavaScript can be broadly categorized depending on the type of action that triggers them. Following are some of the common event types found in JavaScript.
- Mouse Events:
click
: Triggered when a particular element is clicked.dblclick
: Triggered when an element is double-clicked.mouseover
: Triggered when the mouse pointer moves over an element.mouseout
: Triggered when the mouse pointer moves out of an element.
- Keyboard Events:
keydown
: Triggered when a key is pressed down on the keyboard.keyup
: Triggered when a key is released on the keyboard.
- Form Events
submit
: Triggered when a form is submitted.change
: Triggered when the value changes.focus
: Triggered when an element gains focus.blur
: Triggered when an element loses focus.
- Window Events
load
: Triggered when the whole page has loaded.resize
: Triggered when the browser window is resized.scroll
: Triggered when the user scrolls the page.unload
: Triggered when the document is being unloaded.
- Touch Events
touchstart
: Triggered when a touch point is placed on the touch surface.touchmove
: Triggered when a touch point is moved along the touch surface.touchend
: Triggered when a touch point is removed from the touch surface.
Real World Example
Checkout Form Validation
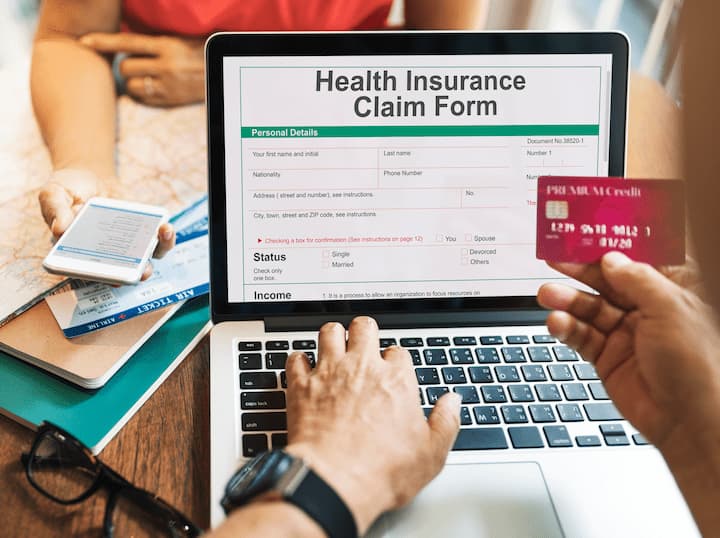
For Example, let’s take a checkout page of an e-commerce website. When a user fills in their shipping information, JavaScript events can be used to validate the input fields in real-time. This not only helps in providing immediate feedback to the user but also ensures that the data entered is correct before submission.
document.getElementById('email').addEventListener('blur', function() {
let email = this.value;
if (!validateEmail(email)) {
// show an alert message to user when input is not valid.
alert('Please enter a valid email address.');
}
});
// function to validate email
function validateEmail(email) {
var re = /^[^\\\\s@]+@[^\\\\s@]+\\\\.[^\\\\s@]+$/;
return re.test(String(email).toLowerCase());
}
Real-time validation is crucial for a seamless user experience. In a checkout form, real-time validation saves time by keeping the user aware of what is expected in each field. This would lead to a better user experience, resulting in an effective web application.
Conclusion
JavaScript events play a crucial role in enhancing user experience by avoiding disruptive full-page reloads. By dynamically updating parts of the page in response to user actions, developers can create a more fluid, responsive, and efficient web experience. Leveraging events like click
for updating shopping carts or blur
for real-time form validation ensures that the user’s interaction with the web application is smooth and uninterrupted. This approach not only improves performance but also increases user satisfaction, making web applications more professional and user-friendly.
FAQs
How do you add an event listener in JavaScript?
- You can add an event listener using the addEventListener method, which attaches an event handler to an element without overwriting existing handlers.
What is the difference between event bubbling and capturing?
- Event bubbling is when an event starts from the deepest element and propagates up to the parent elements. Event capturing is the opposite, starting from the outermost element and moving inward.
Can you remove an event listener?
- Yes, you can remove an event listener using the removeEventListener method, which detaches the specified event handler from an element.
How can JavaScript improve user interactions on a website?
- JavaScript can improve user interactions by making web pages dynamic and responsive to user actions, such as clicks, form submissions, and mouse movements.
Why is it important to understand event handling in JavaScript?
- Understanding event handling is crucial for creating interactive web applications that respond to user actions effectively.