Memory management is one of the fundamental aspects of software development. In JavaScript, despite its reputation as a “garbage-collected” language, developers still need to understand how memory works, how garbage collection (GC) operates, and what best practices to follow in order to avoid common pitfalls like memory leaks. This article offers a comprehensive look at JavaScript memory management, providing clear explanations, practical examples, and actionable tips to help you write efficient, high-performance code.
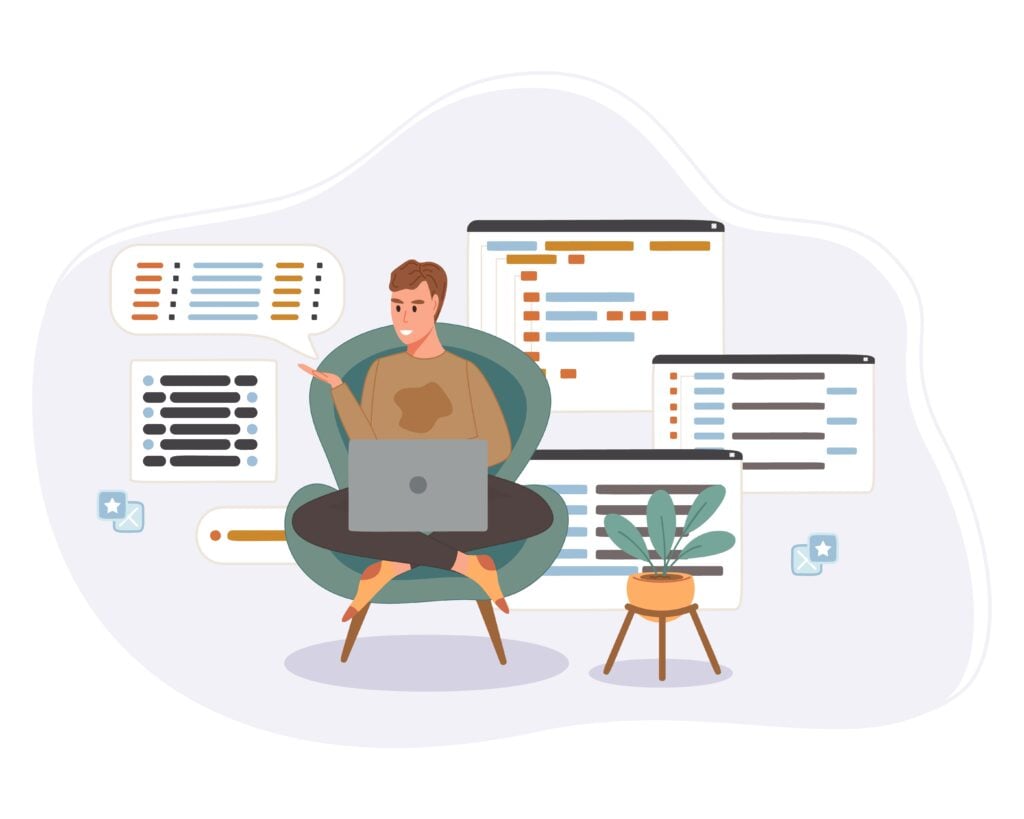
TL;DR
- JavaScript Memory Management: JavaScript automatically handles memory allocation and garbage collection, but developers must still manage resources wisely.
- Garbage Collection: The engine uses algorithms like mark-and-sweep to reclaim unused memory.
- Common Memory Leaks: Occur due to forgotten timers, unintended global variables, and improper handling of closures and DOM elements.
- Best Practices: Include minimizing global variables, cleaning up event listeners and timers, and using modern tools like WeakMap for caching.
- Tools & Techniques: Utilize Chrome DevTools and Node.js memory profilers to detect and fix memory issues.
Understanding Memory Management in JavaScript
What is Memory Management?
Memory management refers to the process by which a programming language allocates, uses, and frees up memory resources. In JavaScript, this process is largely automatic thanks to its garbage collector, which reclaims memory that is no longer needed. However, developers must still understand how memory allocation works to avoid pitfalls like memory leaks.
JavaScript allocates memory for variables, objects, functions, and more as they are created during runtime. Once these data structures are no longer in use, the garbage collector identifies and frees up this memory so it can be reused. Even though these operations happen behind the scenes, the way you structure your code can greatly impact the overall efficiency of memory usage.
Memory Allocation: Stack vs. Heap
In JavaScript, memory is allocated in two main areas:
- Stack Memory: Used for static memory allocation. This includes primitive data types such as numbers, strings, and Booleans. The stack is fast and automatically managed.
- Heap Memory: Used for dynamic memory allocation. This is where objects, arrays, and functions are stored. Memory in the heap is allocated as needed and requires garbage collection to free up memory when it is no longer needed.
How Garbage Collection Works
Garbage collection is the process of reclaiming memory that is no longer in use. While the specifics can vary between JavaScript engines, most modern engines (like V8 in Chrome and Node.js) use a form of the mark-and-sweep algorithm.
The Mark-and-Sweep Algorithm
- Marking Phase – The garbage collector traverses the memory starting from the roots (global objects, active function scopes, etc.) and marks every object that is reachable. This means that any object that can be accessed directly or indirectly is tagged as “in use.”
- Sweeping Phase – After marking all reachable objects, the garbage collector then sweeps through memory to remove any objects that were not marked. These unmarked objects are considered garbage because there are no references to them, meaning they can be safely deallocated.
This process happens periodically, and while it is largely automatic, understanding its principles can help you write code that minimizes the chances of retaining unnecessary memory.
Other Garbage Collection Techniques
Beyond mark-and-sweep, some engines use additional strategies like:
- Reference Counting – This technique involves counting how many references exist to a particular object. When the count reaches zero, the object can be deallocated. However, reference counting has its pitfalls, notably in the case of circular references.
- Generational Collection – Based on the observation that most objects die young, generational collection segregates objects by their lifespan. This allows the garbage collector to focus on newer objects more frequently, optimizing performance for long-running applications.
Common Causes of Memory Leaks
Even though JavaScript handles garbage collection automatically, memory leaks can still occur when references to unused objects are maintained unintentionally. Here are some common causes:
Unintended Global Variables
When variables are declared without the proper keywords (like var
, let
, or const
), they become properties of the global object. This can lead to unexpected memory retention as these variables persist throughout the lifetime of your application.
Forgotten Timers and Callbacks
Timers, intervals, and callbacks that are not cleared or unregistered can continue to hold references to objects. For instance, if you create a timer using setInterval
and forget to clear it with clearInterval
, the memory allocated for the callback function remains in use.
Detached DOM Elements
Memory leaks can also occur in web applications when DOM elements are removed from the document but are still referenced by JavaScript variables or event listeners. This scenario prevents the garbage collector from reclaiming memory, leading to a buildup over time.
Closures Holding References
Closures can be a double-edged sword. While they allow you to maintain state in functions, they can also inadvertently hold onto variables that are no longer needed. This happens when a closure retains a reference to a large object or a DOM node long after it should be garbage collected.
Circular References
Circular references, where two or more objects reference each other, can sometimes prevent the garbage collector from reclaiming memory. While modern garbage collectors can handle many circular references, they can still pose problems in certain edge cases—especially when combined with closures and global variables.
Best Practices for Avoiding Memory Leaks
Avoiding memory leaks requires a proactive approach to coding. Here are some best practices to keep your JavaScript applications lean and efficient:
Declare Variables Properly
Always declare your variables using let
, const
, or var
to avoid unintentionally creating global variables. This small habit goes a long way in preventing unexpected memory retention.
// Good Practice:
let count = 0;
// Bad Practice:
count = 0; // Creates a global variable if not declared within a function or block scope.
Clean Up Timers and Event Listeners
Make sure to clear timers and remove event listeners when they are no longer needed. This is especially important in single-page applications where components are frequently created and destroyed.
// Set a timer
const timerId = setInterval(() => {
// ... do something
}, 1000);
// When done, clear the timer
clearInterval(timerId);
Likewise, remove event listeners when a component is unmounted or when the listener is no longer required.
Manage DOM References Carefully
When working with the DOM, ensure that any references to DOM elements are properly cleaned up when those elements are removed from the document. This prevents memory from being tied up unnecessarily.
// Remove an element and its event listeners
const button = document.getElementById('myButton');
button.removeEventListener('click', handleClick);
button.parentNode.removeChild(button);
Avoid Unnecessary Data Retention in Closures
Be cautious when using closures, as they can inadvertently hold references to large objects or variables that are no longer needed. Consider nullifying references when they are no longer required.
function createCounter() {
let count = 0;
return function() {
count += 1;
return count;
};
}
const counter = createCounter();
// When done using the closure, you might remove references to it.
Utilize Weak References
Where applicable, use data structures like WeakMap
or WeakSet
for caching objects. These structures do not prevent their keys from being garbage collected, helping to minimize memory retention.
const cache = new WeakMap();
function getData(obj) {
if (cache.has(obj)) {
return cache.get(obj);
}
const result = computeExpensiveOperation(obj);
cache.set(obj, result);
return result;
}
Monitor and Profile Your Application
Regularly profile your application using tools like Chrome DevTools or Node.js profilers. Monitoring memory usage can help you identify leaks before they become a problem.
Tools and Techniques for Debugging Memory Issues
Even with the best practices, memory leaks can occur. Fortunately, modern development tools make it easier to detect and fix these issues.
Chrome DevTools
Chrome DevTools provides a suite of features to help you monitor and manage memory usage:
- Memory Profiling: Use the “Memory” tab to take heap snapshots, track allocation timelines, and identify objects that are not being garbage collected.
- Timeline Recordings: Record a timeline to observe memory usage over time and identify potential leaks.
- Debugging Tools: Set breakpoints and watch expressions to track down the source of memory leaks in your code.
Node.js Profilers
For server-side JavaScript, tools such as Node.js’ built-in profiler or third-party solutions like Clinic.js can help you monitor memory usage. These tools offer insights into how memory is allocated and can highlight potential leaks in long-running processes.
Other Utilities
- Heapdump: A Node.js module that allows you to capture heap snapshots for offline analysis.
- Memory Leak Detection Libraries: There are several libraries available that can help detect memory leaks during development and testing.
Regularly using these tools not only helps in identifying issues early but also educates you about how your code interacts with memory—leading to more informed decisions in your development practices.
Advanced Memory Management Techniques
Beyond the basics, several advanced techniques can help you optimize memory usage even further:
Implementing Generational Garbage Collection Insights
Although garbage collection is handled automatically, understanding generational collection concepts can help you design your code to be more efficient. Since most objects die young, try to minimize the long-term retention of temporary objects.
Lazy Loading and Code Splitting
By employing lazy loading and code splitting strategies, you can reduce the initial memory footprint of your application. Only load code when it’s needed, which not only saves memory but also improves load times and overall performance.
Using Immutable Data Structures
Immutable data structures can help prevent unintended side effects that lead to memory leaks. Libraries like Immutable.js provide persistent immutable data structures that can be used to manage state more predictably and efficiently.
Profiling in Production
Consider incorporating memory monitoring in your production environment to catch leaks early. Tools such as New Relic or Dynatrace offer insights into memory usage trends, alerting you to anomalies before they affect end users.
FAQs
What is JavaScript memory management?
- JavaScript memory management refers to the process by which the language allocates, uses, and automatically deallocates memory through its garbage collection system. Understanding how memory is managed helps developers write more efficient and bug-free code.
How does garbage collection work in JavaScript?
- JavaScript typically uses the mark-and-sweep algorithm to perform garbage collection. In this process, the garbage collector marks all objects that are reachable from the roots and then sweeps through memory to remove objects that are no longer accessible.
What are common causes of memory leaks in JavaScript?
- Common causes include unintended global variables, forgotten timers or event listeners, detached DOM elements that remain referenced, closures holding onto unnecessary variables, and circular references.
What best practices can help avoid memory leaks?
- Best practices include declaring variables correctly (using
let
,const
, orvar
), cleaning up timers and event listeners, managing DOM references carefully, nullifying unneeded closures, and using weak references (like WeakMap) when applicable.
How can I detect memory leaks in my JavaScript application?
- Use development tools such as Chrome DevTools or Node.js memory profilers to take heap snapshots and track memory allocation over time. These tools help you identify objects that are not being garbage collected.