Variables are the fundamental building blocks of any programming language. They act as named containers that store data, allowing us to manipulate and reference that data throughout our code. In JavaScript, variables play a crucial role in creating dynamic and interactive web experiences. This comprehensive guide delves into the world of JavaScript variables, equipping you with the knowledge and best practices to effectively manage data in your programs.
Declaring and Initializing Variables
The journey of a variable begins with its declaration. This process involves creating a named storage location in memory and assigning a keyword to identify it. JavaScript offers three keywords for variable declaration:
- var: The traditional keyword used in earlier versions of JavaScript. However, it has limitations regarding scope (explained later) and is generally discouraged in modern development due to potential pitfalls.
- let: Introduced in ES6 (ECMAScript 2015), let provides block-level scoping, making code more predictable and manageable. It’s the recommended keyword for variable declaration in most cases.
- const: Used to declare constant values that cannot be reassigned after initialization. const variables enhance code readability and prevent accidental modifications.
Here’s how you declare and initialize variables using these keywords:
// Using var (not recommended)
var age = 25;
// Using let (recommended)
let name = "Alice";
// Using const (for constants)
const PI = 3.14159;
Variable Naming Conventions:
- Start with a letter, underscore (_), or dollar sign ($). Numbers cannot be at the beginning.
- Can contain letters, numbers, underscores, and dollar signs.
- Case-sensitive (age vs. Age are different variables).
- Avoid reserved keywords (like if, for, function).
- Use meaningful names that reflect the variable’s purpose (e.g., customerName instead of x).
Data Types:
JavaScript is a dynamically typed language, meaning you don’t explicitly specify data types during declaration. Variables can hold various data types, including:
- Numbers: Integers (whole numbers) and floating-point numbers (decimals).
- Strings: Sequences of characters enclosed in quotes (single or double).
- Booleans: Represent logical values (true or false).
- Objects: Complex data structures that hold key-value pairs.
- Arrays: Ordered collections of items, accessed by index.
- Undefined: When a variable is declared but not assigned a value.
- Null: Represents the intentional absence of a value.
Reassignment:
The beauty of variables lies in their ability to change. You can reassign a new value to a variable using the assignment operator (=). For example:
let message = "Hello";
message = "Welcome!"; // Reassigning a new value
Declaring Without Initialization (Implicit Declaration):
While discouraged, omitting the keyword (var) before a variable name implicitly declares it as a global variable (accessible from anywhere in your code). This can lead to naming conflicts and unexpected behavior. Always explicitly declare variables using let or const for better control and code clarity.
Variable Scoping
Scope defines the accessibility of a variable within your code. JavaScript has two primary scoping mechanisms:
- Block Scope (let and const): Variables declared with let or const are only accessible within the block they are declared in (e.g., within curly braces {} of an if statement or a loop). This prevents accidental modification from outer scopes, promoting cleaner and safer code.
- Function Scope (var): Variables declared with var (not recommended) are scoped to the entire function they are declared in. This can cause unintended consequences if you have nested functions that try to access the same variable name.
Here’s an example to illustrate scope:
// Block Scope (let)
if (true) {
let color = "red";
}
console.log(color); // ReferenceError: color is not defined (outside the block)
// Function Scope (var, not recommended)
function greet() {
var message = "Hi";
function sayHi() {
console.log(message); // Has access to message from outer function
}
sayHi();
}
greet();
Hoisting (var only):
A unique behavior specific to var is hoisting. In JavaScript, variable declarations with var are hoisted to the top of their enclosing scope (function or global scope). This means you can reference a var variable before its declaration in the same scope, which can lead to unexpected results if not understood properly. However, let and const variables are not hoisted and must be declared before use.
Temporal Dead Zone (TDZ):
For let and const variables, there exists a concept called the Temporal Dead Zone (TDZ). This is the period between the start of the block where the variable is declared and its initialization. During this time, accessing the variable results in a ReferenceError.
Here’s a table summarizing the key differences between variable declaration keywords:
Keyword | Scope | Hoisting | Reassignment |
var (not recommended) | Function | Yes | Yes |
let (recommended) | Block | No | Yes |
const (for constants) | Block | No | No |
Choosing the Right Keyword:
In modern JavaScript development, it’s generally recommended to use let for variable declaration by default. const should be preferred when dealing with constant values that shouldn’t change. Avoid using var due to its potential for confusion with scope and hoisting.
Data Type Coercion
JavaScript is a forgiving language when it comes to data types. It can sometimes automatically convert values from one type to another during operations. This behavior is called data type coercion and can lead to surprising results if not anticipated.
Here are some common examples of data type coercion:
- Numbers and Strings: When adding a number and a string, JavaScript converts the string to a number (if possible) and performs the addition. For example, 10 + “20” becomes 30.
- Booleans and Numbers: JavaScript treats true as 1 and false as 0 in numeric contexts. For example, 10 * true becomes 10.
- Strings and Booleans: When using comparison operators (== or !=), JavaScript attempts to convert operands to a common type before comparison. For example, “0” equals false.
Understanding data type coercion is crucial to write predictable and robust JavaScript code. Always be mindful of the data types involved in your operations and use explicit type conversion functions (e.g., parseInt(), parseFloat()) if necessary.
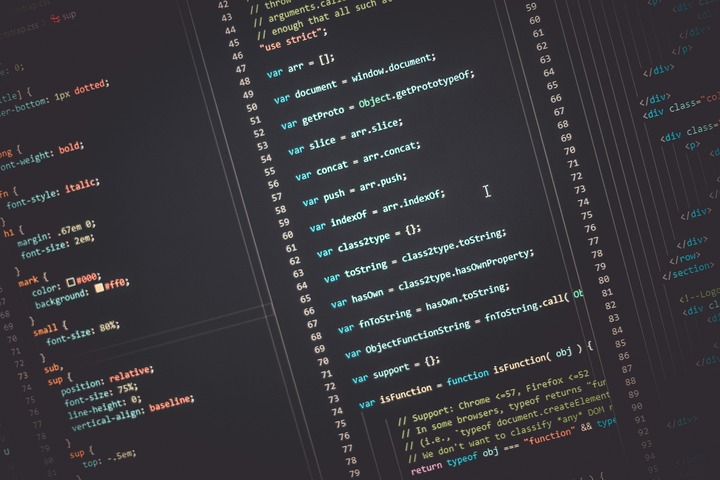
Common Variable-Related Errors
Here are some common errors you might encounter when working with variables in JavaScript:
- ReferenceError: This occurs when you try to access a variable that hasn’t been declared or is outside its scope. Ensure variables are declared with appropriate keywords and accessed within their valid scope.
- TypeError: This error indicates an operation is attempted on a value of the wrong type. For example, trying to add a string and a boolean might result in a TypeError. Double-check data types before performing operations.
- Scope Issues: Accidental modification of variables due to improper scoping can lead to unexpected behavior. Use let and const for block-level scoping and be mindful of the function scope for var (if still used).
Best Practices for Effective Variable Use:
- Descriptive Naming: Use meaningful variable names that reflect their purpose, improving code readability and maintainability.
- Const by Default: Declare variables with const whenever possible to prevent accidental reassignment and enhance code clarity.
- Avoid Implicit Declaration: Always explicitly declare variables using let or const to avoid unintended global variables.
- Be Mindful of Data Types: Understand data types and use type conversion functions when necessary to avoid unexpected behavior due to coercion.
- Proper Scoping: Utilize block-level scoping by default with let and const to prevent naming conflicts and unintended side effects.
By following these best practices, you’ll write cleaner, more maintainable, and less error-prone JavaScript code.
Advanced Variable Techniques
As you delve deeper into JavaScript, you’ll encounter more advanced variable usage techniques:
- Destructuring: A powerful syntax for extracting values from objects and arrays into individual variables.
- The in Operator: Checks if a property exists in an object.
- let/const Block Scoping with for Loops: Ensures loop variables are not accessible outside the loop.
- Closures: Functions that can access and manipulate variables from their outer scope even after the outer function has returned.
These advanced techniques can significantly improve your ability to write complex and efficient JavaScript programs.
Conclusion: JavaScript Variables
By mastering JavaScript variables, you’ve laid the foundation for building effective JavaScript programs. Remember, variables are the workhorses of your code, storing and manipulating data that brings your applications to life. By understanding declaration, scoping, data types, and best practices, you’ll be well on your way to writing clean, maintainable, and powerful JavaScript code.
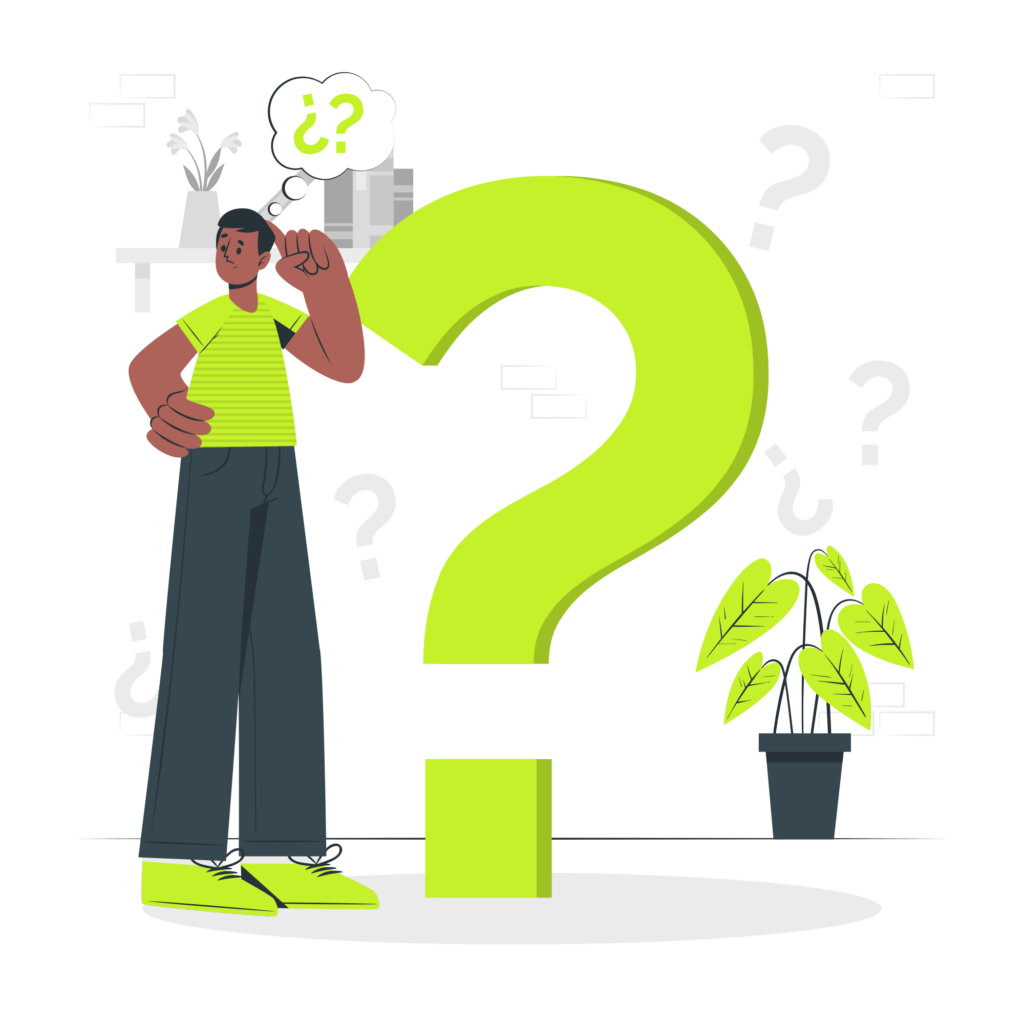
FAQs
What are the types of variables in JavaScript?
- JavaScript supports var, let, and const for variable declarations, each with distinct scope and mutability characteristics.
How do var, let, and const differ in JavaScript?
- Var has function scope, let and const have block scope; let is mutable, while const is for immutable variable declarations.
What is variable scope in JavaScript?
- Variable scope determines the accessibility of variables within different parts of the code, impacting how and where a variable can be used.
Why is understanding variable scope important in JavaScript programming?
- Understanding scope is crucial to avoid bugs and manage variable visibility effectively, ensuring reliable and maintainable code.
What are best practices for using variables in JavaScript?
- Use let and const based on mutability, name variables clearly, and understand scope rules to write clean and effective code.
What is dynamic typing in JavaScript?
- Dynamic typing means variable data types are not declared and can change at runtime, offering flexibility in how variables are used.
How do you declare a variable in JavaScript?
- Declare variables using var, let, or const, followed by a name, and optionally initialize them with a value.
What is the significance of immutable variables in programming?
- Immutable variables, declared with const in JavaScript, enhance code predictability and prevent accidental reassignment.
How can you avoid common variable-related errors in JavaScript?
- Understand scope, use strict mode, and follow naming conventions to avoid errors like undeclared or wrongly accessed variables.
What are some advanced variable management techniques in JavaScript?
- Advanced techniques include using closures for private variables, destructuring assignments, and leveraging scope chain and hoisting wisely.