The world of blockchain technology is full of new and exciting ideas. Blockchains are great because they are very secure and can’t be easily changed, but they don’t have a lot of space to store information. That’s where the InterPlanetary File System (IPFS) comes in. IPFS is a different way to store information that works really well with blockchain. In this article, we’ll talk about how Solidity, which is a special language used to create smart contracts on Ethereum, can work together with IPFS. We’ll look at why this combination is useful and how it works.
What are Solidity and IPFS?
Solidity:
Solidity acts as the building block for smart contracts on the Ethereum blockchain. These self-executing contracts automate agreements and facilitate secure transactions without intermediaries. Solidity allows developers to define the logic and rules governing these contracts, enabling the creation of innovative decentralized applications (DApps).
IPFS:
IPFS, on the other hand, tackles the challenge of data storage in a decentralized manner. Unlike traditional servers, IPFS distributes data across a network of nodes, creating a more robust and censorship-resistant storage solution. Additionally, IPFS leverages a content-addressing system, meaning files are identified by their unique cryptographic hash rather than location. This ensures data integrity and immutability, as any modification to the file results in a different hash.
The Teamwork Between Them
The teamwork between Solidity and IPFS creates a strong partnership that brings many benefits. Decentralized data storage is one major advantage. Normally, blockchains are secure but keeping a lot of data on them can be costly and not very efficient. IPFS offers a smarter way to store data without overloading the blockchain, which only keeps a reference to the data.
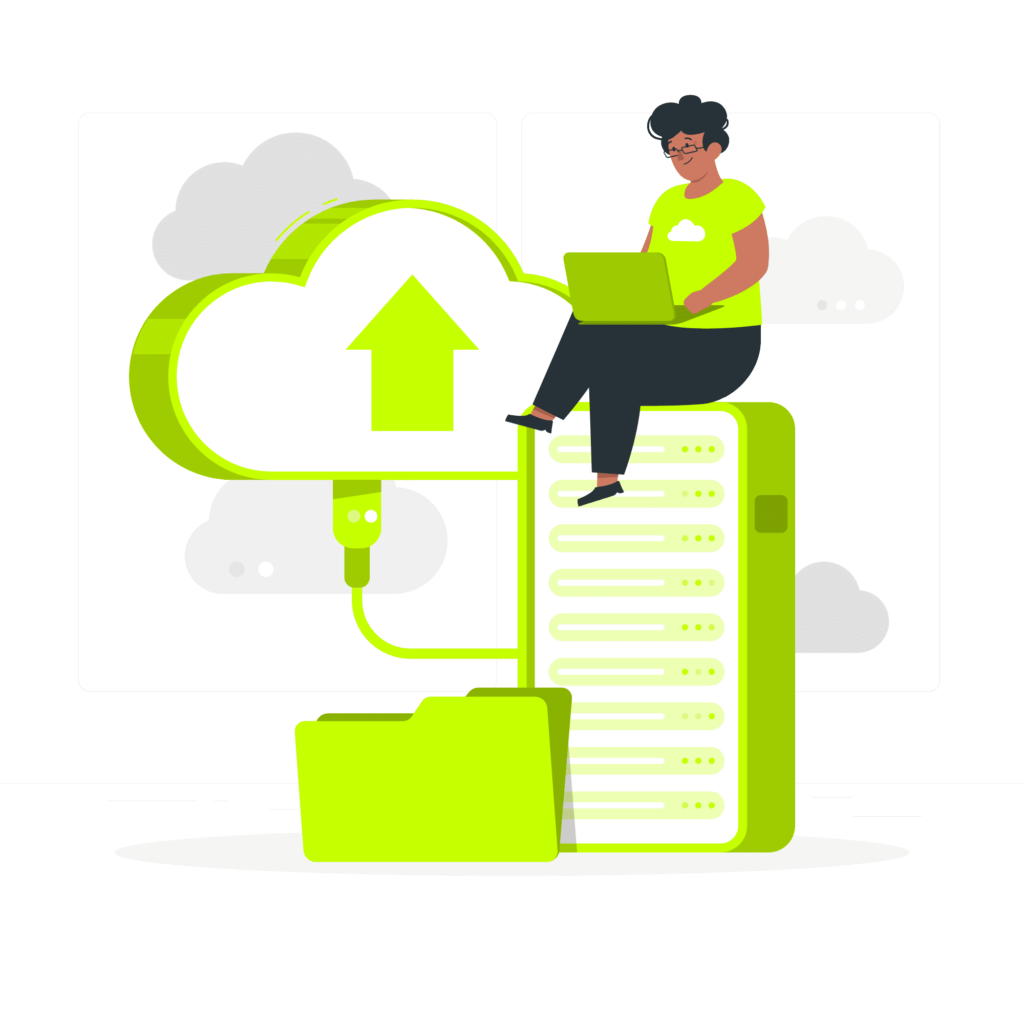
Enhanced security and immutability are also key features of this partnership. Once data is put on IPFS, it can’t be changed, which matches well with blockchain’s goal of keeping data safe and unalterable. There’s also a big plus in censorship resistance because IPFS spreads data across many locations, making it hard to block or remove.
Even if one place tries to delete the data, it’s still available elsewhere. Lastly, improved scalability means that as decentralized apps grow and need to handle more data, IPFS can handle this increase smoothly, unlike traditional ways of storing data that might struggle to keep up.
Use Cases for Solidity and IPFS Integration
The possibilities unlocked by this integration are vast. Here are some exciting use cases:
- Decentralized marketplaces: Imagine a marketplace where product details and images are stored on IPFS, while purchase agreements and transaction history reside on the blockchain. This ensures secure transactions while keeping data readily accessible.
- NFT (Non-Fungible Token) Storage: Store artwork, music, and other digital assets associated with NFTs on IPFS, while the core NFT metadata remains on the blockchain. This reduces gas fees associated with storing large media files on-chain.
- DApp Data Management: DApps can leverage IPFS to store user data, application logic, and other non-critical information. By referencing this data on the blockchain, DApps ensure its immutability and censorship resistance.
- Decentralized Social Media: Build social media platforms where user profiles, posts, and media are stored on IPFS, while user relationships and interactions are recorded on the blockchain. This fosters a more democratic and censorship-resistant social media experience.
Implementing Solidity and IPFS Integration
While the benefits are compelling, implementing this integration requires careful consideration. Here’s a breakdown of the key steps:
01. Setting Up an IPFS Node:
The first step involves establishing an IPFS node, which acts as a client to interact with the IPFS network. Popular IPFS libraries like js-ipfs for Javascript can be used for this purpose.
const IPFS = require('ipfs');
async function createNode() {
const ipfs = await IPFS.create();
console.log('IPFS node created:', ipfs.id);
return ipfs;
}
// Usage
createNode()
.then(ipfs => {
// Use the ipfs node to interact with the IPFS network
})
.catch(err => {
console.error('Error creating IPFS node:', err);
});
02. Uploading Data to IPFS:
Solidity contracts cannot directly interact with IPFS nodes. To upload data to IPFS, you’ll need an external service like an Infura node or a self-hosted IPFS node. Your DApp frontend interacts with this service to upload data and retrieve the corresponding IPFS hash.
async function uploadDataToIPFS(ipfs, data) {
const content = await ipfs.add(data);
const hash = content.cid.string;
console.log('Uploaded data to IPFS, hash:', hash);
return hash;
}
// Usage (assuming you have a string 'my data' to upload)
uploadDataToIPFS(ipfs, 'my data')
.then(hash => {
// Use the hash for further processing
})
.catch(err => {
console.error('Error uploading data to IPFS:', err);
});
03. Storing the IPFS Hash in the Smart Contract:
Once you have the IPFS hash, you can store it within your Solidity smart contract. This hash acts as a reference point for retrieving the actual data from the IPFS network.
pragma solidity ^0.8.0;
contract MyContract {
string public ipfsHash;
function storeData(string memory data) public {
bytes memory bytesData = bytes(data);
ipfsHash = uploadDataToIPFS(bytesData); // Replace with actual upload logic (see external service integration)
}
// Function to retrieve the hash (for informational purposes only)
function getHash() public view returns (string memory) {
return ipfsHash;
}
}
04. Retrieving Data from IPFS:
The DApp frontend, equipped with the IPFS hash obtained from the smart contract, can leverage JavaScript libraries like ipfs-http-client to interact with the IPFS network. Here’s a breakdown of the process:
- Initiate IPFS Client:
The DApp frontend establishes a connection with an IPFS gateway, essentially a public node that acts as an entry point to the IPFS network. Libraries like ipfs-http-client provide methods for connecting to gateways. - Request Data Using Hash:
Once connected, the DApp sends a request to the IPFS gateway, specifying the retrieved IPFS hash. This request instructs the network to locate and retrieve the data associated with that hash. - Receive and Process Data:
Upon successful retrieval, the IPFS gateway delivers the data to the DApp frontend. The frontend can then process and display this data to the user.
const ipfsClient = require('ipfs-http-client');
async function getDataFromIPFS(hash) {
const ipfs = ipfsClient('/ipfs/infura.io/ipfs/'); // Replace with your IPFS gateway URL
const data = await ipfs.cat(hash);
console.log('Retrieved data from IPFS:', data.toString());
return data;
}
// Usage (assuming you have the IPFS hash)
getDataFromIPFS(ipfsHash)
.then(data => {
// Process and display the retrieved data
})
.catch(err => {
console.error('Error retrieving data from IPFS:', err);
});
Note: These are simplified examples for demonstration purposes. Real-world implementations will involve additional security considerations, error handling, and integration with specific tools and frameworks.
Keep In Mind!
Here are some key factors to consider when implementing Solidity and IPFS integration,
- Off-chain Data Availability: Data stored on IPFS is not guaranteed to be permanently available. Nodes hosting the data may go offline, leading to data loss. Implementing pinning services or replicating data across multiple nodes can mitigate this risk.
- Gas Fees: While IPFS reduces on-chain storage costs, interacting with the blockchain by storing and retrieving IPFS hashes still incurs gas fees. Optimizing smart contract functionality and minimizing unnecessary interactions can help manage gas costs.
- Security: While IPFS offers censorship resistance, it doesn’t guarantee complete data privacy. Sensitive data might require additional encryption layers before uploading to IPFS.
Advanced Techniques
For experienced developers, several advanced techniques can further enhance the integration:
- Oracle Networks: Utilize oracle networks to bridge the gap between on-chain and off-chain data. Oracles can verify the availability and integrity of data stored on IPFS before allowing smart contract execution.
- Filecoin Integration: Leverage Filecoin, a blockchain-based storage network, to incentivize nodes to store data on IPFS for extended periods. This can ensure the persistence and reliability of off-chain data.
- Zero-Knowledge Proofs (ZKPs): Explore the use of ZKPs to allow smart contracts to verify the content of data stored on IPFS without actually revealing the data itself. This can be particularly beneficial for privacy-sensitive applications.
The Future of Decentralized Storage
The integration of Solidity and IPFS represents a significant leap forward in creating robust and scalable decentralized applications. As both technologies continue to evolve, we can expect even more sophisticated use cases and functionalities to emerge. By leveraging the strengths of both blockchain and decentralized storage, developers can build applications that are not only secure and transparent but also resistant to censorship and capable of handling massive amounts of data. This collaborative evolution paves the way for a truly decentralized future, fostering innovation and empowering users with greater control over their data.
Conclusion
The potential of Solidity and IPFS integration is vast, opening doors to a future of secure, scalable, and censorship-resistant decentralized applications. By understanding the core concepts, exploring the available resources, and actively engaging with the developer community, you can play a crucial role in shaping this exciting technological landscape. So, embrace the power of this integration, unleash your creativity, and join the movement towards a truly decentralized future!
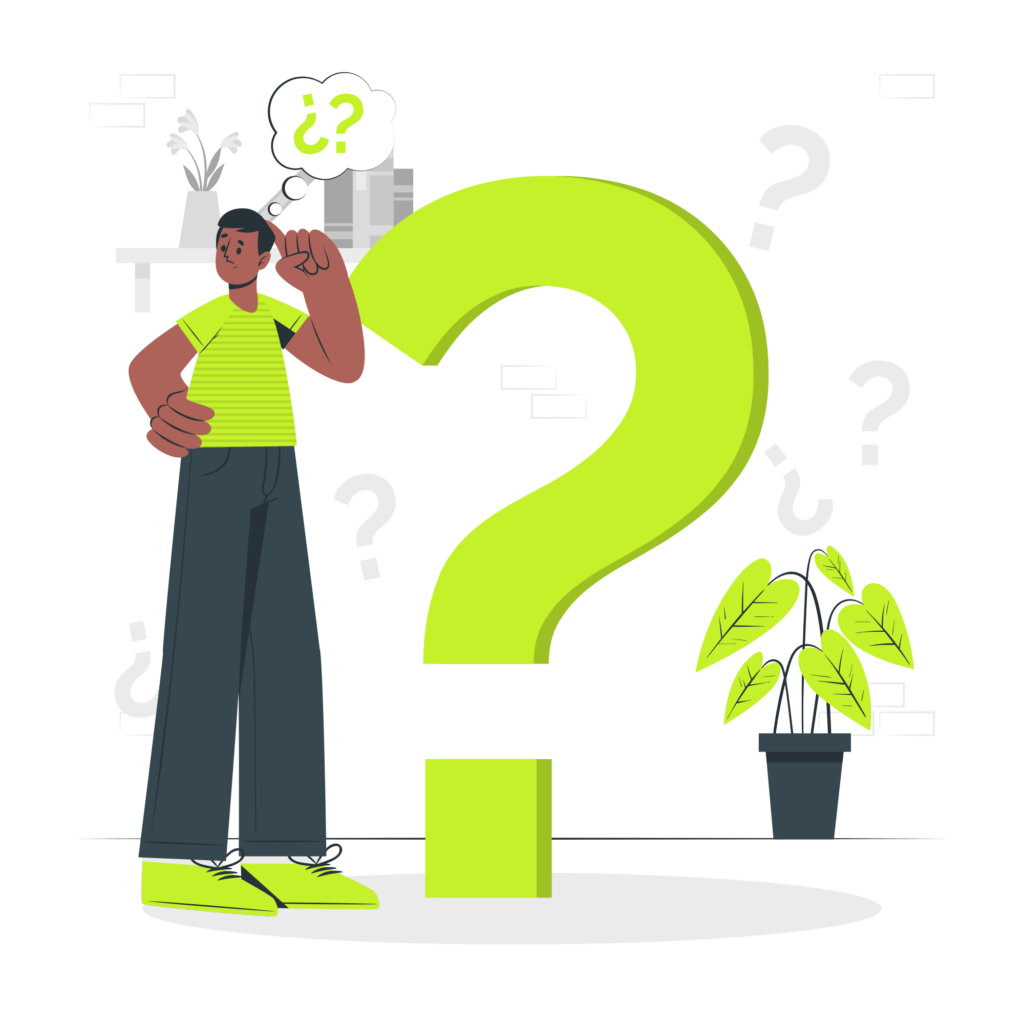
FAQs
What is Solidity and how is it used in blockchain apps?
- Solidity is a programming language designed for developing smart contracts on blockchain platforms, playing a crucial role in creating decentralized applications.
How does IPFS integration benefit blockchain applications?
- IPFS integration provides decentralized and secure storage solutions, enhancing data availability and resilience in blockchain applications.
Can Solidity and IPFS be integrated seamlessly?
- Yes, Solidity and IPFS can be integrated smoothly to leverage the benefits of decentralized storage and smart contracts, improving app functionality.
What are the key steps in integrating Solidity with IPFS?
- Key steps include setting up IPFS, creating Solidity smart contracts, and establishing communication between the contracts and IPFS for data storage and retrieval.
How does IPFS integration improve the security of blockchain apps?
- IPFS offers a decentralized storage solution, reducing points of failure and enhancing data security in blockchain applications.
What is blockchain technology?
- Blockchain technology is a decentralized digital ledger that records transactions across many computers, ensuring security and transparency.
What are smart contracts?
- Smart contracts are self-executing contracts with the terms directly written into code, automating and enforcing agreements on the blockchain.
How does decentralized storage work?
- Decentralized storage distributes data across a network, eliminating single points of failure and enhancing security and accessibility.
What is the significance of decentralized applications (DApps)?
- DApps run on a blockchain or P2P network, offering open-source, decentralized, and secure application solutions.
Why is blockchain development important?
- Blockchain development is crucial for creating secure, transparent, and efficient systems, revolutionizing industries like finance, healthcare, and more.