JavaScript, the popular language for web development, empowers developers to create dynamic and interactive experiences. At the heart of this dynamism lie control structures – the building blocks that determine the flow of your program’s execution. Understanding these structures is fundamental to writing efficient, maintainable, and elegant JavaScript code.
This complete guide dives into the various JavaScript control structures, exploring their functionalities, use cases, and best practices. Whether you’re a experienced developer or just beginning your JavaScript journey, this in-depth exploration will equip you with the knowledge to navigate the flow of your programs with precision.
What are Control Structures?
What do we use control structures for in JavaScript? Control structures are programmatic constructs that determine how your code executes. They provide mechanisms for making decisions, repeating code blocks, and changing the flow of execution based on specific conditions. By effectively utilizing these structures, you can write code that is more responsive, adaptable, and efficient.
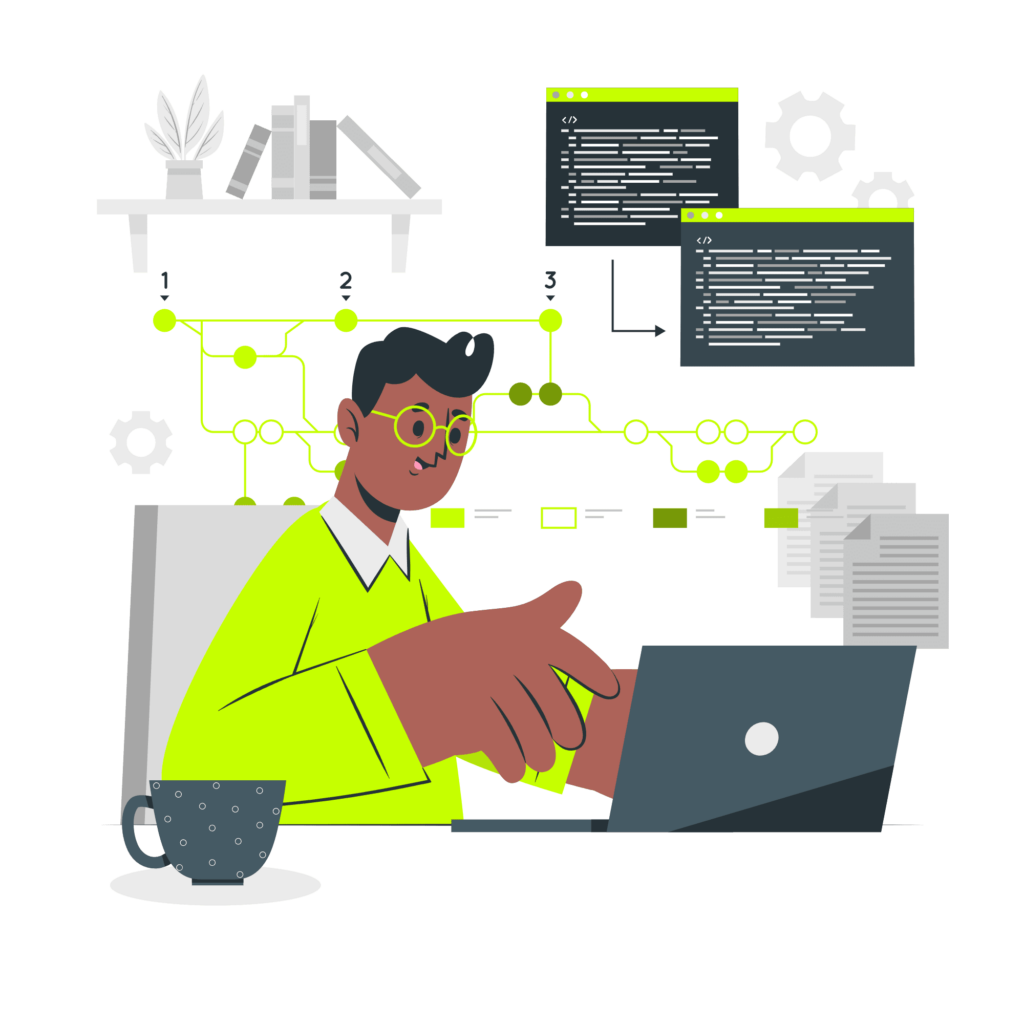
JavaScript offers a rich set of control structures, categorized into three primary types:
- Conditional Statements: These structures allow your code to make decisions based on conditions. They execute specific code blocks only when the defined conditions are met.
- Looping Statements: These structures enable you to repeat a block of code a specific number of times or until a particular condition is met. This is crucial for iterating through data collections and performing repetitive tasks.
- Jumping Statements: These structures alter the normal flow of execution within a loop or conditional statement. They provide mechanisms for exiting loops before the loop stops or skipping iterations.
Understanding how these structures work individually and how they can be combined effectively is key to writing robust and well-structured JavaScript code.
Conditional Statements
Conditional statements are the tools JavaScript uses to make choices. They allow your code to execute different code blocks based on the evaluation of conditions. Here, we explore the essential conditional statements:
- The
if
Statement: This is the most fundamental conditional statement. It evaluates a condition and executes a block of code if the condition is true. Optionally, you can include an else block that executes if the condition is false.
let grade = 85;
if (grade >= 90) {
console.log("Excellent!");
} else {
console.log("Keep practicing!");
}
- The
else if
Statement: This allows for chaining multiple conditions within an if statement. It lets you check several things one by one, and only runs the code for the first thing that’s true.
let grade = 85;
if (grade >= 90) {
console.log("Excellent!");
} else if (grade >= 80) {
console.log("Great job!");
} else {
console.log("Keep practicing!");
}
- The
switch
Statement: This structure is used for multi-way branching based on the value of an expression. It provides a cleaner alternative to nested if statements when dealing with several possible conditions that evaluate to different values.
let day = "Tuesday";
switch (day) {
case "Monday":
console.log("Start of the week!");
break;
case "Tuesday":
case "Wednesday":
case "Thursday":
console.log("Midweek grind!");
break;
case "Friday":
console.loop("TGIF!");
break;
default:
console.log("Enjoy the weekend!");
}
The break keyword is important within the switch statement. It terminates the execution of the switch block once a matching case is found, preventing unintended fall-through. The default case serves as a catch-all for any value that doesn’t match a defined case.
- The Ternary Operator (Conditional Operator): This compact operator provides a shorthand way to write simple conditional expressions. It evaluates a condition and returns one of two values based on the outcome.
let message = age >= 18 ? "You are eligible to vote." : "Not eligible to vote.";
So, what do we use control structures for in JavaScript? They are essential for creating interactive and responsive web applications, allowing you to tailor the behavior of your program based on user input, data validation, and various other factors.
Looping Statements
Looping statements are fundamental for iterating through data collections, executing code blocks repeatedly until a condition is met, and automating repetitive tasks. JavaScript offers three primary looping structures:
- The
for
Loop: This handy loop lets you do something over and over again easily. It keeps track of where you are using a counter, and stops when a condition isn’t met anymore.
for (let i = 0; i < 5; i++) {
console.log("Iteration:", i);
}
In this example, the loop initializes i to 0, checks if i is less than 5 (the condition), and increments i by 1 after each iteration. This allows the loop to iterate five times, printing the value of i in each iteration.
The for loop offers a flexible syntax that can be adapted for various use cases. Here’s an example iterating through an array:
let fruits = ["apple", "banana", "orange"];
for (let i = 0; i < fruits.length; i++) {
console.log("Fruit:", fruits[i]);
}
- The
while
Loop: This loop continues executing a code block as long as a specified condition remains true. The condition is checked before each iteration, and the loop continues as long as the condition evaluates to true.
let count = 0;
while (count < 3) {
console.log("Count:", count);
count++;
}
In this example, the loop continues as long as count is less than 3. The count++ statement increments count after each iteration, eventually causing the condition to become false and the loop to stop.
- The
do-while
Loop: This loop guarantees that the code block executes at least once, even if the initial condition evaluates to false. The condition is checked after the code block executes. The loop continues iterating as long as the condition remains true.
let input = "";
do {
input = prompt("Enter your name (or 'quit' to exit):");
} while (input.toLowerCase() !== "quit");
console.log("Hello,", input);
This example utilizes a do-while loop to continuously prompt the user for input until they enter “quit”. The loop executes at least once, ensuring the user gets a chance to enter their name.
Choosing the appropriate looping structure depends on the specific needs of your program. The for loop is often preferred for its concise syntax when the number of iterations is known beforehand. The while loop is suitable when the loop continuation depends on a dynamic condition. The do-while loop is ideal when you need the code block to execute at least once, regardless of the initial condition.
Jumping Statements
JavaScript provides two primary jumping statements that change the normal flow of execution within loops:
- The
break
Statement: This statement instructs to jump out of the loop, even if the loop isn’t finished yet. It’s often used to exit a loop before the loop stops when a specific condition is met within the loop.
let numbers = [1, 5, 2, 8, 3];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
console.log("Found an even number:", numbers[i]);
break;
}
}
In this example, the break statement within the if block exits the loop as soon as an even number is found.
- The
continue
Statement: This statement skips the current iteration of the enclosing loop and proceeds to the next iteration. It allows you to selectively skip specific iterations based on conditions within the loop.
let numbers = [1, 5, 2, 8, 3];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
continue;
}
console.log("Odd number:", numbers[i]);
}
Here, the continue statement skips any even numbers in the array, printing only the odd numbers.
Jumping statements should be used with good judgment, as excessive use can make code harder to read and maintain. However, when used appropriately, they can improve the control flow of your loops.
Best Practices for Using Control Structures
Understanding what we use control structures for in JavaScript helps in applying best practices that ensure your code is clear, maintainable, and efficient:
- Clarity over Cleverness: Prioritize clear and readable code over overly complex control structures. Use meaningful variable names, proper indentation, and comments to enhance code readability.
- Tend to use switch for Discrete Choices: When dealing with a limited set of separate conditions, the switch statement often provides a cleaner and more readable alternative to nested if statements.
- Break Out Complex Logic: Extracting complex conditional logic into a separate function improves code modularity and reusability.
- Utilize for…of for Iterables: For iterating through iterable objects like arrays and strings, the for…of loop provides shorter syntax and eliminates the need for manual index management.
- Employ forEach for Simple Array Iterations: When you only need to iterate through an array and perform an action on each element without keeping track of the index, the forEach method offers a convenient alternative to a for loop.
- Handle Errors Gracefully: Utilize try…catch blocks to expect and handle potential errors within your control structures, preventing unexpected program termination.
- Test Thoroughly: Write unit tests to ensure your control structures function as expected under various conditions. This promotes code reliability and maintainability.
By adhering to these best practices, you can use control structures to write robust, well-structured, and efficient JavaScript programs.
Advanced Control Flow Techniques
JavaScript offers additional features that enhance control flow beyond the fundamental structures:
- Labeled Statements: These statements allow you to associate a label with a specific statement within your code. You can then use a goto statement (used with caution due to potential readability issues) to jump to the labeled statement from another part of your code. However, due to potential for making code harder to follow, labeled statements and goto are generally discouraged in favor of more structured control flow techniques.
- Destructuring with Conditionals: Destructuring assignments can be combined with conditional statements to create simple and readable code for complex branching logic.
let role = "admin";
let message = role === "admin"
? "Welcome, administrator!"
: "Hello, user!";
console.log(message);
- Recursion: This technique involves a function calling itself within its own body. Recursion can be a powerful tool for solving problems that involve breaking down a task into smaller, similar subtasks. However, it’s important to implement base cases to prevent infinite recursion.
Understanding these advanced techniques expands your ability to write advanced JavaScript programs. However, it’s essential to prioritize code clarity and maintainability when choosing these approaches.
Conclusion
Control structures are the most important things of any programming language. What do we use control structures for in JavaScript? By mastering JavaScript’s control structures, you gain the power to write code that is:
- Responsive: Adapt to user input and dynamic conditions.
- Efficient: Execute repetitive tasks with minimal overhead.
- Maintainable: Organize code in a logical and readable manner.
- Structured: Create well-defined program flow for complex logic.
This complete guide has equipped you with the knowledge to navigate the flow of your JavaScript programs with precision. As you continue your JavaScript journey, practice applying these structures effectively, experiment with different approaches, and always aim for clear and maintainable code. Mastering them empowers you to craft elegant and powerful JavaScript applications.
Wanna Learn more about JavaScript? Check our articles on JavaScript 👇
- How JavaScript Works?
- JavaScript Variables
- JavaScript Operators
- JavaScript Functions
- JavaScript Arrays
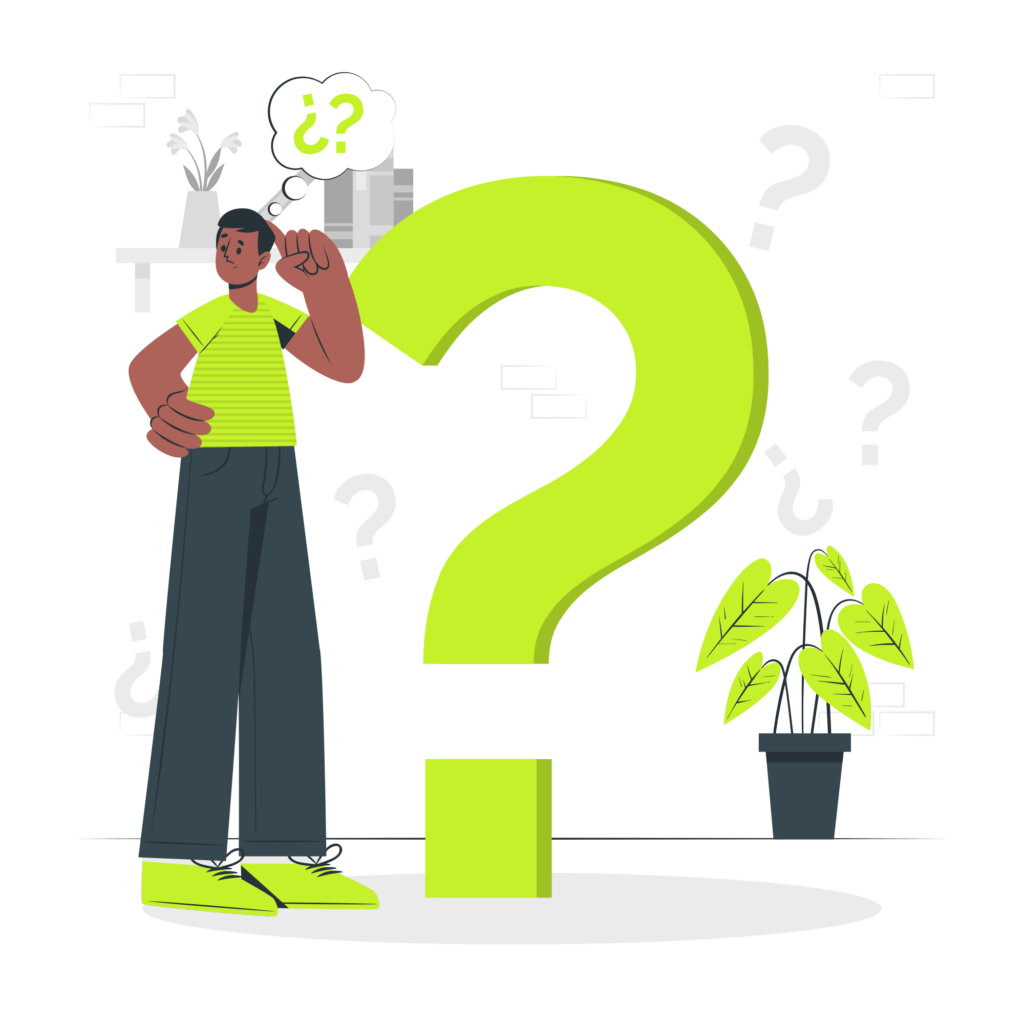
FAQs
What are JavaScript control structures?
- Control structures in JavaScript help manage the flow of code execution, using conditionals and loops to handle decisions and repetitive tasks.
How do loops work in JavaScript?
- Loops in JavaScript, like for and while, repeatedly execute a block of code as long as a specified condition remains true.
What is the purpose of if-else statements in JavaScript?
- If-else statements allow JavaScript programs to execute certain code blocks based on conditions, providing decision-making capabilities.
Can you explain error handling in JavaScript?
- Error handling in JavaScript is managed through try-catch blocks, allowing developers to gracefully handle exceptions and maintain code execution flow.
What is a switch-case statement?
- A switch-case statement in JavaScript lets you execute different parts of code based on the value of an expression, serving as a cleaner alternative to multiple if-elses.
Why are control structures important in programming?
- Control structures are fundamental for creating dynamic and efficient programs, as they control the execution flow based on logic and conditions.
How can mastering control structures improve coding?
- Mastering control structures enhances problem-solving skills and enables the development of more complex, efficient, and maintainable code.
What are common mistakes when using control structures in JavaScript?
- Common mistakes include infinite loops, incorrect conditional statements, and overlooking exception handling, leading to potential bugs and inefficiencies.
How does JavaScript handle asynchronous control structures?
- JavaScript uses features like callbacks, promises, and async/await to manage asynchronous operations, allowing non-blocking code execution.
Are there any best practices for using control structures in JavaScript?
- Best practices include using descriptive conditions, avoiding deep nesting, and employing error handling to improve readability and reliability of code.