TL;DR
- Python’s try-except block helps handle exceptions gracefully, preventing crashes.
- Always catch specific exceptions instead of using generic except Exception.
- Use else for code that runs only if no exceptions occur, improving readability.
- Utilize finally for necessary cleanup, such as closing files or releasing resources.
- Keep try blocks small and scoped to improve error isolation and debugging.
When working with Python, one of the most important aspects of writing robust and error-resilient code is understanding the use of the try-except
block. It is Python’s mechanism for handling exceptions and making sure your application can gracefully recover from unexpected errors.
This article explores the best practices for Python try except, focusing on its implementation, common mistakes to avoid, and how to use it effectively. Whether you’re a Python programmer or someone working with a different programming language, these concepts will provide valuable insights to help you write error-resilient code. Online homeschool math programs simplify challenging subjects for students in much the same way that these practices make error handling in programming easier to understand and apply.
Understanding Try Except in Python
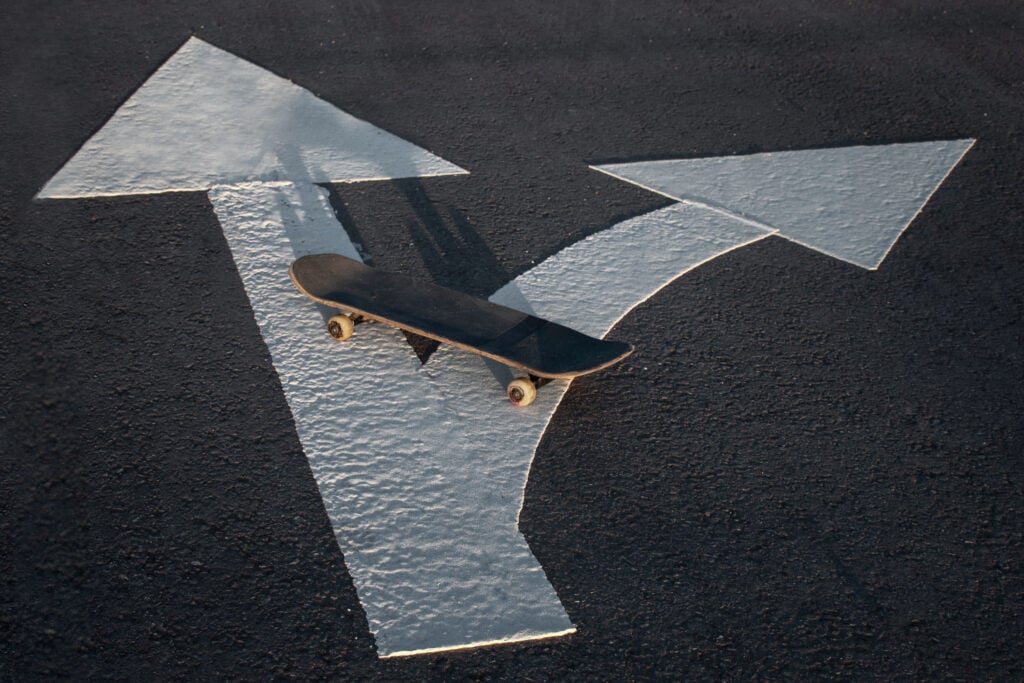
The try-except
mechanism in Python is a powerful tool for managing exceptions and making sure that your code can handle errors gracefully. To understand its utility, it’s essential to understand the structure of try-except
and the different types of errors it can handle.
What is Try Except ?
The try-except
block in Python allows you to catch and respond to exceptions that occur during the execution of your program. Instead of letting the program crash when an error is encountered, try-except
provides a way to gracefully handle errors and continue execution.
Refer to the following code for the basic structure of Try-Except in Python.
try:
# Code that might raise an exception
except SpecificException as e:
# Code to handle the exception
else:
# Code to run if no exception occurs (optional)
finally:
# Code that runs regardless of exceptions (optional)
- Try Block
- Contains the code that might raise an exception. This is the section where you place any code that you anticipate could potentially result in an error.
- Except Block
- Executes if a specific exception is raised in the
try
block. This block handles the exception by defining how to manage it, preventing the program from crashing.
- Executes if a specific exception is raised in the
- Else Block
- Runs only if no exceptions are raised in the
try
block. It’s an optional block that helps to handle the case when everything runs smoothly.
- Runs only if no exceptions are raised in the
- Finally Block
- Executes regardless of whether an exception was raised or not. This block is typically used for cleanup actions, like closing files or releasing resources.
Errors in your code can arise in two scenarios. The first occurs when an unexpected event disrupts the program, causing it to stop and fail to execute further. The second scenario involves the developer intentionally simulating errors based on specific conditions in the code. Regardless of the cause, it is important to handle these errors effectively to make sure that the application can continue running seamlessly by following an alternative path.
Python errors, often referred to as exceptions, fall into various categories. Below are some common exceptions you may encounter.
Error Type | Description |
---|---|
SyntaxError | Occurs when Python detects a violation of its syntax rules. |
NameError | Raised when a variable or function is referenced before it’s defined. |
TypeError | Occurs when an operation is performed on an incompatible type. |
ValueError | Raised when a function receives an argument of the correct type but an invalid value. |
IndexError | Occurs when attempting to access an index outside the range of a list or sequence. |
KeyError | Raised when a dictionary key is accessed but does not exist. |
ZeroDivisionError | Occurs when dividing a number by zero. |
FileNotFoundError | Raised when trying to access a file that doesn’t exist. |
ImportError / ModuleNotFoundError | Occurs when an import statement fails to find the specified module. |
AttributeError | Raised when an object doesn’t have the specified attribute. |
RuntimeError | A general-purpose error for issues detected during runtime that don’t fall into other categories. |
MemoryError | Raised when the program runs out of memory. |
StopIteration | Occurs when there are no more items in an iterator. |
Why Exception Handling Matters ?
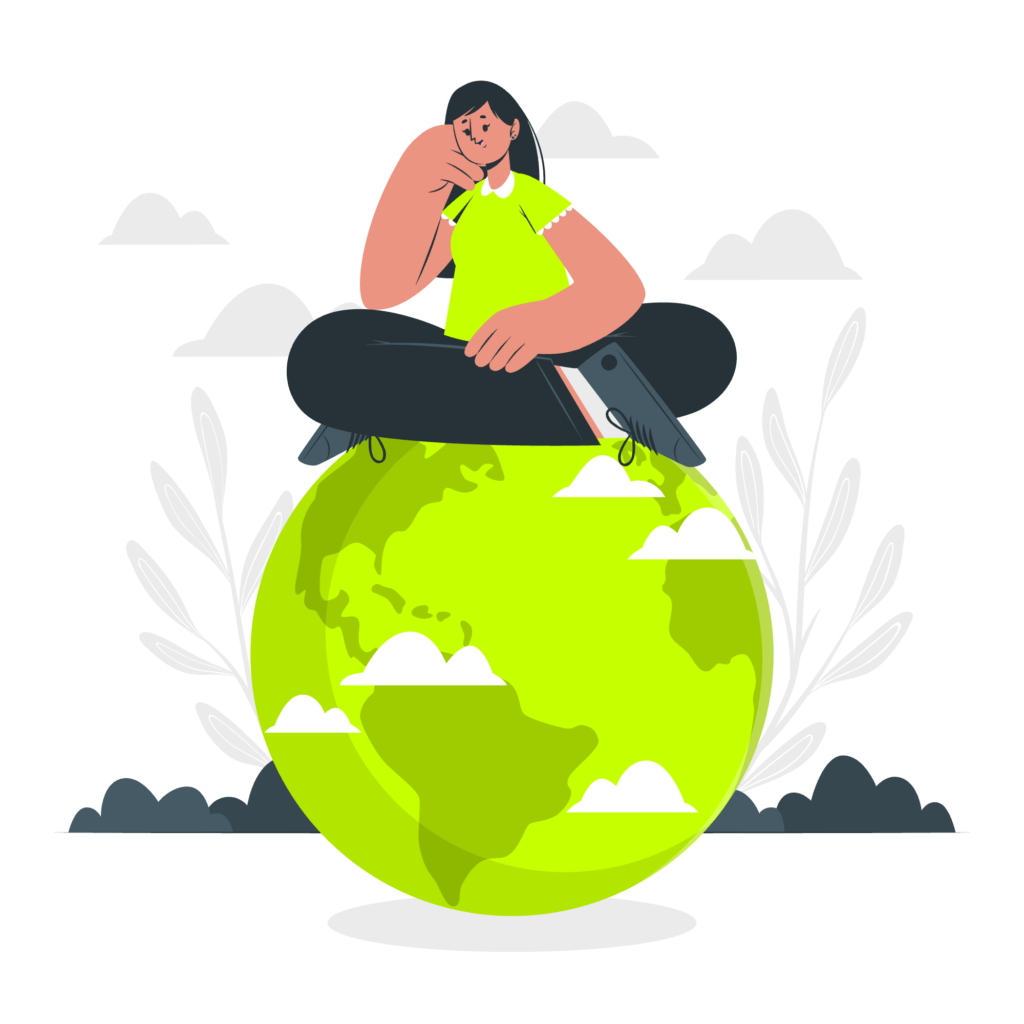
Errors in code are inevitable. They can arise from various sources, such as incorrect user input, network issues, or unexpected edge cases. Without proper handling, these errors can lead to application crashes and a poor user experience. To build resilient applications, it’s essential to adopt effective error-handling strategies.
Using Python’s try-except
block is a powerful way to manage errors. It helps make sure your code provides meaningful error messages, making it easier to identify and fix issues. Additionally, it prevents the application from crashing abruptly, allowing it to handle errors gracefully and continue running when possible.
Another key benefit of the try-except
approach is resource management. By properly handling exceptions, you can make sure that resources like files, network connections, or database sessions are released or closed, even when an error occurs. This guarantees your application remains robust and efficient, even in the face of unexpected challenges.
Best Practices for Exception Handling
Now that we understand the concept of try-except
in Python and how to implement it in our applications, it’s important to explore best practices. Adopting these practices not only enables your code to remain efficient and clean but also keeps it aligned with modern development standards. By following these tips, you can effectively handle errors while maintaining the performance and reliability of your application.
1. Limit the Scope of Try Blocks
Avoid wrapping your entire code in a single try
block. Instead, narrow down the scope to just the statements that might raise an exception. This enables better error isolation and debugging.
# Bad Practice
try:
a = int(input("Enter a number: "))
b = int(input("Enter another number: "))
result = a / b
print(f"Result is {result}")
except Exception as e:
print(f"An error occurred: {e}")
# Best Practice
try:
a = int(input("Enter a number: "))
b = int(input("Enter another number: "))
result = a / b
except ZeroDivisionError:
print("Cannot divide by zero.")
except ValueError:
print("Please enter valid integers.")
else:
print(f"Result is {result}")
2. Catch Specific Exceptions
Catching specific exceptions enables your program to handle only the errors it is equipped to resolve. Avoid generic exception handling unless absolutely necessary.
# Bad Practice
try:
file = open('data.txt', 'r')
content = file.read()
except Exception:
print("An error occurred while reading the file.")
# Best Practice
try:
file = open('data.txt', 'r')
content = file.read()
except FileNotFoundError:
print("The file does not exist.")
except IOError:
print("An error occurred while accessing the file.")
3. Use Else Clause for Clean Separation
The else
clause allows you to separate code that should only run when no exceptions are raised, making your program more readable.
try:
result = perform_operation()
except SomeError as e:
print(f"An error occurred: {e}")
else:
print(f"Operation succeeded: {result}")
4. Avoid Bare Except Statements
Using a bare except
clause can unintentionally catch unexpected exceptions, making debugging harder.
# Bad Practice
try:
risky_operation()
except:
print("Something went wrong.")
# Best Practice
try:
risky_operation()
except SpecificError:
print("Handled SpecificError.")
5. Utilize Finally for Cleanup
The finally
block is important for cleanup tasks that must be executed regardless of whether an exception occurs.
try:
file = open('data.txt', 'r')
content = file.read()
except FileNotFoundError:
print("The file does not exist.")
finally:
file.close()
Conclusion
Learning the try-except
mechanism in Python is needed for creating robust, error-tolerant applications. By understanding its structure and learning to handle specific exceptions, you can enable your program to operate reliably under a wide range of conditions.
Python offers a variety of exception types to manage errors effectively. By familiarizing yourself with common exceptions and the scenarios in which they arise, you can proactively design your programs to handle errors gracefully, improve user experience, and reduce downtime.
Ultimately, the try-except
block is not just about avoiding crashes, it’s about writing professional-grade, resilient Python code that can adapt to real-world challenges.
FAQs
Why should I avoid bare except statements?
- Bare
except
statements catch all exceptions, including system-level ones likeKeyboardInterrupt
, making it harder to debug and potentially masking critical issues.
Can I have multiple except clauses for a single try block?
- Yes, you can have multiple
except
clauses to handle different exceptions specifically.
How can I use the else clause effectively?
- The
else
clause is ideal for code that should run only when no exceptions are raised. It improves readability by separating normal execution from error handling.
What should I do in the finally block?
- The
finally
block is used for cleanup tasks, such as closing files, releasing resources, or ending database transactions.
What are common mistakes to avoid in try-except usage?
- Some common mistakes include:
- Using broad
except
clauses. - Wrapping too much code in the
try
block. - Failing to log exceptions for debugging.
- Using broad
Is exception handling bad for performance?
- Exception handling has a slight performance overhead, so it should not replace validation or condition checks. However, when used appropriately, it enables robust error handling without significant performance impact.