NFTs (Non-Fungible Tokens) have taken the digital world by storm, changing how we perceive ownership of art, music, gaming assets, and even real estate. But behind every successful NFT lies a smart contract—the backbone that powers its uniqueness, security, and transferability.
In this article, we will break down NFT smart contracts and NFT programming, making sure you fully grasp how they work, how to create them, and what factors to consider when deploying them.
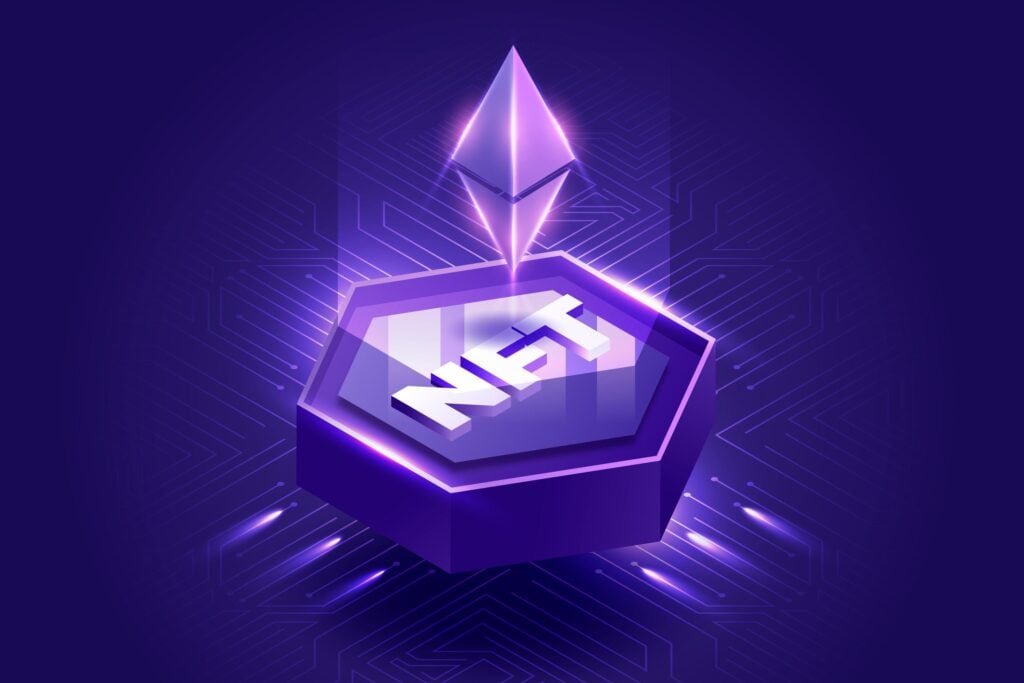
TL;DR
- NFT Blockchain Contracts are self-executing blockchain programs that handle the creation, transfer, and ownership of NFTs.
- They are typically written in Solidity (Ethereum), Rust (Solana), or Vyper and use frameworks like Hardhat, Truffle, and OpenZeppelin.
- Key features include immutability, self-execution, ownership verification, royalties, and programmability.
- Security best practices include avoiding reentrancy attacks, using OpenZeppelin libraries, and optimizing gas fees.
- Future trends involve cross-chain NFTs, AI-generated NFTs, and privacy-enhanced ownership with zero-knowledge proofs.
What is an NFT Smart Contract?
An NFT Blockchain Contracts is a self-executing contract with the terms of the agreement directly written into code. These contracts run on blockchain networks like Ethereum, Solana, Binance Smart Chain, and Polygon, automating the processes of minting, transferring, and managing NFTs.
Key Features of an NFT Smart Contract
- Immutability: Once deployed, smart contracts cannot be changed, ensuring security.
- Self-Execution: No intermediaries are required for transactions.
- Ownership Verification: Proves the authenticity and ownership of digital assets.
- Programmability: Developers can set unique functionalities such as royalties, access permissions, and burning mechanisms.
- Interoperability: NFTs can be traded across multiple platforms.
How NFT Smart Contracts Work
An NFT smart contract operates through a series of automated processes. When an NFT is created, a smart contract generates a unique token containing metadata such as an image, video, or asset details. This metadata is then stored on decentralized solutions like IPFS or Arweave, ensuring permanence. Ownership of the NFT is recorded on the blockchain, allowing for seamless verification. Additionally, many contracts include royalty mechanisms, meaning that creators earn a percentage of revenue every time the NFT is resold. These steps together establish the security, authenticity, and programmability of NFTs in the digital ecosystem.
The Tech Behind NFT Programming
Programming Languages Used in NFT Development
Different blockchain networks use different languages for NFT smart contract development:
- Solidity (Ethereum, Binance Smart Chain, Polygon)
- Rust (Solana, Near Protocol)
- Vyper (Alternative to Solidity for Ethereum)
- Clarity (Stacks Blockchain for Bitcoin NFTs)
Frameworks & Tools for NFT Development
To make NFT development efficient, developers use frameworks and tools like:
- Hardhat & Truffle (Ethereum development and testing)
- OpenZeppelin (Pre-built, secure smart contract templates)
- Metaplex (Solana NFT development framework)
- Alchemy & Infura (Blockchain API providers for seamless interactions)
- Pinata & IPFS (Decentralized file storage for NFT metadata)
How to Create an NFT Smart Contract
Step 1: Set Up Your Environment
- Install Node.js and npm/yarn
- Set up Metamask for blockchain interaction
- Install Hardhat/Truffle for smart contract development
Step 2: Write the Smart Contract in Solidity
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyNFT is ERC721, Ownable {
uint256 private _tokenIdCounter;
constructor() ERC721("MyNFT", "MNFT") {}
function mintNFT(address to) public onlyOwner {
_tokenIdCounter++;
_mint(to, _tokenIdCounter);
}
}
Step 3: Compile & Deploy the Contract
- Compile the contract using Hardhat or Remix
- Deploy to Ethereum (Goerli Testnet or Mainnet) using Infura or Alchemy
- Verify deployment using Etherscan
Step 4: Integrate Metadata and Mint NFTs
- Use IPFS or Arweave for decentralized storage.
- Define metadata in JSON format:
{
"name": "My Unique NFT",
"description": "This is an exclusive digital collectible.",
"image": "ipfs://Qm..."
}
Advanced NFT Smart Contract Features
1. Royalties and Revenue Sharing
To ensure artists and creators earn from secondary sales, ERC-2981 standard allows royalty fees to be automatically distributed.
contract MyNFTWithRoyalties is ERC721, ERC2981 {
constructor() ERC721("MyNFT", "MNFT") {}
function setRoyaltyInfo(address receiver, uint96 feeNumerator) external onlyOwner {
_setDefaultRoyalty(receiver, feeNumerator);
}
}
2. Fractionalized NFTs
Allows multiple people to own fractions of a high-value NFT by splitting ownership into ERC-20 tokens.
3. Staking Mechanisms
Owners can stake their NFTs to earn rewards or access gated privileges in a decentralized ecosystem.
4. Dynamic NFTs
NFTs that evolve over time based on conditions set within the smart contract (e.g., gaming assets that upgrade based on usage).
Security Considerations in NFT Programming
NFT smart contracts must be secure to prevent hacks and exploits. Key security best practices include:
- Use OpenZeppelin libraries (battle-tested, secure smart contract implementations)
- Avoid reentrancy attacks (Use reentrancy guards when transferring funds)
- Implement access controls (Only allow privileged users to mint or modify contract settings)
- Gas optimization (Minimize high gas fees using efficient contract logic)
FAQs
What makes NFT smart contracts different from regular smart contracts?
- NFT smart contracts follow the ERC-721 or ERC-1155 standard, ensuring unique and non-fungible properties, unlike ERC-20, which represents fungible tokens.
Can I create an NFT without programming?
- Yes, platforms like OpenSea, Rarible, and Mintable allow users to create NFTs without coding. However, custom smart contracts provide more flexibility and control.
How do NFT royalties work?
- Royalty settings in smart contracts ensure that the original creator receives a percentage of sales each time the NFT is resold.
Which blockchain is best for NFT smart contracts?
- Ethereum (Most popular but has high gas fees)
- Solana (Fast and low-cost transactions)
- Polygon (Ethereum-compatible with lower fees)
- Tezos & Flow (Eco-friendly alternatives)
Are NFT smart contracts safe?
- Security depends on how well they are programmed. Following best practices and auditing contracts minimize risks.