Fear of loops and conditionals holding you back from building dApps? Imagine them as tiny switches controlling your smart contracts, like traffic lights guiding every step. Solidity control structures are your tools to make your dApp dance, react, and thrive. This article will tell you everything you need to know about Solidity control structures. Let’s explore how these powerful tools and unlock the potential of Solidity.
Solidity is a unique language made for writing smart contracts on blockchain platforms like Ethereum. These smart contracts can do things like create voting systems or digital wallets. In Solidity, we use something called control structures to guide our smart contracts, just like a map guides a hiker. These control structures help us decide what actions our smart contract takes based on different conditions.
But that’s not all! In Solidity, we also use things called methods and global variables. Methods are the actions our smart contracts can take, like moving a digital token from one person to another. Global variables give our contract important information about the world of the blockchain.
Understanding how control structures, methods, and global variables all work together is like solving a puzzle. Each piece fits together to create a complete picture.
All these parts work together to help you create cool, useful apps on the Ethereum blockchain. Let’s jump in and learn more!
What are Control Structures?
Control structures are the decision-making elements of a programming language. They are like traffic signals, guiding the program’s flow based on certain conditions. They enable you to create dynamic, responsive code that adapts to different scenarios.
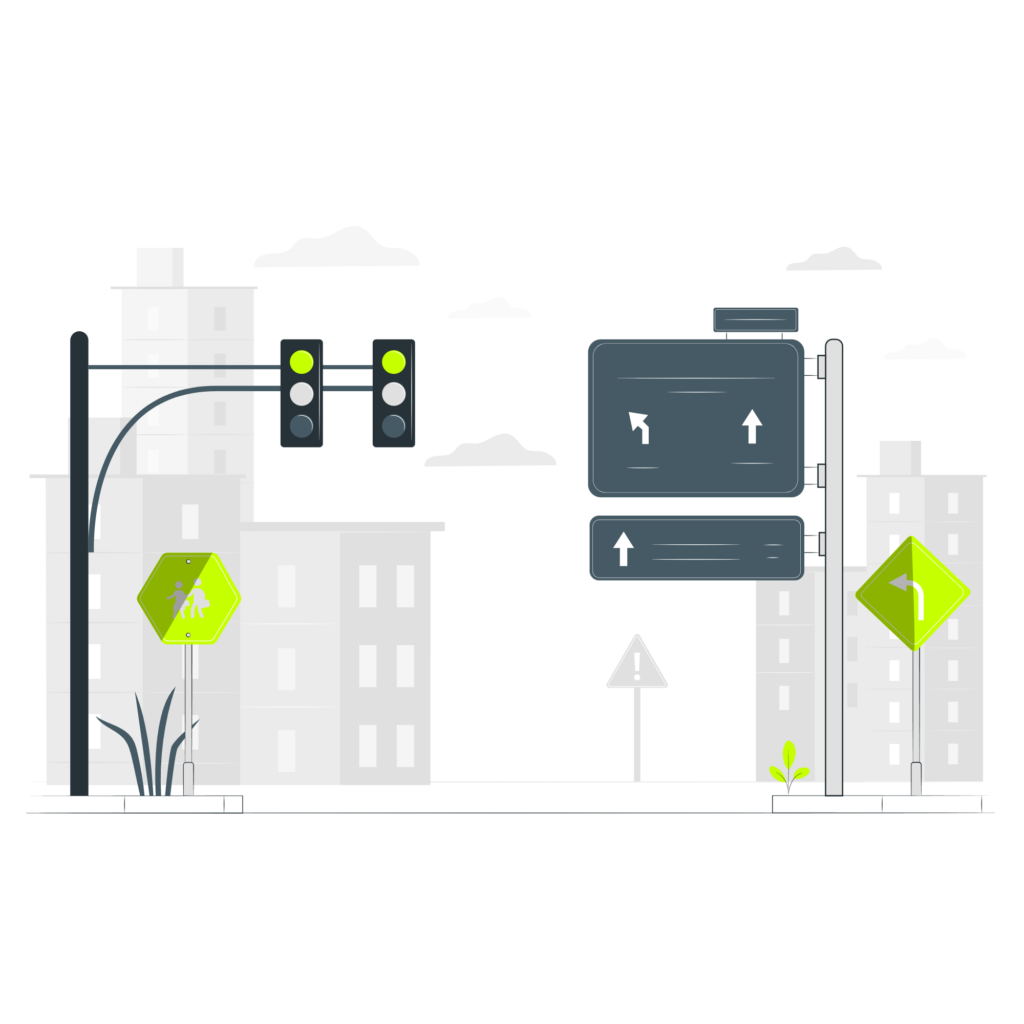
Types of Control Structures in Solidity
Now that we grasp the significance of control structures, let’s dive into the two main types found in Solidity: conditional statements and looping statements.
Conditional Statements
If Statements
Conditional execution is a fundamental aspect of programming. If statements in Solidity allow you to create branches in your code, executing specific blocks only if certain conditions are met.
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Switch Statements
When dealing with multiple possible outcomes, switch statements offer an elegant solution. They simplify complex decision trees, making your code more readable.
uint choice = 2;
switch (choice) {
case 1:
// Code for case 1
break;
case 2:
// Code for case 2
break;
default:
// Code to execute if no case matches
}
Looping Statements
For Loops
For loops are invaluable when you need to iterate through arrays or execute a specific block of code multiple times.
for (uint i = 0; i < 5; i++) {
// Code to execute in each iteration
}
While Loops
While loops continue executing a block of code as long as a specified condition is true.
uint counter = 0;
while (counter < 3) {
// Code to execute as long as the condition is true
counter++;
}
Practical Examples
Let’s put our newfound knowledge into practice by exploring real-world examples of smart contracts that leverage control structures. From basic scenarios to more complex applications, these hands-on examples will solidify your understanding of how to implement logic in Solidity.
Basic Scenario: Voting System
Consider a simple voting system where users can cast their votes for different candidates. We’ll use if statements to check the validity of votes and a for loop to tally the results.
function vote(string memory candidate) public {
require(!hasVoted[msg.sender], "You have already voted.");
// Additional conditions to check the validity of the candidate
voteCount[candidate]++;
hasVoted[msg.sender] = true;
}
function getResult(string memory candidate) public view returns (uint) {
return voteCount[candidate];
}
}
In this simple contract, voters can cast their votes for different candidates, and the contract ensures that each voter can only vote once. The use of control structures guarantees the integrity of the voting process.
Complex Application: Decentralized Autonomous Organization (DAO)
Let’s explore a more complex scenario involving a Decentralized Autonomous Organization (DAO). A DAO is an organization represented by rules encoded as a computer program that is transparent, controlled by organization members, and not influenced by a central government. We’ll use a combination of control structures to manage membership and voting.
contract DAO {
address[] public members;
mapping(address => bool) public isMember;
mapping(address => uint) public votingPower;
function joinDAO() public {
require(!isMember[msg.sender], "You are already a member.");
// Additional conditions for joining the DAO
// ...
members.push(msg.sender);
isMember[msg.sender] = true;
votingPower[msg.sender] = 1;
}
function voteProposal(uint proposalId) public {
require(isMember[msg.sender], "You are not a member of the DAO.");
// Additional conditions for voting on a proposal
// ...
// Update proposal voting results
// ...
}
// Additional functions for proposing and executing actions within the DAO
// ...
}
In this DAO contract, control structures are employed to ensure that only eligible members can join, vote
on proposals, and execute actions. The use of control structures is essential in managing the complex interactions within a decentralized organization.
Best Practices and Common Pitfalls
Now that we’ve explored the implementation of control structures, let’s shift our focus to best practices and common pitfalls associated with their usage in Solidity.
Writing Efficient Control Structures
Efficiency in blockchain development is paramount. Gas costs, which represent the computational effort required to execute operations on the Ethereum network, can accumulate quickly. Here are some tips for writing efficient control structures in Solidity:
Minimize Gas Usage
Each operation in your control structure consumes gas. Be mindful of the gas cost of individual operations, and strive to minimize unnecessary computations.
Limit Loop Iterations
Excessive loop iterations can lead to high gas costs. Consider ways to optimize loops, and avoid scenarios where the number of iterations is unpredictable.
Use View and Pure Functions
If your control structure doesn’t modify the blockchain state, declare your functions as view or pure. View functions are read-only and don’t modify the state, while pure functions don’t read from or modify the state.
Avoiding Common Mistakes
Solidity, like any programming language, has its quirks and potential pitfalls. Here are some common mistakes to avoid when working with control structures:
Reentrancy Vulnerability
Reentrancy occurs when an external call is made from within a contract before the current execution is complete. This can lead to unexpected behavior. Use checks-effects-interactions pattern to minimize reentrancy risks.
Integer Overflow and Underflow
Solidity doesn’t automatically check for integer overflow and underflow, which can lead to unexpected results. Always include checks to ensure that arithmetic operations won’t exceed the limits of the variable type.
Lack of Access Control
Ensure that your control structures include proper access controls. Failing to restrict access to certain functions can result in unauthorized modifications to your contract’s state.
Advanced Concepts
With a solid grasp of the basics, let’s venture into more advanced concepts related to control structures in Solidity.
Nested Control Structures
Sometimes, a single control structure isn’t sufficient to address complex scenarios. That’s where nested control structures come into play. By placing one control structure inside another, you can create intricate decision trees.
function complexFunction(uint x, uint y) public pure returns (string memory) {
if (x > 0) {
if (y > 0) {
return "Both x and y are positive.";
} else {
return "Only x is positive.";
}
} else {
return "Both x and y are non-positive.";
}
}
In this example, the function contains nested if statements to cover various combinations of x and y values.
Exception Handling
Exception handling is crucial in Solidity to gracefully handle errors and ensure the robustness of your smart contracts. Solidity provides mechanisms like try-catch and revert statements for effective exception handling.
function safeTransfer(address to, uint amount) public {
require(balance[msg.sender] >= amount, "Insufficient balance.");
// Use try-catch to handle potential exceptions
try myToken.transfer(to, amount) {
// Code to execute on successful transfer
} catch Error(string memory errorMessage) {
// Handle specific error and revert with a custom message
revert(errorMessage);
} catch (bytes memory) {
// Handle other errors and revert with a generic message
revert("Token transfer failed.");
}
}
In this example, the function attempts to transfer tokens and uses try-catch to handle potential errors, providing a more controlled and secure way to manage exceptions.
Solidity Control Structures in Action
Let’s shift our focus to real-world scenarios where Solidity control structures play a pivotal role in shaping the functionality of smart contracts.
Case Studies
Decentralized Finance (DeFi) Lending Platform
Consider a DeFi lending platform where users can borrow and lend assets. Control structures are crucial in managing loan requests, collateral, interest rates, and liquidation scenarios.
// Simplified example
contract LendingPlatform {
mapping(address => uint) public loanAmount;
mapping(address => uint) public collateral;
function borrow(uint amount) public {
require(collateral[msg.sender] >= amount, "Insufficient collateral.");
// Additional conditions for borrowing
// ...
loanAmount[msg.sender] = amount;
}
function repay() public {
// Repayment logic
// ...
}
}
In this lending platform, control structures ensure that borrowers meet specific conditions before obtaining a loan, and collateral is used as a protective measure.
Non-Fungible Token (NFT) Marketplace
Imagine a marketplace for trading NFTs, where control structures govern the creation, transfer, and auction of unique digital assets.
// Simplified example
contract NFTMarketplace {
mapping(uint => address) public owner;
mapping(uint => bool) public isListed;
function createNFT() public {
// NFT creation logic
// ...
}
function listNFT(uint tokenId, uint price) public {
require(owner[tokenId] == msg.sender, "You don't own this NFT.");
require(!isListed[tokenId], "NFT is already listed.");
// Additional conditions for listing
// ...
// Set the NFT as listed with the specified price
isListed[tokenId] = true;
// ...
}
function purchaseNFT(uint tokenId) public payable {
require(isListed[tokenId], "NFT is not listed.");
// Additional conditions for purchasing
// ...
// Transfer ownership of the NFT and handle payments
// ...
}
}
In this NFT marketplace, control structures ensure that NFTs are created by the rightful owner, listed with appropriate conditions, and purchased securely.
Success Stories
Uniswap: Decentralized Exchange Protocol
Uniswap, a decentralized exchange (DEX) protocol, is a prime example of how control structures can revolutionize the world of decentralized finance. Uniswap employs automated market makers (AMMs) and sophisticated control structures to enable users to swap various ERC-20 tokens seamlessly.
Control structures within Uniswap manage liquidity pools, token swaps, and rewards distribution. By implementing dynamic algorithms and intelligent decision-making processes, Uniswap has become a cornerstone in the DeFi ecosystem.
CryptoKitties: Blockchain Collectibles
CryptoKitties, a blockchain-based game that allows users to collect, breed, and trade virtual cats, showcases the creative potential of control structures in the realm of blockchain-based applications.
The game employs control structures to manage the creation of unique CryptoKitties, determine breeding outcomes, and enforce scarcity. Through these control structures, CryptoKitties has created a captivating and decentralized virtual pet ecosystem.
Certainly! Let’s continue with Section 9 on “Tips for Optimizing Control Structures.”
Tips for Optimizing Control Structures
Efficient control structures are crucial for the success of your smart contracts. Optimizing control structures not only ensures that your contracts consume less gas but also enhances their overall performance and responsiveness. Let’s explore some tips for optimizing your control structures in Solidity.
Gas Efficiency
Minimize External Calls
External calls to other contracts come with a cost. When designing your smart contracts, carefully consider the necessity of external calls and aim to minimize them whenever possible.
// Inefficient
function makeExternalCall(address externalContract) public {
externalContract.someFunction();
}
// Efficient
function makeInternalCall() internal {
// Perform necessary logic without external calls
}
By minimizing external calls, you reduce the gas costs associated with interacting with other contracts on the Ethereum blockchain.
Batch External Calls
If your smart contract requires multiple external calls, consider batching them to reduce the overall gas costs. This involves consolidating multiple calls into a single transaction.
// Inefficient
function multipleExternalCalls(address[] calldata externalContracts) public {
for (uint i = 0; i < externalContracts.length; i++) {
externalContracts[i].someFunction();
}
}
// Efficient
function batchExternalCalls(address[] calldata externalContracts) public {
for (uint i = 0; i < externalContracts.length; i++) {
// Accumulate function calls and execute in a single external call
}
}
Batching external calls helps optimize gas usage by minimizing the overhead associated with individual transactions.
Code Readability
Favor Clarity Over Conciseness
While concise code is often desirable, prioritize clarity over brevity. Clearly written code is easier to understand, maintain, and audit.
// Less clear
function unclearFunction(uint x) public pure returns (uint) {
return x > 0 ? x : 0;
}
// Clearer
function clearerFunction(uint x) public pure returns (uint) {
if (x > 0) {
return x;
} else {
return 0;
}
}
In the example above, the clearer version of the function is preferred for its readability, even though it may be slightly longer.
Use Helper Functions
Breaking down complex logic into smaller, well-named helper functions can significantly improve code readability. This approach makes it easier to understand the purpose of each part of your smart contract.
// Less readable
function processComplexLogic(uint a, uint b) internal pure returns (uint) {
// Complex logic here
}
// More readable
function calculateSomething(uint a, uint b) internal pure returns (uint) {
return processComplexLogic(a, b);
}
By using helper functions, you create a modular structure that enhances code organization and comprehension.
Error Handling
Utilize Require and Revert
Error handling is an essential aspect of writing robust smart contracts. The `require` statement is commonly used for input validation and to ensure certain conditions are met before proceeding.
// Inefficient error handling
function inefficientErrorHandling(uint x) public {
if (x == 0) {
// Handle error
} else {
// Continue with execution
}
}
// Efficient error handling
function efficientErrorHandling(uint x) public {
require(x != 0, "Input must be nonzero");
// Continue with execution
}
Using `require` not only makes your code more readable but also ensures that the conditions are met before any gas is consumed.
Loop Optimization
Avoiding Infinite Loops
Infinite loops can lead to high gas consumption and are generally undesirable. Always ensure that your loops have exit conditions to prevent unintended behavior.
// Infinite loop (bad)
function infiniteLoop() public {
while (true) {
// Do something
}
}
// Finite loop (good)
function finiteLoop(uint iterations) public {
for (uint i = 0; i < iterations; i++) {
// Do something
}
}
By using finite loops with well-defined exit conditions, you prevent the risk of consuming excessive gas and potentially rendering your smart contract unusable.
Control Structure Complexity
Simplify Nested Control Structures
While nested control structures provide flexibility, overly complex structures can hinder readability. Aim to simplify your control structures to make your code more understandable.
// Complex nested structure
function complexNestedStructure(uint x, uint y) public pure returns (uint) {
if (x > 0) {
if (y > 0) {
return x + y;
} else {
return x - y;
}
} else {
return 0;
}
}
// Simplified structure
function simplifiedStructure(uint x, uint y) public pure returns (uint) {
if (x <= 0) {
return 0;
}
if (y > 0) {
return x + y;
} else {
return x - y;
}
}
Simplifying nested structures improves code readability and reduces the risk of logic errors.
Future Trends and Developments
As the blockchain space continues to evolve, so does Solidity. Let’s explore the future trends and developments that may shape the landscape of Solidity control structures and smart contract development.
Integration with Layer 2 Solutions
Layer 2 solutions aim to address scalability issues on the Ethereum blockchain by moving some transactions off the main chain. As these solutions become more widely adopted, Solidity control structures may need to adapt to the unique challenges and opportunities presented by Layer 2 environments.
Developers may explore new patterns and best practices to optimize control structures for Layer 2 interactions, ensuring efficient and secure smart contract execution.
Enhanced Security Measures
Security is a top priority in blockchain development, and Solidity is no exception. Future versions of Solidity may introduce enhanced security measures and features to mitigate potential vulnerabilities in control structures.
Developers should stay informed about security best practices and regularly update their knowledge as new security features are introduced in Solidity.
Continued Language Improvements
The Solidity language will likely undergo continuous improvements to enhance its expressiveness and developer experience. Future updates may introduce syntactic sugar, additional control structures, and other language features that make smart contract development more efficient and enjoyable.
Developers should stay engaged with the Solidity community to keep abreast of language updates and leverage new features to enhance their smart contracts.
Conclusion
To wrap up, understanding control structures in Solidity is key for anyone diving into blockchain development. In Solidity, we use simple if
, else
statements for making choices, and loops like for
and while
to repeat actions. These tools are essential for telling our smart contracts how to act under different circumstances. But remember, while these controls are powerful, they need to be used carefully. Using them the right way helps avoid common problems, like overusing resources or security issues. As the world of Ethereum and blockchain keeps growing, knowing how to use these structures effectively is super important for any developer looking to make their mark in this exciting field.
Happy coding, and may your Solidity journey be both rewarding and transformative.
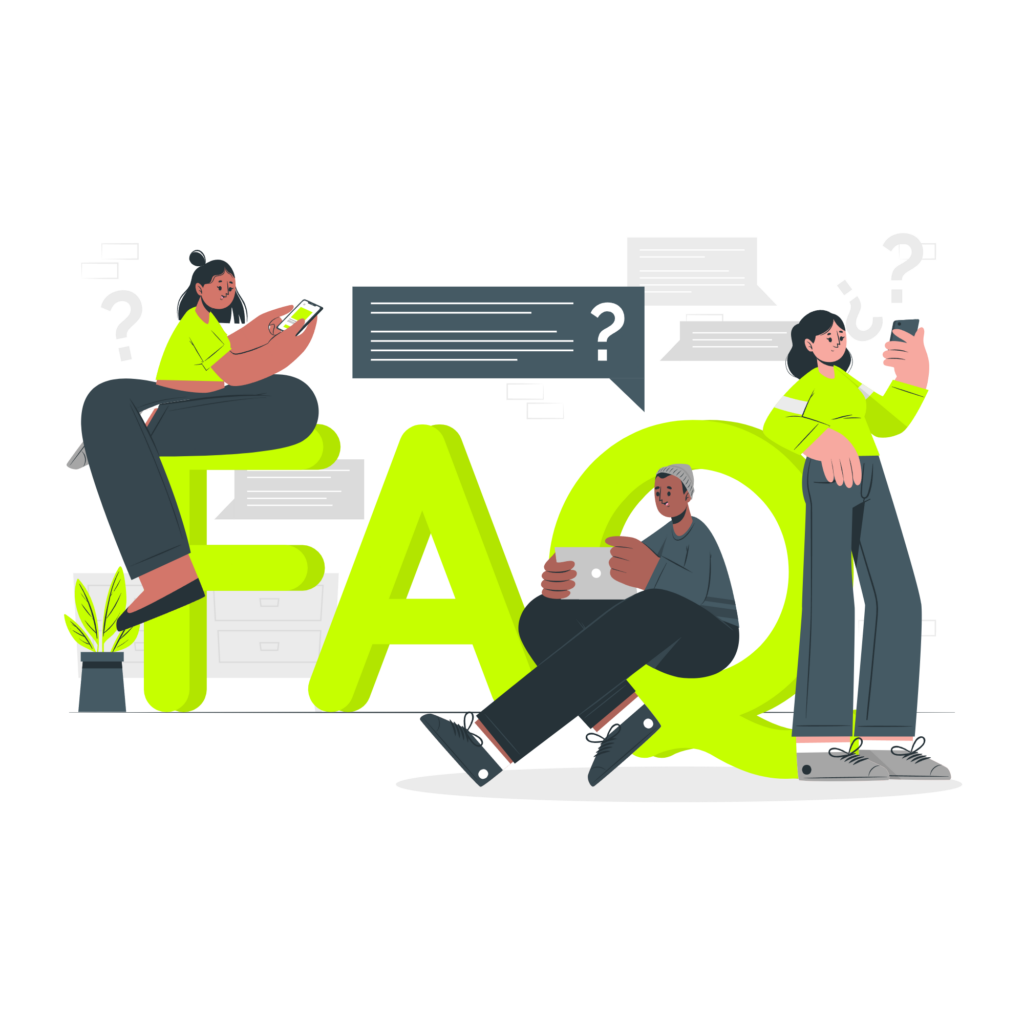
What are the basic control structures available in Solidity?
- Control structures in Solidity include conditional statements like
if
,else
, andelse if
, as well as loop constructs such asfor
,while
, anddo while
. These structures are fundamental for directing the flow of execution in a smart contract.
How does the if-else
statement work in Solidity?
- The
if-else
statement in Solidity works similarly to other programming languages. It allows the execution of certain code blocks based on a condition. If the condition is true, theif
block executes; otherwise, theelse
block executes.
Can I use a switch
case in Solidity?
- Solidity does not currently support
switch
case statements. Developers typically use multipleif
,else if
, andelse
statements to achieve similar functionality.
Are loops in Solidity gas efficient?
- Using loops in Solidity can be gas-intensive, especially if the number of iterations is high or unpredictable. It’s important to optimize loops to prevent excessive gas consumption and potential out-of-gas errors.
How can I break out of a loop in Solidity?
- You can use the
break
statement to exit a loop prematurely in Solidity. This is particularly useful when a certain condition is met, and further iteration is unnecessary or undesirable.
What is gas optimization in Solidity and why is it important?
- Gas optimization in Solidity involves writing code that uses the least amount of gas possible. It’s crucial because users pay gas fees for transactions and contract executions on the Ethereum network. Efficient code reduces costs and enhances performance.
How do smart contracts handle exceptions in Solidity?
- Solidity handles exceptions using
require
,revert
, andassert
functions. These functions provide ways to handle errors and revert transactions if certain conditions are not met.
What is the difference between a view
and pure
function in Solidity?
- A
view
function declares that no state will be changed, while apure
function indicates that it neither reads nor alters the state. Both are read-only but differ in their interaction with the blockchain state.
How does inheritance work in Solidity?
- Solidity supports inheritance, allowing one contract to inherit properties and functions of another. This feature facilitates code reuse and organization in smart contract development.
What are modifiers in Solidity, and how are they used?
- Modifiers are code snippets in Solidity that can be used to change the behavior of functions in a declarative way. They’re often used for precondition checks, such as verifying if a caller is authorized to execute a function.